Procedure Division
This chapter describes the structure of the procedure division and the usage of each statement.
1. Procedure Division Structure
The following is the basic structure of the procedure division.
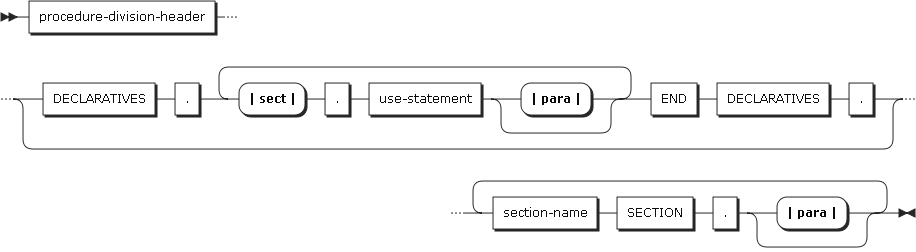

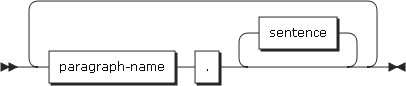
The procedure division consists of the following.
-
Header
-
Declaratives
-
Procedures
-
Arithmetic expressions
-
Conditional expressions
-
Statement categories
-
Statement operations
1.1. Header
A procedure division header consists of the USING paragraph and the RETURNING paragraph.
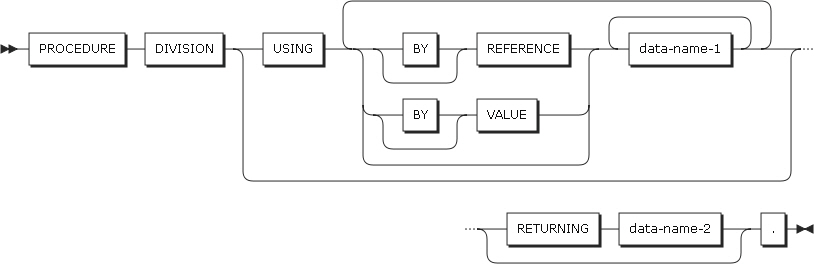
Each paragraph is described as follows:
-
USING paragraph
-
The USING paragraph is specified to receive parameters from a called program.
-
To receive data through USING, a data item must be described as level-01 or 77 in the linkage section of the called program. To pass data to a subprogram using CALL, a data item from the parameter to be transmitted must be described in the data division of the called program.
Items specified in the linkage section must satisfy one of the following conditions.
-
Are operands specified in the USAGE paragraph
-
Are operands of SET ADDRESS OF and CALL … BY REFERENCE ADDRESS OF
-
Are operands which satisfy the previous conditions and where REDEFINES or RENAMES is defined
-
Are subitems of an item that satisfies the previous conditions
-
Are condition-names or index-names that satisfy any of the previous conditions
-
-
The USING paragraph can be used to pass data from non-COBOL programs to COBOL programs, and vice versa.
-
The relationship between parameters passed from calling programs and those passed to called programs is determined by the positions of the specified parameters rather than by their names. Corresponding identifiers in calling and called programs do not need to have the same data description, but the number of bytes must be the same.
-
Parameters can be passed either in the BY REFERENCE or the BY VALUE phrase. For further information, refer to CALL.
-
-
RETURNING paragraph
-
The RETURNING paragraph is specified to pass a value to a calling program.
-
data-name2 must be specified as level-01 or level-77 in the linkage section of the program.
-
The RETURNING data item is an output-only data item. In the initial state of the program, the value of a data item is unpredictable. Initialize the RETURNING data item before referencing it. For more information, refer to CALL.
-
1.2. Declaratives
Declarative sections describe the actions that will be executed when an exceptional condition occurs while a program is running.
Declarative sections must be described in the first part of the procedure division, and all procedures must be divided into sections. Each declarative section starts with a USE statement and is followed by the actions that will be executed when an exceptional condition occurs.
All declarative sections must be described within DECLARATIVES and END DECLARATIVES statements.
A declarative section ends with END DECLARATIVES, or a USE statement which indicates the beginning of another declarative section. The USE statement itself is not executed, but it defines exceptional conditions and actions to be executed when an exception occurs. After the procedure is executed due to an exception, control is returned to the routine that caused the exceptional condition.
A declarative procedure can be used as follows:
-
A declarative procedure can be executed from a non-declarative procedure.
-
A non-declarative procedure can be executed from a declarative procedure.
-
A declarative procedure can be referenced in a GO TO statement described in a declarative procedure.
-
A non-declarative procedure can be referenced in a GO TO statement described in a declarative procedure.
1.3. Procedures
In the procedure division, a procedure consists of a section or a section group, and a paragraph or a paragraph group.
A procedure-name is a user-defined name that identifies a section or a paragraph.
-
Section
A section is composed of a section-header, a section-name, a priority-number, and a period (.).
Item Description section-header
A section-header consists of a section-name, the keyword SECTION, a priority-number, and a period. A section-header can be omitted.
section-headers can be described after the keywords END DECLARATIVES or if there are no declaratives.
section-name
A section-name is a user-defined word used to identify a section. It must be unique within the procedure division.
priority-number
A number from 0 to 99, which does not influence the program. This is a fixed segment or a section that includes a segment. A section in the declaratives must include numbers in the range of 0 to 49.
-
Paragraph
A paragraph consists of a paragraph-name, a period(.), and sentences. Each paragraph must be divided by a period.
Item Description paragraph-name
A user-defined word used to identify a paragraph. It can be qualified.
Sentence
A sentence is composed of one or more statements divided by a period.
-
Statement
-
A statement starts with a COBOL verb, and then syntactically valid identifiers, literals, and operators follow.
-
The program is executed from the first statement in the procedure division.
-
The end of the procedure division can be indicated by a following identification division, which indicates the start of a nested program, an END PROGRAM marker, or the physical end of the file.
-
-
1.4. Arithmetic Expressions
Arithmetic expressions are used as operands of conditional or arithmetic statements. Any of the following can be an arithmetic expression.
-
A numeric elementary item identifier.
-
A numeric literal.
-
The figurative constant ZERO.
-
An arithmetic expression that consists of identifiers, arithmetic symbols, and numeric literals.
-
An arithmetic expression that consists of an arithmetic expression and an arithmetic symbol expression.
-
An arithmetic expression enclosed in parentheses.
A unary arithmetic operator can be specified before an arithmetic expression. Identifiers and literals in arithmetic expressions must be numeric elementary items or numeric literals on which arithmetic can be operated.
If both a positive number and a negative number result from an exponential expression, the positive number will always be selected as the result. If the base number of an expression is zero, the exponent must be larger than zero. Otherwise, the size error condition will occur.
Arithmetic Operators
Binary operators and unary operators can be used. The meaning of each operator is described in the following table.
-
Binary operators
Operator Meaning +
Addition
-
Subtraction
*
Multiplication
/
Division
**
Exponentiation
-
Unary operators
Operator Meaning +
Multiplying by +1
-
Multiplying by -1
Arithmetic expressions operate from left to right, and expressions between parentheses are evaluated first.
The following priority order applies.
-
Unary operator
-
Exponentiation
-
Multiplication and division (A multiplication operator and a division operator are at the same level, and the expression is evaluated from left to right in order.)
-
Addition and subtraction (An addition operator and a subtraction operator are at the same level, and the expression is evaluated from left to right in order.)
An arithmetic expression must start with a unary operator, a left parenthesis '(', or an operand and must end with a right parenthesis ')' or an operand. An arithmetic expression can contain one identifier or a literal. In an arithmetic expression, a left parenthesis must always be followed by an expression and then a right parenthesis, and neither can be used without the another.
The following table shows available matches between arithmetic symbols. The leftmost column in the table indicates the first operator or operand, and the first row indicates the second operator or operand.
Identifier or literal constant | * / ** + - | Unary operator (+, -) | Parenthesis ( | Parenthesis ) | |
---|---|---|---|---|---|
Identifier or literal constant |
O |
O |
|||
* / ** + - |
O |
O |
O |
||
Unary operator + - |
O |
O |
|||
Parenthesis ( |
O |
O |
O |
||
Parenthesis ) |
O |
O |
1.5. Conditional Expressions
A conditional expression is used for the program to selectively modify the execution logic by determining the truth value. Conditional expressions can be used in EVALUATE, IF, PERFORM, and SEARCH statements.
A conditional expression can be specified in either of the following.
-
Simple conditions
-
Complex conditions
1.5.1. Simple Conditions
A simple condition has either a true or false value.
There are four kinds of simple conditions.
-
Class conditions
-
Condition-name conditions
-
Relation conditions
A relation condition compares two operands. A comparison is defined as follows:
-
Two alphabetic class operands
-
Two alphanumeric class operands
-
Two DBCS class operands
-
Two numeric class operands
-
An alphabetic class operand and an alphanumeric class operand
-
A numeric integer operand and an alphanumeric class operand
-
Comparison between indexes or index data items
-
Two data pointer operands
-
Two procedure pointer operands
-
Two function pointer operands
-
Alphanumeric groups, and operands with the DISPLAY or DISPLAY-1 usage
There are two kinds of relation conditions.
-
General relation conditions
-
Data pointer relation conditions
-
-
Sign conditions
-
Switch-status conditions
Class Conditions
The class condition determines if the content of a data item is alphabetic, alphabetic-lower, alphabetic-upper, numeric, DBCS, KANJI, or includes the characters specified in the CLASS clause that is defined in the SPECIAL-NAMES paragraph of the environment division.
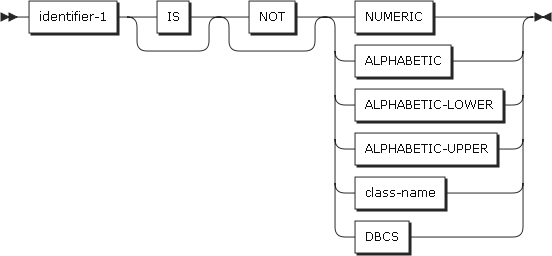
-
identifier-1
-
A data item with one of the following usages must be referenced.
-
When NUMERIC is specified: DISPLAY, COMPUTATIONAL-3, or PACKED-DECIMAL
-
When DBCS is specified: DISPLAY-1
-
When ALPHABETIC, ALPHABETIC-UPPER, or ALPHABETIC-LOWER is specified: DISPLAY
-
When class-name is specified: DISPLAY
-
-
If identifier-1 is a function-identifier, referencing the alphanumeric function is required.
-
One of the following must be set.
Item Description NOT
Negates a truth value.
For instance, the result of NOT NUMERIC becomes true if the test of NUMERIC class is false.
NUMERIC
identifier-1 all consists of the numbers from 0 to 9.
ALPHABETIC
identifier-1 consists of lowercase or uppercase alphabetical characters from A to Z, and space characters.
ALPHABETIC-LOWER
identifier-1 consists of lowercase alphabetical characters from a to z, and space characters.
ALPHABETIC-UPPER
identifier-1 consists of uppercase alphabetical characters from A to Z, and space characters.
class-name
identifier-1 consists of the characters defined in the SPECIAL-NAMES paragraph class-name.
DBCS
identifier-1 consists of DBCS characters.
-
The following table shows the relationship between available data item types and the class condition.
Type of Data Item Referenced by identifier-1 | Valid Forms of the Class Condition | |
---|---|---|
Alphabetic |
ALPHABETIC ALPHABETIC-LOWER ALPHABETIC-UPPER class-name |
NOT ALPHABETIC NOT ALPHABETIC-LOWER NOT ALPHABETIC-UPPER NOT class-name |
Alphabetic, Alphabetic-edited, Numeric-edited |
ALPHABETIC ALPHABETIC-LOWER ALPHABETIC-UPPER NUMERIC class-name |
NOT ALPHABETIC NOT ALPHABETIC-LOWER NOT ALPHABETIC-UPPER NOT NUMERIC NOT class-name |
External-decimal, Internal-decimal |
NUMERIC |
NOT NUMERIC |
DBCS |
DBCS |
NOT DBCS |
Numeric |
NUMERIC class name |
NOT NUMERIC NOT class name |
Condition-name Conditions
A condition-name tests a conditional variable, and determines which value of condition-name is equal to its value.

Item | Description |
---|---|
condition-name-1 |
Used as an abbreviation for the relation condition. The rules of comparison between a conditional variable and a condition-name value are the same as those of relation conditions. If condition-name-1 is a range of values, the conditional variable is tested to see whether its value is included in the range. If the value of the conditional variable is the same as one of the values of condition-name, the result will be true. |
The following example shows how to use conditional variables and condition-names.
01 AGE-GROUP PIC 99. 88 INFANT VALUE 0. 88 BABY VALUE 1, 2. 88 CHILD VALUE 3 THRU 12. 88 TEENAGER VALUE 13 THRU 19.
AGE-GROUP is the conditional variable. INFANT, BABY, CHILD, and TEENAGER are condition-names.
The following IF statement determines the age group with the values of AGE-GROUP.
IF INFANT... (Tests for value 0) IF BABY... (Tests for values 1, 2) IF CHILD... (Tests for values 3 through 12) IF TEENAGER... (Tests for values 13 through 19)
General Relation Conditions
A general relation condition compares two operands. The operands can be an identifier, literal, arithmetic expression, or index-name.
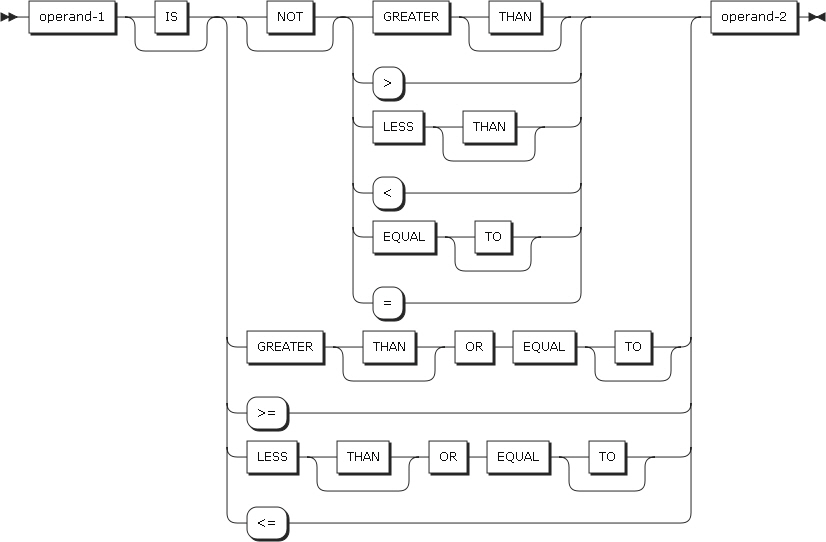
Item | Description |
---|---|
operand-1 |
The subject of the relation condition. Can be an identifier, literal, function-identifier, arithmetic expression, or index-name. |
operand-2 |
The object of the relation condition. Can be an identifier, literal, function-identifier, arithmetic expression, or index-name. |
In a general relation condition, data items, literals, and figurative constants can be compared using the following comparison types.
Comparison type | Description |
---|---|
Alphanumeric |
Comparison between alphanumeric characters of two operands. |
DBCS |
Comparison between DBCS characters of two operands. |
Numeric |
Comparison between the algebraic values of two operands. |
GROUP |
Comparison between alphanumeric characters of two operands, when one of the operands is an alphanumeric group item. |
(Int) |
Comparison only between integer items (co-used with Alph, Num, or Group). |
Blank |
Comparison is unavailable. |
The following tables show pairs of comparison between different operand types.
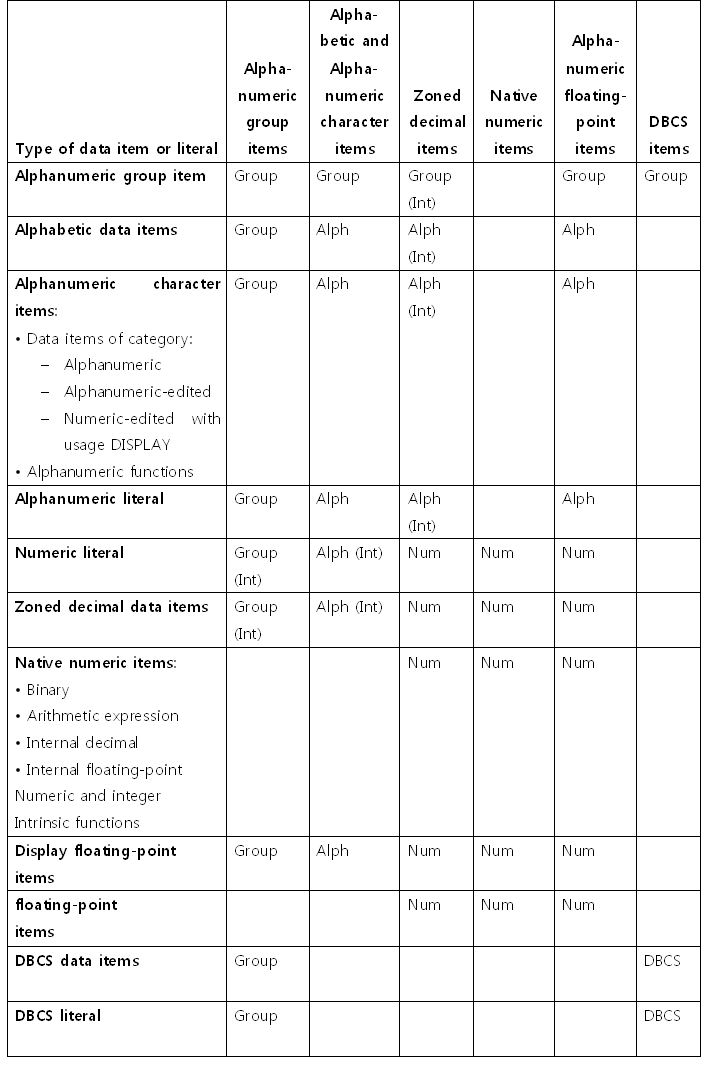
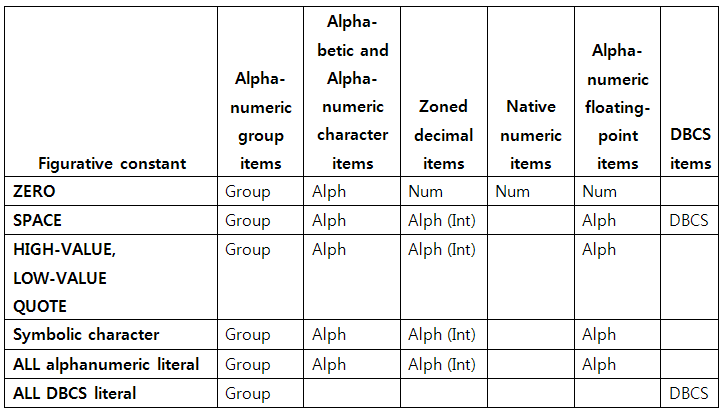
-
Alphanumeric comparisons
-
Compares the single-byte characters of two operands using the collating sequence.
-
If an operand is not alphanumeric or alphabetic, the following is performed.
-
A zoned decimal integer operand is treated as a zoned decimal alphanumeric item with the same size.
-
A display floating-point item is treated as an alphanumeric item, not a numeric value.
-
-
If both operands have the same size, they are compared from the leftmost character to the rightmost character. When the first difference is encountered, the priority is determined by comparing each character’s position in the collating sequence.
-
If the operands have different sizes, spaces are added to the shorter operand to make them the same size before comparing them.
-
-
DBCS comparisons
Performs the same comparison as alphanumeric comparison by using the binary hexadecimal collating sequence.
-
Numeric comparisons
Performs algebraic comparison of two operands.
-
Group comparisons
Performs an alphanumeric character comparison of two operands. This is the same as the alphanumeric elementary data item comparison.
-
Index comparisons
Compares occurrence numbers of two index items. If an index is compared with a data item or literal, its occurrence number is compared with the data or literal value.
Data Pointer Relation Conditions
For pointer data items, only the 'equal' and 'not equal' comparisons are available. Pointer data items are items defined as USAGE POINTER, or are ADDRESS OF special registers.
The relation condition can be used in IF, PERFORM, EVALUATE, and SEARCH (format-1) statements. It cannot be used in SEARCH format-2(SEARCH ALL) statements.
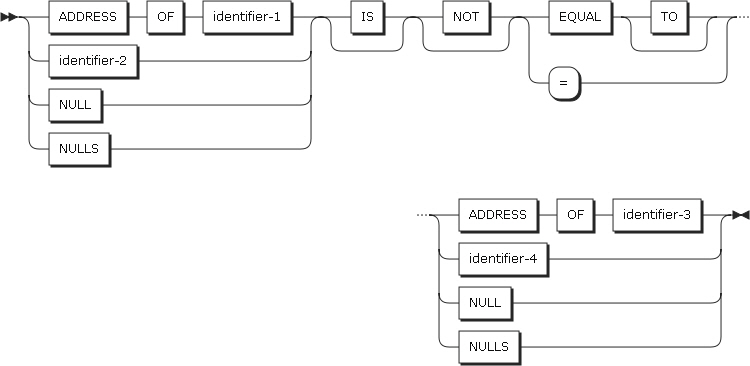
Item | Description |
---|---|
identifier-1, identifier-3 |
Any data items except level-66 and level-88 can be used. |
identifier-2, identifier-4 |
Data item defined as USAGE POINTER. |
NULL, NULLS |
NULL pointer. |
Procedure-pointer and Function-pointer Relation Conditions
The procedure-pointer and function-pointer relation condition only determines whether the items are equal or not.

Item | Description |
---|---|
identifier-1, identifier-3 |
Either USAGE PROCEDURE-POINTER or FUNCTION-POINTER. |
NULL, NULLS |
NULL pointer. |
Sign Conditions
The sign condition determines whether the value of a numeric operand is larger than, smaller than, or equal to zero.
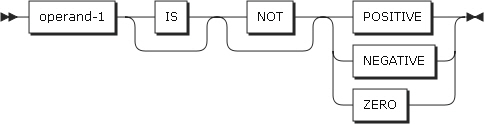
Item | Description |
---|---|
operand-1 |
Numeric identifier or an arithmetic expression. It is POSITIVE if its value is larger than zero, NEGATIVE if it is smaller than zero, and ZERO if it is equal to zero. An operand without a sign can be either POSITIVE or ZERO. |
NOT |
Negatives a true or false value. For instance, NOT ZERO is determined as true when the operand is a positive or negative value which is not zero. |
Switch-status Conditions
The switch-status condition determines the On or Off status of an UPSI switch. The result is determined according to the status value of the UPSI switch.
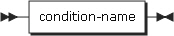
Item | Description |
---|---|
condition-name |
UPSI switch defined in the special-names paragraph. |
1.5.2. Complex Conditions
A complex condition is composed of a combination of simple conditions and combined conditions with logical operators, or negations of conditions with logical negation. Space characters are needed before and after each logical operator.
Unless parentheses are used, the following priority order applies.
-
Arithmetic operator
-
Simple conditions
-
NOT
-
AND
-
OR
The truth value of a complex condition is determined by the mutual relationship between the truth value of each simple condition and the logical operator.
A complex condition is either of the following.
-
Negated simple condition
-
Combined condition (which can be negated)
Negated Simple Conditions
A simple condition can change the true or false value by using the logical operator NOT.

Item | Description |
---|---|
conditions-1 |
Gives the opposite result to the true or false value of condition-1. If condition-1 is true, the result will be false. If condition-1 is false, the result will be true. |
Even if condition-1 is enclosed with parentheses, the result does not change. For example, the following two sentences are equivalent.
NOT A IS EQUAL TO B NOT ( A IS EQUAL TO B )
Combined Conditions
A combined condition consists of two or more conditions connected by a logical operator.
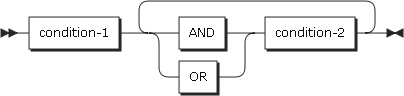
condition-1 or condition-2 can be any of the following.
-
Simple condition
-
Negated simple condition
-
Combined condition
-
Negated combined condition (when a combined condition enclosed within parentheses appears after the logical operator NOT)
The following table shows a relationship between the logical operator, condition C1, and C2.
C1 | C2 | C1 AND C2 | C1 OR C2 | NOT (C1 AND C2) | NOT C1 AND C2 | NOT (C1 OR C2) | NOT C1 OR C2 |
---|---|---|---|---|---|---|---|
True |
True |
True |
True |
False |
False |
False |
True |
False |
True |
False |
True |
True |
True |
False |
True |
True |
False |
False |
True |
True |
False |
False |
False |
False |
False |
False |
False |
True |
False |
True |
True |
Abbreviated Combined Relation Conditions
If relation conditions are consecutively written, a relation condition after the first relation condition can be abbreviated in either of the following ways.
-
Omitting the subject
-
Omitting the subject and relation operator

In associated relation conditions, both kinds of abbreviation can be applied. The abbreviated condition can be determined as follows:
-
The last described subject is the omitted subject.
-
The last described relation operator is the omitted operator.
The following table shows examples of abbreviated conditions.
Abbreviated Combined Relation Condition | Equivalent |
---|---|
A = B AND NOT < C OR D |
((A = B) AND (A NOT < C)) OR (A NOT < D) |
A NOT > B OR C |
(A NOT > B) OR (A NOT > C) |
NOT A = B OR C |
(NOT (A = B)) OR (A = C) |
NOT (A = B OR < C) |
NOT ((A = B) OR (A < C)) |
NOT (A NOT = B AND C AND NOT D) |
NOT ((((A NOT = B) AND (A NOT = C)) AND (NOT (A NOT = D)))) |
1.6. Statement Categories
This section describes components of statement categories.
1.6.1. Imperative Statements
An imperative statement is an unconditionally executed statement, or a conditional statement terminated by specifying END-ADD or END-DIVIDE. An imperative statement can be described wherever such statements are allowed.
The following table shows the COBOL imperative statements.
Item | Description |
---|---|
Arithmetic |
When the ON SIZE ERROR or NOT ON SIZE ERROR phrase is not specified. |
Data movement |
(*): When the ON OVERFLOW or NOT ON OVERFLOW phrase is not specified. |
Ending |
|
Input-output |
(*): When the INVALID KEY or NOT INVALID KEY phrase is not specified. (+): When the AT END, NOT AT END, INVALID KEY, or NOT INVALID KEY phrase is not specified. (-): When the INVALID KEY, NOT INVALID KEY, END-OF-PAGE, or NOT END-OF-PAGE phrase is not specified. |
Ordering |
(*): When the AT END or NOT AT END phrase is not specified. |
Procedure-branching |
|
Program linkage |
(*): When the ON OVERFLOW, ON EXCEPTION, or NOT ON EXCEPTION phrase is not specified. |
Table-handling |
|
1.6.2. Conditional Statements
A conditional statement describes actions to be executed if the specified conditional statement is true.
The following table shows the COBOL conditional statements.
Item | Description |
---|---|
Arithmetic |
|
Data movement |
|
Decision |
|
Input-output |
|
Ordering |
|
Program linkage |
|
Table-handling |
|
1.6.3. Delimited Scope Statements
A delimited scope statement converts a conditional statement into an imperative statement using an explicit scope terminator.
1.6.4. Explicit Scope Terminators
An explicit scope terminator marks a scope of certain statements of the procedure division. A conditional statement delimited by an explicit scope terminator is regarded as an imperative statement, and it must follow the rules for imperative statements.
The following explicit scope terminators are used in the procedure division.
-
END-ADD
-
END-CALL
-
END-COMPUTE
-
END-DELETE
-
END-DIVIDE
-
END-EVALUATE
-
END-IF
-
END-MULTIPLY
-
END-PERFORM
-
END-READ
-
END-REWRITE
-
END-RETURN
-
END-SEARCH
-
END-START
-
END-STRING
-
END-SUBTRACT
-
END-UNSTRING
-
END-WRITE
1.6.5. Implicit Scope Terminators
As an implicit scope terminator, a period is used at the end of a sentence. A period terminates the scope of all previous statements which have not yet terminated. Another statement cannot be include in an unterminated conditional statement.
Only imperative statements can be contained in another statement, except IF statements that have nested conditional statements.
1.7. Statement Operations
This section describes phrases used in arithmetic or data manipulation statements.
1.7.1. CORRESPONDING Phrase
The CORRESPONDING phrase performs ADD, SUBTRACT, and MOVE operations for elementary data items with the same name in operations of the specified group data item, if the phrase is stated in ADD, SUBTRACT, and MOVE statements.
Data items that follow the keyword CORRESPONDING must specify group items. Here, identifier-1 indicates a sending group item, and identifier-2 indicates a receiving group item.
To perform the corresponding operation, the following conditions must be satisfied for data items belonging to identifier-1 and identifier-2.
-
In an ADD or SUBTRACT statement, both of the elementary data items must be numeric data items. For other items, operations for addition or subtraction will be not performed.
-
Subordinate data items in the group must have the same name, and share the same qualifiers except for the names of identifier-1 and identifier-2.
-
The keyword FILLER is excluded.
-
Items for which level-66, level-77, level-88, an index data item, or reference-modified is specified cannot be described as identifer-1 or identifier-2.
-
The items for which USAGE POINT, USAGE FUNCTION-POINT, or USAGE PROCEDURE-POINTER is specified cannot be specified as identifier-1 or identifier-2.
-
If USAGE POINTER, USAGE PROCEDURE-POINTER, REDEFINES, RENAMES, or OCCURS is specified for the subordinate items of identifier-1 or identifier-2, the items will be excluded.
1.7.2. GIVING Phrase
The identifier specified after GIVING is not involved in the arithmetic operation, but stores the result of the operation. It is also possible to specify a numeric-edited item.
1.7.3. ROUNDED Phrase
After aligning the decimal point, the result of an arithmetic operation is compared with the number of places described where the result value will be saved. If the result size is greater than the number of places, truncation occurs. If the ROUNDED phrase is specified, and the leftmost digit of the exceeded places is greater than or equal to 5, the rightmost digit of the result is increased by 1.
If the result size is greater than the number of places in the PICTURE clause, truncation or rounding occurs.
In a floating-point operation, the result is always rounded regardless of whether the ROUNDED phrase is specified.
1.7.4. SIZE ERROR Phrase
A size error occurs in the following conditions.
-
When the absolute value of the result of an arithmetic operation exceeds the largest value of the field in which the result will be saved
-
When dividing by zero
The size error only applies to a final result, rather than to an intermediate result. The largest value of a result that USAGE BINARY, COMP, COMP-4, or COMP-5 data items can be saved is determined by the PICTURE character-string of the item.
If the ROUNDED phrase is specified, rounding occurs before checking for a size error.
The actions that are performed after a size error condition occurs depend on whether or not the ON SIZE ERROR phrase is specified.
-
If the ON SIZE ERROR phrase is not specified, and a size error condition occurs, the value is truncated by the truncation rules and is saved as a result.
-
If the ON SIZE ERROR phrase is specified and a size error condition occurs, the calculated result is not saved. Instead, the imperative statement specified in ON SIZE ERROR is executed, and control is transferred to the next statement.
If a size error condition occurs while an individual operation is performed for the ADD CORRESPONDING and SUBTRACT CORRESPONDING statements, the imperative statement specified in the ON SIZE ERROR phrase is not executed before the operation completes.
If the NOT ON SIZE ERROR phrase is specified, and a size error does not occur, the imperative statement described in the NOT ON SIZE ERROR phrase is executed.
1.7.5. Arithmetic Statement Operands
In an arithmetic operation, the compiler performs data conversion and decimal point alignment if needed, so arithmetic statement operands do not need to have the same data definition.
-
Size of operands
An operand can be specified up to 31 decimal digits.
For all operation statements, it is important to specify sufficient decimal places and integer places for the precision of results.
-
The composite operands are data items that are used for compiler operations by aligning the operands at the decimal point and re-sorting the operand values.
As shown in the following addition operation example, the compiler converts each operand into data items like PIC 9(12)V9(7).
A PIC 9(7)V9(5) c PIC 9(11)V99 B PIC 9(12)V9(3) ADD A B TO C
-
Overlapping operands
If the operands in an arithmetic statement share memory storage (that is, if the operands overlap), the result of the operation is unpredictable.
-
Multiple results
If an arithmetic statement saves multiple results, it is conceptually processed as follows:
-
An intermediate result obtained from an arithmetic operation described before TO or FROM is saved in a temporary variable.
-
An arithmetic operation is executed on the intermediate result saved in the temporary variable, and the result is saved.
-
1.7.6. Data Manipulation Statements
The following statements are used to move and inspect data.
-
ACCEPT
-
INITIALIZE
-
INSPECT
-
MOVE
-
READ
-
RELEASE
-
RETURN
-
REWRITE
-
SET
-
STRING
-
UNSTRING
-
WRITE
If the sending and receiving fields of a statement share storage, the result is unpredictable. |
1.7.7. Input-Output Statements
Input-output statements save data in files, or get data from files. In COBOL, the unit of data used in files is a record.
The available input-output statements for the procedure division depend on the description of the file in the environment division and the data division.
1.7.8. Common Processing Facilities
The following common processing facilities are provided.
-
File status key
If the FILE STATUS clause is described in the file-control entry, the result of the statement on that file is saved in the specified file status key (the two-character data item specified by the FILE STATUS KEY clause). The result is saved before the EXCEPTION/ERROR declarative, INVALID KEY phrase, or AT END phrase is executed.
There are two file status key data-names. One is data-name-1 of the FILE STATUS clause, a two-character data item. The first character is file status key 1, and the second character is file status key 2.
The values of the file status key are described in the following table.
File status key Meaning 00
Successfully completed.
02
Applies to indexed files. The statement was successfully completed, and a duplicate key was found.
07
Successfully completed. For a CLOSE statement with the NO REWIND, REEL/UNIT, or FOR REMOVAL phrase, or for an OPEN statement with the NO REWIND phrase, the file is not a tape.
10
The at-end condition. There are no more records for the executed of the sequential READ statement.
14
The at-end condition. The sequential READ statement was executed for a relative file, and the relative key exceeded the scope.
22
Invalid key condition. Attempted to write a record in a relative file, or write or rewrite a record in an indexed file.
23
Invalid key condition. For the READ or START statements, the record corresponding to the key does not exist in the file.
37
Attempted to use a random access to a sequential dataset, or use an extend mode to a relative file.
38
Attempted to use an OPEN statement on a file closed by CLOSE WITH LOCK.
39
The file attributes described in the COBOL program are different from the attributes of the physical file. (RECORD FORMAT, RECORD LENGTH, FILE ORGANIZATION, etc)
41
The file is already open.
42
The file to be closed has not been opened.
43
While attempting to execute a REWRITE or DELETE statement in a sequential access mode, the final executed I/O statement was not a READ statement.
44
For a file using the RECORD IS VARYING clause, attempted to write or rewrite a record that exceeded the limitation of the record length.
46
While executing a sequential READ statement, the previous READ statement was terminated by the at-end condition, so the file pointer indicator for the next record has not been specified.
47
While executing a READ statement, a file was not opened in INPUT or I-O mode.
48
While executing a WRITE statement, a file was not opened in OUTPUT, I-O or EXTEND mode.
49
While executing a DELETE or REWRITE statement, a file was not opened in I-O mode.
92
While executing a READ statement, the length of the record was longer than the length described in the COBOL program.
The other file status key data-name is data-name-8 of the FILE STATUS clause. This is not used.
-
Invalid key condition
While a START, READ, WRITE, REWRITE, or DELETE statement is being executed, an invalid key condition can occur. If the invalid key condition occurs, the following actions will be taken.
-
If the FILE STATUS clause is described in the file-control entry, a value corresponding to the invalid key condition is saved in the file status key.
-
If the INVALID KEY phrase is specified, the imperative statement described in the phrase is executed. The EXCEPTION/ERROR declarative procedure described for this file is not executed.
-
If the INVALID KEY phrase is not specified, and the EXCEPTION/ERROR declarative procedure for this file is described, it is executed. The NOT INVALID KEY phrase will be ignored.
-
2. Statements
The following table contains a list of statements. For more information, refer to the relevant section.
Statement | Description |
---|---|
Data area referenced by the described identifier. Used to transmit data and system date information. |
|
Stores the result of addition of two or more operands in a certain operand. |
|
Changes control from one program to another while the program is running. |
|
Used to process the program, specified as an operand, in initial state. |
|
Terminates the processing of files. |
|
Stores the result of an arithmetic expression in one or more data items. |
|
Does not perform anything in the program. |
|
Deletes a record from an indexed file or a relative file. |
|
Displays the contents of each operand. |
|
Divides a numeric data item or a literal constant by a data item specified after INTO, and stores the quotient. |
|
Used as an entry point that is specified to call a subprogram with a separate name other than the file name or the program name defined in PROGRAM-ID, when invoking a subprogram. |
|
Enable a nested IF statement to be easily used. |
|
The end of the procedure |
|
Terminates the current paragraph, and passes control to the following procedure. |
|
Terminates the called program, and returns control to the calling program. |
|
In a subprogram, this passes control to the main program, and in the main program, this terminates the program. |
|
Transfers control to another point within the procedure division. |
|
Evaluates a condition, and offers actions depending on the evaluation. |
|
Sets certain values in identifier-1 depending on the data categories described in identifier-1. If the REPLACING phrase is specified, it sets identifier-1 with the value in identifier-2 or literal-1. |
|
Performs various operations such as counting occurrences of a specific character in the string. |
|
Merges sequenced files. |
|
Copies a certain memory area into one or more memory areas. |
|
Multiplies numeric items, and stores the results in the specified data item. |
|
Initiates file processing. |
|
Explicitly transfers control to one or more procedures while the program is running. |
|
Reads the next record from a file for sequential access. |
|
Transmits records to a sorting operation area before starting the sorting operation processing. |
|
Transmits records from a sorting operation area to an output procedure after completing the sorting operation processing. |
|
Replaces an existing record of a file. |
|
Searches a table to find an element that satisfies the given condition. |
|
Specifies INDEX values for table management, or increases or decreases an INDEX item to a specified value. |
|
Receives records from one or more files, and sorts them based on a described key. |
|
Sets the record position to read a sequential record in an indexed file or a relative file. |
|
Temporarily or permanently stops execution of the program. |
|
Makes one data item by combining the partial or complete contents of multiple data item or literals. |
|
Subtracts one numeric item or the sum of numeric items from numeric data items, and stores the result in the data item specified after FROM. |
|
Divides consecutive data in a source field into multiple fields. |
|
Writes a record in an output file. |
|
Converts data to XML format. |
|
COBOL language interface depending on the setting of the XMLPARSE compiler option. |
2.1. ACCEPT
The ACCEPT statement transmits data or system data information to the data area referenced by the specified identifier. It does not perform any editing or error checking on the data.
Data transfer
Transfers data from an input device described in the FROM phrase to a data item referenced by idenfifier-1. If the FROM phrase is omitted, the input device is assumed to be the system input device.
Format 1 is useful for a program in which an operator needs to be involved. The operator must provide a proper message as a response.

The following items are specified in the statement.
-
identifier-1
-
The area to which data is sent.
-
The data item can be the following.
-
Alphanumeric group item
-
Elementary data item whose usage is DISPLAY or DISPLAY-1
-
-
-
mnemonic-name-1
-
Specifies the input device described in the SPECIAL-NAMES paragraph with environment-name.
-
-
environment-name-1
-
Specifies the source of input data, such as CONSOLE or SYSIN.
-
System Date-Related Information Transfer
Transfers system information in the DATE, DATE YYYYMMDD, DAY, DAY YYYYDDD, DAY-OF-WEEK, or TIME data items to the data item referenced by identifier-2. This transfer follows the MOVE statement rules without the CORRESPONDING phrase.
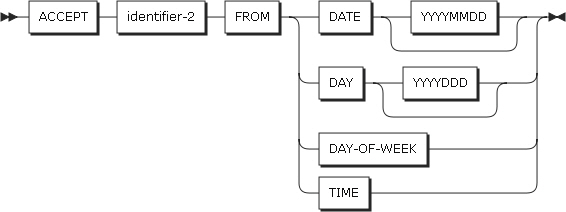
-
identifier-2
-
The data item can be the following.
-
Alphanumeric group item
-
-
Elementary data item that belongs to one of the following categories.
-
alphanumeric
-
alphanumeric-edited
-
numeric-edited (with USAGE DISPLAY)
-
numeric
-
internal floating-point
-
external floating-point (with USAGE DISPLAY)
-
-
Format 2 gets the current date in the 'day of the week' or 'time of day' format. The current date and time can be obtained using the intrinsic function CURRENT-DATE.
DATE, DATE YYYYMMDD, DAY, DAY YYYYDDD, DAY-OF-WEEK, TIME
DATE, DATE YYYYMMDD, DAY, DAY YYYYDDD, DAY-OF-WEEK, and TIME are data items that implicitly contain USAGE DISPLAY.
Item | Description |
---|---|
DATE |
Expressed as PICTURE 9(6). The first two digits indicate the year, the next two indicate the month, and the last two indicate the day. For instance, April 30th in the year 2012 is 120430. |
DATE YYYYMMDD |
Expressed as PICTURE 9(8). Expresses the year in four digits. The first four digits indicate the year, the next two indicate the month, and the next two indicate the day. For instance, April 30 in the year of 2012 is 20120430. |
DAY |
Expressed as PICTURE 9(5). The first two digits indicate the year and the next three indicate the day. The three digit day is the number of days since January 1. For instance, April 30 in the year 2012 is 12121. |
DAY YYYYDDD |
Expressed as PICTURE 9(7). Expresses the year in four digits. The first four digits indicate the year and the next three indicate the day. The three digit day is the number of days since January 1. For instance, April 30 in the year 2012 is 2012121. |
DAY-OF-WEEK |
Expressed as PICTURE 9(1). Expresses the day of the week in numbers. Monday, Tuesday, Wednesday, Thursday, Friday, Saturday, and Sunday represent 1, 2, 3, 4, 5, 6, and 7 respectively. For instance, Wednesday is 3. |
TIME |
Expressed as PICTURE 9(8). Expresses the time. The first two digits indicate the hour, the next two indicate the minutes, the next two indicate the seconds, and the last two indicate the hundredths of seconds. For instance, 2:41 PM is 14410000. |
2.2. ADD
The ADD statement stores the result of addition of two or more operands.
-
Format 1
In format 1, the sum of literal constants or the identifier before TO is added to identifier-2 after TO, and the result is stored in identifier-2. This process repeats from left to right for each identifier specified in identifier-2.
ADD Statement Format 1 -
Format 2
In format 2, the sum of operands specified before GIVING is stored in the data item in identifier-3.
ADD Statement Format 2 -
Format 3
In format 3, elementary data items in identifier-1 are added to those with the same names in identifier-2, and the results are stored in elementary data items in identifier-2.
ADD Statement Format 3
The following items are specified in the statement.
-
identifier
-
For format 1, elementary numeric items must be specified.
-
For format 2, elementary numeric items must be specified except for the identifier described after GIVING. The identifier after GIVING must be either an elementary numeric item or a numeric-edited item.
-
For format 3, alphanumeric group items must be specified.
-
-
literal
-
Numeric literals.
-
-
ROUND phrase
-
For information about the ROUNDED phrase, refer to ROUNDED Phrase.
-
-
SIZE ERROR phrase
-
For information about the SIZE ERROR phrase, refer to SIZE ERROR Phrase.
-
-
CORRESPONDING phrase
-
For information about the CORRESPONDING phrase, refer to CORRESPONDING Phrase.
-
-
END-ADD phrase
-
The END-ADD phrase is used to explicitly mark the scope of the ADD statement. This phrase can be used when the ADD statement is nested in another conditional statement.
-
2.3. CALL
The CALL statement transfers control from one program to another while the program is running. The program that calls another program with the CALL statement is the calling program, and the one that is called by the calling program is the called program.
The called program can also call another program with the CALL statement. For a program to directly or indirectly call itself, the program needs to have been defined with RECURSIVE.
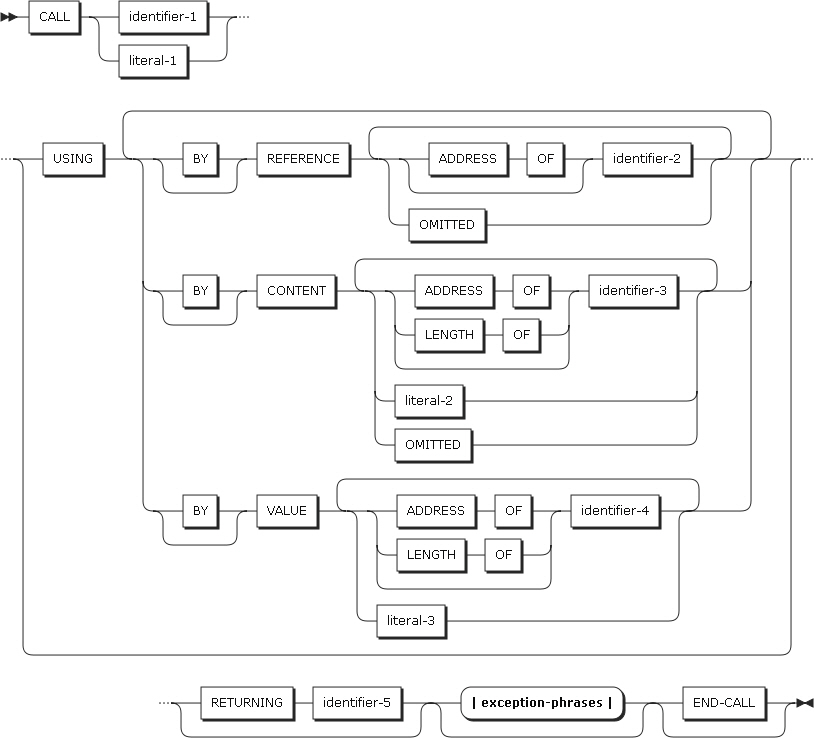


The following items are specified in the statement.
-
identifier-1, literal-1
-
identifier-1 must be defined as an alphanumeric, alphabetical or numeric item to specify a program name.
-
identifier-1cannot be a windowed date field. literal-1 must be an alphanumeric literal.
-
USAGE TYPE must be DISPLAY.
-
To execute the called program at the beginning of the procedure division, the data in literal-1 or identifier-1 must specify the program name as defined in the identification division of the called program.
To execute the called program from a specific part defined by an ENTRY statement in the called program, the name declared in the ENTRY statement must be specified.
-
-
procedure-pointer-1, function-pointer-1
-
Must be a data item that has a valid program entry point.
-
-
USING phrase
-
Specifies arguments to pass to the called program.
The number of arguments in the procedure division of the called program or that of the ENTRY statement must be identical to that of the USING phrase. The arguments are transferred in order. The value of the arguments declared by the USING phrase are valid while the called program is being executed.
-
The BY CONTENT, BY REFERENCE, and BY VALUE phrases are applied until another BY CONTENT, BY REFERENCE, and BY VALUE phrases appears.
If BY CONTENT or BY VALUE is not specified, the default is BY REFERENCE.
-
-
BY REFERENCE phrase
-
The BY REFERENCE phrase passes the address value of the data item as an argument, rather than passing a copy of the data from the calling program. If the value of the argument passed from the called program to the calling program is modified, the modified value will remain even after the called program terminates.
-
The following items are specified in the phrase.
Item Description identifier-2
Any data item of any level defined in the data division can be specified.
file-name-1
File name for a QSAM file
ADDRESS OF identifier-2
identifier-2 must be a data item of level-01 or level-77 defined in the linkage section.
OMITTED
Does not pass any data item as an argument.
-
-
BY CONTENT phrase
-
The BY CONTENT phrase copies a value of an argument in a temporary point to pass it from the calling program to the called program. Even if the called program modifies a data value of an argument, data in the calling program will not change.
-
The following items are specified in the phrase.
Item Description identifier-3
Any data item of any level defined in the data division can be specified.
literal-2
Can be an alphanumeric literal, figurative constant, or DBCS literal.
LENGTH OF special register
For information about the LENGTH OF special register, refer to the "LENGTH OF" special register in COBOL Words.
ADDRESS of identifier-3
identifier-3 must be a data item of level-66 or level-88 defined in the linkage section, the working-storage section, or the local-storage section.
OMITTED
Does not pass any data item as an argument.
-
-
BY VALUE phrase
-
The BY VALUE phrase passes the values of data as arguments from the calling program to the called program. Thus, the called program cannot modify data of the calling program.
-
The BY VALUE phrase was primarily introduced for the interface between COBOL programs and non-COBOL languages (for example, the C language), but it can also be used between COBOL programs.
-
The following items are specified in the phrase.
Item Description identifier-4
Data items, not group items defined in the data division.
One of the following can be specified.
-
Binary (USAGE BINARY, COMP, COMP-4, COMP-5)
-
Floating Point (USAGE COMP-1, COMP-2)
-
Function-pointer (USAGE FUNCTION-POINTER)
-
Pointer (USAGE POINTER)
-
Procedure-pointer (USAGE PROCEDURE-POINTER)
-
One single-byte alphanumeric character (such as PIC X or PIC A)
If the BY VALUE phrase is also used, the following are available.
-
Reference-modified item of usage display and length 1
-
SHIFT-IN and SHIFT-OUT special registers
-
LINAGE-COUNTER special register when this has usage binary
ADDRESS OF identifier-4
Data items except level-66 or level-88 defined in the linkage-section, the working-storage section, or the local-storage section.
LENGTH OF special register
If a LENGTH OF special register is used, BY VALUE must be specified as a PIC 9(9) binary type.
literal-3
One of the following can be specified.
-
A numeric literal
-
A Figurative constant ZERO
-
A one-character alphanumeric literal
-
A symbolic character
-
A single-byte Figurative constant (SPACE, QUOTE, HIGH-VALUE, LOW-VALUE)
The following rules apply.
-
ZERO is treated as a numeric value (a fullword binary zero is passed.).
-
If a fixed-point numeric literal, it has 9 or fewer digit precision.
-
If a floating-point numeric literal, an 8 byte internal floating-point (COMP-2) value is passed.
-
-
-
RETURNING phrase
-
The following items are specified in the phrase.
Item Description identifier-5
identifier-5 can be any data item defined in the data division. The return value specified by the called program is stored.
-
If the called program has been written in COBOL or C, the RETURNING phrase of the CALL statement can be specified. To specify the RETURNING phrase in a CALL statement that calls a sub-program written in COBOL, note the following.
-
The called program must specify the RETURNING phrase in the procedure division.
-
identifier-5 specified in the RETURNING phrase of the calling program and the RETURNING phrase specified in the target program must share the same PICTURE, USAGE, SIGN, SYNCHRONIZE, JUSTIFIED, and BLANK WHEN ZERO clauses. (In the PICTURE clause, the currency sign may be different from each other, and periods and commas can vary based on the DECIMAL POINT IS COMMA clause.)
-
If the returned identifier-5 value is of USAGE INDEX, POINTER, FUNCTION-POINTER, or PROCEDURE-POINTER, it is treated as modified through a SET statement internally. If not, it is internally treated as modified through a MOVE statement .
-
If an EXCEPTION or OVERFLOW occurs, identifier-5 is not modified. identifier-5 must not be reference-modified.
-
RETURN-CODE is not set when executing a CALL statement that includes the RETURNING phrase.
-
-
-
ON EXCEPTION phrase
-
If an exception occurs while the called program is being executed, one of the following things occurs.
-
If the ON EXCEPTION phrase is specified, control is moved to imperative-statement-1.
-
If the ON EXCEPTION phrase is not specified in the CALL statement, NOT ON EXCEPTION, if specified, will be ignored.
-
-
-
NOT ON EXCEPTION phrase
-
If the called program executed successfully, control is moved to imperative-statement-2.
-
-
ON OVERFLOW phrase
-
The ON OVERFLOW phrase performs the same roles as those of the ON EXCEPTION phrase.
-
-
END-CALL phrase
-
The END-CALL phrase explicitly specifies that the CALL statement ends.
-
2.4. CANCEL
The CANCEL statement is used to process a referenced program in its initial state the next time when it is called.
If a COBOL program calls a sub-program with the CALL statement, the data values that have been used while the CALL statement is valid are stored in data items of the sub-program, even after the CALL statement terminates. The CANCEL statement initializes the values of data items in the sub-program, except when variables declared in the EXTERNAL clause are used. When calling a sub-program with the CALL statement, and calling the CALL statement again after calling the CANCEL statement, it is possible to use the sub-program as if it is being called for the first time.
After the CANCEL statement is called, all sub-programs referenced by the CANCEL statement are also canceled. The CANCEL statement is effectively executed for each sub-program.
The CANCEL statement automatically closes all files opened by the specified program.
It is also possible for a sub-program called with the CALL statement to execute the CANCEL statement. However, that CANCEL statement can affect program itself or programs that called it.
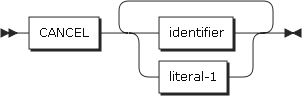
The following items are specified in the statement.
-
identifier-1, literal-1
-
literal-1 and identifier-1 must specify a program-name.
-
literal-1 must be an alphanumeric literal, and identifier-1 must be an alphanumeric, alphabetic or zoned decimal data item.
-
2.5. CLOSE
The CLOSE statement terminates file processing. If the FILE STATUS clause is described in the file-control entry, the CLOSE statement is executed, and the file status key is updated to the corresponding value.
-
Format 1
REEL, UNIT, and NO REWIND phrases are not available for VSAM files.
CLOSE Statement Format 1 -
Format 2
CLOSE Statement Format 2 -
Format 3
CLOSE Statement Format 3
The following items are specified in the statement.
-
file-name-1
-
The file name used in the OPEN statement must be specified. The organization and access mode for each file does not need to be the same to specify multiple files.
-
Sort and merge files cannot be used.
-
-
REEL, UNIT
-
The attributes of the device where the files are stored.
-
-
WITH NO REWIND, FOR REMOVAL
-
Only apply to tape files. If these are set for a device to which they cannot apply, the CLOSE statement will still execute successfully, and the file status will be set to a value which indicates that the device is not a tape file.
-
2.6. COMPUTE
The COMPUTE statement stores the result of an arithmetic expression in one or more data items. There is no restriction on the combination of arithmetic expressions (including addition, subtraction, multiplication, and division) specified in a COMPUTE statement.
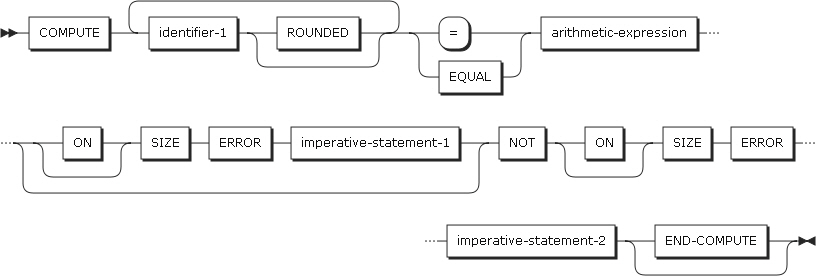
The following items are specified in the statement.
-
identifier-1
-
identifier-1 must be an elementary numeric item, elementary numeric-edited item, or elementary floating-point item.
-
-
arithmetic-expression
-
Any arithmetic expression that includes addition, subtraction, multiplication, and division can be specified.
-
After the COMPUTE statement is executed, the result of the arithmetic expression is computed, and it is stored in a data item specified in identifier-1.
-
If an arithmetic expression consists of a single identifier, numeric function, or literal, the COMPUTE statement functions in the same way as moving the identifier, numeric function, or literal to identifer-1.
-
-
ROUNDED phrase
-
For information about the ROUNDED phrase, refer to ROUNDED Phrase.
-
-
SIZE ERROR phrase
-
For information about the SIZE ERROR phrase, refer to SIZE ERROR Phrase.
-
-
END-COMPUTE phrase
-
The END-COMPUTE phrase is specified to explicitly express the scope of the COMPUTE statement.
-
2.7. CONTINUE
The CONTINUE statement does not perform any action in the program. It indicates that there is no statement to be executed.
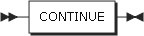
2.8. DELETE
The DELETE statement deletes a record in a file.
To use the DELETE statement, the file must be opened in I-O mode. After the DELETE statement is successfully executed, the record is removed, and can no longer be used.
If FILE STATUS is described in the file-control entry, the file status key will be updated after the DELETE statement is executed.
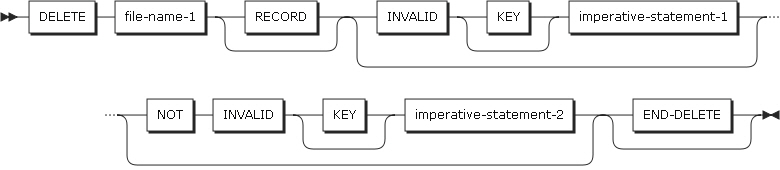
The following items are specified in the statement.
-
file-name-1
-
Described in an FD entry of the data division, and must be the name of an indexed file or a relative file.
-
-
END-DELETE phrase
-
Explicitly indicates the end of the DELETE statement.
-
Each mode of the DELETE statement is described as follows:
-
Sequential access mode
-
The READ statement must successfully be executed before the DELETE statement is executed. After the DELETE statement is executed, the system removes the record that was previously retrieved by the READ statement.
-
The INVALID KEY and NOT INVALID KEY phrases must not be used for a file in sequential access mode.
-
-
Random or dynamic access mode
-
In random or dynamic access mode, the execution result of the DELETE statement depends on the file organization (indexed or relative).
-
When the DELETE statement is executed, the system removes the record depending on the prime RECORD KEY data item of an indexed file, or RELATIVE KEY data item of a relative file.
-
If the file does not contain the record, an invalid key condition occurs.
-
2.9. DISPLAY
The DISPLAY statement is used to transmit the contents of the operands to the output device. The contents are shown from left to right in the order in which the operands are arranged.

The following items are specified in the statement.
-
identifier-1
-
identifier-1 references the data to be displayed on the output device.
-
identifier-1 can be specified with any data item except USAGE INDEX, PROCEDURE-POINTER, and FUNCTION-POINTER. If identifier-1 is a binary, internal decimal, or internal floating-pointer, identifier-1 is automatically converted to an external format.
-
Binary and internal decimal items are converted into zoned decimal numbers.
-
Internal floating-point numbers are converted into external floating-point numbers as follows:
-
A COMP-1 item is displayed as an external floating-point with a PICTURE clause of -.9(7)E-99.
-
A COMP-2 item is displayed as an external floating-point with a PICTURE clause of -.9(15)E-99.
-
-
-
A data item defined with USAGE POINTER is converted to a zoned decimal number with PIC 9(10). Other categories do not need to be converted. DBCS and non-DBCS operands can be used together in a DISPLAY statement.
-
-
literal-1
-
Can be specified with any literal or figurative constant. Only a single occurrence is displayed for a figurative constant.
-
-
UPON
-
environment-name-1 or mnemonic-name-1 must be related to the output device. (For more information about environment-name-1 or mnemonic-name-1, refer to SPECIAL-NAMES Paragraph.)
-
If the UPON phrase is omitted, the default system output device (for example, stdout) is used.
-
-
WITH NO ADVANCING
-
If this phrase is specified, the contents of the operands are transferred to the output device with the DISPLAY statement, and then the position of the device will stay the same instead of moving to the leftmost position of the next line.
-
OFCOBOL does not directly control the output device, so the WITH NO ADVANCING phrase may not have any effect. (e.g. UPON CONSOLE) |
2.10. DIVIDE
The DIVIDE statement divides a numeric data item or a literal constant by the data item specified after INTO, and stores the quotient.
-
Format 1
Format 1 performs a division operation with identifier-1 or literal-1 as the divisor and identifier-2 as the dividend, and stores the quotient in identifier-2. If multiple items are specified for identifier-2, the division operation is repeatedly performed from left to right, following the order in which identifier-2 is specified, and each quotient of the operation is stored in the corresponding identifier-2.
DIVIDE Statement Format 1 -
Format 2
Format 2 performs a division operation with identifier-1 or literal-1 as the divisor and identifier-2 or literal-2 as the dividend, and stores the quotient in identifier-3.
DIVIDE Statement Format 2 -
Format 3
Format 3 performs a division operation with identifier-1 or literal-1 as the dividend and identifier-2 or literal-2 as the divisor, and stores the quotient in identifier-3.
DIVIDE Statement Format 3 -
Format 4
Format 4 performs a division operation with identifier-1 or literal-1 as the divisor and identifier-2 or literal-2 as the dividend, and stores the quotient and the remainder in identifier-3 and identifier-4.
DIVIDE Statement Format 4 -
Format 5
Format 5 performs a division operation with identifier-1 or literal-1 as the dividend and identifier-2 and literal-2 as the divisor, and stores the quotient and the remainder in identifier-3 and identifier-4.
DIVIDE Statement Format 5
The following items are specified in the statement.
-
identifer-1, identifier-2
-
identifier-1 and identifier-2 must be elementary numeric data items.
-
-
identifier-3, identifier-4
-
identifier-3 and identifier-4 must be either elementary numeric or numeric-edited items.
-
-
literal-1, literal-2
-
literal-1 and literal-2 must be numeric literals.
-
-
ROUNDED phrase
-
For more information about format 1, 2 and 3, refer to Statement Operations of ROUNDED Phrase.
-
For format 4 and 5, the quotient used to get the remainder is stored in an intermediate value, and the value is truncated, not rounded.
-
-
REMAINDER phrase
-
The result of subtracting the value of multiplying the quotient by the divisor from the dividend is stored in identifier-4. If identifier-3 is a numeric-edited item, an intermediate value that has not yet been edited is used as the quotient to calculate the remainder.
-
If a floating-point is used as an operand or a receiver, the REMAINDER phrase cannot be used.
-
-
SIZE ERROR phrase
-
For more information about format 1, 2 and 3, refer to Statement Operations of SIZE ERROR Phrase.
-
For format 4 and 5, if a size error occurs in the quotient, the calculation for the remainder is not meaningful. Thus, the existing values will remain in identifier-3 and identifier-4.
-
If a size error occurs in the remainder, the existing value remain in identifier-4.
-
-
END-DIVIDE phrase
-
The END-DIVIDE phrase is used to explicitly specify the scope of the DIVIDE phrase.
-
A floating-point can be used as a data item for format 1, 2 and 3, but cannot be used for format 4 and 5. |
2.11. ENTRY
The ENTRY statement is used as an entry point that is specified when calling a subprogram with a separate name other than the file name or the program name defined in PROGRAM-ID. The ENTRY statement cannot be specified for programs where the RETURNING phrase is specified in the procedure division or programs which are nested.
When a CALL statement calls a sub-program with the name specified in the ENTRY statement, the statement following the ENTRY statement is performed.
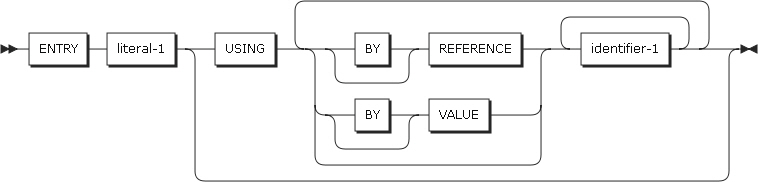
-
literal-1
-
The entry name called by a CALL statement. An alphanumeric literal must be specified, and it must follow the program naming rules.
-
Entry names must be unique in a program, and a figurative constant must not be specified.
-
literal-1 must not be duplicated with another entry name within the program.
-
-
USING phrase
-
For more information about the USING phrase, refer to CALL.
-
2.12. EVALUATE
The EVALUATE statement enables an easy way to write nested IF statements. The EVALUATE statement can evaluate multiple conditions. The actions are determined by the result of the evaluations.
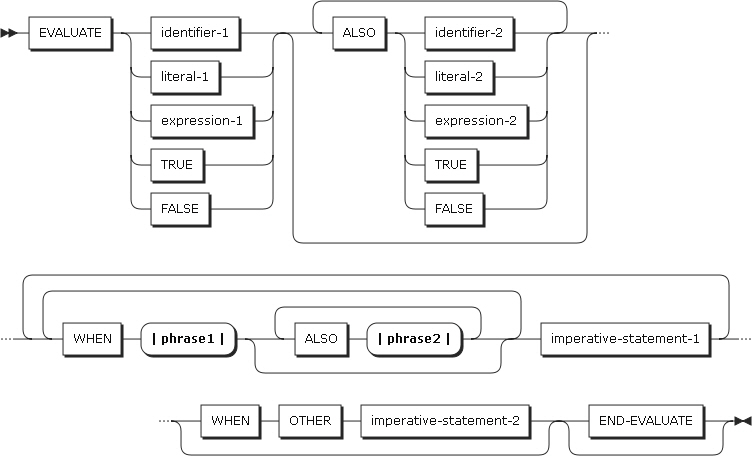
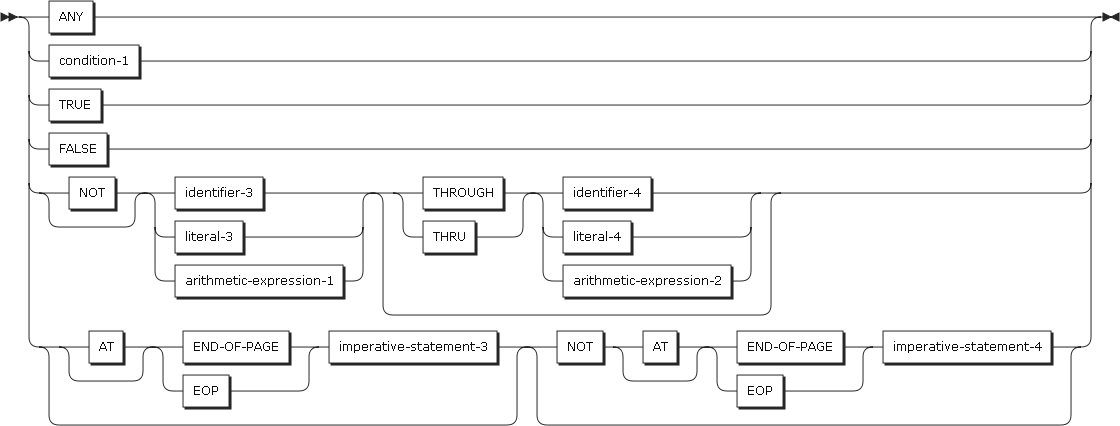
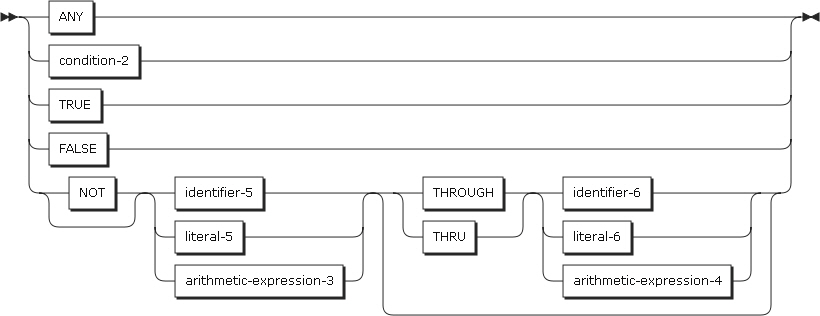
The following items are specified in the statement.
-
Operands before the WHEN phrase
-
Each operand is called a selection subject.
-
As a group the operands are called the set of selection subjects.
-
-
Operands within the WHEN phrase
-
Each operand is called a selection object.
-
As a group the operands are called the set of selection objects.
-
-
ALSO
-
Separates each selection subject or selection object in a set of selection subjects or selection objects.
-
-
THROUGH and THRU
-
Used to specify a value which has a certain scope.
-
Two operands connected with a THRU phrase must be of the same class. The number of selection objects in each set of selection objects must be identical to the number of selection subjects. Based on the following rules, selection objects in each set of selection objects must correspond to selection subjects that are in the same relative position.
-
Identifiers, literals, and arithmetic expressions in selection objects must be valid operands that can be compared with operands in selection subjects.
-
condition-1, condition-2, TRUE, or FALSE as a selection object can be used when the corresponding selection subject is a conditional expression, TRUE, or FALSE.
-
ANY can correspond to any type of selection subject.
Rules for Comparing Selection Subjects and Objects
Selection subjects can be compared with objects as follows:
-
If a selection subject is identifier-1, literal-1, or an arithmetic expression, it is compared with the corresponding selection object that is in the same position in the set of selection objects. If it does not include THRU, an EQUAL comparison is performed, and if it includes THRU, the selection subject will be checked to determine if it is within the scope of selection objects.
-
If a selection subject is a conditional expression, a corresponding selection object in the set of selection objects must be specified as a conditional expression, TRUE or FALSE. If the selection subject is true, the selection object must also be true to satisfy the comparison. If the selection object is false, the comparison is not satisfied. If the selection subject is false, the selection object must be false to satisfy the comparison.
-
If expression-1 and expression-2 are arithmetic expressions and the object is also an arithmetic expression, apply the rule for evaluating a numeric value.
-
If a selection subject is TRUE, a corresponding selection object in a set of selection objects must be specified as a conditional expression, TRUE or FALSE. If the conditional expression of the selection object is TRUE, the comparison must also be TRUE to be satisfied. If the selection object is FALSE, the comparison is not satisfied.
-
If a selection subject is FALSE, a corresponding selection object in a set of selection objects must be specified to a conditional expression, TRUE or FALSE. If the conditional expression of the selection object is FALSE, the comparison is satisfied. If the selection object is TRUE, the comparison is not satisfied.
-
If a selection object is specified with the ANY phrase, the comparison is always satisfied.
-
If a selection object is specified with the NOT phrase, the not equal operation is performed with the subject.
-
If the comparison is satisfied for all selection subjects in a set of selection subjects and all selection objects in a set of selection objects, the WHEN phrase is satisfied.
If the comparison is not satisfied, the following comparison is performed for the WHEN phrase.
-
If there is a satisfying WHEN phrase, statements from imperative-statement-1described in the phrase will be executed.
-
If there is no satisfying WHEN phrase and there is a WHEN OTHER phrase, statements from imperative-statement-2 will be executed. If there is not a WHEN OTHER phrase, the next statement following the EVALUATE statement will be executed.
-
2.13. EXIT
The EXIT statement indicates the end of the procedure.
The EXIT statement plays the same role as the CONTINUE statement in the program. Statements following the EXIT statement are executed.
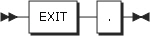
2.14. EXIT PARAGRAPH
Terminates the current paragraph, and passes control to the following procedure.

2.15. EXIT PROGRAM
The EXIT PROGRAM statement terminates the program, and returns control to the calling program. The EXIT PROGRAM statement can be used only in the procedure division of the program.

In the main program and subprograms, the EXIT PROGRAM statement is executed as follows:
-
In the main program, a subprogram is invoked with a CALL statement.
-
If the sub-program encounters an EXIT PROGRAM statement during runtime, control is transferred to the CALL statement.
-
Then, the statement following the CALL statement of the main program is executed.
The EXIT PROGRAM statement must be the last statement, as control changes. If there are no more executable statements in a sub-program, the EXIT PROGRAM statement is implicitly executed.
2.16. GOBACK
In a subprogram, the GOBACK statement passes control to the main program, and in the main program, it terminates the program. This has the same role as the EXIT PROGRAM statement of a subprogram or the STOP RUN statement of a main program. The GOBACK statement indicates the logical end of a subprogram.
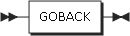
If a GOBACK statement appears in a subprogram, control is transferred back to the main program. The GOBACK statement must be used independently, or must be positioned at the very end of a subprogram. Statements following the GOBACK statement in a subprogram are not executed.
If a subprogram was invoked with a CALL statement by the main program, control is moved to the next statement following the CALL statement of the main program after the subprogram processes the GOBACK statement.
2.17. GO TO
The GO TO statement transfers control to another point within the procedure division.
There are the following two types of GO TO statements.
-
Unconditional GO TO
-
Conditional GO TO
Unconditional GO TO
The unconditional GO TO statement transfers control to a paragraph or a section defined as procedure-name-1.
Control is passed to other procedures or sections of the program by the GO TO statement, so statements that follow the GO TO statement are not executed.

Item | Description |
---|---|
procedure-name-1 |
The name of the procedure or section to which control will be transferred. |
Conditional GO TO
The conditional GO TO statement, if there is a list of procedure-name-1, determines a procedure-name from the list to which control will be moved according to the value of identifier-1.

Item | Description |
---|---|
procedure-name-1 |
A procedure or section that is in the same procedure division as the GO TO statement. Up to 255 procedure-name-1s can be specified. |
identifier-1 |
A numeric data item that is an integer. identifier-1 cannot be a windowed data field. If Identifier-1 is 1, control is passed to the first procedure in the procedure-name list, and if identifier-1 is 2, it is moved to the second procedure in the procedure-name list. If the value of identifier-1 exceeds the number of procedure-names in the list, the GO TO statement will not pass control, and the next statement will be executed. |
2.18. IF
The IF statement evaluates a condition, and performs actions based on the evaluation.

The following items are specified in the statement.
-
condition-1
-
A conditional expression described in Conditional Expressions of the procedure division structure.
-
-
statement-1, statement-2
-
The statements to be executed based on the condition. If the condition is true, statements described in statement-1 of the THEN phrase are executed, or processed by NEXT SENTENCE. If it is false, statements described in statement-2 of the ELSE phrase are executed, or processed by NEXT SENTENCE.
-
This can include nested IF statements. In nested IF statements, a matched IF statement is checked using IF, ELSE, and END-IF. END-IF is matched with the nearest IF statement.
-
-
NEXT SENTENCE
-
Executes a sentence after a period (.).
-
Even if the END-IF statement is specified, the statement after the closest period is executed, rather than executing the next statement following the END-IF statement.
-
-
END-IF phrases
-
Indicates the end of the IF statement.
-
2.19. INITIALIZE
The INITIALIZE statement sets certain values in identifier-1 based on the data categories described in identifier-1. If the REPLACING phrase is specified, it sets identifier-1 with values in identifier-2 or literal-1. It is functionally identical to one or more MOVE statements.
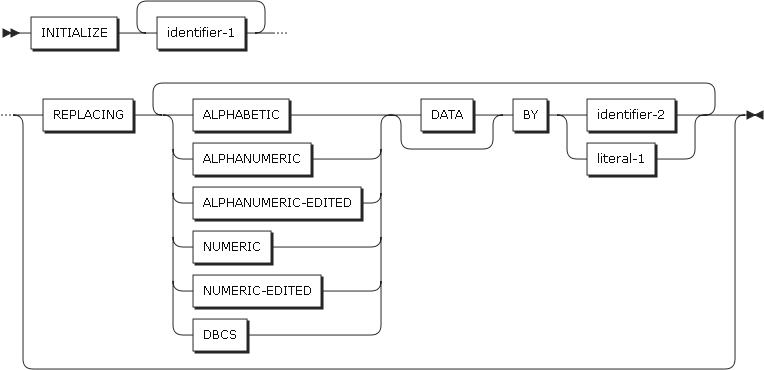
The following items are specified in the statement.
-
identifier-1
-
Must not contain the RENAMES clause.
-
The receiving area where values are set. The following items can be specified.
Item Description Group item
Alphanumeric data. If the REPLACING phrase is specified and identifier-1 is an alphanumeric group item, subordinate items of identifier-1 are initialized according to the rule for the REPLACING clause.
Elementary data item
A data item from the alphabetic, alphanumeric, alphanumeric-edited, DBCS, numeric, numeric-edited, and internal/external floating-point categories.
-
-
identifier-2, literal-1
-
Specifies an elementary data item or a literal that can be specified as a sending area in a MOVE or SET statement.
To specify special registers in identifier-1 and identifier-2, they must be able to be specified as a receiving area or a sending area in each MOVE statement.
-
-
REPLACING phrase
-
If the data category specified in the REPLACING phrase is identical to the category of identifier-1, the value of identifier-2 or literal-1 is set in identifier-1.
-
A floating-point item is treated as it is in the NUMERIC category.
-
The same categories must not be duplicated in a REPLACING phrase.
-
The category validity for identifier-1, identifier-2, and the REPLACING phrase is not internally checked, so the user must specify a data item or category that is appropriate for the MOVE or SET rules. |
If the REPLACING phrase is not specified, the following values can be set in identifier-1.
-
If the category of identifier-1 is alphabetic, alphanumeric, alphanumeric-edited, or DBCS, figurative constant space is set.
-
If the category of identifier-1 is numeric, numeric-edited, or external floating-point, figurative constant zero is set.
-
If the category of identifier-1 is a pointer, figurative constant null is set.
2.20. INSPECT
The INSPECT statement performs the following by examining characters or character groups.
-
Counts occurrences of a specific character in a string (format 1 and 3).
-
Counts occurrences of a specific character and replaces the character with a specified character (format 2 and 3).
-
Replaces specific characters with given characters.
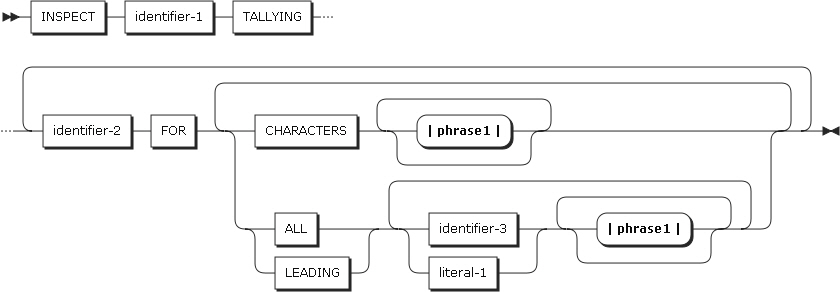

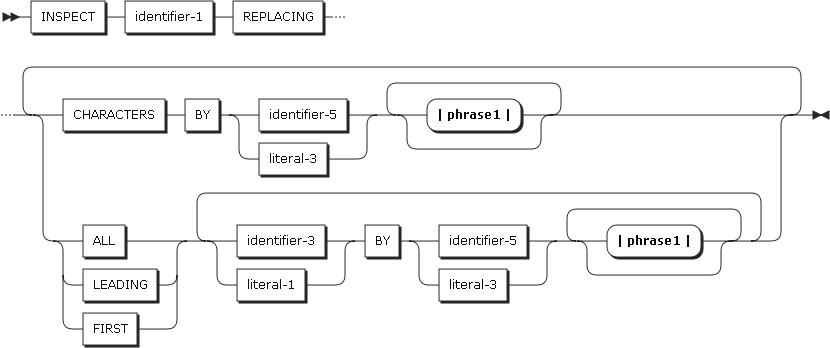

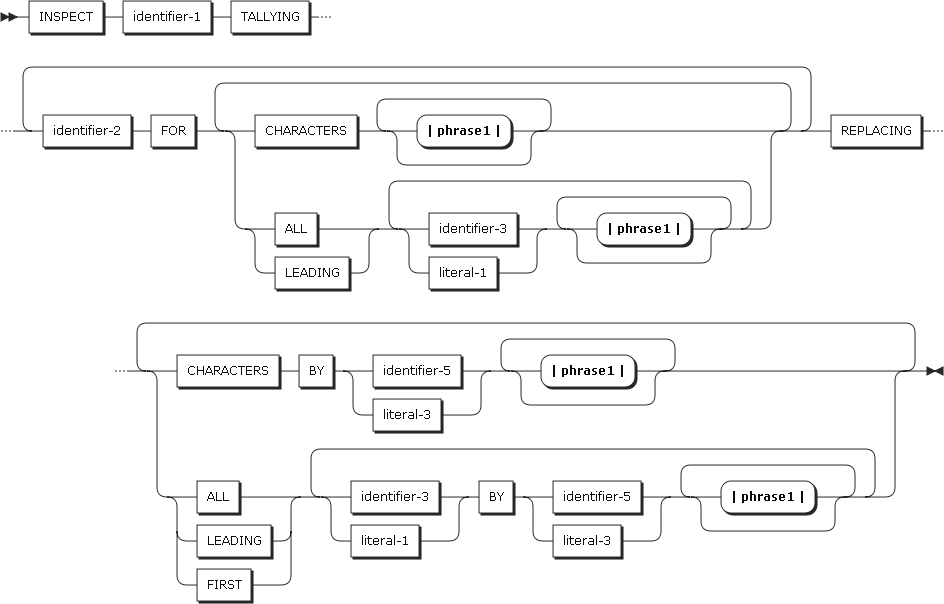

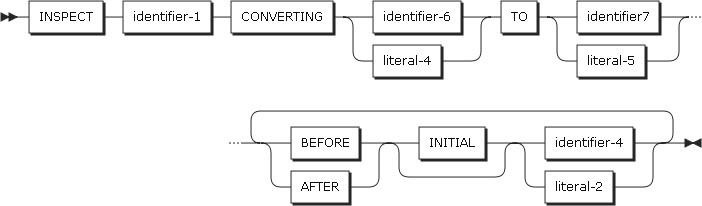
The following items are specified in the statement.
-
identifier-1
-
An alphanumeric group item, elementary item with USAGE DISPLAY or DISPLAY-1, or a special register of alphanumeric type.
-
If USAGE matches, data can be of any category.
-
-
identifier-3, identifier-4, identifier-5, identifier-6, identifier-7
-
An elementary item of USAGE DISPLAY or DISPLAY-1.
-
-
literal-1, literal-2, literal-3, literal-4
-
Must be an alphanumeric literal or a DBCS literal. Each literal has the same category as identifier-1.
-
When identifier-1 is of usage DISPLAY, a figurative constant that does not start with the word ALL can be specified.
-
All identifiers except for identifier-2 have the same USAGE type as identifier-1.
-
TALLYING phrase (format 1, 3)
-
The TALLYING phrase counts occurrences of a specific character.
-
identifier-2 is the COUNT field and must be an elementary integer item without the symbol P in its PICTURE string. identifier-3 or literal-1 is the tallying field.
Item Description CHARACTERS
The count field (identifier-2) is increased by 1 for each character, including a space, in all identifier-1 items.
ALL
The count field (identifier-2) is increased by 1 for each nonoverlapping occurrence when comparing identifier-3 or literal-1 with a character in identifier-1 from the leftmost character position to the rightmost character position.
LEADING
The count field (identifier-2) is increased by 1 for each contiguous nonoverlapping occurrence when comparing identifier-3 or literal-1 with a character in identifier-1. The comparison starts in the position of the first occurrence as many as the number of contiguous occurrences.
-
-
REPLACING phrase (format 2, 3)
-
The REPLACING phrase replaces specific characters in a data item with given characters.
-
identifier-3 or literal-1 is the subject item to be replaced, and identifier-5 or literal-3 is the item that replaces the subject item. The length of subject and substitution items must be the same.
Item Description CHRACTERS BY
The substitution item replaces each character in the subject item starting from the leftmost to the rightmost character position. The substitution item length must be one character position in length.
ALL
The substitution item replaces each nonoverlapping occurrence of the subject item starting from the leftmost to the rightmost character position.
LEADING
The substitution item replaces each contiguous nonoverlapping occurrence of the subject item. The comparison starts in the position of the first occurrence as many as the number of contiguous occurrences.
FIRST
The substitution item replaces the leftmost occurrence of the subject item.
-
-
Replacement Rule
-
The following replacement rules apply.
-
If the subject item is a figurative constant, a substitution item replaces each character that is equivalent to the figurative constant.
-
If the subject and substitution items are character strings, the character string in the substitution item replaces each nonoverlapping occurrence of the subject item.
-
-
-
BEFORE and AFTER phrases (all format)
-
identifier-4 and literal-2 are delimiters. Delimiters are not counted or replaced.
-
INITIAL is the first occurrence of the specified item.
Item Description BEFORE
Counting or replacing is performed from the leftmost to the first occurrence of the delimiter. If no delimiter is encountered, the operation continues to the rightmost position.
AFTER
Counting or replacing is performed from the first character position to the right of the delimiter to the rightmost position. If no delimiter is encountered, the operation is not performed.
-
-
CONVERTING phrase (format 4)
-
The CONVERTING phrase replaces specific characters in a data item with the given characters.
-
identifier-6 or literal-4 specifies the character string to be replaced, and identifier-7 or literal-5 specifies the replacement character string. The length of the replaced and replacement strings must be the same.
-
2.21. MERGE
The MERGE statement merges sequenced files. Records of each file must be sorted according to the keys.
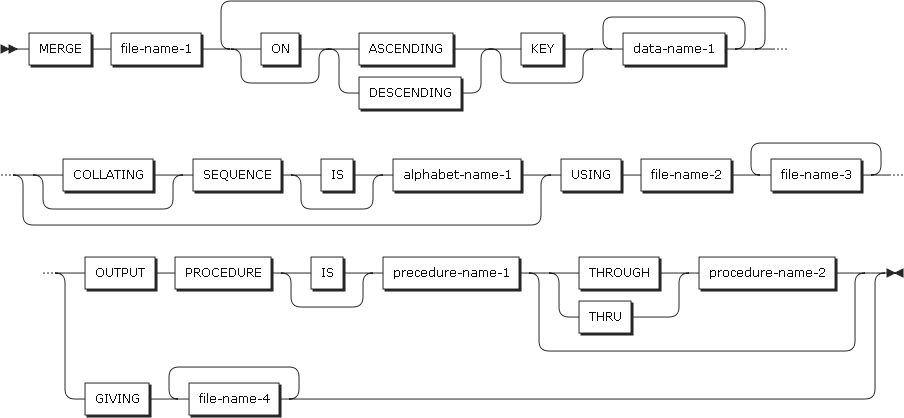
The following items are specified in the statement.
-
file-name-1
-
The name described in the SD entry.
-
-
ASCENDING KEY and DESCENDING KEY phrases
-
Specifies an ascending or descending order for the merge keys.
Item Description data-name-1
Specifies a KEY data item to be used in the MERGE statement. data-name-1 must be a data item included in a record for file-name-1.
The first specified key is the major key, and the next data item is the second major key.
-
A KEY data item must be located in the same position and share the same data format in each input file. It does not need to have the same data-name.
-
If one or more records are described in file-name-1, the KEY data items need to be described in one of the records.
-
KEY data items cannot include an OCCURS clause, and cannot belong to an item containing an OCCURS clause.
-
KEY data items can be qualified.
KEY data items can be one of the following data categories.
-
Alphabetical, alphanumeric, alphanumeric-edited
-
Numeric
-
Numeric-edited (with usage DISPLAY)
-
Internal floating-point or display floating-point
-
-
-
-
COLLATING SEQUENCE phrase
-
Specifies the collating sequence used in alphanumeric comparison of key data items in a merge statement.
Item Description alphabet-name-1
Must be defined in the ALPHABET clause of the SPECIAL-NAMES paragraph.
-
-
USING phrase
-
Specifies the input files.
Item Description file-name-2,…
Specifies the input files. If a USING phrase is used, all records of input files(file-name-2,…,) are automatically transmitted to file-name-1.
All input files must be described in the FD entries of the data division.
-
-
GIVING phrase
-
Specifies the output files.
Item Description file-name-3,…
Specifies the output files.
If a GIVING phrase is used, all sorted records are automatically transmitted to the output files. All output files must be described in the FD entries of the data division.
-
-
OUTPUT PROCEDURE phrase
-
Specifies the procedure name to select and modify output records after the merge operation.
Item Description procedure-name-1
Specifies the first (or unique) section or paragraph of the output procedure.
procedure-name-2
Specifies the last section or paragraph of the output procedure.
-
Because the OUTPUT PROCEDURE phrase includes a RETURN statement, records are transmitted one at a time from the file referenced by file-name-1. Various phrases like OPEN, WRITE, and CLOSE can be used to select, copy, or modify output records.
-
After a MERGE statement is executed, all records included in file-name2, file-name3, … are merged according to the specified keys. |
2.22. MOVE
The MOVE statement copies one memory area into one or more other memory areas.
There are two kinds of formats to describe a MOVE statement.
-
Format 1
MOVE Statement Format 1 -
Format 2
MOVE Statement Format 2
The following items are specified in the statement.
-
identifier-1, literal-1
-
identifier-1 and literal-1 are the sending areas.
-
-
identifier-2
-
identifier-2 is the receiving area. An intrinsic function cannot be specified.
Item Description Format 1
For identifier-1, alphanumeric group items or elementary items can be specified.
If multiple identifier-2s are specified, items are copied from identifier-2 to identifier-1 according to the specified sequence.
Format 2
Both identifier-1 and identifier-2 must be group items.
The items in identifier-1 are copied to identifier-2 according to the CORRESPONDING rules.
-
Any length, subscripting, and reference-modification of identifier-2 is evaluated before the first item is copied.
-
-
CORR is an abbreviation of CORRESPONDING.
-
Usage phrase
A data item defined with INDEX, POINTER, FUNCTION-POINTER, or PROCEDURE-POINTER is an elementary item and cannot be specified for identifier-1 or identifier-2.
In the MOVE CORRESPONDING statement format, a data item defined with INDEX, POINTER, FUNCTION-POINTER, or PROCEDURE-POINTER can be a member of an alphanumeric group item. For those data items, no data is copied or moved. The length of the sending or receiving area is determined by the DEPENDING ON phrase.
If identifier-1 contains a reference-modified or a subscription, or if it is an alphanumeric function-identifier, reference-modified, subscripting, or alphanumeric function-identifier of identifier-1 is evaluated before the first item of identifier-2 is copied. If a sending area and a receiving area overlap, the result is unpredictable.
Elementary move
An elementary move is a move in which the receiving item is an elementary data item, and the sending item is an elementary data item or a literal.
The following data categories can be described for sending items and receiving items.
-
Data catalogs or literals that can be specified
-
Data catalogs or literals that can be specified
-
Alphanumeric data items, alphanumeric functions, alphanumeric literals, ALL alphanumeric-literals, and figurative constant (excluding NULL)
-
Alphanumeric-edited data items
-
DBCS data items, DBCS literals, and ALL DBCS-literals
-
External floating-point data items and floating-point literals
-
Internal floating-point data items
-
Numeric data items, numeric literals, and ZEROs
-
Numeric-edited data items
If a receiving item is one of the following, the rules described apply. For information about alignment rules, refer to PICTURE Clause.
-
Alphabetic
-
Data conversion does not occur.
-
Data alignment is done according to the alignment rules, and space padding or data truncation occurs.
-
If the length of the sending item is different from that of the receiving item, truncation occurs based on the description in the JUSTIFIED clause of the receiving item. By default, a right truncation is executed. For further information, refer to JUSTIFIED Clause.
-
-
Alphanumeric or alphanumeric-edited
-
Data alignment is done according to the alignment rules, and space padding or data truncation occurs.
-
If the length of the sending item is greater than that of the receiving item, excess characters of the sending item are truncated from the right.
-
If the initial sending item contains a sign, the value without the sign is used. If the sign occupies a separate memory space, the sign character is not copied, and the length of the sending item does not include the length of the sign.
-
-
DBCS
-
Data conversion does not occur.
-
If the length of the sending item is different from that of the receiving item, the sending item is truncated from the right, or DBCS space padding occurs.
-
-
External floating-point
-
If the sending item is a floating-point, the floating-point value is converted to the usage specified in the receiving item, and copied.
-
If the sending item is not a floating-point, the numeric value is converted to an internal floating-point, and then converted to the usage of the receiving item.
-
-
Internal floating-point
-
If the sending item is not an internal floating-point, the value of the sending item is converted to an internal floating-point format.
-
-
Numeric or numeric-edited
-
Decimal point alignment and zero padding are performed according to the alignment rules.
-
If the receiving item is a signed item, the sign of the sending item is located in the sign position of the receiving item. If the sending item is an unsigned item, a plus sign(+) is created and located in the sign position of the receiving item.
-
If the sending item is an alphanumeric or alphanumeric-edited item, the sending item is copied as an unsigned integer.
-
If the sending item is floating-point, data is converted to a fixed-decimal point format according to the picture phrase of the receiving item, and then copied.
-
If the receiving item is a numeric-edited item, the sending item is edited according to the format specified in the picture phrase.
-
If the sending item is a numeric-edited item, numeric literal values except edit signs are copied to the receiving item. If the receiving item is also numeric-edited, data is copied according to the edit signs specified in the picture phrase of the receiving item.
-
If the category of the receiving item is alphanumeric, alphanumeric-edited, or numeric-edited, and the sending item is numeric, the symbol P in the sending item’s picture phrase is treated as a zero.
-
The following table contains the data items that are valid for each category.
-
The column headings are categories of the receiving item.
-
The row headings are categories of the sending item.
Alpha- betic | Alpha- numeric | Alpha- numeric- edited | Numeric | Numeric- edited | External floating- point | Internal floating- point | DBCS | |
---|---|---|---|---|---|---|---|---|
Alphabetic and SPACE |
O |
O |
O |
X |
X |
X |
X |
X(+) |
Alphanumeric and alphanumeric literal |
O |
O |
O |
O(*) |
O(*) |
O(*) |
O(*) |
X |
Alpha- numeric-edited |
O |
O |
O |
X |
X |
X |
X |
X |
Numeric integer, ZERO and integer numeric literal |
X |
O |
O |
O |
O |
O |
O |
X |
Numeric noninteger and noninteger numeric literal |
X |
X |
X |
O |
O |
O |
O |
X |
Numeric-edited |
X |
O |
O |
O |
O |
O |
O |
X |
Floating -point (floating-point literal, external floating-point data items and internal floating-point data items) |
X |
X |
X |
O |
O |
O |
O |
X |
DBCS |
X |
X |
X |
X |
X |
X |
X |
O |
(*) Figurative constants and alphanumeric literals must only consist of numeric characters. (+) The figurative constant SPACE can be used. |
Group move
A group move is a move in which an alphanumeric group item is a sending or receiving item, or both the sending item and the receiving item are group items. In a group move, data is copied in the same way as data that is copied from an alphanumeric item to an alphanumeric elementary item. Data is not converted.
-
If the receiving item is an alphanumeric group item, the sending item can be one of the following.
-
A valid elementary data item
-
A literal or constant
-
A figurative constant
-
-
If a sending item is an alphanumeric group item, the receiving item can be one of the following.
-
A valid elementary data item
-
An alphanumeric group item
-
2.23. MULTIPLY
The MULTIPLY statement multiplies numeric items and stores the results in the specified data item.
-
Format 1
In format 1, identifier-1 or literal-1 is multiplied by identifier-2, and the result is stored in identifier-2.
If multiple identifier-2s are specified, a multiplication operation between identifier-2 and identifier-1 or literal-1 is repeated from left to right, and the results are stored in identifier-2.
MULTIPLY Statement Format 1 -
Format 2
In format 2, identifier-1 or literal-1 is multiplied by idetifier-2, and the result is stored in identifier-3.
MULTIPLY Statement Format 2
The following items are specified in the statement.
-
identifier-1, identifier-2
-
identifier-1 and identifier-2 must be elementary numeric data items.
-
-
literal-1, literal-2
-
literal-1 and literal-2 must be numeric literals.
-
-
identifier-3
-
identifier-3 must be an elementary numeric data item or an elementary numeric-edited data item.
-
-
ROUNDED phrase
-
For more information about the ROUNDED phrase, refer to ROUNDED Phrase.
-
-
SIZE ERROR phrase
-
For more information about the SIZE ERROR phrase, refer to SIZE ERROR Phrase.
-
-
END-MULTIPLY phrase
-
The END-MULTIPLY phrase is used to explicitly specify the scope of the MULTIPLY statement.
-
2.24. OPEN
The OPEN statement initiates file processing.
-
Format 1
OPEN Statement Format 1 -
Format 2
OPEN Statement Format 2 -
Format 3
OPEN Statement Format 3
The following items are specified in the statement.
-
INPUT
-
Opens input files.
-
-
OUTPUT
-
Opens output files.
-
Can be used for newly created files. If the file for which the OUTPUT phrase is to be used contains records, the existing records are removed to open the file.
-
-
I-O
-
Opens both input and output files.
-
-
EXTEND
-
Opens an output file to append to existing records or to create a new file.
-
If the file already exists, records are added to the existing records, and if not, a new file is created.
-
-
file-name-1, file-name-2, file-name-3 , file-name-4
-
Describes files to be opened.
-
Must be described in the FD entry.
-
-
REVERSED, NO REWIND
-
Only applies to tape files.
-
General Rules
The OPEN INPUT statement or the OPEN I-O statement sets the file position indicator on the position of the first record.
If the EXTEND phrase is specified, the OPEN statement sets the file position after the last record of the file. Successive WRITE statements add records as if the file had been opened with OUTPUT. The EXTEND phrase can be specified for a newly created file.
For VSAM files, if there is no record in the file, the file position indicator is set so that the execution result of the READ statement of format 1 causes an AT END condition.
The following tables show the relationship between OPEN modes and the available input/output statements.
-
Permissible statements for sequential files
Statement Input Open Mode Output Open Mode I/O Open Mode Extend Open Mode READ
X
X
WRITE
X
X
REWRITE
X
-
Permissible statements for indexed and relative files
Fille Access Mode Statement Input Open Mode Output Open Mode I/O Open Mode Extend Open Mode Sequential
READ
X
X
WRITE
X
X
REWRITE
X
START
X
DELETE
X
Random
READ
X
X
WRITE
X
X
REWRITE
X
START
DELETE
X
Dynamic
READ
X
X
WRITE
X
X
X
REWRITE
X
START
X
X
DELETE
X
-
Permissible statements for line-sequential files
Statement Input Open Mode Output Open Mode I/O Open Mode Extend Open Mode READ
X
WRITE
X
X
REWRITE
'X' indicates an allowed statement. |
2.25. PERFORM
The PERFORM statement explicitly transfers control to one or more procedures while the program is running. After the procedure is completed, control is passed to the next statement after the PERFORM statement.
The PERFORM statement can be used in one of the following two ways.
-
out-of-line PERFORM statement
An out-of-line PERFORM statement does not describe a statement in the PERFORM statement, but calls the described procedure. The procedure name must be specified.
-
in-line PERFORM statement
An in-line PERFORM statement does not specify the procedure-name, but describes a statement in the PERFORM statement. The END-PERFORM phrase must be specified to mark the end of the statement.
There are four types of PERFORM statements as follows:
Basic PERFORM Statement
The basic PERFORM statement executes the called procedures once. When the procedures called by PERFORM are completed, statements after the PERFORM statement are processed. A PERFORM statement cannot invoke itself to be executed.
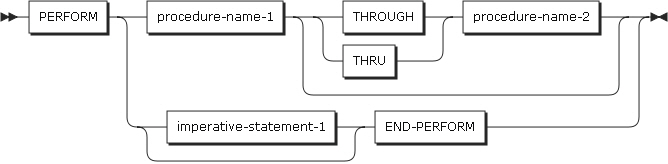
The following items are specified in the statement.
-
procedure-name-1, procedure-name-2
-
Both procedure-name-1 and procedure-name-2 must be the procedure names used in the procedure division.
-
When both procedure-name1 and procedure-name-2 are used, if one of them is a procedure defined in a declarative procedure, the other must also be defined in the same declarative procedure.
-
If only procedure-name-1 is used, imperative-statement-1 and END-PERFORM cannot be specified.
-
If procedure-name-1 is omitted, imperative-statement-1 and END-PERFORM must be specified.
-
-
imperative-statement-1
-
Statement to be executed by the PERFORM statement.
-
When an out-of-line PERFORM statement is executed, control is passed to the first statement of procedure-name-1. When procedure-name-1 completes, control is transferred to the next statement after the PERFORM statement.
After the procedure is performed, control is returned from the following points.
-
If procedure-name-1 is a paragraph, and procedure-name-2 is not specified, control is returned to the PERFORM statement after executing the last statement of procedure-name-1.
-
If procedure-name-1 is a section, and procedure-name-2 is not specified, control is returned to the PERFORM statement after executing the last statement of the final paragraph in the procedure-name-1 section.
-
If procedure-name-2 is a paragraph, control is returned after executing the last statement of the procedure-name-2 paragraph.
-
If procedure-name-2 is a section, control is returned after executing the last statement of the final paragraph in the procedure-name-2 section.
If both procedure-name-1 and procedure-name-2 are specified, the PERFORM statement is executed from procedure-name-1 to procedure-name-2. procedure-name-1 must precede procedure-name-2 in the program order.
A PERFORM statement can be specified in the procedure executed by a PERFORM statement.
PERFORM Statement with TIMES Phrase
In a PERFORM statement with the TIMES phrase, the procedures are called or imperative-statements are executed as many times as the number specified by the value of identifier or integer in the TIMES phrase.
After the PERFORM statement is completed, control is passed to the next statement after the PERFORM statement. If procedure-name-1 is specified, imperative-statement-1 and END-PERFORM cannot be specified.
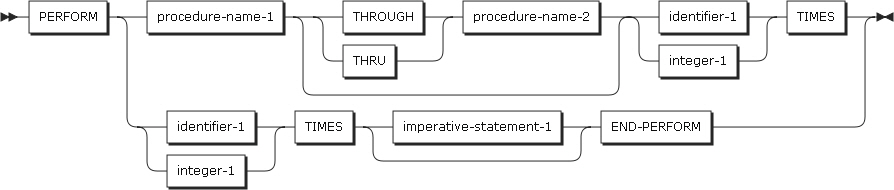
The following items are specified in the statement.
-
identifier-1
-
Specifies an integer item.
-
If identifier-1 is negative or zero, rather than positive, the PERFORM statement is not executed, and the statement following the PERFORM statement is performed.
-
After the PERFORM statement has been initiated, changes to identifier-1 do not affect the number of times the procedures are invoked.
-
-
integer-1
-
Must be a positive integer.
-
PERFORM Statement with UNTIL Phrase
The PERFORM statement with UNTIL phrase executes the procedures or imperative statements until the UNTIL phrase is true.
If the condition specified by the UNTIL phrase is true, control is transferred to the next statement following the PERFORM statement. If procedure-name-1 is specified, imperative-statement-1 and END-PERFORM must not be specified.
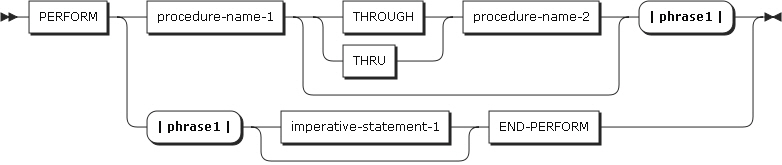

The following items are specified in the statement.
-
condition-1
-
Can be any condition used in OFCOBOL.
-
If the condition of the statement is true, the PERFORM statement is not executed.
-
If the TEST BEFORE phrase is specified, the condition is checked before executing the statement. If the TEST AFTER phrase is specified, the statement is executed at least once before the conditional statement is performed. If neither TEST BEFORE nor TEST AFTER is specified, it is assumed that the TEST BEFORE phrase is specified. If the condition is true, the next executable statement that comes after the PERFORM statement is executed.
PERFORM Statement with VARYING Phrases
The PERFORM statement with VARYING phrases increases or decreases the corresponding variable with one or more variables, and executes the procedures or imperative statements until the condition specified by the variable is true.
If procedure-name-1 is specified, imperative-statement-1 and END-PERFORM must not be specified. If procedure-name-1 is omitted, the AFTER phrase must not be specified.

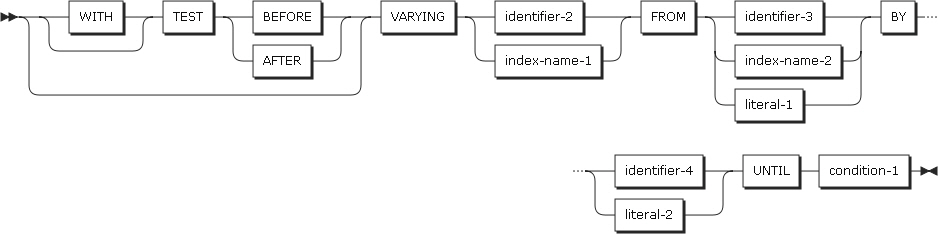
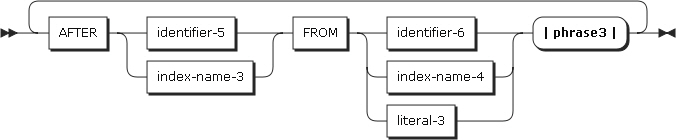

The following items are specified in the statement.
-
identifier-2 through identifier-7
-
Must be a numeric data item.
-
Can be a floating-point data item.
-
-
literal-1 through literal-4
-
Must be a numeric literal.
-
Can be a floating-point data item.
-
-
condition-1, condition-2
-
Can be any condition used in OFCOBOL.
-
If the condition of the statement is true, the PERFORM statement is not executed.
-
Varying Identifiers
Whether or not the TEST BEFORE phrase is specified, each corresponding condition must be checked before executing the statement. If the TEST AFTER phrase is specified, the statement must be executed at least once before performing the conditional statement. If neither TEST BEFORE nor TEST AFTER is specified, it is assumed that the TEST BEFORE phrase is specified.
-
When varying one identifier,
-
If identifier-2 or identifier-5 is subscripted, the value is calculated whenever the data item is set or modified.
-
If identifier-3, identifier-4, identifier-6, or identifier-7 is subscripted, the value is calculated whenever each data value is set or modified.
-
The following figure shows the logic of the PERFORM statement with TEST BEFORE phrase.
Logic of the PERFORM Statement with TEST BEFORE Phrase -
The following figure shows the logic of the PERFORM statement with TEST AFTER phrase.
Logic of the PERFORM Statement with TEST AFTER Phrase
-
-
When varying two identifiers,
-
In the following example, OFCOBOL checks the PERFORM statement in the following sequence.
-
Initializes IDENTIFIER-2 and IDENTIFIER-5 to IDENTIFIER-3 and IDENTIFIER-6 respectively.
-
Processes the following according to the result of condition-1 evaluation.
-
If condition-1 is true, the PERFORM statement is terminated, and the next statement after the PERFORM statement is executed.
-
If condition-1 is false, steps 3 through 7 are executed.
-
-
Processes the following according to the result of condition-2 evaluation.
-
If condition-2 is true, IDENTIFIER-4 is added to IDENTIFIER-2 and IDENTIFIER-5 is set to a current value of IDENTIFIER-6, and then step 2 is repeated.
-
If condition-2 is false, steps 4 through 6 are executed.
-
-
Executes the procedures from procedure-name-1 to procedure-name-2.
-
Increases identifier-5 by identifier-7.
-
Repeats steps 3 through 5 until condition-2 is true.
-
Repeats steps 2 through 6 until condition-1 is true.
When the PERFORM statement completes, IDENTIFIER-5 has the current value of IDENTIFIER-6. IDENTIFIER-2 has a value that is greater than the last-used setting by the increment or decrement value.
-
-
The following figure is the logic of a PERFORM statement that contains TEST BEFORE phrase.
Logic of the PERFORM Statement with TEST BEFORE Phrase -
The following figure shows the logic of a PERFORM statement that contains a VARYING phrase with TEST AFTER phrase.
Logic of the PERFORM Statement with TEST AFTER Phrase
-
-
When varying three identifiers,
-
A PERFORM statement that uses three identifiers is processed in the same way as a statement with two identifiers. It differs only in that when identifier-5 increases by identifier-7, identifier-10 is added to identifier-8, and condition-3 is checked.
-
When the PERFORM statement completes, IDENTIFIER-5 and IDENTIFIER-8 has the current value of IDENTIFIER-6 and IDENTIFIER-9 respectively. IDENTIFIER-2 has a value that is greater than the last-used setting by the increment or decrement value.
-
For varying more than three identifiers, AFTER phrase(s) can be added to the previous example.
-
The following rules apply to the VARYING phrase in the PERFORM statement regardless of the number of identifiers that are specified.
-
When index-name is specified in the VARYING or AFTER phrases.
-
The index-name is initialized, and increased or decreased according to the index-name rules of OFCOBOL.
-
In the FROM phrase, an identifier or a literal must be a positive integer.
-
In the BY phrase, an identifier must be an integer, and a literal must be a nonzero integer.
-
-
When index-name is specified in the FROM phrase.
-
In the VARYING or AFTER phrase, an identifier must be an integer. The identifier is initialized internally by the SET statement.
-
In the BY phrase, an identifier must be a positive integer, and a literal must be a nonzero integer.
-
-
In the BY phrase, an identifier and a literal must be nonzero values.
-
In the VARYING, FROM, or BY phrase, changing the value of an identifier or index-name modifies the number of times that the procedures are executed in the PERFORM statement.
2.26. READ
The READ Statement reads a record from a file.
To execute the READ statement, the related file must be opened in INPUT or I-O mode.
-
Format1
Reads the next record from a file for sequential access.
READ Statement Format 1 -
Format 2
Reads the record of the given key for random access.
READ Statement Format 2
The following items are specified in the statement.
-
file-name-1
-
Must be described in the data vision FD entry.
-
-
NEXT RECORD
-
Reads the next record.
-
This is not necessary for sequential access mode. This does not affect READ statement execution.
-
To sequentially retrieve records in dynamic access mode, the NEXT RECORD phrase has to be specified.
-
-
INTO identifier-1
-
Specifies the field that receives a record.
-
identifier-1 must be a valid receiving item so that record-name-1 can be a sending item according to the MOVE statement rules.
-
identifier-1 and record-name-1 cannot reference the same storage area.
-
For INTO’s identifier-1, the execution result of the a READ statement with INTO phrase is the same as that of the following two statements.
READ file-name-1. MOVE record-name-1 TO identifier-1.
-
-
KEY IS phrases
-
Can only be used for indexed files.
-
data-name-1 must specify a record key for the file related to file-name-1. data-name-1 can be qualified, but cannot be subscripted.
-
-
AT END phrases
-
For sequential access, both the AT END phrase and the EXCEPTION/ERROR procedure can be omitted.
-
-
INVALID KEY phrases
-
Both the INVALID KEY phrase and the EXCEPTION/ERROR procedure can be omitted.
-
-
END-READ phrases
-
Explicitly indicates the end of the READ statement.
-
Multiple Record Processing
If two or more records are specified in file-name-1, they automatically share the same storage area.
Sequential Access Mode
Format 1 can be used for all files in sequential access mode. By executing a format-1 READ statement, the next record can be retrieved from the file. The next record is determined by the file organization.
-
Sequential files
-
Retrieves the next record in a logical order of records.
-
The NEXT RECORD phrase does not need to be specified.
-
AT END condition
If no next record exists, the at-end condition occurs, and the following actions are performed.
-
If the FILE STATUS clause is described in the file-control entry, the result value is stored in the file status key.
-
If the AT END phrase is described in the READ statement, statements described in imperative-statement-1 are executed. Any USE AFTER STANDARD EXCEPTION procedure related to file-name-1 is not executed.
-
If the AT END phrase is not described in the READ statement, and an appropriate USE AFTER STANDARD EXCEPTION procedure exists, the procedure is executed.
Both the AT END phrase and the USE AFTER STANDARD EXCEPTION procedure can be omitted.
If the at-end condition occurs, it is as if the READ statement fails, and the contents of the record area cannot be defined. The file position indicator is set to indicate that no valid next record has been created.
If the at-end condition does not occur, the AT END phrase is ignored, and the following actions are performed.
-
If the FILE STATUS clause is described in the file-control entry, the result value is stored in the file status key.
-
If the NOT AT END phrase is described in the READ statement, statements described in imperative-statement-1 are executed. Any USE AFTER STANDARD EXCEPTION procedure related to file-name-1 is not executed.
-
If the AT END phrase is not described in the READ statement, and an appropriate USE AFTER STANDARD EXCEPTION procedure exists, the procedure is executed.
-
-
-
Indexed or relative files
-
Retrieves the next logical record in the order of the keys. The NEXT RECORD phrase must be specified.
-
Before executing the READ statement, the OPEN, START, or READ statement must successfully be executed to set the file position indicator.
-
If the files are in sequential access mode, the NEXT RECORD phrase is not required.
-
If the files are in dynamic access mode, the NEXT RECORD phrase must be specified to sequentially retrieve the records.
-
If no next record exists, the at-end condition occurs.
-
If the RELATIVE KEY clause is specified for the relative file, the RELATIVE KEY data item is updated to the relative key of the retrieved record by executing the READ statement.
-
Random Access Mode
Format 2 can be used for indexed files and relative files in random access mode or dynamic access mode
-
Indexed files
-
By executing the format-2 READ statement, the record of the given key is retrieved. The file position indicator specifies the record.
-
If there is no such record for the given key, the INVALID KEY condition occurs, and the READ statement fails.
-
If the KEY phrase is not described, the prime RECORD KEY is used as the key to execute the READ statement. If the KEY phrase is described, data-name-1 is used as the key to execute the READ statement.
-
-
Relative files
-
By executing the format-2 READ statement, the record corresponding to the relative record number in the RELATIVE KEY data item is retrieved, and the record is specified by the file position indicator.
-
If that record is not in the file, the INVALID KEY condition occurs, and the READ statement fails.
-
The KEY phrase must not be described for relative files.
-
Dynamic Access Mode
Can be used for indexed or relative organization files. In dynamic access mode, records can be retrieved sequentially or out of order depending on the format of the READ statement.
To retrieve records in sequence, the NEXT RECORD phrase must be described in format 1.
2.27. RELEASE
The RELEASE statement transmits records to an operation area before starting the sorting or merging operation. The statement can be used only within an area in which an INPUT PROCEDURE of the SORT or MERGE statement is executed. In an INPUT PROCEDURE, at least one RELEASE statement must be specified.

The following items are specified in the statement.
-
record-name-1
-
Must be specified with a record name described in the data vision SD entry.
-
record-name-1 can be qualified.
-
After the RELEASE statement is executed, the current contents of record-name-1 are moved to the sorting operation area.
-
-
identifiler-1
-
identifier-1 must reference one of the following.
-
A data item in the working-storage section, the local-storage section, or the linkage section.
-
A record description of another file that is already opened.
-
An alphanumeric function.
-
-
identifier-1 must be a valid sending item with record-name-1 that can be a receiving item according to the MOVE statement rules.
-
identifier-1 and record-name-1 must not reference the same storage area.
-
After execution of the RELEASE statement, the information about identifier-1 is still valid.
-
The execution result of the RELEASE statement with FROM identifier-1 phrase is the same as that of the following statements.
MOVE identifier-1 TO record-name-1. RELEASE record-name-1.
2.28. RETURN
The RETURN statement transmits records from an operation area to an output procedure after completing the sorting or merging operation. The RETURN statement can be used only within the area in which an OUTPUT PROCEDURE of the SORT or MERGE statement is executed.
In an OUTPUT PROCEDURE, at least one RETURN statement must be specified.

The following items are specified in the statement.
-
file-name-1
-
Must be specified in the data division SD entry.
-
If one or more records are specified, the records share the same storage area. After the RETURN statement is executed, only the current record is available.
-
-
INTO phrase
-
Moves the transmitted record to identifier-1.
-
-
AT END phrases
-
After all records of file-name-1 are processed by the RETURN statement, an at-end condition occurs, and imperative-statement-1 is executed.
-
If an at-end condition does not occur while executing the RETURN statement, imperative-statement-2 is executed for the NOT AT END phrase.
-
-
END-RETURN phrase
-
Indicates the end of the RETURN statement.
-
2.29. REWRITE
The REWRITE statement replaces an existing record of an indexed file or a relative file.
When the REWRITE statement is executed, the file must be opened in I-O mode.
For sequential access mode, the REWRITE statement can be executed after successful execution of the READ statement.

The following items are specified in the statement.
-
record-name-1
-
Must be specified with a record name described in the data division FD entry.
-
record-name-1 can be qualified.
-
-
FROM identifier-1 phrase
-
The execution result of the REWRITE statement with FROM identifier-1 phrase is the same as that of the following statements.
MOVE identifier-1 TO record-name-1. REWRITE record-name-1
-
-
identifier-1
-
identifier-1 must reference one of the following.
-
A data item in the working-storage section, the local-storage section, or the linkage section.
-
A record description of another file that is already open.
-
An alphanumeric function.
-
-
identifier-1 must be a valid sending item for record-name-1 that can be a receiving item according to the MOVE statement rules.
-
identifier-1 and record-name-1 must not reference the same storage area. After execution of the REWRITE statement, the information about identifier-1 is still valid.
-
-
INVALID KEY phrases
-
An INVALID KEY condition occurs in the following cases.
-
The value of the prime RECORD KEY of the record to be replaced is different from that of a record that was last retrieved from the file In sequential access mode.
-
The value of the prime RECORD KEY does not exist in any record of the file.
-
A record identical to the value of ALTERNATE RECORD KEY already exists in the file and DUPLICATES has not been specified.
-
-
-
END-REWRITE phrases
-
Indicates the end of the REWRITE statement.
-
The REWRITE statement is executed based on the organization of each file as follows:
-
Sequential files
-
For sequential files, the last input/output statement which had been executed before the REWRITE statement was executed must be a successful READ statement. After the REWRITE statement is executed, records retrieved by the READ statement are replaced.
-
The INVALID KEY phrase must not be specified. An EXCEPTION/ERROR procedure can be specified.
-
-
Indexed files
-
If the access mode is sequential, the record corresponding to the value of the prime RECORD KEY is replaced. When the REWRITE statement is executed, the value must be identical to that of the prime record key of the last retrieved record. In other words, if the access mode is sequential, only the last retrieved record can be updated. Data corresponding to the prime RECORD KEY cannot be replaced, but others are replaced to update the record.
-
The INVALID KEY phrase and an EXCEPTION/ERROR procedure can be omitted.
-
If the access mode is random or dynamic, the record corresponding to the value of the prime RECORD KEY is replaced.
-
If an invalid key condition occurs, the REWRITE statement fails, and no record is replaced.
-
-
Relative files
-
In sequential access mode, the INVALID KEY phrase must not be specified. An EXCEPTION/ERROR procedure can be specified. After the REWRITE statement is executed, records retrieved by the READ statement are replaced.
-
In random or dynamic access mode, the INVALID KEY phrase or an EXCEPTION/ERROR procedure can be specified, and both can be omitted. In these modes, the record corresponding to the RELATIVE KEY data item is replaced. If there is no such a record in the file, an invalid key condition occurs.
-
2.30. SEARCH
The SEARCH statement searches a table to find an element satisfying the given condition. The END-SEARCH phrase indicates the end of the SEARCH statement.
Format 1 (Serial Search)
Format 1 (serial search) is used to search an unsorted table. It can also be used to serially search a sorted table, or search a table by controlling the subscripts or indexes.
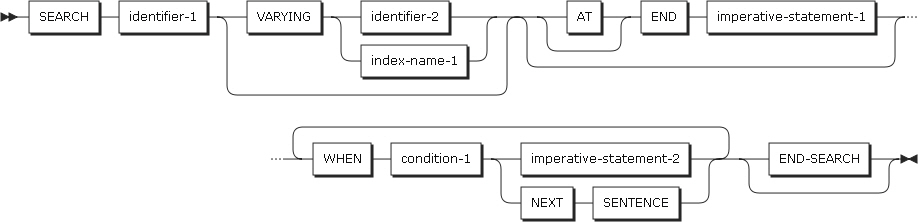
The following items are specified in the statement.
-
identifier-1
-
Specifies the table to be searched.
-
The data description entry for identifier-1 must contain an OCCURS clause with the INDEXED BY phrase.
-
identifier-1 must not be subscripted or reference-modified.
-
identifier-1 can reference subordinate data items included in a multidimensional table. To do so, an INDEXED BY phrase must be specified for each dimension of the table.
-
-
AT END
-
Specifies what to do if the search terminates without satisfying the conditions described in the WHEN phrase. If the AT END condition is satisfied, statements of imperative-statement-1 are executed.
-
Before executing a serial search, the first or only index value of identifier-1 must be set to specify the first occurrence for the search. Before executing a serial search for a multidimensional table, the index value for each superordinate dimension must be specified.
The SEARCH statement modifies only the value of the search index and index-name-1 or identifier-2. To search an entire table that has two or more dimensions, a SEARCH statement must be executed for each dimension. In WHEN phrases, indexes for every dimension can be used. Before executing a SEARCH statement, the associated indexes must be specified with SET statements.
When a search begins, the index value of identifier-1 is determined in the following way.
-
The conditions in the WHEN phrase are evaluated in order.
-
If no condition in the phrase is satisfied, the index of identifier-1 is increased by 1.
-
If one of the conditions is satisfied, the search is immediately terminated, and the imperative-statement-2 related to the condition is executed. The index contains the value that satisfied the condition. If NEXT SENTENCE is described, the statement after the closest period is executed.
If the condition has not been satisfied when the end of the table is reached, the search is terminated, and statements of imperative-statement-1 are executed if the AT END phrase exists. If the index value of identifier-1 is greater than the maximum value when the search begins, the search is immediately terminated.
-
VARYING phrase
Item Description index-name-1
If the index is in identifier-1, the index is used as index for search. If the index is the one of others, the value of index-name-1 and the index of identifier-1 are increased by the same amount. If index-name-1 is omitted, the first index of identifier-1 is used for the search.
identifier-2
Must be an index data item or an integer data item. If it is an index data item, the value of index-name-1 and the index of identifier-1 are increased by the same amount. If it is an integer data item, the data item is increased by 1 whenever the index of identifier-1 used for the search is increased.
-
WHEN phrase
Item Description condition-1
Any condition introduced in "Conditional expressions" can be used.
Format 2 (Binary Search)
Format 2 (binary search) is used to more efficiently search all occurrences in the table. The table must be sorted.
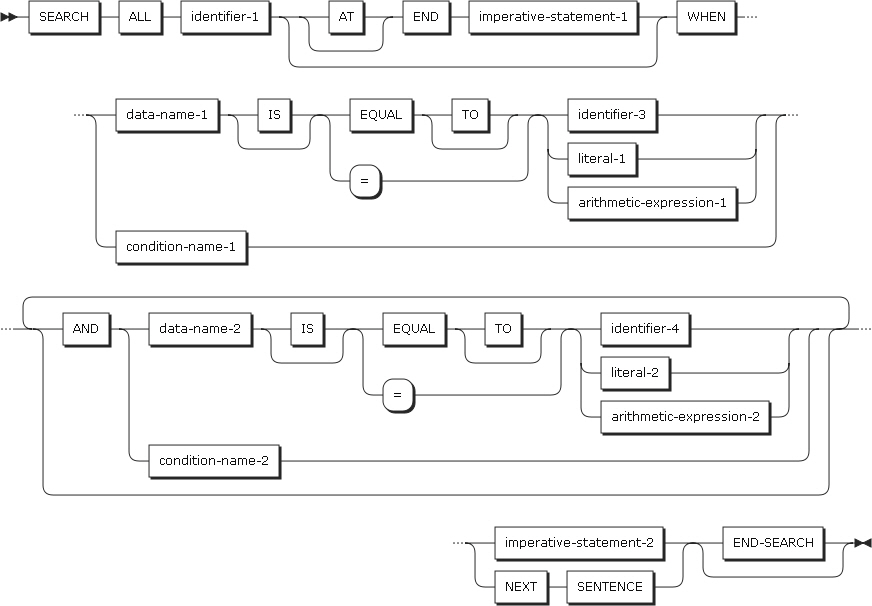
The following items are specified in the statement.
-
identifier-1
-
Specifies the table to be searched.
-
The data description entry for identifier-1 must contain an OCCURS clause with the INDEXED BY phrase and the KEY IS phrase.
-
identifier-1 must not be subscripted or reference-modified.
-
identifier-1 can reference subordinate data items included in a multidimensional table. To do so, an INDEXED BY phrase must be described for each dimension of the table.
-
-
AT END
-
Specifies the action to take when the search terminates without satisfying the conditions described in the WHEN phrase.
-
If the AT END condition is satisfied, statements of imperative-statement-1 are executed.
-
The SEARCH ALL statement executes a binary search. Because the entire table is searched, the index (search index) of identifier-1 does not need to be initialized with SET statements. The first index-name specified in the OCCURS clause is always used. To execute a binary search for a multidimensional table, SET statements must be used to set the index value for each superordinate dimension before executing the SEARCH ALL statement.
The SEARCH statement modifies only the value of the search index. To search a table which has two or more (up to seven) dimensions, the SEARCH statement must be executed for each dimension. If data in the table is not aligned in the order of ASCENDING or DESCENDING KEY, the results are unpredictable.
-
WHEN phrase
-
If the conditions in the WHEN phrase are satisfied, the search terminates and the statements of imperative-statement-2 are executed, or if NEXT SENTENCE is described the statement after the closest period is executed.
-
If no condition in the table is satisfied, the search is terminated, and an at-end condition occurs. If the AT END phrase exists, statements of imperative-statement-1 are executed. In this case, the last index value is not predictable.
-
The following items are specified.
Item Description condition-name-1, condition-name-2
Each condition-name must have a single value, and must be related to an ASCENDING or DESCENDING KEY data item for the table element.
data-name-1,
data-name-2
Must be an ASCENDING or DESCENDING KEY data item, and must be subscripted by the first index of identifier-1. Each data-name can be qualified.
data-name-1 must be a valid operand that can be compared with identifier-3, literal-1, or arithmetic-expression-1.
data-name-2 must be a valid operand that can be compared with identifier-4, literal-2, or arithmetic-expression-2.
data-name-1 and data-name-2 must not reference floating-point data items.
data-name-1 and data-name-2 must also not reference group items that include variable-occurrence data items.
identifier-3, identifier-4
Must not be an ASCENDING or DESCENDING KEY data item, and must not be subscripted by the first index of identifier-1.
Cannot be a data item that uses POINTER, FUNCTION-POINTER, or PROCEDURE-POINTER as usage.
data-name-2 must be a valid operand that can be compared with identifier4, literal-2, or arithmetic-expression-2.
literal-1, literal-2
literal-1 must be a valid operand that can be compared with data-name-1.
literal-2 must be a valid operand that can be compared with data-name-2.
arithmetic-expression
Can be one of expressions described in Arithmetic Expressions.
Must not contain an ASCENDING or DESCENDING KEY data item of identifier-1. Must not contain a data item that has been subscripted by the first index of identifier-1.
-
Considerations for Search Statements
Index items cannot be used as data subscripts to avoid a direct access to a table.
To ensure accurate execution of a SEARCH statement for a multi-dimensional table, the OCCURS DEPENDING ON clause must specify the correct table length.
NEXT SENTENCE
Moves to the nearest separator period.
2.31. SET
The SET statement performs the following operations in COBOL programs.
-
Specifies INDEX values for table management.
-
Increases or decreases an INDEX item by a specified value.
-
Specifies ON or OFF for external switches.
-
Specifies TRUE or FALSE for condition-names.
-
Specifies a data address for a USAGE POINTER item.
-
Specifies an entry address for a USAGE PROCEDURE-POINTER/FUNCTION-POINTER.
An index specified through USAGE INDEX and an index specified through the OCCURS INDEXED BY phrase are not distinguished in OFCOBOL. The value of either index can be specified through the SET statement.
Format 1
Format 1 is used for table management, and sets the current value of index-name-2, identifier-2, or integer-1 in index-name-1 or identifier-1.
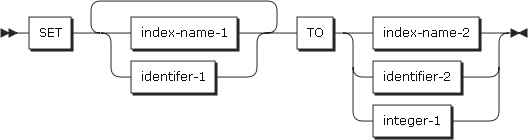
The following items are specified in the statement.
-
index-name-1
-
The field to set, called the receiving field.
-
Must be an item that is declared in the INDEXED BY phrase of an OCCURS clause in the data division.
-
-
identifier-1
-
The field to set, called the receiving field.
-
Must be an index item or a data item specified as an integer.
-
-
index-name-2
-
The field to specify the value to be set, called the sending field.
-
Must be specified by the INDEXED BY phrase of an OCCURS clause.
-
-
identifier-2
-
The field to specify the value to be set, called the sending field.
-
Must be an index item or data item specified as an integer.
-
-
integer-1
-
The field to specify the value to be set, called the sending field. Must be positive.
-
Format 2
Format 2 is used to add or subtract a specified value to or from the current value, rather than replacing the current index value by the specified value.

The following items are specified in the statement.
-
index-name-3
-
The field where the SET statement modifies the value, called the receiving field. index-name-3 must be included in the range of the index in a related table both before and after executing the SET statement.
-
identifier-3, the value to add or subtract with, called the sending field. It must be an elementary integer data item, not a group item, and integer-2 must be a nonzero integer.
-
When the format-2 SET statement is executed, index-name-3 increases (UP BY) or decreases (DOWN BY) by a value specified by identifier-3 or integer-2. Multiple fields can be specified for index-name-3, and the addition and subtraction operations are performed in the left-to-right order for all specified fields.
-
Format 3
Format 3 is the SET statement for external switches. It is used to specify ON or OFF for an external switch specified with mnemonic-name.
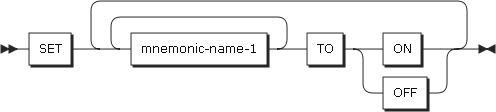
The following items are specified in the statement.
-
memonic-name-1
Specifies an external switch whose status can be modified with ON or OFF.
Format 4
Format 4 is the SET statement to specify a condition. It sets TRUE for a specified condition name so that the condition can be executed in the following statements.

The following items are specified in the statement.
-
condition-name-1
-
Specifies a conditional variable.
-
If multiple literals exist in the VALUE clause of condition-name-1, the same value as the first literal is set. If two or more condition-name-1s are specified, it has the same effect as specifying SET statements for each condition-name in the order they appear in the SET statement.
-
Format 5
Format 5 is the SET statement to specify a USAGE IS POINTER data item. It modifies the current value to the address value of a specified value.
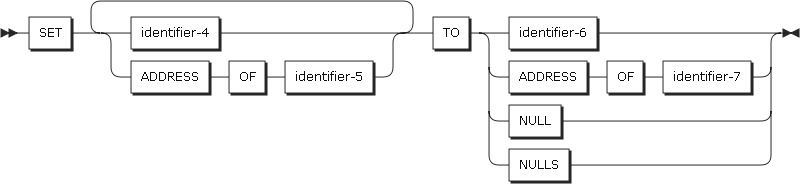
The following items are specified in the statement.
-
identifier-4
-
The receiving field. It must be specified as USAGE IS POINTER.
-
-
ADDRESS OF identifier-5
-
identifier-5 must be described as level-01 or level-77 in the linkage section.
-
-
identifier-6
-
The address value to be replaced, called the sending field. It must be specified as USAGE IS POINTER.
-
-
ADDRESS OF identifier-7
-
identifier-7 must be a data item described in the linkage section, the working-storage section, or the local-storage section.
-
level-66 or level-88 items cannot be specified.
-
ADDRESS OF identifier-7 specifies the address of identifier-7, rather than the identifier value itself.
-
-
NULL, NULLS
-
Used to specify an invalid address for identifier-4 or identifier-5.
-
2.32. SORT
The SORT statement receives records from one or more files, and sorts them based on a described key.
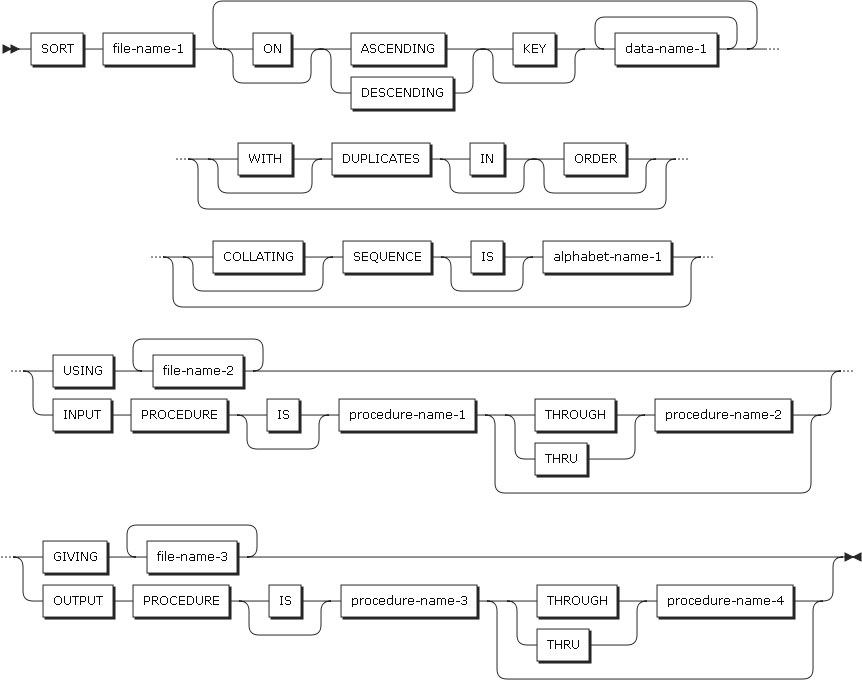
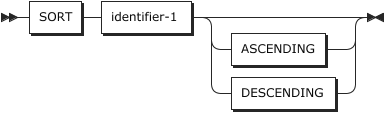
The following items are specified in the statement.
-
file-name-1
-
The name of the file in which the record to be merged is to be included. It is described in the SD entry.
-
-
identifier-1
-
The data description that includes the record to be sorted. It is a group type and must contain data to be sorted.
-
-
ASCENDING KEY and DESCENDING KEY phrases
-
Specifies either an ascending or descending sequence for the sort key.
Item Description data-name-1
Describes a KEY data item to be used in the SORT statement. data-name-1 must be a data item that is included in the record for file-name-1.
The leftmost data item is the major key, and the next data item is the second major key, based on the order in which the data items are specified.
-
In each input file, a KEY data item must be located in the same position and share the same data format. They do not need to have the same data-name.
-
If one or more records are described in file-name-1, the KEY data items must be specified in one of the records.
-
KEY data items cannot include an OCCURS clause, and cannot belong to an item containing an OCCURS clause.
-
KEY data items can be qualified. KEY data items can be one of the following data categories.
-
Alphabetical, alphanumeric, alphanumeric-edited
-
Numeric
-
Numeric-edited (with usage DISPLAY)
-
Internal floating-point or display floating-point
-
If file-name-3 references an indexed file, data-name-1 must be described with an ASCENDING phrase, and data-item must specify the same position as that of the major record key of the indexed file.
-
-
-
DUPLICATES phrase
-
If the DUPLICATES phrase is not described, the order of records that have the same key is unpredictable.
-
If the phrase is specified, the records with the same key are sorted in the following order.
-
They are sorted in the same order as the records in the input files.
-
If an input procedure exists, the records are sorted in the order that records are used in the RELEASE statement.
-
-
-
COLLATING SEQUENCE phrase
-
Only syntax checking is performed.
-
-
USING phrase
-
Describes input files.
Item Description file-name-2,…
Defines input files. If the USING phrase is specified, all records of the input files (file-name-2,…,) are automatically transmitted to file-name-1. All input files must be described in the FD entries in the data division.
-
-
INPUT PROCEDURE phrase
-
The INPUT PROCEDURE phrase contains the RELEASE statement, and transfers one record at a time to the file referenced by file-name-1. By using various statements such as OPEN, READ, and CLOSE, input records can be selected, copied, and modified.
-
Select an input record before executing a sort operation, and describe a procedure name to be modified.
Item Description procedure-name-1
Must be described with the first or only section or paragraph of the input procedure.
procedure-name-2
Must be described with the last section or paragraph of the input procedure.
-
-
GIVING phrase
-
Describes output files.
Item Description file-name-3,…
Defines output files. If the GIVING phrase is specified, all sorted records are automatically transferred to the output files. All output files must be described in the FD entries in the data division.
-
-
OUTPUT PROCEDURE phrase
-
The OUTPUT PROCEDURE phrase contains the RETURN statement, so that one record is transferred at a time from the file referenced by file-name-1. By using various statements such as OPEN, WRITE, and CLOSE, output records can be selected, copied, and modified.
-
Select an output record after executing a sort operation, and specify the name of a procedure to be modified.
Item Description procedure-name-3
Must be described with the first or only section or paragraph of the output procedure.
procedure-name-4
Must be described with the last section or paragraph of the output procedure.
-
2.33. START
The START statement sets the record position to read a sequential record in an indexed file or a relative file. When the statement is executed, the associated files must be open in INPUT or I-O mode.
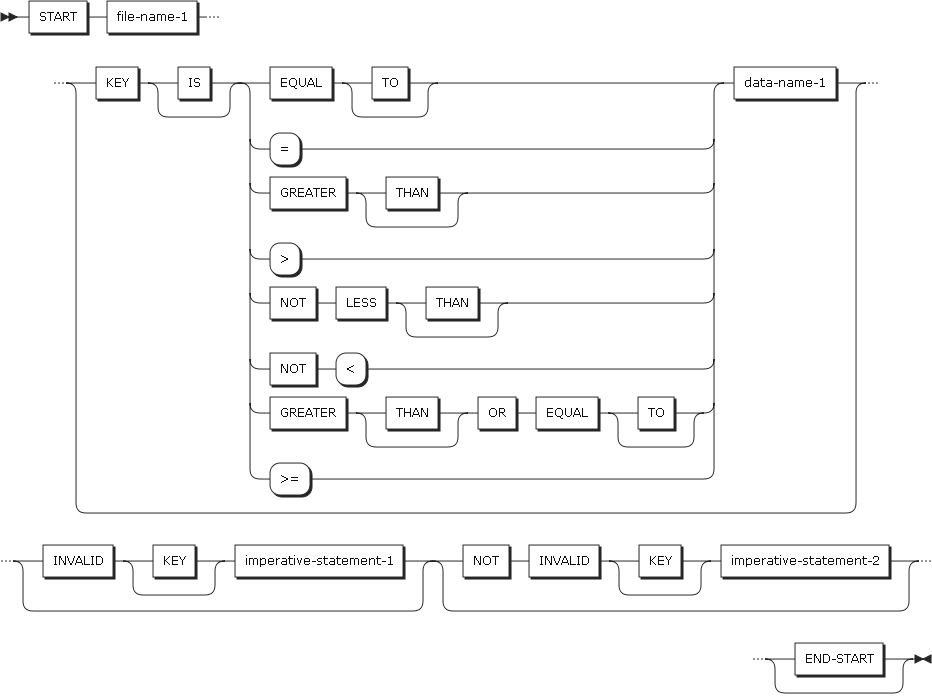
The following items are specified in the statement.
-
file-name-1
-
Must be the name of a file with sequential or dynamic access mode.
-
file-name-1 must be specified in the FD entry in the data division.
-
-
KEY phrases
-
If the KEY phrase is described, the file position indicator is located at the record with the key that satisfies the condition.
Then, the READ statement is used to read subsequent records.
Item Description data-name-1
Can be qualified, but cannot be subscripted.
When the START statement is executed, the current value of data-name-1 is compared with the corresponding key in the file.
-
If the FILE STATUS clause is described in the file-control entry, the related file status key is modified when the START statement is executed.
-
-
INVALID KEY phrases
-
An invalid key condition occurs in the following cases.
-
If the prime record key value is not greater than the previous record when an indexed file that is opened as OUTPUT is accessed sequentially.
-
If the prime record key value is the same as the prime record key value of an already existing record when an indexed file is opened as I-O or OUTPUT.
-
If the relative key is the same as the relative key of an already existing record when a relative file is accessed randomly or dynamically.
-
-
-
END-START phrases
-
Indicates the end of the START statement.
-
2.34. STOP
The STOP statement temporarily or permanently stops execution of the program.
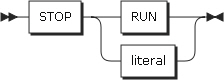
The following items are specified in the statement.
-
literal
-
Can be any numeric or alphanumeric literal, including a fixed-point number.
-
Can be any figurative constant except ALL literal.
-
When the STOP statement is executed with a literal, the literal is output and program execution is temporarily suspended. If the user performs a certain action, the next statement after the STOP statement is executed.
By specifying STOP RUN, control can be transferred to the system after the program is suspended. The statements following the STOP RUN statement are not executed, and all the files that were opened during program execution are closed.
If the STOP RUN statement is executed, the following results occur.
Classification | Result |
---|---|
Main Program |
Control is returned to the system. That is, the program is terminated. |
Subprogram |
Control is returned to the program that called the main program. That is, the subprogram is terminated. |
2.35. STRING
The STRING statement creates a single data item by combining the partial or complete contents of multiple data item or literals. A single STRING statement can be used instead of using multiple MOVE statements.
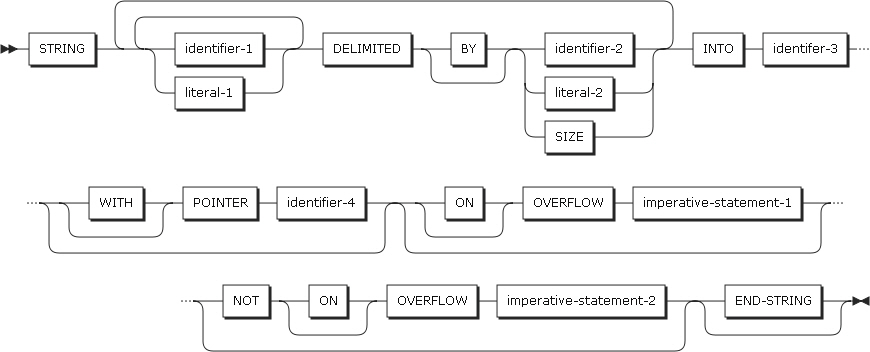
The following items are specified in the statement.
-
identifier-1, literal-1
-
Specifies the source data field. It must be a data item that is explicitly or implicitly specified as usage DISPLAY or usage DISPLAY-1. It can be a special register of alphanumeric type.
-
literal-1 must be alphanumeric. Any figurative constant except NULL that does not begin with the word ALL can be specified. Each figurative constant is regarded as a one-character alphanumeric literal.
-
-
DELIMITED BY phrase
-
Specifies the delimiter used to establish the limits of the string.
-
Sets the following items.
Item Description identifier-2, literal-2
The delimiter used to establish the limits of the string.
Used to delimit the data to be moved. If SIZE is specified, all data items specified in identifier-1 or literal-1 are moved.
If a figurative constant except ALL is specified, it is represented as a one-byte string. literal-2 must be alphanumeric.
SIZE
Indicates that the complete string is specified.
-
-
INTO phrase
-
Specifies the destination field.
-
Sets the following item.
Item Description identifier-3
Represents the destination string. Specifies a data item where the last created string will be included. The JUSTIFIED clause cannot be specified.
If identifier-3 is usage DISPLAY, identifier-1 and identifier-2 are usage DISPLAY, and all literals must be alphanumeric literals. Any figurative constant except for ALL can be specified. A figurative constant is treated as an alphanumeric literal with 1 character.
If identifier-3 is usage DISPLAY-1, identifier-1 and identifier-2 must be usage DISPLAY-1 and all literals must be DBCS literals. Only figurative constant that can be specified is SPACE.
-
-
WITH POINTER phrase
-
Indicates the length of a string in the data field that contains result data. The POINTER field is the length of a relative string when the source string’s usage is DISPLAY or DISPLAY-1.
-
The POINTER field specifies a data field of sufficient size that can contain a value equal to the length of the result data plus 1.
-
Sets the following item.
Item Description identifier-4
Specifies the pointer field. If specified, the programmer can explicitly use it, and a proper initial value needs to be specified.
The initial value of identifier-4 must be a positive integer.
-
All items except for identifier-4 must be either usage DISPLAY or usage DISPLAY-1.
If identifier-1 or identifier-2 references a numeric category data item, each numeric item must be described as an integer that does not specify the symbol P in its PICTURE string.
If identifier-1 or identifier-2 references an elementary data of the usage DISPLAY and of the category numeric, numeric-edited, or alphanumeric-edited item, it is treated as an alphanumeric item.
Evaluation of subscript, reference modification, variable-length, function identifier, etc. is performed at the start of the STRING statement execution.
-
ON OVERFLOW phrase
-
An overflow occurs in the following cases.
-
The value of the pointer field is less than 1.
-
The value of the pointer field is greater than the entered data field.
If an overflow occurs, data is no longer moved. When an overflow occurs, control is transferred to the command that was specified with ON OVERFLOW.
-
-
If the command that explicitly transfers control is executed, control is passed according to the rules for the command. Otherwise, the end of the STRING statement is executed after the execution of the command is completed.
-
If an overflow condition did not occur when the STRING statement was executed, ON OVERFLOW is ignored. Control is passed to the end of the STRING statement, or to the command in NOT ON OVERFLOW, if it is specified.
-
Sets the following item.
Item Description imperative-statement-1
Specifies a statement to be executed when an overflow condition occurs.
-
-
END-STRING phrase
-
Explicitly indicates the end of the STRING statement.
-
The END-STRING phrase can be used to nest a STRING statement as a conditional expression in another conditional statement.
-
Data Flow
The STRING statement transmits characters from the source fields to the target field that contains the final result. The source fields are processed in the order they are specified in the STRING statement.
The following rules apply.
-
Characters are transferred from the source fields to the receiving field in the following ways.
-
For a two-byte character, the rules of the MOVE statement between two-byte characters are applied.
-
For other characters, the rules of the MOVE statements for elementary moves between alphanumeric characters are applied.
-
-
If DELIMITED BY identifier-2 or literal-2 is specified, each character in each source field is moved, beginning from the leftmost character, until one of the following things occurs.
-
A delimiter for the source field is encountered. The delimiter itself is not moved.
-
The rightmost character in the source field has been moved.
-
-
If DELIMITED BY SIZE is specified, each entire source field is moved.
-
If the receiving field is filled, or all the source fields have been processed, the data transfer operation is completed.
-
If the POINTER phrase is specified, the user must explicitly set the pointer value of the source field.
If data processing is completed in the STRING statement, only the part of the target field into which data is moved is modified. The remaining unmodified part of the target field still contains the data that was stored before executing the STRING statement.
2.36. SUBTRACT
The SUBTRACT statement subtracts a numeric item or the sum of numeric items from numeric data items that specified after FROM, and stores the result in the data item that is specified after FROM.
-
Format 1
In format 1, the sum of all identifiers or literals specified before FROM is subtracted from identifier-2, which is specified after FROM, and the result is stored in identifier-2. If multiple identifier-2s are specified, a subtraction operation for each identifier-2 is performed from left to right, and the results are stored in the corresponding identifier-2.
SUBTRACT Statement Format 1 -
Format 2
In format 2, the sum of all identifiers or literals that are specified before FROM is subtracted from identifier-2, which is specified after FROM, and the result is stored in identifier-3.
SUBTRACT Statement Format 2 -
Format 3
In format 3, a subtraction operation is performed for identifier-1’s elementary items whose names are the same as those of identifier-2’s elementary data items, and the results are stored in the corresponding elementary data items of identifier-2.
SUBTRACT Statement Format 3
The following items are specified in the statement.
-
identifier
-
In format 1, identifier-1 and identifier-2 must be an elementary numeric data item.
-
In format 2, identifier-1 and identifier-2 must be an elementary numeric data item, and identifier-3 must be either a numeric data item or numeric-edited data item.
-
In format 3, identifier must be an alphanumeric group item.
-
-
literal
-
Must be a numeric and floating-point literal.
-
-
ROUNDED phrase
-
For more information about the ROUNDED phrase, refer to ROUNDED Phrase.
-
-
SIZE ERROR phrase
-
For more information about the SIZE ERROR phrase, refer to SIZE ERROR Phrase.
-
-
CORRESPONDING phrase
-
For more information about the CORRESPONDING phrase, refer to CORRESPONDING Phrase.
-
-
END-SUBTRACT phrase
-
Indicates the explicit end of the SUBTRACT statement.
-
2.37. UNSTRING
The UNSTRING statement divides consecutive data in a source field into multiple fields.
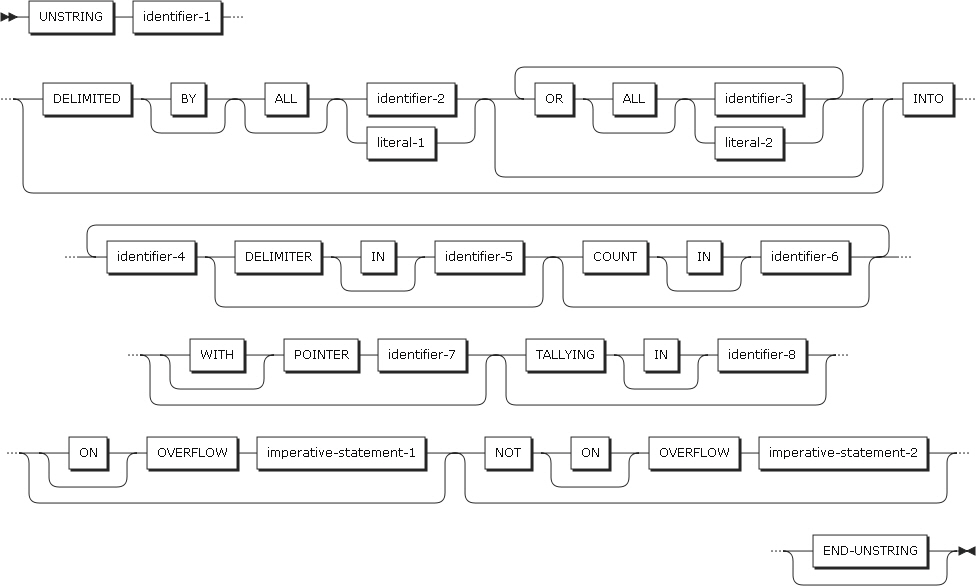
The following items are specified in the statement.
-
identifier-1
-
Specifies the source data field.
-
Data from the specified field is moved to the data field identifier-4 specified with INTO.
-
identifier-1 must be alphabetic, alphanumeric, alphanumeric-edited, or DBCS data.
-
-
DELIMITED BY phrase
-
Specifies delimiters to delimit the data to be transmitted.
-
If the DELIMITED BY phrase is not specified, the DELIMITER IN phrase and the COUNT IN phrase cannot be specified.
-
Set the following items.
Item Description identifier-2,identifier-3
Specifies one or more delimiters. They must be alphabetic, alphanumeric, alphanumeric-edited, or DBCS data.
literal-1, literal-2
Specifies one or more delimiters. They can be alphanumeric or DBCS data. The figurative constant ALL literal cannot be specified.
-
When ALL is specified
Multiple consecutive delimiters are treated as a single delimiter. If identifier-5 is specified, the delimiter is moved to identifier-5, the receiving field. Multiple delimiting characters are treated as an elementary item of the same usage and moved to the current delimiter receiving field.
-
Delimiter with two or more characters
If two or more sequential characters that are included in a data item specified as a source field are specified, they can be recognized as a delimiter.
-
Two or more delimiters
If two or more delimiters are specified, an OR condition applies. Whenever any overlapping part of the delimiters appears, it is treated as a delimiter in the specified source data field.
In the following example, if a data field with either AB or BC is encountered, it is treated as a delimiter. If ABC occurs, it is first matched to AB.
DELIMITED BY "AB" or "BC"
-
-
-
INTO phrase
-
Specifies the fields to contain the delimited data.
Item Description identifier-4
Specifies destination fields. Specifies one or more data fields.
identifier-4 must be an alphabetical, alphanumeric, numeric, or DBCS data item. If the data item is numeric, it cannot include the symbol 'P' in the corresponding PICTURE string. If identifier-4 refers to a data item of usage DISPLAY, identifer-1, identifier-2, identifier-3, and identifer-5 must also refer to usage DISPLAY. All literals must be alphanumeric. Likewise, if it refers to a data item of usage DISPLAY-1, the items must also refer to usage DISPLAY-1 or DBCS literals.
Can be specified with any figurative constant except one that begins with the word ALL. This is the same as specifying a one-byte alphanumeric literal.
identifier-5
Specifies a field to contain data delimited by the delimiter. It must be alphabetic, alphanumeric, or DBCS data.
identifier-6
Specifies a field to contain the length of data that is moved to identifier-4. It is an integer data item and must not include the PICTURE symbol 'P'.
-
-
DELIMITER IN phrase
-
Specifies the fields where data that has been deleted by the delimiter will be stored.
Item Description identifier-5
Must be either an alphabetical or alphanumeric category data item.
-
-
COUNT IN phrase
-
Specifies the field where the length of data that satisfies the conditions evaluated by the delimiters will be stored. The delimiters are not included in the length.
Item Description identifier-6
Specifies a field to contain the length of data that is moved to identifier-4.
identifier-6 must be an integer data item that does not use the symbol 'P' in the PICTURE string.
-
-
POINTER phrase
-
If the POINTER phrase is specified, the value of the pointer field, identifier-7, increases by 1 whenever each piece of data is moved. When the UNSTRING statement is completed, a value obtained by adding the initial value to the length of data satisfying the delimiters' conditions in the source data field is contained in the pointer field.
-
The user must initialize the pointer field before the UNSTRING statement is executed.
-
Set the following item.
Item Description identifier-7
Specifies a data field to contain the length of processed data.
Specifies an integer data item specified without the symbol 'P' in the PICTURE string.
The data item must be large enough to contain 1 plus the length of data referenced by identifier-1.
-
-
TALLYING IN phrase
-
If the TALLYING IN phrase is specified, the value obtained by adding the initial value to the total length of data items that were received is stored in the count field, identifier-8.
-
The user must initialize the field before the UNSTRING statement is executed.
-
Set the following item.
Item Description identifier-8
Specifies a data field where the length of data items that are removed by the delimiters will be stored.
Specifies an integer data item that does not specify the symbol 'P' in the PICTURE string.
-
-
ON OVERFLOW phrase
-
An overflow occurs when.
-
The value of the pointer field is less than 1.
-
The value of the pointer field exceeds the length of the output field.
-
The source field still contains data after all the input fields have been processed.
If an overflow occurs, the UNSTRING operation is terminated and if the ON OVERFLOW phrase is specified, control is moved to the command. If a command that explicitly transfers control is executed, control is moved according to the rules for the command. Otherwise, the UNSTRING statement is terminated after the command is executed.
If an overflow has not occurred and the NOT ON OVERFLOW phrase is specified, control is moved to the specified command. If the phrase is not specified, the UNSTRING operation is terminated.
-
-
Set the following item.
Item Description imperative-statement-1
Describes a statement to be executed when an overflow occurs.
-
-
END-UNSTRING phrase
-
Explicitly indicates the end of the UNSTRING statement. By using the END-UNSTRING phrase, an UNSTRING statement can be nested as a conditional expression in another conditional statement.
-
2.38. WRITE
The WRITE statement writes a record to an output file.
A sequential file must be open in OUTPUT or EXTEND mode.
An indexed file or relative file must be open in OUTPUT, I-O, or EXTEND mode.
-
Format 1
WRITE Statement Format 1WRITE Statement Format 1 - phrase1WRITE Statement Format 1 - invalid_keyWRITE Statement Format 1 - not_invalid_key -
Format 2
WRITE Statement Format 2 -
Format 3
WRITE Statement Format 3
The following items are specified in the statement.
-
record-name-1
-
Must be described in the FD entry in the data vision.
-
record-name-1 can be qualified, but must not be related to a sort or merge file.
-
-
FROM phrase
-
The execution result of the WRITE statement with the FROM phrase is the same as executing the following statements.
MOVE identifier-1 TO record-name-1. WRITE record-name-1.
-
-
identifier-1
-
identifier-1 references any of the following.
-
An entry in the working-storage section, the local-storage section, or the linkage section.
-
A record description for another opened file.
-
An alphanumeric function.
-
-
identifier-1 must be a valid sending item whose record-name-1 can be a receiving item according to the rules for a MOVE statement.
-
identifier-1 and record-name-1 must not reference the same storage area. After executing the WRITE statement, the information of identifier-1 is still valid.
-
-
identifier-2
Must be an integer data item.
-
ADVACING phrases
The ADVANCING phrase adjusts the position of the output record on the page.
-
ADVANCING phrase rules
If the ADVANCING phrase is specified, the following rules must apply.
-
If BEFORE ADVANCING is specified, the line is printed first, and then the page is advanced.
-
If AFTER ADVANCING is specified, the page is advanced, and then the line is printed.
-
If identifier-2 is specified, the page is advanced as many lines as are specified in identifier-2. identifier-2 must be an integer data item.
-
If integer-1 is specified, the page is advanced as many lines as are specified in integer-1.
-
The value in integer-1 or identifier-2 can be zero.
-
If PAGE is specified, the record is printed before or after the page is advanced to the next logical page, based on the specification of the BEFORE or AFTER phrase.
-
If mnemonic-name is specified, a skip to channels (from channel 1 to 12) or space suppression occurs. mnemonic-name must be described in the SPECIAL-NAMES paragraph.
If the ADVANCING phrase is specified in the WRITE statement, or the LINAGE clause exists in the file, a carriage control character is created to be added to the record. If the ADVANCING phrase is omitted, actions are performed as if AFTER ADVANCING 1 LINE has been specified.
-
-
LINAGE-COUNTER rules
If the LINAGE clause is described on the file, the LINAGE-COUNTER special register is modified according to the following rules whenever the WRITE statement is executed.
-
If ADVANCING PAGE is specified, LINAGE-COUNTER is 1.
-
If ADVANCING identifier-2 or integer-1 is specified, LINAGE-COUNTER increases by the value in identifier-2 or integer-1.
-
If the ADVANCING phrase is omitted, LINAGE-COUNTER increases by 1.
-
If the device is positioned on a new page, LINAGE-COUNTER is 1.
-
-
-
END-OF-PAGE phrases
-
If the END-OF-PAGE phrase is specified, and the logical end of the page is reached by the WRITE statement, the statement specified in imperative-statement-3 of the phrase is executed. If the END-OF-PAGE phrase is specified, the FD entry must contain a LINAGE clause.
-
An END-OF-PAGE condition occurs if a record line or space is printed in the footing area of the page body. In other words, an END-OF-PAGE condition occurs if the value of the LINAGE-COUNTER special register is greater than or equal to the value specified in the WITH FOOTING phrase of the LINAGE clause.
-
An automatic page overflow condition occurs if the WRITE statement cannot be completed within the current page body, and it has to continue to the next page.
-
If an END-OF-PAGE condition or an automatic page overflow condition occurs, and the END-OF-PAGE phrase is described, and the statement specified in imperative-statement-3 is executed.
-
-
INVALID KEY phrases
-
An invalid key condition occurs in the following cases.
-
For indexed files, a sequential access is specified and the file is opened in OUTPUT mode, and the value of the prime record key is not larger than that of the previous record key.
-
For indexed files, the file is opened in I-O or OUTPUT mode, and the value of the prime record key is equal to that of the existing record.
-
For relative files, random or dynamic access is specified, and RELATIVE KEY is identical to that of the existing record.
-
-
-
END-WRITE phrases
-
Explicitly indicates the end of the WRITE statement.
-
In each I-O mode, the WRITE statement functions as follows:
-
Sequential files
-
The greatest record length of sequential files is determined when the file is created, and is never changed.
-
Execution of the WRITE statement has no effect on the file position indicator.
-
If the FILE STATUS clause is specified in the file control entry, the file status key is updated after the WRITE statement is executed.
-
-
Indexed files
-
Before executing the WRITE statement for indexed files, the prime record key (the data item which is defined as RECORD KEY in the file control entry) must be set to any value. The value must be unique in the file.
-
If ACCESS IS SEQUENTIAL is specified in the file control entry, records must be written in the order of RECORD KEY values.
-
-
Relative files
-
If ACCESS IS SEQUENTIAL is specified in the file control entry, the first record is written as relative record number 1, the second record written as relative record number 2, the third record written as relative record number 3, and so on. If RELATIVE KEY is described in the file control entry, the relative number of the written record is stored in RELATIVE KEY.
-
If ACCESS IS RANDOM or ACCESS IS DYNAMIC is specified in the file control entry, the RELATIVE KEY must have the specified value before the WRITE statement is executed. After the WRITE statement is executed, the record is stored at the relative record number in the file.
-
2.39. XML GENERATE
The XML GENERATE statement converts data to XML format.
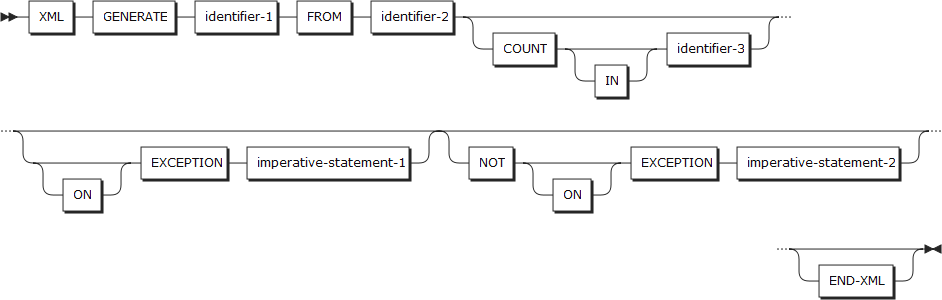
The following items are specified in the statement.
-
identifier-1
-
Receives a generated XML document.
-
References one of the following.
-
An alphanumeric elementary data item
-
An alphanumeric group item
If identifier-1 references an alphanumeric group item, it is treated as an alphanumeric elementary data item.
identifier-1 must not be described with the JUSTIFIED clause and cannot be a function identifier. It can be subscripted or reference modified. It must not overlap identifier-2 and identifier-3.
identifier-1 must be large enough to contain the generated XML document. Typically, it must be from 5 to 10 times the size of identifier-2, depending on the length of the data-name or data-names within identifier-2. If identifier-1 is not large enough, an error condition exists at the end of the XML GENERATE statement.
-
-
-
identifier-2
-
Group or elementary data item to be converted to XML format.
-
Cannot be a function identifier or be referenced modified, but can be subscripted. It must not overlap identifier-1 and identifier-3. It must not specify the RENAMES clause.
The following data items specified in identifier-2 are ignored by the XML GENERATE statement.
-
Subordinate unnamed elementary data item or elementary FILLER data items
-
Slack bytes inserted for SYNCHRONIZED items
-
Data items subordinate to a data item that is described with the REDEFINES clause or that is subordinate to such a redefining item
-
Data items subordinate to a data item that is described with the RENAMES clause
-
Group data items whose subordinate data items are ignored
-
-
Data items specified in identifier-2 that are not ignored according to the previous rules must satisfy the following.
-
Each elementary data item must be alphabetic, alphanumeric, numeric, or index data item.
-
There must be at least one such elementary data item.
-
Each data name that is not FILLER must be unique within any immediately superordinate group data item.
-
Data items must not specify the DATA FORMAT clause.
-
-
-
identifier-3
-
Contains the number of generated XML character encoding units after the XML GENERATE statement execution, if the COUNT IN phrase is specified. (Unit: bytes)
-
Integer data item defined without the symbol P in the picture string. It must not overlap identifier-1 and identifier-2.
-
-
ON EXCEPTION phrase
-
There are exception conditions when an error occurs during an XML document generation. For example, if identifier-1 is not large enough to contain a generated XML document, the generation stops and identifier-1 is undefined. If the COUNT IN phrase is specified, identifier-3 is defined as the number of generated character positions, which can be from 0 to the length of identifier-1.
-
If the ON EXCEPTION phrase is specified, control is sent to imperative-statement-1. Otherwise, the NOT ON EXCEPTION phrase, if any, is ignored and control is sent to the end of the XML GENERATE statement.
-
-
NOT ON EXCEPTION phrase
-
When this phrase is specified, if an exception condition does not occur during an XML document generation, control is sent to imperative-statement-2. If an exception condition occurs, control is sent to the end of the XML GENERATE statement. If the ON EXCEPTION phrase is specified, this phrase is ignored.
-
-
END-XML phrase
-
Indicates the end of the XML GENERATE or XML PARSE statement.
-
2.40. XML PARSE
The XML PARSE statement is the COBOL language interface to an XML parser depending on the setting of the XMLPARSE compiler option.
There are two XML parsers: XMLPARSE(XMLSS) and XMLPARSE(COMPAT). OFCOBOL uses XMLPARSE(XMLSS).
The XML PARSE statement parses an XML document into its individual pieces and passes each piece to a user-written processing procedure.
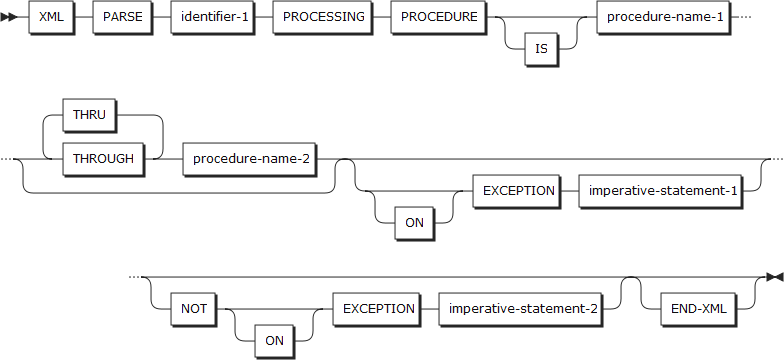
The following items are specified in the statement.
-
identifier-1
-
Contains XML document characters. Must be an alphanumeric data item or alphanumeric group data item.
-
-
PROCESSING PROCEDURE phrase
-
Procedure name to handle various events generated by the XML parser.
-
procedure-name-1, procedure-name-2
Section or paragraph names in the procedure division. If both procedure-name-1 and procedure-name-2 are specified, they must be in the same procedure. If either is a name in a declarative procedure, both of them must be in the declarative procedure.
-
procedure-name-1
First or only section or paragraph in the processing procedure.
-
procedure-name-2
Last section or paragraph in the processing procedure.
-
-
For each XML event, the parser sends control to the first statement in the procedure named procedure-name-1. Control is always returned from the processing procedure to the XML parser.
The point from which control is returned is determined as follows:
-
If procedure-name-1 is a paragraph name and procedure-name-2 is not specified, the control is returned after the last statement in the procedure-name-1 paragraph is executed.
-
If procedure-name-1 is a section name and procedure-name-2 is not specified, the control is returned after the last statement in the last paragraph in the procedure-name-1 section is executed.
-
If procedure-name-2 is specified as a paragraph name, the control is returned after the last statement in the procedure-name-2 paragraph is executed.
-
If procedure-name-2 is specified as a section name, the control is returned after the last statement in the last paragraph in the procedure-name-2 section is executed.
An execution sequence from the procedure named procedure-name-1 to the procedure named procedure-name-2 must be defined. If there are two or more paths to a return point, procedure-name-2 can be a name of a paragraph that consists of only an EXIT statement. All the paths to the return point must lead to the paragraph.
-
-
The processing procedure consists of all the statements for handling XML events. The procedure range includes all statements executed by CALL, EXIT, GO TO, GOBACK, and PERFORM statements in the range. The range must not cause a GOBACK or EXIT PROGRAM statement execution. The procedure can end the run unit with a STOP RUN statement.
-
-
ON EXCEPTION phrase
-
Executed when the XML PARSE statement causes an exception condition.
-
An exception condition exists when the XML parser detects an error during XML document processing. The parser signals an XML exception by sending control to the processing procedure by using the XML-EVENT special register containing 'EXCEPTION'. An exception condition also exists when the processing procedure sets XML-CODE to -1. In this case, XML-EVENT does not contain 'EXCEPTION' and the parsing ends.
-
If this phrase is specified, the parser sends control to imperative-statement-1. If this phrase is not specified and the NOT ON EXCEPTION phrase is specified, the NOT ON EXCEPTION phrase is ignored and control is sent to the end of the XML PARSE statement. If the processing procedure handles an XML exception event, sets XML-CODE to 0, and then sends control to the parser, the exception condition no longer exists.
-
-
NOT ON EXCEPTION phrase
-
Executed when there is no exception condition at the end of XML PARSE processing.
If an exception condition does not exist when XML PARSE processing ends, control is sent to imperative-statement-2 in the NOT ON EXCEPTION phrase if the phrase is specified. If the phrase is not specified, control is sent to the end of the XML PARSE statement. If the ON EXCEPTION phrase is specified, it is ignored.
-
The special register XML-CODE contains 0 after the XML PARSE statement execution.
-
-
END-XML phrase
-
Indicates the end of the XML GENERATE or XML PARSE statement.
-