Application Creation
This chapter describes how to create applications.
1. Process of Creating Applications
The process of creating applications can be separated into application design, programming, debugging, and execution.
Each step includes resources that must be defined in the OSI and programming that users randomly create.
Application Design
The application design process proceeds as follows:
-
A developer must decide what type of user server applications will run in.
There are two types of user server that process applications; MPP user servers and BMP user servers. It must be determined which server type will be implemented.
-
Once the type of the server is determined, the relationship between the application and the user server must be defined.
The relationship can be defined in the system resource definition.
The following is an example of system resource definition.
APPLCTN PSB=OIVPI001,PGMTYPE=(,,),SCHDTYP=PARALLEL TRANSACT CODE=OIVPMPP1,MSGTYPE=(SNGLSEG,RESPONSE,1),PRTY=(1,5), X MODE=SNGL APPLCTN PSB=OIVPI002,PGMTYPE=(TP,,1),SCHDTYP=PARALLEL TRANSACT CODE=OIVPMPP2,MSGTYPE=(SNGLSEG,RESPONSE,1),PRTY=(1,5), X MODE=SNGL,MAXRGN=1 APPLCTN PSB=OIVPIL05,PGMTYPE=BATCH,SCHDTYP=PARALLEL TRANSACT CODE=OIVPBMP5,MSGTYPE=(SNGLSEG,RESPONSE,1),PRTY=(1,5), X MODE=SNGL
-
Applications need to be designed with consideration to the program structure, taking maintenance and scalability into account, as well as module design. When designing applications, note the following.
-
Effectively use the CBLTDLI function call.
-
Minimize the number of times data is being input or output.
-
Consider the data structure of the database and the processing options (search, add, and delete).
-
Consider the configuration of the processing mode (interactive mode processing and general transaction mode).
-
Consider the message path.
-
Programming
Write OSI applications in COBOL and note the following.
-
Configuring the start conditions of the program.
-
Configuring DB-PCB, IO-PCB, and ALT-PCB.
-
Configuring the function call interface.
-
Configuring the checking status codes and handling errors.
-
Integrity during data processing. (e.g. Data length)
-
Configuring the termination conditions of the program. (e.g. Return code)
-
Regardless of input and output data size or SSA size, the data transfer area when programming must be large enough.
Batch Applications
Batch applications are implemented by tjesmgr, which is OpenFrame’s utility. The way of creating batch applications is the same as that of MPP applications. A difference is that it runs through JCL. tjesmgr is an utility to run JCL effectively.
Batch applications are not for online tasks. Therefore, there is no need to define the data area to communicate with terminals. Logic that can connect programs to databases, logic that can load messages on Message Queue (MQ), and logic that can stack data on MQ are only needed.
2. MPP Applications
Message Processing Program (MPP) and Message Processing Region (MPR) process messages in the IMS/DC Online environment. OSI separates them into the system server and the user server.
The following is a picture of the OSI system and application configuration of online environments.
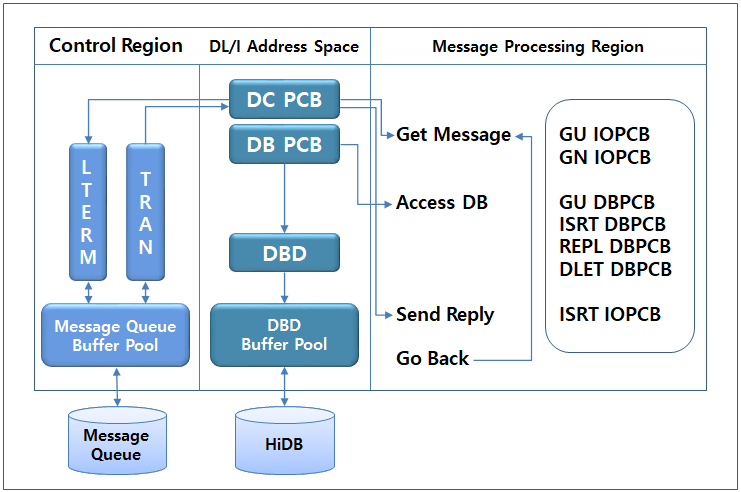
In general, the transaction input from the terminal (LTERM) is analyzed by the system server (Control Region), and the MPP application that processes this transaction is loaded and executed in the MPP user server, which is one of the user servers of OSI. The data area that this transaction delivers to the OSI system consists of a transaction code (up to 8 characters) and a data area.The system server acquires class information from the RunTime System Definition (RTSD) table using the received transaction code, and schedules it with the MPP user server that processes the class.
The MPP application can get the message input from the terminal with Get Unique(GU) Call. It enables to process multiple MPP applications by starting multiple user servers and to access the database at the same time. In addition to LTERM, MPP applications can be started between transactions using ALT-PCB.
2.1. MPP User Server Preparation
To run MPP user servers, Tmax environment file settings and an MPP start JCL file are required. OSI can start MPP servers that process different class information, by using Tmax’s target option with a OSIMPPSVR binary file.
Tmax Environment File Settings
Register user servers on the Tmax server to normally run OSI as it runs in the Tmax environment. The method of registering a server is the same as that of registering a server in Tmax. When registering Tmax servers, related services must be registered as well.
################################################################################ # OpenFrame OSI Dependent Region Servers # ################################################################################ OSIMPPSVR SVGNAME = svg_node1, MIN = 1, MAX = 10, RESTART = NO ################################################################################ # OSI, example in which IMSID is IMSA # ################################################################################ IMSASCHD SVGNAME = svg_node1, MAX = 1, SVRTYPE = UCS, RESTART = NO, TARGET = osisschd IMSACMMD SVGNAME = svg_node1, MAX = 1, SVRTYPE = UCS, RESTART = NO, TARGET = osicmdsv IMSAMPP_TCL1 SVGNAME = svg_node1, MIN = 1, MAX = 10, RESTART = NO, TARGET = OSIMPPSVR IMSAMPP_TCL2 SVGNAME = svg_node1, MIN = 1, MAX = 10, RESTART = NO, TARGET = OSIMPPSVR IMSAMPP_TCL3 SVGNAME = svg_node1, MIN = 1, MAX = 10, RESTART = NO, TARGET = OSIMPPSVR IMSAMPP_TCL4 SVGNAME = svg_node1, MIN = 1, MAX = 10, RESTART = NO, TARGET = OSIMPPSVR *SERVICE ################################################################################ # OSI USER APPLICATION SERVER DEFAULT # ################################################################################ OSIMPPSVR_SVC SVRNAME = OSIMPPSVR, SVCTIME=60
MPP Server Start JCL
The following is a JCL file that starts the MPP user server corresponding to a user server (Dependent Region) created by the administrator when a system server’s (Control Region) name is IMSA. (Classes to be processed are 1, 2, 3, and 4.)
//IMSAMSG JOB //STEP1 EXEC PGM=DFSRRC00, // PARM='MSG,001002003004,W00099000,,,R,,,,IMSA,,,,D2PA' //STEPLIB DD DISP=SHR,DSN=OSI.IMSA.STEPLIB //SYSPRINT DD SYSOUT=* //SYSOUT DD SYSOUT=* //SYSDBOUT DD SYSOUT=* //
2.2. MPP Programming
The basic MPP programing consists of GU and ISRT calls. When the program issues a GU call to process a message, the CBLTDLI function is called through IO-PCB. When a message consists of more than one segment, read the message using GN Call from the second segment. (In the case of a transaction using the SPA, read the SPA using GU and then process using GN). The results of the GU and GN Call are configured in the status code field of IO-PCB. The MPP program must check the status code after processing the call. When message segments are sent to the terminal or other transactions, configure the message segments in the data area in a specified format and call the CBLTDLI function using ISRT Call.
When a message is sent to another MPP program or terminal, specify the destination using ALT-PCB.
Note
Note the following during MPP programing.
-
MPP is dynamically loaded onto the empty user server whenever a transaction is input. After processing MPP is complete, the user server is allocated to another MPP program or changes to IDLE state. Therefore, information cannot be saved on the MPP user server. Use the SPA to save the data.
-
To run MPP, OSI has to do a lot of pre-processing. Once MPP is loaded, create a program that can process multiple messages. However, if too much time is spent processing messages, equalized transactions cannot be scheduled in accordance with the priority that is specified when the system is defined. In this case, use the PROCLIM specification in the TRANSACT macro.
-
If the database is updated using MPP, care will be needed because processing of the database is implemented. If applications are too big, the database will be exclusively accessed. This situation should be avoided.
-
To run equalized scheduling services, create MPP using multiple transactions rather than one transaction.
-
During the MPP process, check the status code. If there are any errors, further processing will be impossible. If it is necessary to exit a database while processing is being carried out, terminate it abnormally (ABEND).
-
If USE_DBD_DBMS_LOCK option in HIDB_DEFAULT section and hidb subject of OpenFrame configuration is set to YES, lock is created with the DBD name used by the transaction when the transaction starts, and lock is released when the transaction ends. For more information, refer to OpenFrame Configuration Guide.
2.3. Message Segment Format
The following describes receiving message segments, sending message segments, and message segments transferred between programs.
Received Message Segments
COBOL programs (applications) receive messages from terminals or from other transactions. Using the GU and GN Call of the CBLTDLI function, data is received in the data area that COBOL defines.
The message segment format that applications receive is as follows:
LL ZZ DATA
Field | Description |
---|---|
LL |
The length of a message segment that includes the length of the LL and ZZ fields. It is a binary number and is 2 Bytes. (LL = DATA length+4) For applications written by PL/I, it is 4 Bytes and its length is the actual length minus 2. |
ZZ |
It is an area for data access and is 2 Bytes. A developer does not need to configure it. If it is randomly modified, errors will occur. |
DATA |
A message segment that is input from the terminal. A message segment consists of a transaction code and a data area. Front 8Byte of the data that is sent to the terminal becomes a transaction name and the rest becomes the actual data. This is not configured by the OSI system but MFS Map information. For messages that are input as multiple message segments, a transaction code must be included within the first 8 Bytes of the first message segment. |
Sending Message Segment
When messages are sent to the terminal, other transactions, or other terminals in applications, send messages to the Destination that is configured in ALT-PCB or IO-PCB using the ISRT Call of the CBLTDLI function.
The format for sending message segments is as follows:
LL ZZ DATA
Field | Description |
---|---|
LL |
The length of a message segment that includes the length of the LL and ZZ fields. It is a binary number and is 2 Bytes. (LL = DATA length + 4) For applications written in PL/I, it is 4 Bytes long. This field must be configured in applications. |
Z1 |
It is an area for data access and is 1 Byte. In applications, 0 must be input as a binary number. |
Z2 |
Its length is 1 Byte. For paging logic, input X'40' if the start of a new page needs to be notified to MFS. Otherwise, input 0 as a binary number. Refer to "MFS Reference Guide" for detailed information. |
DATA |
A data area that is sent to the specific Destination(the current terminal, other transactions, and other terminals). The length of a message segment depends on the Destination. Multiple message segments are fine as well. |
Message Segments between Programs
Messages can be sent to another program from a specific program. Messages are transmitted between programs by using the previously mentioned method of sending message segments.
The format of delivering message segments between programs is as follows:
LL ZZ DATA
Field | Description |
---|---|
LL, ZZ |
The format is the same as that of sending message segments. |
DATA |
Sends messages to the target program. |
Note
Note the following when using message segments that are delivered between programs. The ALT-PCB that applications use must be defined at PSB in advance and PCBs (LINKAGE SECTION and ENTRY syntax) that applications use must be defined in the same order of PCBs which are defined in PSB.
-
When application AP1 sends a message to application AP2, use ALT-PCB. After configuring the Destination of ALT-PCB as the name of AP2, use the DL/I function in the order of CHNG, ISRT, and PURG.
-
When receiving a message from AP2, specify IO-PCB to get the message. ALT-PCB of AP1 must be configured at IO-PCB so that data can be normally received (data structure integrity).
-
If a message needs to be sent to AP1 after processing a received message in AP2, specify the ALT-PCB of AP1. In this case, AP1 receives a message from AP2 and processes it. When a message is output to the terminal, send it by specifying IO-PCB. AP2 can receive a message in the case of MPP or BMP.
3. BMP Applications
Unlike MPP, a user uses data management in addition to the ability to access a database and access user data set in OSI. How to process a database or a message is the same as MPP. A difference from MPP programing is that data which is loaded onto MQ is not processed in real time and is processed by running BMP applications through JCL when required by a user.
For example, when the output processed through the MPP application is sent to the BMP application using ALT-PCB (Alternative Program PCB), OSI loads the result into an MQ table, waits, and then when the user executes the corresponding BMP application with JCL, the MQ table sends all messages loaded in the BMP application to the destination.
The following is an example of JCL that starts the BMP program.
<OSIBMP.jcl>
//OSIBMPT JOB CLASS=A,MSGCLASS=X,MSGLEVEL=(1,1) //TSTEP1 EXEC PGM=DFSRRC00, // PARM=(BMP,OIVPIL04,,OIVPBMP4,,,,,,,,,,IMSA,) //SYSOUT DD SYSOUT=*
The following is an example of implementing a tjesmgr utility that executes above JCL.
$ tjesmgr run OSIBMP.jcl > Command : [run OSIBMP.jcl] Node name : NODE1 /home/oframe/OpenFrame/volume_default/SYS1.JCLLIB/OSIBMP.jcl is submitted as OSIBMPT(JOB00001). $ tjesmgr ps > Command : [ps] JOBNAME JOBID CLASS STATUS RC NODE JCL ------------------------------------------------------------------------------ OSIBMPT JOB00001 A Done R00000 NODE1 OSIBMP.jcl