Tmax Applications
This chapter describes the basic concepts, client/server programs, APIs, and errors for developing Tmax applications.
1. Application Configuration
A Tmax application consists of a client program and a server program. The client program handles user interfaces (presentation logic), while the service routine for servers handles tasks and implements access logic.
If a client has a request, a server handles the request. Although there are N clients, a single call starts a single service. When a client has a request, the following occurs: the client connects to CLL, a tpcall is made for the request, CLL handles the request, and the request is returned if it is successfully handled. When a client program connects to Tmax, it needs information from Tmax such as the host address and the port number. The information is saved in the .profile or the tmax.env file and set in the TMAX_HOST_ADDR and TMAX_HOST_PORT properties, respectively.
A server program consists of main() that is provided by Tmax and service routines written by a developer.
Tmax provides various API functions to handle connection data requests from clients and servers. Tmax API complies with the international standard for distributed processing (X/Open DTP model). When an application is developed, only a service routine is developed.
The following figure shows the configuration of a Tmax application.
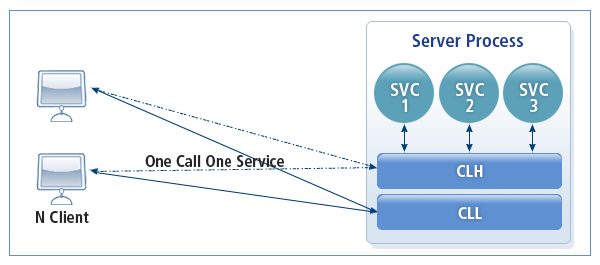
2. Buffer Types
When a client requests a service from a server, it uses the following communication buffer for communication.
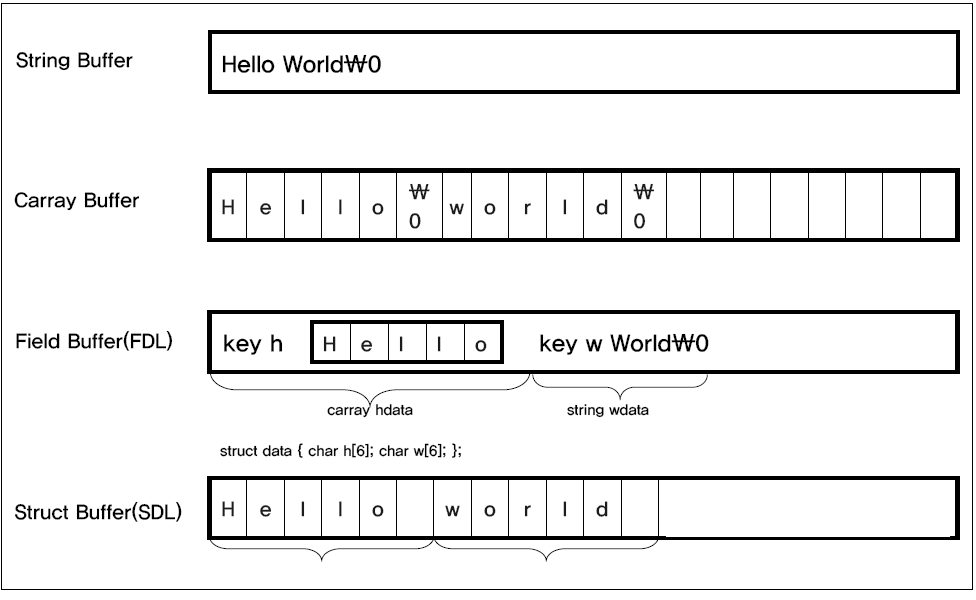
-
STRING buffer
Used to save a string that ends with NULL. The buffer length does not need to be specified. There is no problem caused by platform differences.
-
CARRAY and X_OCTET buffers
Used to save binary type data that has the specified number of bytes. The length of a buffer must be specified to exchange data. There is no problem caused by platform differences.
-
STRUCT and X_C_TYPE buffers
Used to use a C language struct. All primitive types can be used as members of the struct. A declared struct and an array of structs can also be used as a member of the struct.
-
X_COMMON buffer
Used to save a C language struct. Only the char, int, and long types can be used as members of the struct.
-
FIELD buffer
Used to save field key and data value pairs. All primitive types can be saved in this buffer. It is used when an exchanged data type can be changed. It provides various APIs for accessing and converting data.
Field Definition Language (FDL)
FDL can operate and handle specific data from the desired information using a field key buffer, unlike a general structure.
FDL specifies names for each field key (NAME, ADDR, and TEL), numbers, and types in a <XXX.f> field buffer file. If <XXX.f> is compiled using FDLC, a file mapped into <XXX_fdl.h> is created. Field keys and values selected by a user can be accessed by referring to the <XXX_fdl.h> included during compilation.
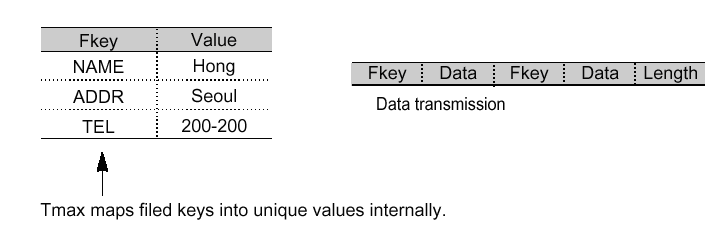
3. Client/Server Program
The client program handles user interfaces (presentation logic), while the service routine for servers handles tasks and implements access logic.
3.1. Client Program
A client program receives input from a user, requests a service from a server, receives a response from the server, and outputs the service response to the user.
Client Program Flow
The following is the flow of a client program:
main() { Connect to Tmax Allocate a buffer for transmission/reception Write client business logic (receives a user request and saves it to the transmit message buffer) Request and respond to a service (sends the transmit message buffer to Tmax CLH and saves the response data to the reception message buffer through Tmax CLH) Write client business logic (displays the response data to the user) Release the buffer for transmission/reception Release the connection to Tmax }
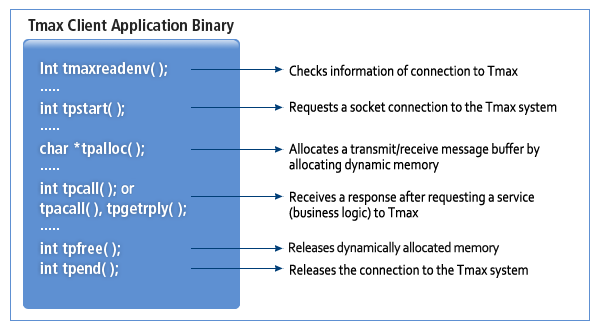
The following are descriptions for the major functions of a client program.
-
Function for Tmax communication environment
Function Description tmaxreadenv()
Specifies Tmax environment variables that are referenced when a Tmax client program is executed in a specific file. When writing a program that calls the tmaxreadenv() API, a specific file path and name can be set. The file can then be parsed with a file pointer when the corresponding client program is executed.
Tmax environment variables, such as TMAX_HOST_ADDR and TMAX_HOST_PORT, are loaded into memory that is allocated when a client program is executed and used to call related API functions.
-
Functions for connecting to a client and a server
Function Description tpstart()
Requests a socket connection to CLL and passes the accepted connection to CLH. When this function is called, values set in TMAX_HOST_ADDR and TMAX_HOST_PORT are used.
tpend()
Terminates a socket connection.
-
Functions for allocating and releasing a buffer
Function Description tpalloc()
Allocates memory used for data transmission/reception between clients and servers via Tmax. The memory allocated is the size required for data transmission/reception.
Dynamically allocated memory must be explicitly released.
tpfree()
Releases memory that was dynamically allocated using tpalloc(). An allocated memory buffer must be returned. If the memory is not returned, garbage (memory leakage) occurs.
-
Function for synchronous communication
Function Description tpcall()
Requests a service from CLH and transfers transmission data. CLH checks a service requested by a client and then transfers it to a server application process.
A client waits until receiving a reply for the service request.
-
Functions for asynchronous communication
Function Description tpacall()
Requests a service from CLH and transfers transmission data. CLH checks a service requested by a client and then transfers it to a server application process.
A client performs logic after calling tpacall() regardless of whether it receives a reply to the service request.
tpgetrply()
Requests reply data from CLH through the parameter (cd) value for tpacall().
If there is reply data to be sent to a corresponding client in CLH, the data is immediately transferred.
It waits until the reply data is received, or sends a reception error to the client according to flags.
The following figure shows the process of major functions in a client program.
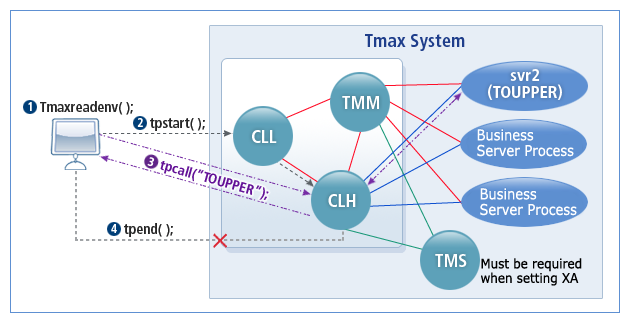
For more information about each function, refer to Tmax Application Development Guide and Tmax Reference Guide. |
Client Program Configuration
To create an executable file, compile a client program after coding is completed.
To compile a client program, the following must be defined: a client program written by a developer, the Tmax client library, a structure file if a structure buffer is used, and a field key buffer file in which field tables are defined.
The following figure shows the configuration of a client program:
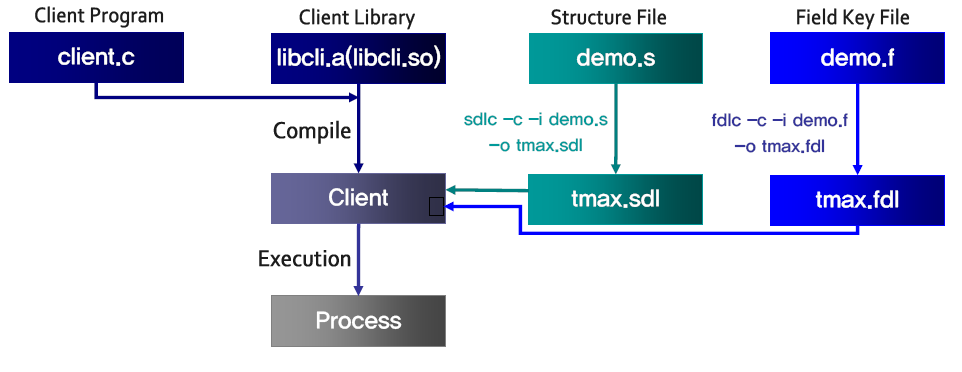
-
Client program
A client program written by a developer.
-
Tmax client library (libcli.a / libcli.so)
The library provided by Tmax. It is the object code of functions used to develop a client program.
-
Structure file
If a client program uses a structure such as STRUCT, X_C_TYPE, or X_COMMON, a structure file that ends with <file_name.s> is needed. The structure file must be compiled with the sdlc command in advance. After compilation, the data of a structure is converted to standard communication type data and a binary type file is created. The binary file is used to send and receive data with the standard communication method when a client program is executed.
-
Field key file
If a field key structure is used, a field definition file that ends with <.f> is needed. If the file is compiled using the fdlc command, a field key buffer file creates <field_key_buffer_name_fdl.h> using the key mapping method and uses it when a program is executed. Unlike an existing structure file, it can reduce resource waste because some user desired fields can be operated and transferred. However, overhead can occur when mapping keys.
3.2. Server Program
A server program receives a user request, handles it, and returns a reply to the client.
Server Program Flow
The following is the flow of a server program.
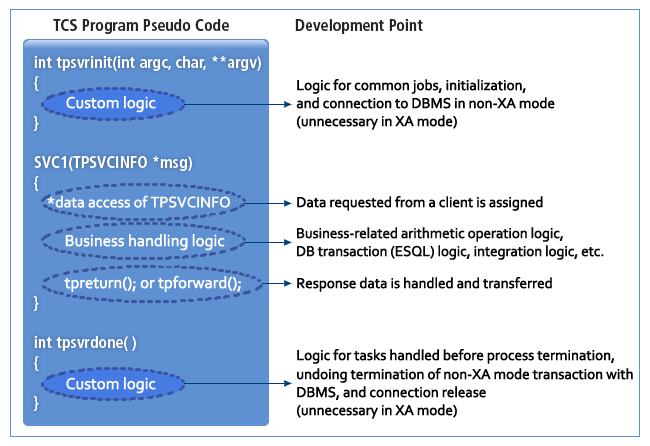
int tpsvrinit(int argc, char **argv) { Initialize a server (connect to DBMS in the non-XA mode) } SVC_NAME(TPSVCINFO *msg) { Handle DB transactions (ESQL) Business handling logic Send response result to a client } int tpsvrdone() { Tasks handled before server termination (release connection to DBMS in non-XA mode) } void tpsvctimeout() { Related to SVCTIME properties Called from a service timeout handler }
The following are descriptions of major functions of a server program.
-
Function for initializing a server
Function Description tpsvrinit()
Called when a server application process starts.
The standard input of tpsvrinit() is made through the configuration for the Tmax configuration file. A developer redefines and then uses tpsvrinit(). If it is not redefined, tpsvrinit(), which basic logic of the Tmax server library is applied to, is called.
-
Function for terminating a server
Function Description tpsvrdone()
Called just before a server application process is normally terminated.
A developer redefines and then uses tpsvrdone(). If it is not redefined, tpsvrdone(), which basic logic of the Tmax server library is applied to, is called.
-
Tmax response functions
Function Description tpreturn()
Notifies CLH of the termination of service logic and transfers reply data. CLH checks the information of a service caller and immediately sends reply data.
If the dynamically allocated memory pointed to by the reply data buffer is freed, the server application stops any running processes and readies to receive the next reply.
tpforward()
Notifies CLH of the termination of service logic and transfers data to SVC. CLH checks whether a server process that includes a service to be transferred is available and immediately sends data.
If the dynamically allocated memory pointed to by the reply data buffer is freed, the server application stops any running processes and readies to receive the next reply.
The following figure shows the process of major functions in a server program.
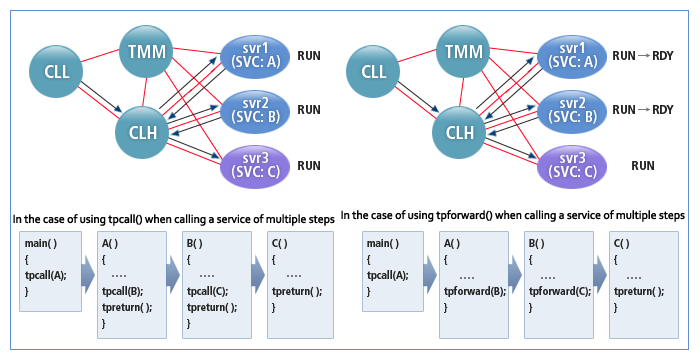
Server Program Configuration
A server program consists of main(), which is provided by Tmax, and service routines written by a developer.
The following figure shows the configuration of a server program.
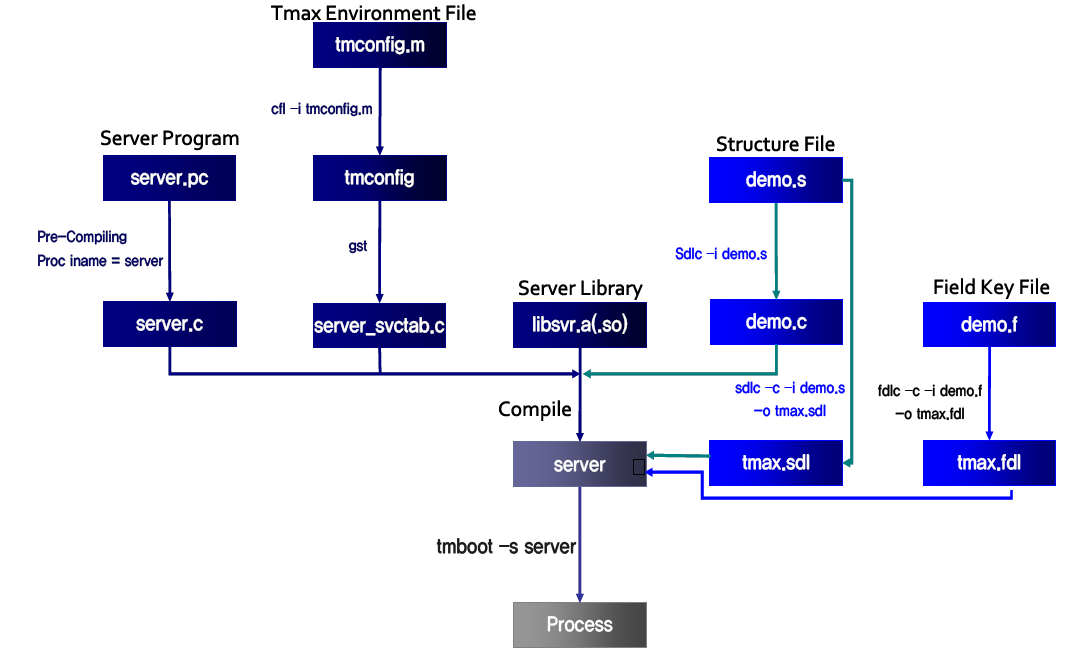
-
Server program
A developer written service routine that handles a client request.
If a SQL statement is used, it must be precompiled using a tool provided by the corresponding database vendor.
-
Tmax server library (libsvr.a / libsvr.so)
The server library provided by Tmax. It has server main(), tpsvrinit(), tpsvrdone(), and various Tmax functions.
-
Service table
A file that lists the names of services provided by each server. It is created by referring to the Tmax configuration file.
The service table is used to search for the location of a corresponding service routine in a server when a service is performed. It is provided by a system administrator. For more information, refer to Tmax Administration Guide.
-
Structure binary table (SDLFILE)
If a structural buffer (STRUCT, X_C_TYPE, and X_COMMON) is used, a structure defined in the <xxx.s> format is needed.
There are two types of structure files: standard communication type (structure_file_name_sdl.c) and structure header file (structure_file_name_sdl.h). If a structure file is compiled using the sdlc –c command, a binary table used to convert the types of structure members to those of standard communication is created.
If a structure is not used, $TMAXDIR/lib/sdl.o must be compiled with an application server.
-
Field buffer binary table (FDLFILE)
If a field buffer is used, a field buffer file defined in the format <xxxx.f> is needed. If the file is compiled using the fdlc command, a binary table, which is used to match field keys and data, and a header file (xxx_fdl.h), which is used to match field keys and field key names, are created. Unlike an existing structure file, the field buffer binary table can reduce resource waste because only the user desired fields can be operated and transferred. However, it can cause an opposite effect if there are too few fields because data values and field key values are managed together.
-
Structure and standard buffer conversion/reversion program
To use a structure buffer in a server program, a structure and a standard buffer conversion/reversion program (xxxx_sdl.c and xxxx_sdl.h) created with the sdlc command must be compiled and linked together. If a structure is not used, TMAXDIR/lib/sdl.o must be linked.
4. System Configuration File
A system configuration file includes information necessary for the Tmax system. The Tmax configuration file sets the configuration of the Tmax system and is written by a Tmax administrator. This file is used to create a service table and start the Tmax system.
The configuration file consists of the following 8 sections.
Section | Description | Required / Optional |
---|---|---|
DOMAIN section |
Defines a single independent Tmax system environment. |
Required |
NODE section |
Defines an environment related to each node configuring domains. |
Required |
SVRGROUP section |
Defines properties related to server groups and databases. |
Required |
SERVER section |
Defines properties related to servers. |
Required |
SERVICE section |
Defines properties related to services. |
Required |
GATEWAY section |
Defines properties related to gateways across domains. |
Optional |
ROUTING section |
Defines properties related to data dependent routing. |
Optional |
RQ section |
Defines properties related to reliable queues. |
Optional |
-
Names of sections start with an asterisk (*) (for example, *DOMAIN, *NODE, etc.)
-
Names of a section and its child elements must start in the first space of a line.
-
Definitions of child elements are separated by a comma (,).
The configuration file is a general text file and compiled with the cfl command.
cfl -i Tmax Environment File Name
The following is an example of the Tmax configuration file. Values inside "< >" must be modified.
*DOMAIN
<resrc_name> SHMKEY = <UNIQUE IPCKEY>,
MAXUSER = <256>,
TPORTNO = <8999>
*NODE
<uname> TMAXDIR = <TMAX installed directory>
APPDIR = <APPLICATION directory>
PATHDIR = <PATH directory>
*SVRGROUP
<svg_name> NODENAME = <uname>,
DBNAME = <ORACLE>,
OPENINFO = "ORACLE_XA+Acc=P/tmaxsoft/tmaxsoft+SesTm=60"
*SERVER
<svr_name> SVGNAME = <svg_name>,
MIN = <5>,
MAX = <10>
*SERVICE
<svc_name> SVRNAME = <svr_name>
For more information about each clause, refer to Tmax Administration Guide. |
5. API
To ease program development, a header file with defined API prototypes must be used. The APIs are implemented in the client/server library. For more information about APIs, refer to Tmax Application Development Guide and Tmax Reference Guide.
5.1. Tmax Standard API
X/Open ATMI
The X/Open Application Transaction Monitor Interface (ATMI) API is provided as a standard of the X/Open DTP model. It can be used as a method of communication between application programs and TP-Monitor.
The functions defined in atmi.h are divided into functions related to a buffer, service request and response, conversational mode, and service termination.
-
Functions related to buffer allocation and release
Function Description tpalloc()
Allocates a buffer to send and receive data.
tprealloc()
Changes the size of a buffer.
tpfree()
Frees an allocated buffer.
tptypes()
Provides information about the buffer size and type.
-
Functions related to service request and response
Function Description tpcall()
Requests a service and waits for the reply.
tpacall()
Requests a service and then performs other tasks while waiting for the result when tpgetrply() is called.
tpcancel()
Cancels the response to a service request.
tpgetrply()
Receives the response to tpacall().
-
Functions related to the conversational mode
Function Description tpconnect()
Establishes a connection for sending and receiving messages in the conversational mode.
tpdiscon()
Forcefully terminates a connection to a service in the conversational mode.
tprecv()
Receives a message in the conversational mode.
tpsend()
Sends a message in the conversational mode.
-
Function related to service termination
Function Description tpreturn()
Sends a response to a service request to a client and terminates the service routine.
X/Open TX API
The X/Open TX API provides communication methods for transactions between application programs and TP-Monitor. The functions defined in tx.h are transaction management functions.
The following is a list of TX APIs.
-
Functions related to transactions
Function Description tx_begin()
Starts a transaction.
tx_commit()
Commits a transaction and saves the result.
tx_rollback()
Rolls back a transaction.
tx_open()
An internal function that starts a resource manager.
tx_close()
An internal function that terminates a resource manager.
tx_set_transaction_timeout()
Sets timeout for terminating a transaction.
tx_info()
Returns information about a global transaction.
tx_set_commit_return()
Sets when a global transaction is permitted.
tx_set_transaction_control()
Automatically starts the next transaction after the current transaction completes.
5.2. Non-standard API
Tmax ATMI
Tmax ATMI functions defined in tmaxapi.h are divided into functions related to unrequested messages, RQ, error settings, timeout settings, etc. Defined APIs are non-standard interfaces and developed to improve the developer’s productivity. It can be used as a method of communication between application programs written by a developer and TP-Monitor.
The following is a list of non-standard APIs defined in tmaxapi.h.
-
Functions related to unrequested data
Function Description tpbroadcast()
Sends unrequested data to clients registered in a system.
tpsetunsol()
Specifies a function for handling unrequested data.
tpgetunsol()
Receives unrequested data.
tpsetunsol_flag()
Sets a flag for receiving unrequested data.
tpchkunsol()
Checks whether unrequested data arrives.
-
Functions related to errors
Function Description tpstrerror()
Outputs an error as a string.
Userlog()
Logs an error in a buffer.
ulogsync()
Saves the contents of 'ulog' in a memory buffer on a disk.
UserLog()
Has the features of userlog() and ulogsync().
gettperrno()
Returns an error number that occurs when the Tmax system is called.
gettpurcode()
Returns the urcode set by a developer.
tperrordetail()
Returns information about an error that occurs when the Tmax system is called.
-
Functions related to socket information
Function Description tpgetpeer_ipaddr()
Returns the IP address of a connected client.
tpgetpeername()
Returns the name of a connected client.
tpgetsockname()
Returns the socket name of a connected client.
-
Function related to block timeout
Function Description tpset_timeout()
Sets block timeout.
-
Function related to failover
Function Description tptobackup()
Establishes a connection to a backup machine.
-
Functions related to connection
Function Description tpstart()
Starts a connection to the Tmax system.
tpend()
Terminates a connection to the Tmax system.
-
Functions related to RQ
Function Description tpenq()
Saves a request of a client in RQ.
tpdeq()
Fetches data in RQ.
tpqstat()
Requests statistics about data saved in RQ.
tpextsvcname()
Requests a service name from data saved in RQ.
-
Functions related to environment variables
Function Description tmaxreadenv()
Fetches the environment variables from the file.
tpputenv()
Sets the environment variables.
tpgetenv()
Returns the values of the environment variables.
-
Functions related to a window operation
Function Description WinTmaxStart()
Connects to the Tmax system.
WinTmaxEnd()
Disconnects from the Tmax system.
WinTmaxSetContext()
Specifies a Window handle.
WinTmaxSend()
Sends data.
WinTmaxAcall()
Asynchronous function for Windows.
WinTmaxAcall2()
Asynchronous function that handles data reception with a callback function.
-
Other functions
Function Description tpscmt()
Invalidate settings related to transaction control in the configuration file.
tpgetlev()
Checks the transaction mode.
tpchkauth()
Checks whether certification is required.
tpgprio()
Checks the priority of a service request.
tpsprio()
Sets the priority of a service request.
tpsleep()
Waits for a message for a specified amount of time.
tp_sleep()
Waits for data to arrive (in seconds).
tp_usleep()
Waits for data to arrive (in microseconds).
tpschedule()
Allocates tasks in a queue to UCS so the tasks can be processed.
tpuschedule()
Waits a specified amount of time for data in a UCS server process.
tpsvrinit()
Initializes a Tmax server process.
tpsvrdone()
Calls a termination routine for a Tmax server process.
tpsvctimeout()
Shuts downs a UCS server process.
tmadmin()
Manages a system as a type of a service call.
The following is a list of non-standard APIs defined in atmi.h.
-
Function related to service termination
Function Description tpforward()
Terminates its own service handling and transfers a client request to another service routine.
-
Functions related to client connection
Function Description tpstart()
Connects a client application to Tmax.
tpend()
Disconnects a client application from Tmax.
FDL API
FDL (Field Definition Language) is non-standard API developed to improve developer productivity. FDL is associative-typed data that manages indexes called field keys and data together. APIs related to a FIELD buffer are defined in fbuf.h.
Data is saved in a field key buffer, which is provided by the Tmax system. To operate the buffer, the following functions are provided:
-
Functions for mapping field keys
Function Description fbget_fldkey()
Returns the field key value of a file name.
fbget_fldname()
Returns a field key name.
fbget_fldno()
Fetches the field number from a field key.
fbget_fldtype()
Fetches the type (integer) from a field key.
fbget_strfldtype()
Fetches the pointer value for the type from a field key.
-
Functions related to buffer allocation
Function Description fbisfbuf()
Checks whether the specified buffer is a field key buffer.
fbinit()
Initializes the memory space allocated to a field key buffer.
fbcalcsize()
Calculates the size of a field buffer.
fballoc()
Dynamically allocates a field key buffer.
fbfree()
Frees a field buffer.
fbget_fbsize()
Returns the size of a field key buffer in bytes.
fbget_unused()
Checks unused field buffer space.
fbget_used()
Returns used field key buffer space in bytes.
fbrealloc()
Adjusts the buffer size.
-
Functions for accessing and modifying fields
Function Description fbput()
Adds a field key to a field buffer.
fbinsert()
Specifies a field key and its location and saves the field value in a field buffer.
fbchg_tu()
Moves a specified field buffer before sending data.
fbdelete()
Deletes the field data of a buffer.
fbdelall()
Deletes all field values.
fbdelall_tu()
Deletes all field data enumerated in a field key array (fieldkey[]).
fbget()
Gets a field value in a buffer.
fbgetf()
Gets the field value of a specified field key in a field buffer.
fbget_tu()
Gets the value of a specific field key in a specified location.
fbnext_tu()
Gets the field values of a specific field key in a field buffer in order.
fbgetalloc_tu()
Internally allocates another buffer to save returned data and returns the pointer of the allocated buffer.
fbgetval_last_tu()
Gets the occurrence of a specific field key of a field buffer and recent data.
fbgetlast_tu()
Gets the most recent entered data of a field specified in a field buffer.
fbgetnth()
Searches a specific field value.
fbfldcount()
Returns the number of fields included in a specific buffer.
fbkeyoccur()
Returns the field number specified in a field key.
fbispres()
Checks whether the requested data exists in a field buffer.
fbgetval()
Returns the length of the requested data and a pointer to its location.
fbgetvall_tu()
Returns the actual value of a field in the long type.
fbupdate()
Updates the field value of a field key in a field buffer at a specified location.
fbgetlen()
Returns the first occurrence value of a specified field key in a field buffer.
-
Functions for conversion
Function Description fbtypecvt()
Converts a data type.
fbputt()
Attaches new data and its type into a field buffer.
fbget_tut()
Gets the field data of a specified location and specifies the field key type.
fbgetalloc_tut()
Converts the returned data type into a defined data type and internally allocates another buffer to save it.
fbgetvalt()
Returns a pointer to the returned value.
fbgetvali()
Returns the field data of the integer type.
fbgetvals()
Returns the field data of the string type.
fbgetvals_tu()
Returns the field data of the string type at a specified location.
fbgetntht()
Returns a converted value.
fbchg_tut()
Modifies the field key value from a specific starting point of a field buffer.
-
Function related to buffer operation
Function Description fbbufop()
Compares, copies, moves, and modifies the data of two field buffers.
-
I/O-related function
Function Description fbbufop_proj()
Modifies a buffer corresponding to a field key.
fbread()
Used together with the standard input/output library. Reads a field buffer from a file.
fbwrite()
Used together with the standard input/output library. Writes to a file.
fbprint()
Standard input/output. Outputs buffer data.
fbfprint()
Outputs the available data of a field buffer in a file string.
-
Error-related functions
Function Description fbstrerror()
Gets the error message that occurs while operating a field buffer in a string.
getfberrno()
Returns an error number.
-
Other functions
Function Description fbmake_fldkey()
Automatically creates a new field key without recording in FDLFILE.
fbftos()
Moves data saved in a field buffer to a C structure (stname).
fbstof()
Moves data saved in a C structure to a field buffer mapped to a structure file.
fbsnull()
Checks whether a member variable of a C structure mapped to the occurrence of the field key specified by a field buffer is NULL.
fbstelinit()
Initializes the field buffer and member variables of a C structure as NULL.
fbstinit()
Initializes the C structure mapped to a field buffer as NULL.
6. Error Message
6.1. X/Open DTP related Error
If an error occurs while using the standard interfaces provided by X/Open DTP and non-standard interfaces provided by the Tmax system, an error value is saved to a global variable called tperrno. By checking tperrno, a developer can handle the error.
The following is a list of tperrno error messages.
Error Message (tperrno) | Description |
---|---|
TPEBADDESC(2) |
Occurs when an invalid descriptor is used for an asynchronous or conversational type. |
TPEBLOCK(3) |
Occurs due to a network error. |
TPEINVAL(4) |
Occurs when an invalid argument is entered. |
TPELIMIT(5) |
Occurs when one or more various limit values provided by a system are exceeded. |
TPENOENT(6) |
Occurs when a service is not provided. |
TPEOS(7) |
Occurs when a connection cannot be established due to a system error. |
TPEPROTO(9) |
Occurs due to a protocol error. |
TPESVCERR(10) |
Occurs when a buffer of the Tmax system is damaged because an application program failed. |
TPESVCFAIL(11) |
Occurs due to a level service error of an application program. |
TPESYSTEM(12) |
Occurs due to a Tmax internal error (log message check). |
TPETIME(13) |
Occurs due to transaction timeout (BLOCKTIME). |
TPETRAN(14) |
Occurs when a transaction is cancelled due to a failure. |
TPGOTSIG(15) |
Occurs when a signal occurs. |
TPEITYPE(17) |
Occurs when an unregistered structure type or field key is used. |
TPEOTYPE(18) |
Occurs due to buffer usage or a type error. |
TPEEVENT(22) |
Occurs when an event occurs in the conversational mode. |
TPEMATCH(23) |
Occurs when a proper service does not exist for the tpdeq() function of RQ. |
TPENOREADY(24) |
Occurs when a server process is not ready. |
TPESECURITY(25) |
Occurs due to a security error. |
TPEQFULL(26) |
Occurs when the queue wait time of a server process exceeds timeout. |
TPEQPURGE(27) |
Occurs when an item in a queue is deleted due to a queue purge. |
TPECLOSE(28) |
Occurs when a connection to the Tmax system is released. |
TPESVRDOWN(29) |
Occurs when a server process is terminated due to an application program error. |
TPEPRESVC(30) |
Occurs when an error occurs while a previous service is processing. |
TPEMAXNO(31) |
Occurs when the number of concurrent users reaches the limit value. |
For more information about errors and handling methods, refer to Tmax Application Development Guide and Tmax Error Message Reference Guide. |
6.2. FDL-related Error
A FDL interface related error value is saved to a global variable called fberrno. By checking fberrno, a developer can handle the error.
The following is the list of fberror error messages:
Error Message (fberror) | Description |
---|---|
FBEBADFB(3) |
Occurs when an improper buffer (not a field key buffer) is used. |
FBEINVAL(4) |
Occurs when an improper argument is used. |
FBELIMIT(5) |
Occurs when one or more various limit values provided by a system are exceeded. |
FBENOENT(6) |
Occurs when a corresponding field key does not exist in a buffer. |
FBEOS(7) |
Occurs when an error occurs in the OS. |
FBEBADFLD(8) |
Occurs when an improper field key is used. |
FBEPROTO(9) |
Occurs due to a protocol error. |
FBENOSPACE(10) |
Occurs when there is insufficient buffer space. |
FBEMALLOC(11) |
Occurs when an error occurs while allocating memory. |
FBESYSTEM(12) |
Occurs when an error occurs in a system. |
FBETYPE(13) |
Occurs when an error occurs due to a type. |
FBEMATCH(14) |
Occurs when there is no matched value. |
FBEBADSTRUCT(15) |
Occurs when an unregistered structure is used. |
FBEMAXNO(19) |
Occurs when an error number that does not exist is used. |
For more information about errors and handling methods, refer to Tmax FDL Reference Guide and Tmax Error Message Reference Guide. |