Compiler-directing statement
A compiler-directing statement is a statement that is used to direct the compilation of a program. The COPY and REPLACE statements are used to control the source text before compilation. This chapter explains how to use compiler-directing statements.
1. COPY
The COPY statement provides a function to insert prewritten texts into the program source.
The prewritten codes are stored in a copybook file and the source codes are included in a compilation unit. To use the copybook file, register the path to the copybook with the environment variable, $OFCOBCPY.
The following shows the format of the COPY statement.
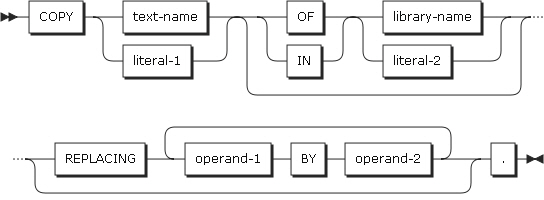
The following items are specified in the statement.
-
text-name, literal-1
-
Defines the name of the copybook file which contains the source code to be converted. The corresponding copybook is looked up in the path to the copybook that is specified in the environment variable.
-
The rules for naming files apply to copybook names.
-
The name of the copybook is restricted to 8 characters on the COPY statement.
-
To use a name exceeding 8 characters, use the compile option --disable-cpy-limit.
-
-
library-name, literal-2
-
Specifies the copybook file path.
-
-
REPLACING phrase
-
The REPLACING phrase finds an item that matches the text corresponding operand-1 and replaces it with the specified operand-2.
-
The following values can be specified as operands.
Item Description pseudo-text
A series of character strings and separators bounded by the pseudo-text delimiter (==)
The pseudo-text delimiter (==) must appear on a single line, but the pseudo-text itself can span multiple lines.
ID
Can be named in any section in the data division.
Literal
A numeric, alphanumeric, or two-byte character literal
COBOL word (except COPY)
Any COBOL word excluding COPY
Function ID
A function ID
-
The following rules apply to using the COPY statement.
-
A COPY statement can be used in any context where a character string or separator is allowed.
-
Each COPY statement converts the statement into the contents of the copybook, starting with the COPY word and finishing with a period (.). When the REPLACING phrase is specified, text conversion is performed according to the given rules.
-
A COPY statement can be duplicate within a COPY statement. If duplicated, REPLACING phrase cannot be specified. In addition, the copybook for which REPLACING phrase is specified cannot be set in the COPY statement. 10 or more duplicate copybooks cannot be used.
-
Recursive use of the COPY statement is not allowed. For example, if COPY X exists, and the copybook X contains COPY Y, a COPY X or COPY Y statement cannot be included in the copybook Y.
-
The source validity of the copybook is not checked. Syntax checking is performed after all COPY and REPLACE statement processes are completed. The source text written in the copybook is inserted without changes, so it must be written in a suitable format for source text when the copybook is created.
Replacement and Comparison Rules
The following are the rules of replacement and comparison.
-
Arithmetic and logical operands are considered text words and can be replaced by pseudo-text. Leading and trailing blanks of the pseudo-text are excluded. However, any blanks within the text are used.
-
When operand-1 is a figurative constant, it matches only the same figurative constant.
For example, if ALL "AB" is specified, "ABAB" is not matched.
-
When replacing a PICTURE character string, the pseudo-text delimiter must be specified. To avoid ambiguities when replacing a PICTURE character string, it is recommended to specify the entire PICTURE character string, including the keyword, such as PICTURE and PIC.
-
Any delimiting comma (,) semicolon (;), or blank which appears in the copybook file is inserted.
-
operand-1 only matches the library text if the ordered sequence of the texts in operand-1 is exactly the same as that of the library text. Comparing the text repeats until a match is found or there are no more REPLACING operands.
Every time a text that matches operand-1 is found, the contents of operand-2 are inserted.
-
A COPY statement that includes the REPLACING phrase can replace parts of words. Defined dummy operands delimited by a colon (;) can be replaced in the desired format that best suits a situation.
-
Comment and empty lines are ignored in the process of comparison.
Examples
In the following example, the copybook PAYLIB is used to illustrate how to define data items.
01 A. 02 B PIC S99. 02 C PIC S9(5)V99. 02 D PIC S9999 OCCURS 1 TO 52 TIMES DEPENDING ON B OF A.
Programmers can use a COPY statement in the data division of a program as follows:
COPY PAYLIB.
The source defined in the copybook PAYLIB is inserted into programs.
The following illustrates how to change the name using REPLACING.
COPY PAYLIB REPLACING A BY PAROLL B BY PAY-CODE C BY GROSS-PAY D BY HOURS.
In this program, the library text is inserted in the following format.
01 PAYROLL. 02 PAY-CODE PIC S99. 02 GROSS-PAY PIC S9(5)V99. 02 HOURS PIC S9999 OCCURS 1 TO 52 TIMES DEPENDING ON PAY-CODE OF PAYROLL.
The specified replacement is performed only for the program. The copybook PAYLIB is not changed.
A copybook with the following rules can be changed by specifying REPLACING in the part of the name; for example, as a prefix.
01 :TAG:. 02 :TAG:-WEEK PIC S99. 02 :TAG:-GROSS-PAY PIC S9(5)V99. 02 :TAG:-HOURS PIC S9999 OCCURS 1 TO 52 TIMES DEPENDING ON :TAG:-WEEK OF :TAG:.
Programmers can use a COPY statement in the data division of the program as follows:
COPY PAYLIB REPLACING ==:TAG:== BY ==Payroll==. or COPY PAYLIB REPLACING 'TAG' BY Payroll. =.
The result of the inserted program will be like the following.
01 PAYROLL. 02 PAYROLL-WEEK PIC S99. 02 PAYROLL-GROSS-PAY PIC S9(5)V99. 02 PAYROLL-HOURS PIC S9999 OCCURS 1 TO 52 TIMES DEPENDING ON PAYROLL-WEEK OF PAYROLL.
The following example illustrates how to replace level numbers in the PICTURE character string.
COPY xxx REPLACING ==(01)== BY ==(01)== == 01 == BY == 05 ==.
2. REPLACE
The REPLACE statement is used to convert text within the source program. A REPLACE statement can be used in any context where a character string is allowed.
The REPLACE statement is used to specify the entire COBOL compilation group or a compilation unit for the parts that require changes. This is similar to comparison and replacement using REPLACING with the COPY statement, except that it applies to the entire source of the program, not just to the process of inserting a specified copybook.
-
Format 1
Whenever a text that matches pseudo-text-1 appears within the source program, it is replaced by the corresponding pseudo-text-2.
REPLACE Statement Format 1 -
Format 2
The replacement rule specified by REPLACE statement is applied until REPLACE-OFF appears. Without REPLACE-OFF, the REPLACE rule takes effect from the point where it is specified until the next REPLACE statement appears or the end of the program is reached.
REPLACE Statement Format 2
The following items are specified in the statement.
-
pseudo-text-1
-
Must contain one or more text words.
-
pseudo-text-1 can only have a separator comma (,) or a separator semicolon (;).
-
-
pseudo-text-2
-
Can zero or more texts. No words indicate deletion.
-
pseudo-text-1 and pseudo-text-2 can use any text words which can be written in source program, except COPY.
The compiler first processes any COPY statements and then processes the REPLACE statement within the source program, as the complete source must first be created first. After the complete source program is created, conversion of simple character strings is accomplished using the REPLACE statement. The REPLACE statement cannot contain a COPY statement.
Syntax checking for the source program cannot be performed until the processes for the COPY and REPLACE statements are complete. |
3. READY/RESET TRACE
The READY or RESET TRACE statement is used to trace procedure executions.
This statement can be used in any context where a general statement is allowed using the compile option --trace in OFCOBOL.
If the compilation option is not specified, this statement is ignored.
-
READY TRACE
READY TRACE STATEMENTDisplays the names of the procedures that are executed after a READY TRACE statement execution.
-
RESET TRACE
RESET TRACE STATEMENTDoes not display the names of the procedures that are executed after a RESET TRACE statement execution.