SAF_EXIT APIs
This chapter describes the header file and APIs that support the user-exit function.
1. Overview
TACF provides a plugin SAF_EXIT module that allows to create customized APIs. Internal modules of TACF do not need to be modified because TACF internally calls the API when processing customer applications.
The following function replaces a customer’s function name with a TACF function name.
/* -------------------------- compatible API switch ------------------------- */ #define SAF_EXIT_IMPL_NAME_LEN 31 typedef struct { /* identification */ char saf_exit_impl_name[SAF_EXIT_IMPL_NAME_LEN + 1]; int saf_exit_version; /* group management */ int (*saf_exit_add_group_entry)(char *aceeusri, char *groupname, char *owner, char *supgroup); int (*saf_exit_alter_group_entry)(char *aceeusri, char *groupname, char *owner, char *supgroup); int (*saf_exit_delete_group_entry)(char *aceeusri, char *groupname); /* user management */ int (*saf_exit_add_user_entry)(char *aceeusri, char *userid, char *owner, char *dfltgrp); int (*saf_exit_alter_user_entry)(char *aceeusri, char *userid, char *owner, char *dfltgrp); int (*saf_exit_delete_user_entry)(char *aceeusri, char *userid); /* connect & remove */ int (*saf_exit_connect_entry)(char *aceeusri, char *userid, char *groupname, char *owner); int (*saf_exit_remove_entry)(char *aceeusri, char *userid, char *groupname, char *owner); /* password check */ int (*saf_exit_password_entry)(char *userid, char *password, int count, char *history[]); /* dataset management */ int (*saf_exit_add_dsd_entry)(char *aceeusri, char *profname, char *owner, char *notify); int (*saf_exit_alter_dsd_entry)(char *aceeusri, char *profname, char *owner, char *notify); int (*saf_exit_delete_dsd_entry)(char *aceeusri, char *profname); /* resource management */ int (*saf_exit_define_resource_entry)(char *aceeusri, char *classname, char *profname, char *owner, char *notify); int (*saf_exit_alter_resource_entry)(char *aceeusri, char *classname, char *profname, char *owner, char *notify); int (*saf_exit_delete_resource_entry)(char *aceeusri, char *classname, char *profname); /* permission management */ int (*saf_exit_permit_access_entry)(char *aceeusri, char *classname, char *profname, char *access, char *id, char *reset); int (*saf_exit_permit_delete_entry)(char *aceeusri, char *classname, char *profname, char *id, char *reset); } saf_exit_switch_t;
The following tables include APIs of the header file. Refer to each function in the tables for more information.
-
Group API
Function Description Adds a customer-specific rule that adds a new group to TACF.
Adds a customer-specific rule that changes a group in TACF.
Adds a customer-specific rule that deletes a group from TACF.
-
User API
Function Description Adds a customer-specific rule that adds a new user to TACF.
Adds a customer-specific rule that changes a user in TACF.
Adds a customer-specific rule that deletes a user from TACF.
-
Connection API
Function Description Adds a customer-specific rule that sets a user in a connection group.
Adds a customer-specific rule that removes a user from a connection group.
-
Password API
Function Description Adds a customer-specific rule that sets or changes user passwords.
-
Data Set API
Function Description Adds a customer-specific rule that adds a new discrete or generic data set profile to TACF.
Adds a customer-specific rule that changes a discrete or generic data set profile to TACF.
Adds a customer-specific rule that deletes a discrete or generic data set profile from TACF.
-
Resource API
Function Description Adds a customer-specific rule that defines a resource profile.
Adds a customer-specific rule that changes a resource profile.
Adds a customer-specific rule that deletes a resource profile.
-
Permission API
Function Description Adds a customer-specific rule that grants a resource permission to a user or group.
Adds a customer-specific rule that removes a resource permission of a user or group.
2. GROUP API
Adds customer-specific rules that manage groups in TACF. The rules are checked before using the GROUP API.
2.1. saf_exit_add_group
Adds a customer-specific rule that adds a new group to TACF.
The following figure shows when the function is called.
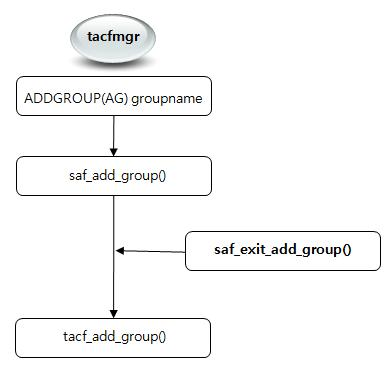
-
Prototype
saf_exit_add_group(aceeusri, groupname, owner, supgroup) (*(saf_exit_sw.saf_exit_add_group_entry))(aceeusri, groupname, owner, supgroup)
-
Parameters
Parameter Description aceeusri
User ID of the current user.
groupname
Name of the group to be added.
owner
Owner of the group to be added. The owner is either the user ID or the group name.
supgroup
Superior group of the group to be added.
2.2. saf_exit_alter_group
Adds a customer-specific rule that changes a group in TACF.
The following figure shows when the function is called.
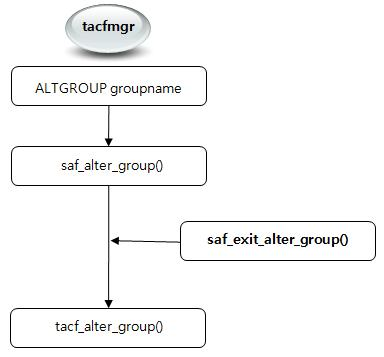
-
Prototype
saf_exit_alter_group(aceeusri, groupname, owner, supgroup) (*(saf_exit_sw.saf_exit_alter_group_entry))(aceeusri, groupname, owner, supgroup)
-
Parameters
Parameter Description aceeusri
User ID of the current user.
groupname
Name of the group to be changed.
owner
User ID or group name of the owner or group to be changed.
supgroup
Superior group of the group to be changed.
2.3. saf_exit_delete_group
Adds a customer-specific rule that deletes a group from TACF.
The following figure shows when the function is called.
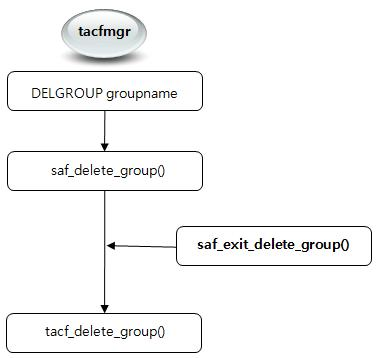
-
Prototype
saf_exit_delete_group(aceeusri, groupname) (*(saf_exit_sw.saf_exit_delete_group_entry))(aceeusri, groupname)
-
Parameters
Parameter Description aceeusri
User ID of the current user.
groupname
Name of the group to be deleted.
3. USER API
Adds customer-specific rules that manage users in TACF.
3.1. saf_exit_add_user
Adds a customer-specific rule that adds a new user to TACF.
The following figure shows when the function is called.
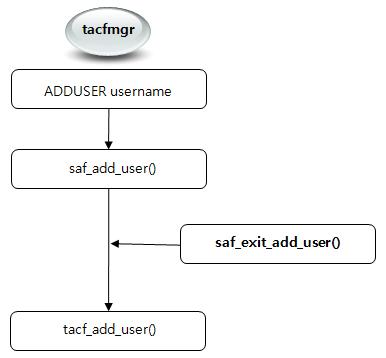
-
Prototype
saf_exit_add_user(aceeusri, userid, owner, dfltgrp) (*(saf_exit_sw.saf_exit_add_user_entry))(aceeusri, userid, owner, dfltgrp)
-
Parameters
Parameter Description aceeusri
User ID of the current user.
userid
User ID of the new user to be added.
owner
User ID or group name of the owner of the user profile to be added.
dfltgrp
Default group of the user to be added.
3.2. saf_exit_alter_user
Adds a customer-specific rule that changes a user in TACF. The rule is checked before changing a user profile in TACF.
The following figure shows when the function is called.
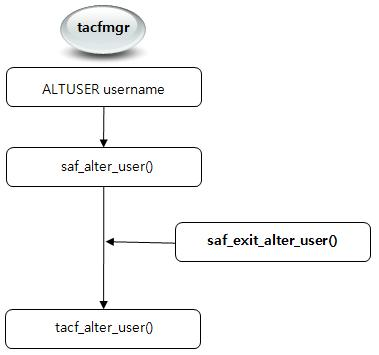
-
Prototype
saf_exit_alter_user(aceeusri, userid, owner, dfltgrp) (*(saf_exit_sw.saf_exit_alter_user_entry))(aceeusri, userid, owner, dfltgrp)
-
Parameters
Parameter Description aceeusri
User ID of the current user.
userid
User ID of the user to be changed.
owner
User ID or group name of the owner of the user profile to be changed.
dfltgrp
Default group of the user to be changed.
3.3. saf_exit_delete_user
Adds a customer-specific rule that deletes a user from TACF.
The following figure shows when the function is called.
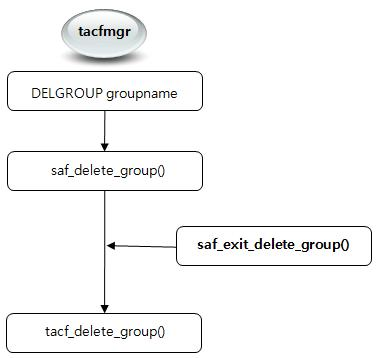
-
Prototype
saf_exit_add_user(acee, userid, owner, dfltgrp) (*(saf_exit_sw.saf_exit_add_user_entry))(aceeusri, userid, owner, dfltgrp)
-
Parameters
Parameter Description aceeusri
User ID of the current user.
userid
User ID of the user to be deleted.
4. CONNECTION API
Adds customer-specific rules that manage user connection groups.
4.1. saf_exit_connect
Adds a customer-specific rule that sets a user in a connection group. The rule is checked when setting a user in the connection group.
The following figure shows when the function is called.
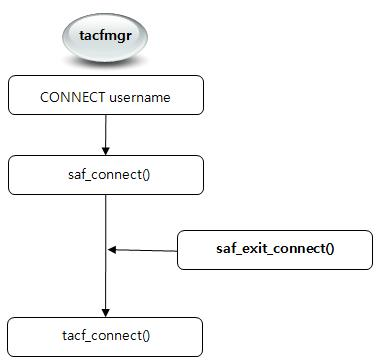
-
Prototype
saf_exit_connect(aceeusri, userid, groupname, owner) (*(saf_exit_sw.saf_exit_connect_entry))(aceeusri, userid, groupname, owner)
-
Parameters
Parameter Description aceeusri
User ID of the current user.
userid
User ID of the user to be connected to a group.
groupname
Name of the group to be connected to a user.
owner
User ID or group name of the owner of the group profile to be connected to a user.
4.2. saf_exit_remove
Adds a customer-specific rule that removes a user from a connection group. The rule is checked when removing a user from the connection group.
The following figure shows when the function is called.
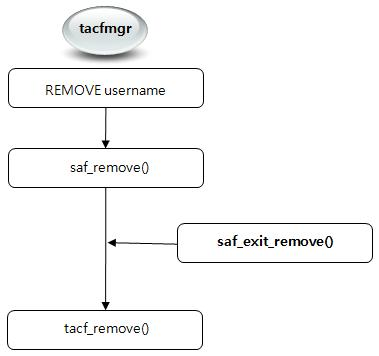
-
Prototype
saf_exit_remove(aceeusri, userid, groupname, owner) (*(saf_exit_sw.saf_exit_remove_entry))(aceeusri, userid, groupname, owner)
-
Parameters
Parameter Description aceeusri
User ID of the current user.
userid
User ID of the user to be disconnected from a group.
groupname
Name of the group to be disconnected from a user.
owner
User ID or group name of the owner of the group profile to be disconnected from a user.
5. PASSWORD API
Adds customer-specific rules that manage user passwords.
5.1. saf_exit_password
Adds a customer rule that sets or changes user passwords. The rule is checked when setting a user password in TACF.
The following figure shows when the function is called. It is called through the PASSWORD and ALTUSER commands of tacfmgr and when authenticating users.
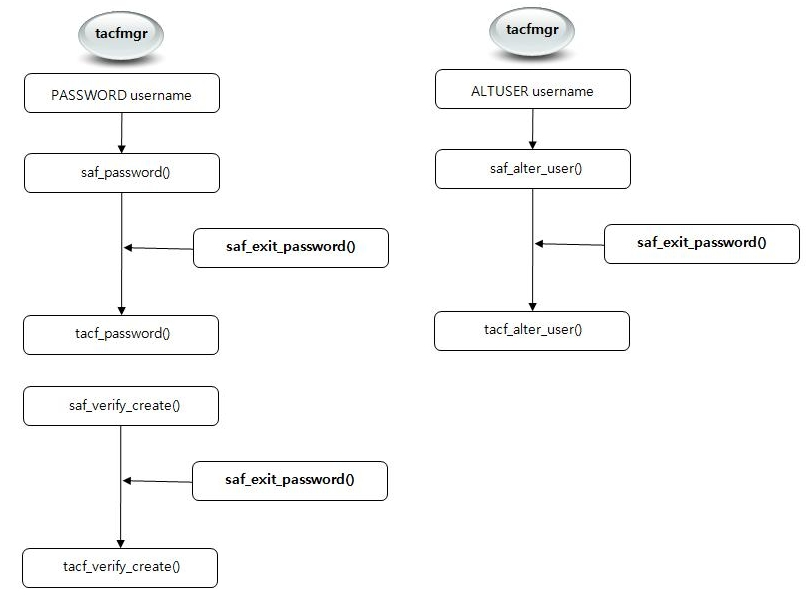
-
Prototype
saf_exit_password(userid, password, count, history) (*(saf_exit_sw.saf_exit_password_entry))(userid, password, count, history)
-
Parameters
Parameter Description userid
User ID of the current user.
password
New password.
count
Number of changed passwords.
history
History of the previous passwords.
6. DATA SET API
Adds customer-specific rules that manage discrete or generic data set profiles in TACF.
6.1. saf_exit_add_dsd
Adds a customer-specific rule that adds a new discrete or generic data set profile to TACF. The rule is checked when adding a new data set profile to TACF.
The following figure shows when the function is called.
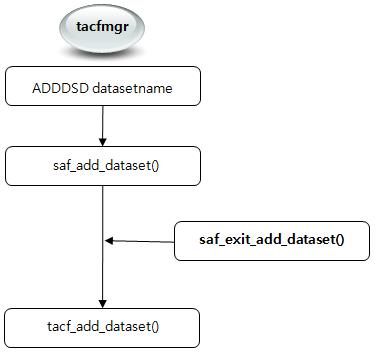
-
Prototype
saf_exit_add_dsd(aceeusri, profname, owner, notify) (*(saf_exit_sw.saf_exit_add_dsd_entry))(aceeusri, profname, owner, notify)
-
Parameters
Parameter Description aceeusri
User ID of the current user.
profname
Name of the data set profile to be added.
owner
User ID or group name of the owner of the data set profile to be added.
notify
User ID of the user who notifies of denial of access to the data set.
6.2. saf_exit_alter_dsd
Adds a customer-specific rule that changes a discrete or generic data set profile in TACF. The rule is checked when changing a new data set profile in TACF.
The following figure shows when the function is called.
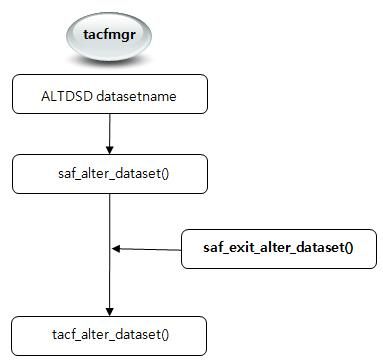
-
Prototype
saf_exit_alter_dsd(aceeusri, profname, owner, notify) (*(saf_exit_sw.saf_exit_alter_dsd_entry))(aceeusri, profname, owner, notify)
-
Parameters
Parameter Description aceeusri
User ID of the current user.
profname
Name of the data set profile to be modified.
owner
User ID or group name of the owner of the data set profile to be modified.
notify
User ID of the user who notifies of denial of access to the data set.
6.3. saf_exit_delete_dsd
Adds a customer-specific rule that deletes a discrete or generic data set profile from TACF. The rule is checked when deleting a new data set profile from TACF.
The following figure shows when the function is called.
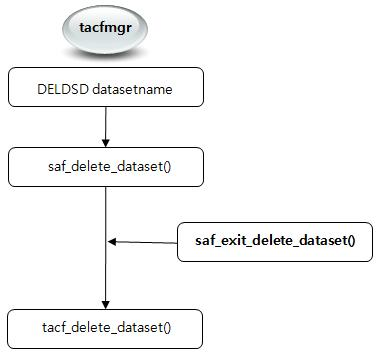
-
Prototype
saf_exit_delete_dsd(aceeusri, profname) (*(saf_exit_sw.saf_exit_delete_dsd_entry))(aceeusri, profname)
-
Parameters
Parameter Description aceeusri
User ID of the current user.
groupname
Name of the data set profile to be deleted.
7. RESOURCE API
Adds customer-specific rules that manage resource profiles.
7.1. saf_exit_define_resource
Adds a customer-specific that defines a resource profile. The rule is checked when adding a new resource profile to TACF.
The following figure shows when the function is called.
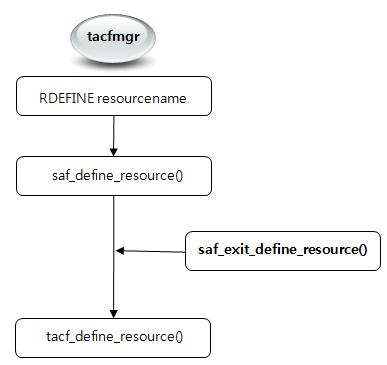
-
Prototype
saf_exit_define_resource(aceeusri, classname, profname, owner, notify) (*(saf_exit_sw.saf_exit_define_resource_entry))(aceeusri, classname, profname, owner, notify)
-
Parameters
Parameter Description aceeusri
User ID of the current user.
classname
Class name of the resource profile to be added.
owner
User ID or group name of the owner of the resource profile to be added.
notify
User ID of the user who notifies of denial of access to the resource.
7.2. saf_exit_alter_resource
Adds a customer-specific rule that changes a resource profile. The rule is checked when changing a resource profile in TACF.
The following figure shows when the function is called.
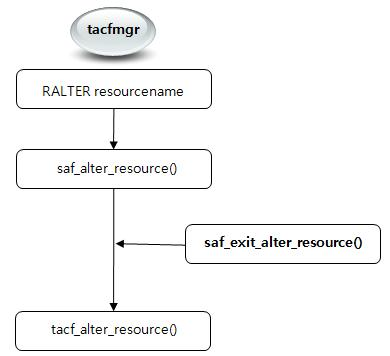
-
Prototype
saf_exit_alter_resource(aceeusri, classname, profname, owner, notify) (*(saf_exit_sw.saf_exit_alter_resource_entry))(aceeusri, classname, profname, owner, notify)
-
Parameters
Parameter Description aceeusri
User ID of the current user.
classname
Class name of the resource profile to be modified.
owner
User ID or group name of the owner of the resource profile to be modified.
notify
User ID of the user who notifies of denial of access to the resource.
7.3. saf_exit_delete_resource
Adds a customer-specific rule that deletes a resource profile. The rule is checked when deleting a resource profile from TACF.
The following figure shows when the function is called.
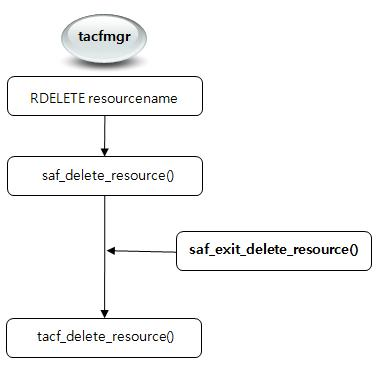
-
Prototype
saf_exit_delete_resource(aceeusri, classname, profname) (*(saf_exit_sw.saf_exit_delete_resource_entry))(aceeusri, classname, profname)
-
Parameters
Parameter Description aceeusri
User ID of the current user.
classname
Class name of the resource profile to be deleted.
profname
Name of the resource profile to be deleted.
8. PERMISSION API
Adds customer-specific rules that manage resource permissions of users and groups.
8.1. saf_exit_permit_access
Adds a customer-specific rule that grants a resource permission to a user or group. The rule is checked when granting a resource permission to a user or group.
The following figure shows when the function is called.
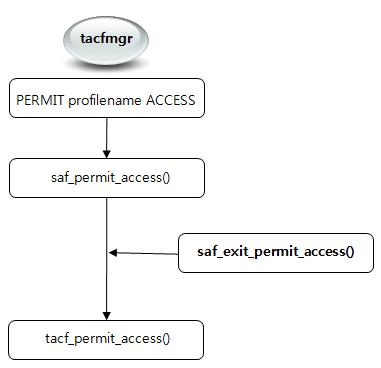
-
Prototype
saf_exit_permit_access(aceeusri, classname, profname, access, id, reset) (*(saf_exit_sw.saf_exit_permit_access_entry))(aceeusri, classname, profname, access, id, reset)
-
Parameters
Parameter Description aceeusri
User ID of the current user.
classname
Class name of the resource for which to grant permission.
profname
Profile name of resource for which to grand permission.
access
Access permission level
id
User ID of the user to be granted access permission.
reset
Level to remove from the access list of the profile.
8.2. saf_exit_permit_delete
Adds a customer-specific rule that removes a resource permission of a user or group. The rule is checked when removing a resource permission of a user or group.
The following figure shows when the function is called.
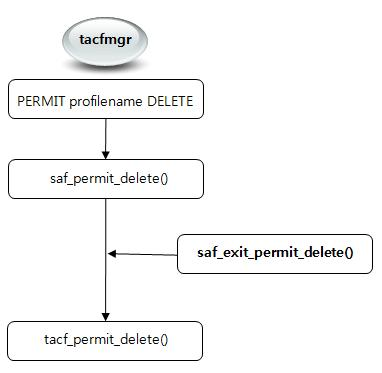
-
Prototype
saf_exit_permit_delete(aceeusri, classname, profname, id, reset) (*(saf_exit_sw.saf_exit_permit_delete_entry))(aceeusri, classname, profname, id, reset)
-
Parameters
Parameter Description aceeusri
User ID of the current user.
classname
Class name of the resource for which to remove permission.
profname
Profile name of the resource for which to remove permission.
id
User ID of the user who loses access permission.
reset
Level to remove from the access list of the profile.
9. Sample Code
The following is an example of sat_exit.c in which only the password is created according to a user-defined rule.
<saf_exit.c>
#include <stdio.h> #include <stdlib.h> #include <string.h> #include "saf_exit.h" /* group management */ /* Can use customer name. */ int customer_saf_exit_add_group(char *aceeusri, char *groupname, char *owner, char *supgroup); int customer_saf_exit_alter_group(char *aceeusri, char *groupname, char *owner, char *supgroup); int customer_saf_exit_delete_group(char *aceeusri, char *groupname); ... (omitted) /* user management, connect & remove are omitted. */ ... /* password check */ int customer_saf_exit_password(char *userid, char *password, int count, char *history[]); ... (omitted) /* dataset management, resource management are removed as well. */ ... /* permission management */ int customer_saf_exit_permit_access(char *aceeusri, char *classname, char *profname, char *access, char *id, char *reset); int customer_saf_exit_permit_delete(char *aceeusri, char *classname, char *profname, char *id, char *reset); /* compatible API switch */ saf_exit_switch_t saf_exit_sw = { /* identification */ "CUSTOMER SAF EXIT", 1, /* group management */ customer_saf_exit_add_group, customer_saf_exit_alter_group, customer_saf_exit_delete_group, /* user management */ customer_saf_exit_add_user, customer_saf_exit_alter_user, customer_saf_exit_delete_user, /* connect & remove */ customer_saf_exit_connect, customer_saf_exit_remove, /* password check */ customer_saf_exit_password, /* dataset management */ customer_saf_exit_add_dsd, customer_saf_exit_alter_dsd, customer_saf_exit_delete_dsd, /* resource management */ customer_saf_exit_define_resource, customer_saf_exit_alter_resource, customer_saf_exit_delete_resource, /* permission management */ customer_saf_exit_permit_access, customer_saf_exit_permit_delete, }; /******************************************************************************/ /* group management */ /******************************************************************************/ int customer_saf_exit_add_group(char *aceeusri, char *groupname, char *owner, char *supgroup) { return 0; } int customer_saf_exit_alter_group(char *aceeusri, char *groupname, char *owner, char *supgroup) { return 0; } int customer_saf_exit_delete_group(char *aceeusri, char *groupname) { return 0; } ... (omitted) ... /******************************************************************************/ /* password check */ /******************************************************************************/ int customer_saf_exit_password(char *userid, char *password, int count, char *history[]) { int i; /* check if password length is greater than 4 */ /* Password must contain 4 or more characters. */ /* If failed to conform to the user rule, -1 is returned. */ if( strlen(password) <= 4 ) return -1; /* check if password is not same as userid */ /* Password cannot be the same as the user ID. */ if( ! strcmp(password, userid) ) return -1; /* check if password is not used before */ /* Check if the password had been already used. */ for( i = 0; i < count; i++ ) { if( ! strcmp(password, history[i]) ) return -1; } /* return success code */ /* If all user rules are satisfied, 0 is returned. */ return 0; } ... (omitted) ... /******************************************************************************/ /* permission management */ /******************************************************************************/ int customer_saf_exit_permit_access(char *aceeusri, char *classname, char *profname, char *access, char *id, char *reset) { return 0; } int customer_saf_exit_permit_delete(char *aceeusri, char *classname, char *profname, char *id, char *reset) { return 0; }
Note
After compiling saf_exit.c file in the form of a shared object, link it to $OPENFRAME_HOME/lib/libsafexit.sl ($OPENFRAME_HOME/lib/libsafexit.so depending on your machine). The following shows how to link the object.
ln -s libsafexit_openframe.sl libsafexit.sl