Overview
This chapter introduces the features and interfaces provided by OSC.
1. Introduction to OSC Programming
You can create transaction services by developing new application programs or by migrating existing CICS application programs. OSC offers most of the resources provided by CICS, allowing programs to be migrated easily.
OSC Programming Features
The following are the features provided by OSC for the development of OSC application programs. Refer to OSC Programming Features for more information about each feature.
OSC Management Features
The following feature are provided for creating and managing OSC application programs. Refer to OSC Management Features for more information about each feature.
Category | Description |
---|---|
Program Control |
Provides functions for processing program execution. |
Interval Control |
Provides time-related functions. |
Task Control |
Provides functions for processing the connections between tasks. |
Dynamic Area Control |
Provides dynamic memory allocation and deletion functions. |
Temporary Storage Queue (TSQ) |
Provides queue functions for temporarily storing data. |
Transient Data Queue (TDQ) |
Provides queue functions for transferring data. |
Channels and Containers |
Provides functions for sending and receiving data between application programs. |
Journal Control |
Provides functions for logging system events and modifications. |
Data Management Features
The following features are provided in order to manage data in OSC application programs. Refer to Data Management Features for more information about each feature.
Category | Description |
---|---|
File Control |
Provides functions for managing Tmax VSAM (TSAM) data set access. |
Named Counter Services |
Provides counter management functions. |
Data Communication Features
The following features are provided to connect OSC application programs with users or other products. Refer to Data Communication Features for more information about each feature.
Category | Description |
---|---|
Terminal Control |
Provides terminal connection management functions. |
Mapping Support |
Provides map processing functions. |
SPOOL |
Provides the interface function for Tmax Job Entry Subsystem (TJES). |
Security Features
The OSC system provides user access and resource access services based on Tmax Access Control Facility (hereafter TACF), an OpenFrame security module. Refer to Security Features for more information about security features.
Database Features
The following interfaces are provided so that OSC application programs can access HiDB which is both a hierarchical database and a DL/I database of OpenFrame.
-
EXEC CICS interface
-
EXEC DLI interface
-
CBLTDLI interface
Most services are provided by OSC user application programs through either the EXEC CICS or EXEC DLI interfaces. OpenFrame/HiDB, however, can use either the EXEC DLI interface or CBLTDLI call interface.
Another interface, called EXEC SQL, is used by OSC application programs to access relational databases. However, that interface is beyond the scope of this guide. The access to relational databases is performed either by the EXEC SQL interface of the database or database management function of Tmax, TP-Monitor. The database to be used by the oscbuild tool must be specified when creating the binaries of the application server.
|
2. Executing OSC Application Program
Application program source files are pre-processed and compiled into binaries. For a new application program, the PROGRAM and TRANSACTION resource definitions must be registered. The binary will then be hot-deployed on an actual system through the deployment process. After the hot deployment, the application program can be run on the OSC application server.
Application programs are executed in the following manner:
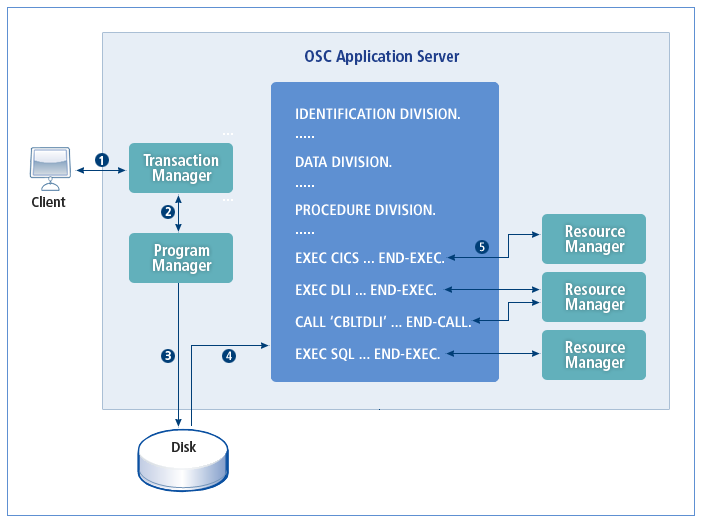
-
A client requests a transaction.
-
The application server selects a program according to the transaction request.
-
The application server sends a request to the disk to load the requested program.
-
The program is loaded from the disk.
-
The resource manager, service provider, and program are connected to each other.
3. EXEC CICS Interface
The EXEC CICS interface is divided into application programming commands and system programming commands.
-
Application programming commands
Commands used by developers to access resources for developing applications.
-
System programming commands
Commands for system programmers. The commands start with either INQUIRE or SET and are used to retrieve resource information.
The rest of this section explains the EXEC CICS interface syntax and describes the RESP and NOHANDLE options. The last part deals with the EXEC Interface Block (EIB) and the CICS Value Data Area (CVDA) that assist users in using the EXEC CICS interface.
3.1. EXEC CICS Interface Syntax
EXEC CICS follows the following syntax in COBOL source files:
EXEC CICS command [option …] END-EXEC.
Element | Description |
---|---|
command |
Enters a command. Required. |
option |
Depending on the given command, some options are valid or invalid, and some may be required while others are optional. Additionally, some options may require additional arguments. |
In the following example, a record that matches an RIDFLD of KEY-01, from file FILE01, is read into AREA-01 with the UPDATE method. Here, READ is the command and FILE, INTO, RIDFLD, and UPDATE are all options.
EXEC CICS READ FILE('FILE01') INTO(AREA-01) RIDFLD(KEY-01) UPDATE END-EXEC.
Refer to Application Programming Interface for more information about EXEC CICS syntax, as well as a list of options supported by OSC application servers. |
3.2. RESP Codes
Whenever executing an EXEC CICS command, the OSC application server returns an execution value, which is called an RESP code. An RESP code is defined in the system, and all values except NORMAL represent exception conditions. An RESP code indicates a general exception condition, while an RESP2 code provides detailed information.
RESP and RESP2 codes can be set in user-specified variables using the command option, or accessed through the EIBRESP and EIBRESP2 fields in the EIB. The RESP and RESP2 codes are then compared with a result of the DFHRESP macro function, which accepts a string corresponding to an RESP code and converts it to a binary value. The RESP code strings that DFHRESP uses are predefined in the system.
The following example shows how to handle the exception NOTFND. If the value in MY-RESP matches the value corresponding to NOTFND, an action will occur.
EXEC CICS ... RESP(MY-RESP) END-EXEC. IF MY-RESP=DFHRESP(NOTFND) THEN ... END-IF
If an RESP code is specified in a command, that command overrides the default action specified for that RESP code.
The OSC application server is designed to follow the same default actions as CICS. Refer to IBM CICS Application Programming Reference or IBM CICS System Application Programming Reference for more information. |
3.3. NOHANDLE Option
When an exception occurs while a command received through the EXEC CICS interface is being executed, the system will follow the default action as a response. In most cases, ABEND (abort) is carried out, but other actions may be taken depending on the exception.
You can modify the default action by using certain EXEC CICS commands. Refer to Exception Condition Handling Commands and ABEND Handling Commands for more information.
If the RESP or NOHANDLE option is specified for a command, it is assumed that the user will handle an exception condition and the default action is not taken.
3.4. EXEC Interface Block (EIB)
The EXEC Interface Block (EIB) is a system control block that provides an interface between user applications and the system. The EIB stores as fields the transaction identifier, transaction start time, terminal identifier, and command execution result values. These values, which are accessible by users, allow users to view detailed information about any transactions that are currently being processed.
The following is a list of EIB fields supported by the OSC application server.
Field | Description |
---|---|
EIBAID |
Last Attention Identifier (AID) value received from the terminal. |
EIBCALEN |
Contains the COMMAREA length passed to the corresponding program. |
EIBCOMPL |
Indicates whether all data has been received for the RECEIVE command. |
EIBCPOSN |
Cursor address (position) received from the terminal. |
EIBDATE |
Task start date. It is updated by the ASKTIME command. |
EIBFN |
Last command executed through the EXEC CICS interface. |
EIBRCODE |
Last return code from the EXEC CICS interface. |
EIBRECV |
Specifies whether the application program is to accept data with the RECEIVE command. |
EIBREQID |
Request identifier specified by the interval control command. |
EIBRESP |
Last RESP code returned by an EXEC CICS command. |
EIBRESP2 |
Last RESP2 code returned by an EXEC CICS command. |
EIBRSRCE |
Resource identifier that was last accessed. |
EIBTASKN |
Task number allocated to a task by the system. |
EIBTIME |
Task start time. It is updated by the ASKTIME command. |
EIBTRMID |
Identifier of the principal facility associated with a task. |
EIBTRNID |
Identifier of a task’s transaction. |
3.5. CICS-value Data Area (CVDA)
A CICS-value data area (CVDA) is a fullword string value that corresponds to a pre-defined numerical value in the system. When writing an OSC application program and calling a pre-defined value, the user can pass the CVDA of that value (a string) to the DFHVALUE macro function, which takes a numeric value represented by the CVDA, instead of manually entering it.
The CVDA values used in OpenFrame are the same as the IBM CICS CDVA values. For more information about them refer to IBM CICS Application Programming Reference. |
4. EXEC DLI Interface and CBLTDLI Invocation Interface
A DL/I interface is used to access HiDB from an OSC application program. The DL/I interfaces provided by OSC are EXEC DLI, a command level interface, and CBLTDLI, a COBOL level interface.
Refer to DL/I Database Features for more information about DL/I interface. |