DL/I Functions
This chapter describes how to use DL/I functions.
1. DL/I Call
Data Language/I (DL/I) is an interface module called by COBOL or PL/I applications to access a HiDB database. When an application searches or updates data in HiDB, it uses DL/I functions.
The following shows a DL/I call syntax used by COBOL applications.
CALL 'CBLTDLI' USING DLI-function PCB-mask Segment-io-area Segment-search-arguments
CBLTDLI is the interface module used by COBOL programs to call a DL/I function.
The following shows a DL/I call syntax called by COBOL programs using AIB.
CALL 'AERTDLI' USING DLI-function AIB-mask Segment-io-area Segment-search-arguments
AERTDLI is the interface module used by COBOL programs to call a DL/I function using AIB.
The following shows a DL/I call syntax used by PL/I applications.
CALL 'PLITDLI' ( Parameter count DLI-function PCB-mask Segment-io-area Segment-search-arguments )
PLITDLI is the interface module used by PL/I programs to call a DL/I function.
When a program issues a DL/I call, its parameters are validated before they are passed to the function.
The following describes the parameters of the DL/I call. The Parameter count must be specified for PLITDLI.
Parameter | Description |
---|---|
Parameter count |
Number of parameters excluding this parameter. This parameter is used only for a PLITDLI module. |
DLI-function |
DL/I function such as segment search, insert, delete, or update. |
PCB-mask |
Data structure to store the status code and segment name, which are returned by the DL/I call. |
Segment-io-area |
Parameter area used to return data after retrieving a segment, or to send data for inserting or updating a segment to DL/I. |
Segment-search-arguments |
Search criteria to access a segment for selecting or processing. This parameter consists of one or more segment names and qualification statements. |
AIB-mask |
Data struct parameter to store the return code or PCB-mask address returned by the application after calling a DL/I function using the AERTDLI interface. |
2. DL/I Functions
You can specify a DL/I function you want to use in the first parameter of the DL/I call. The functions provided by DL/I are largely classified into the following three types.
-
Get
Retrieves segment data from database.
Function Description GU (Get Unique)
*GHU (Get Hold Unique)
Directly retrieves a particular segment and establishes the starting position in the database for sequential processing. The qualification statements are specified in the SSA parameter.
GN (Get Next)
*GHN (Get Hold Next)
Retrieves subsequent segment instances in the hierarchical structure.
GNP (Get Next in Parent)
*GHNP (Get Hold Next in Parent)
Serves the same function as GN. Retrieves only the dependent segments under the specified parent. The parent is already set through a GU call.
-
Update
Inserts, replaces, or deletes data in the database.
Function Description ISRT (Insert)
Adds new data to a segment.
DLET (Delete)
Deletes data from a segment.
REPL (Replace)
Replaces data of a segment.
-
Others
System service functions.
Function Description CHKP, SYMCHKP (Checkpoint)
Applies all changes made by an application up to now to the database. This is similar to RDB commit function. Backs up data at the address passed through the SYMCHKP (Symbolic checkpoint).
XRST (Extended Restart)
Returns the data at the area passed through SYMCHKP back to the original address.
SYNC (Synchronization Checkpoint)
Serves the same function as CHKP.
ROLL, ROLB (Rollback)
Rolls back all data changes since the last commit. In case of ROLL, the program is closed by using the 'U007788' code.
APSB (Allocate PSB)
Allocates memory by loading the resource (PSB) to be used. This function must be called before calling the DL/I function using the AERTDLI interface.
DPSB (Deallocate PSB)
Deallocates memory through APSB. This is called to close the AERTDLI interface.
The Get function name that includes the letter H (Hold) is used before replacing or deleting a segment. Before issuing the REPL or DLET function, the program must first search for a segment by issuing the Get function with the letter H to set a lock on the data to prevent it from being modified or deleted by other users. |
3. PCB Mask
In an IMS system, application’s DL/I call information is stored in PCB. To check the success or failure of a DL/I call, an application refers to PCB Mask with structure of it and each field of the PCB Mask.
PCB Mask is the second parameter of a DL/I call, and it has nine items, such as database name, segment level number, and status code. Five of the items for developer use. The status code field stores the result code, and an application frequently uses this value in a condition to determine which processing route to take.
The segment level number or segment name item contains the information of the last segment accessed by the DL/I. This also has the information of the last segment accessed by an unqualified GN or GNP call, and allows you to figure out which segment is causing the problem when an error occurs.
The following is an example of PCB Mask implemented in a COBOL program.
01 SEGMENT1-PCB-MASK. 05 SPCB-DBD-NAME PIC X(8). 05 SPCB-SEGMENT-LEVEL PIC X(2). 05 SPCB-STATUS-CODE PIC X(2). 05 SPCB-PROC-OPTIOINS PIC X(4). 05 FILLER PIC S9(5) COMP. 05 SPCB-SEGMENT-NAME PIC X(8). 05 SPCB-KEY-LENGTH PIC S9(5) COMP. 05 SPCB-NUMB-SENS-SEGS PIC S9(5) COMP. 05 SPCB-KEY PIC X(11).
The following describes each field of PCB MASK.
Field Name | Length (Byte) | Description |
---|---|---|
Database name |
8 |
Name of the database where the current segment belongs. |
Segment level number |
2 |
Level number of the current segment in the database. If the segment is root, this field is set to "01". |
Status Code |
2 |
Status code that represents the result of DL/I call. For details, refer to DL/I Status Codes. |
Processing options |
4 |
Call type code that the program may issue. This code indicates what processing options are allowed on the database. The value of the PROCOPT parameter is passed instead when you execute PSBGEN. |
Reserved for IMS |
4 |
This field is for IMS internal use and is not used by applications. |
Segment name |
8 |
After a successful call processing, IMS saves the name of the last accessed segment. If the call fails, IMS stores the name of the last requested segment. |
Length of key |
4 |
Current length of the key feedback area. |
Number of sensitive segments |
4 |
Binary field that stores the number of the segment types that the application is sensitive to. |
Key feedback area |
variable |
Stores the concatenated key of the segment accessed during a select or insert operation. The concatenated key stores the concatenation of the key value of all segment occurrences along the hierarchical path. |
Status Code
Status code indicates the processing result of a DL/I call, and the application can refer to it to take appropriate action. A successful DL/I call returns a blank status code.
The following describes each status code.
Code | Description |
---|---|
GA |
Indicates that a segment is returned is at a higher level in the hierarchical sequence than the last segment of the path. This code is not applicable for qualified codes. It is generated when there is no more segment instances at the lowest-level. This code is used for information-only and does not affect the data. |
GB |
Indicates that the sequential search through the hierarchical path has reached the end of the database. This only occurs in GN but not in GNP. |
GE |
Indicates that there are no segment instances that match the given criteria. |
GK |
Indicates that a segment that satisfies an unqualified GNP or GN call is found, but the segment is of a different segment type at the same level. This code is used for information-only, and it does not affect the data. |
GA and GK are used for information-only, and they are returned after a successful call. GA and GB are the most commonly used codes, and you need to have a good understanding of their meanings. |
The following shows the main status codes returned for each function. For more information, refer to DL/I Status Codes.
GU/GHU | GN/GHN | GNP/GHNP | REPL/DLET | ISRT | |
---|---|---|---|---|---|
GA |
− |
Y |
Y |
− |
− |
GB |
− |
Y |
− |
− |
− |
GE |
Y |
Y |
Y |
− |
− |
GK |
− |
Y |
Y |
− |
− |
For more information about status codes, refer to DL/I Status Codes. |
4. AIB Mask
In the AERTDLI interface, AIB Mask is used to call a DL/I function.
The resource name that application uses to access the IMS system is configured in AIB Mask. In AERTDLI, the PCB-mask address is passed to AIB-mask and referenced by the application.
The following shows an example of AIB Mask implemented in a COBOL program.
01 AIB. 05 AIBRID PIC x(8). 05 AIBRLEN PIC 9(9) USAGE BINARY. 05 AIBRSFUNC PIC x(8). 05 AIBRSNM1 PIC x(8). 05 AIBRSNM2 PIC x(8). 05 AIBRESV1 PIC x(8). 05 AIBOALEN PIC 9(9) USAGE BINARY. 05 AIBOAUSE PIC 9(9) USAGE BINARY. 05 AIBRESV2 PIC x(12). 05 AIBRETRN PIC 9(9) USAGE BINARY. 05 AIBREASN PIC 9(9) USAGE BINARY. 05 AIBERRXT PIC 9(9) USAGE BINARY. 05 AIBRESA1 USAGE POINTER. 05 AIBRESA2 USAGE POINTER. 05 AIBRESA3 USAGE POINTER. 05 AIBRESV4 PIC x(40). 05 AIBRSAVE OCCURS 18 TIMES USAGE POINTER. 05 AIBRTOKN OCCURS 6 TIMES USAGE POINTER. 05 AIBRTOKC PIC x(16). 05 AIBRTOKV PIC x(16). 05 AIBRTOKA OCCURS 2 TIMES PIC 9(9) USAGE BINARY.
The following describes each field of AIB MASK. This is only applicable to the fields used in HiDB AERTDLI.
Field Name | Length (Byte) | Description |
---|---|---|
Resource name |
8 |
PSB name to call APSB or DPSB. Set PCB name to use other DL/I calls. (e.g: AIBRSNM1, AIBRSNM2) |
Resource address |
Address of PCB Mask returned after calling a DL/I function. (e.g: AIBRESA1, AIBRESA2..) |
|
Return code |
4 |
Return code of AIBTDLI. 0 is returned on success, and an error code is returned for others. (e.g: AIBRETRN) |
5. SSA
Segment search argument (SSA) contains search criteria used to process DL/I calls and serves the same purpose as the WHERE statement in RDB. Each SSA is processed as qualified if it contains at least one qualification statement and unqualified otherwise.
Unqualified SSAs
An unqualified SSA only contains the name of the segment to access. The maximum length of an SSA variable is 8 bytes and you must add a blank byte to the end of the name. If the variable is less than 8 bytes, it must be padded on the right with blanks.
It uses the following format.
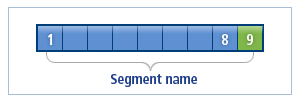
The following is an example of an unqualified SSA in a COBOL program.
01 UNQUALIFIED-SSA. 05 UNQUAL-SSA-SEGMENT-NAME PIC X(08). 05 FILLER PIC X VALUE SPACE.
Qualified SSAs
A qualified SSA contains qualification statements for a segment search. It uses the following format.
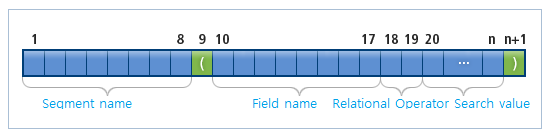
-
Segment name
Name of the segment to access.
-
Field name
Name of the field to use as the comparative value. The field name must match the name defined with a FIELD statement in the DBD script.
-
Relational Operator
Operator that compares the specified field and search value. The length of the operator is 2 bytes, and the available relational operators are:
Relationship Operator Equal to
EQ
=X
X=
Not equal to
NE
^=
=^
Greater than
GT
>X
X>
Greater than or equal to
GE
>=
=>
Less than
LT
<X
X<
Less than or equal to
LE
<=
=<
X represents one blank byte.
-
Search value
Comparative value used as an operand of the relational operator.
The following is an example of a qualified SSA in a COBOL program.
01 SEGMENT-SSA. 05 FILLER PIC X(9) VALUE 'SEGMENT1('. 05 FILLER PIC X(10) VALUE 'SEG1CODE ='. 05 SEG1-SSA-CODE PIC X(3). 05 FILLER PIC X VALUE ')'.
Command Code
By specifying a command code in SSA, you can extend and use various functions of DL/I call. Each command code is represented by one character. You can specify an asterisk (*), which separates the segment name from the command code, in the 9th position followed by a command code in the 10th position.
Command code can use both unqualified and qualified SSA and the format is as follows:
-
Unqualified SSA Format
Unqualified SSA Format with a Single Command Code -
Qualified SSA Format
Qualified SSA Format with a Single Command Code
The following is an example of an unqualified SSA with command code in a COBOL program.
01 UNQUALIFIED-SSA. 05 UNQUAL-SSA-SEGMENT-NAME PIC X(08). 05 FILLER PIC X VALUE “*”. 05 UNQUAL-SSA-COMMAND-CODE PIC X VALUE “-”. 05 FILLER PIC X VALUE SPACE.
The following describes the command codes.
Code | Description |
---|---|
C |
Searches for or inserts data by using segment’s concatenated key. |
D |
Retrieves a sequence of segments in a hierarchical path and returns data of all accessed segments to the I/O area. It is also called a path call. |
F |
Indicates the first occurrence of a segment. Executes a search or insert operation on the first occurrence of the segment. That is, Get Next operates like Get Unique. |
L |
Indicates the last occurrence of a segment. Executes a search or insert operation on the last occurrence of the segment. |
*M |
Moves a subset pointer to the next segment instance. |
N |
Used in a REPL call to prevent data, which is retrieved as a path call via the D command code, from being updated. |
P |
Specifies a particular segment’s parentage to be used when issuing a GNP call. Generally, the lowest level of a segment is set as a parentage. Use this code to set a segment at a higher level as the parentage. Even though the get request using the P command code fails, if the search is successful up to the specified segment level where the P command code is specified, the level can be set as parentage. |
Q |
Prevents the retrieved segment from being updated by other programs. |
*R |
Searches for the first occurrence of a segment in a subset. |
*S |
Unconditionally sets a subset pointer to the current segment. |
U |
Maintains the current segment position. When a segment is searched, the DL/I call moves through the hierarchy until it finds a desired data or there is no more data. This code is used to maintain the current position of a segment. |
V |
Serves the same basic function of U command code. In addition to maintaining the hierarchical level at the current position, it also maintains all superior levels of the segment where V command code is specified. |
*W |
Sets a subset pointer to the current position when the subset pointer is not yet set. |
*Z |
Allows you to reuse the subset pointer by initializing it to 0. |
― |
Represents a null command code. If a command code is set to a hyphen (-), the DL/I call is processed as an unqualified SSA. You can change this command code while the program is running to process a desired job. |
A code with an asterisk (*) can only be used in DEDB. |
The following are DL/I calls available for each command code.
GU/GHU | GN/GHN | GNP/GHNP | REPL | ISRT | DLET | |
---|---|---|---|---|---|---|
C |
Y |
Y |
Y |
− |
Y |
− |
D |
Y |
Y |
Y |
− |
Y |
− |
F |
Y |
Y |
Y |
− |
Y |
− |
L |
Y |
Y |
Y |
Y |
Y |
− |
M |
Y |
Y |
Y |
Y |
Y |
− |
N |
− |
− |
− |
Y |
− |
− |
P |
Y |
Y |
Y |
− |
Y |
− |
Q |
Y |
Y |
Y |
− |
Y |
− |
R |
Y |
− |
− |
− |
− |
− |
S |
Y |
Y |
Y |
Y |
Y |
− |
U |
Y |
Y |
Y |
− |
Y |
− |
V |
Y |
Y |
Y |
− |
Y |
− |
W |
Y |
Y |
Y |
Y |
Y |
− |
Z |
Y |
Y |
Y |
Y |
Y |
Y |
― |
Y |
Y |
Y |
Y |
Y |
Y |
Multiple Qualification Statements
Multiple qualifications can be defined in SSA by using Boolean operators.
Relationship | Operator |
---|---|
AND (2 or more qualifications are satisfied) |
'&' or '*' |
OR (1 or more qualifications are satisfied) |
'|' or '+' |
The following is an example of SSA implemented by using multiple qualification statements in a COBOL program.
01 SEGMENT1-SSA. 05 FILLER PIC X(9) VALUE ‘SEGMENT1(‘. 05 FILLER PIC X(10) VALUE ‘SEG1CODE>=‘. 05 SEG1-SSA-LOW-CODE PIC X(3). 05 FILLER PIC X VALUE ‘&‘. 05 FILLER PIC X(10) VALUE ‘SEG1CODE<=‘. 05 SEG1-SSA-HIGH-CODE PIC X(3). 05 FILLER PIC X VALUE ‘)‘.