Built-in Functions
This chapter describes how to declare and invoke the built-in functions, pseudo variables, and subroutines of OpenFrame PL/I. It also explains how to use the functions of each category.
1. Declaring and Invoking Functions
This chapter describes how to declare and invoke functions.
1.1. Declaring Functions
Built-in functions, pseudo variables, and subroutines can be declared contextually or explicitly. The abbreviations for built-in functions have separate declarations (explicit or contextual) and name ranges.
For example, the following is not a multiple declaration:
DCL (DIM, DIMENSION) BUILTIN;
In the following example, a multiple declaration is possible even through BIN is an abbreviation of binary:
DCL BINARY FILE; X = BIN(Y,3,4);
1.1.1. BUILTIN attribute
The BUILTIN attribute specifies a name is a built-in function, pseudo variable, or a subroutine.
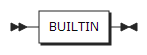
A built-in NAME can be defined by a programmer.
The following is an example:
Accccccccccccccccccccc PROCEDURE; DECLARE SQRT FLOAT BINARY; /*SQRT is a name defined by the programmer.*/ X = SQRT; /*Assigns the user-defined name of SQRT to the variable X.*/ B : BEGIN; DECLARE SQRT BUILTIN; /*SQRT is a BUILTIN attribute.*/ Y = SQRT(Z); /*References to the build-in function, not to the user-defined SQRT in in the second line.*/ END B; END A;
2. Subroutines
Unlike built-in functions, built-in subroutines perform tasks that require results to be returned.
The following describes each subroutine. For more information, refer to the relevant section.
Subroutine | Description |
---|---|
Cancels auto restart facility. |
|
Specifies a checkpoint for auto restart. |
|
Dumps the information such as currently open files and the calling path to the current location. |
|
Restarts program execution. |
|
Sets the PL/I return code value. |
|
Uses DFSORT, which sorts an input file, to create a sorted output file. |
|
Creates sorted records that are processed by an E35 PL/1 termination procedure that uses DFSORT, which sorts input records provided by an E15 PL/I termination procedure.
|
2.1. PLICANC
PLICANC cancels the auto restart facility.
OpenFrame PL/I does not support PLICANC, and an error message is thrown if the subroutine is called.
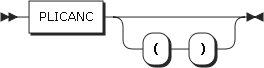
2.2. PLICKPT
PLICKPT specifies a checkpoint for auto restart.
OpenFrame PL/I does not support PLICKPT, and an error message is thrown if the subroutine is called.
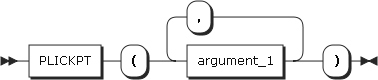
-
Argument
Component Description argument-1
Checkpoint expressions such as ddname and check-id
2.3. PLIDUMP
PLIDUMP dumps the information of currently open files, the calling path to the current location, etc. PLIDUMP allows users to obtain a formatted dump of selected parts of storage used by a program.
OpenFrame PL/I does not support PLIDUMP, and an error message is thrown if the subroutine is called.

-
Argument
Component Description argument_1
Expression of a string
argument_2
Expression of a string
2.4. PLIREST
PLIREST restarts program execution.
OpenFrame PL/I does not support PLIREST, and an error message is thrown if the subroutine is called.
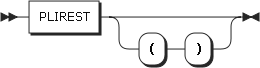
2.5. PLIRETC
PLIRETC allows users to set a return code that can be recognized by the program that invokes the PL/I program or another PL/I procedure through the built-in PLIRETV function.

-
Argument
Component Description argument_1
Expression that calculates a return code that contains FIXED BINARY(31,0).
2.6. PLISRTA
PLISRTA creates a sorted output file through DFSORT that sorts an input file.
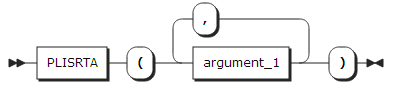
-
Argument
Component Description argument_1
Expression related to a sort operation such as SORT and READ statements.
2.7. PLISRTD
PLISRTD creates sorted records that are processed by an E35 PL/1 termination procedure. The termination procedure uses a DFSORT that sorts input records provided by an E15 PL/I termination procedure.
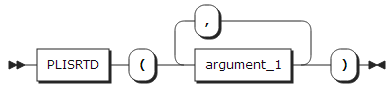
-
Argument
Component Description argument_1
Expression related to a sort operation such as SORT and READ statements.
3. Pseudo Variables
Pseudo variables represent receiving fields. Pseudo variables cannot be nested.
A pseudo variable can only appear in the following cases:
-
When it is placed on the left side of an assignment statement.
-
When only SUBSTR is a target in a DO-specification.
-
When it is in the data list of a GET statement or a STRING option of a PUT statement.
-
When it is the string name of a KETO or REPLY option.
The following describes each pseudo variable. For more information, refer to the relevant section.
Function | Description |
---|---|
Sets a character string that raises a CONVERSION condition. |
|
Sets a string that raises a CONVERSION condition. |
|
Assigns a string that is the concentration of all the elements of a string. |
|
Assigns a substring. |
|
Assigns a bit string that is the internal form of a value. |
3.1. ONCHAR
ONCHAR sets a character string that raises a CONVERSION condition.
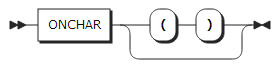
-
Return Value
-
ONCHAR pseudo variable sets the current value of an ONCHAR built-in function. The assigned element value is converted to a character value of length 1. The new character is used when data conversion is tried again.
-
ONCHAR pseudo variables cannot be used out of context.
-
3.2. ONSOURCE
ONSOURCE sets a character string that raises a CONVERSION condition.
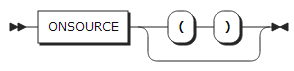
-
Return Value
-
ONSOURCE pseudo variable sets the current value of the ONSOURCE built-in function. The assigned element value is converted to a string. If necessary, the element value will be padded on the right side with blanks, or truncated to match the length of the field in which the CONVERSION condition was raised. The string is used when data conversion is tried again.
-
ONSOURCE pseudo variable cannot be used out of context.
-
A string assigned during data conversion is processed as a single data-item.
If data conversion occurs when GET LIST or GET DATA statements are executed, an error collection process must not assign a string that contains one or more data-items. The blank space or comma in a string may cause a conversion to execute again.
-
3.3. STRING
STRING assigns a string that is the concentration of all the elements of a string.

-
Argument
Component Description argument_1
Aggregate or element reference
-
The basic elements of each argument_1 must be a bit string or a character string.
-
-
Return Value
-
STRING pseudo variables assigns argument_1 as a string if argument_1 is a scalar string. The remainder of argument 1 is padded with blank spaces or bit 0. If it is a variable length string, the length will become 0.
-
STRING pseudo variables cannot be used out of context.
-
3.4. SUBSTR
SUBSTR assigns a substring.

-
Argument
Argument Description argument_1
String expression
-
argument_1 cannot be a numeric character.
argument_2
Expression
-
argument_2 that can be converted to a fixed binary value specifies the starting position of a substring in argument_1.
argument_3
Expression
-
argument_3 specifies the length of a substring in argument_1. It can be converted to a fixed binary value.
-
If the value of argument_3 is set to 0, a null string will be returned. If argument_3 is omitted, a substring returned will be position argument_2 in argument_1, to the end of argument_1.
argument_2 and argument_3 can be an array only when argument_1 is an array.
-
-
Return Value
-
SUBSTR pseudo variable assigns a value to a substring specified by argument_2 and argument_3 in argument_1.
-
The remainder of argument_1 is not changed. The assignment to the changed string does not change the length of the string.
-
3.5. UNSPEC
UNSPEC assigns a bit string that is the internal form of values.

-
Argument
Component Description argument_1
Reference
-
Return Value
-
The UNSPEC pseudo variable directly assigns a bit value to argument_1 without conversion. If necessary, the bit value will be padded to the right with 'O’B to match the length of argument_1.
-
If argument_1 is a string of variable length, a 2 byte prefix will be included in the part where a bit value is assigned. If argument_1 is an area, its control information will be included in the receiving field.
-
The use of UNSPEC may affect the portability of a developed program. |
4. Built-in Functions
This section describes how to use each built-in functions.
Built-in functions, except for subroutines and pseudo variables, are divided as follows:
4.1. Arithmetic Built-in Functions
Arithmetic built-in functions determine the properties of arithmetic values and perform arithmetic operations. Some of the arithmetic functions determine their resulting data types using one or more arguments. If multiple arguments have different data types, they are converted according to the data conversion rule.
The following describes each function. For more information, refer to the relevant section.
Function | Description |
---|---|
Calculates the absolute value of an argument. |
|
Calculates the smallest integer value greater than or equal to an argument. |
|
Calculates the largest integer value less than or equal to an argument. |
|
Calculates the largest value from two or more arguments. |
|
Calculates the smallest value from a set of one or more arguments. |
|
Calculates the smallest non-negative value that must be subtracted from the first argument to make it divisible by the second argument. |
|
Rounds off an argument to a specified place. |
|
Returns a -1, 0, or 1 according to whether an argument is negative, zero, or positive. |
|
Truncates an argument to make it an integer value. |
4.1.1. ABS
The ABS function calculates the absolute value of an argument.

-
Argument
Argument Description argument_1
Expression.
-
Return Value
-
The absolute value (positive) of argument_1.
-
The result mode is REAL. The result has the base, scale, and precision values of argument_1 except when argument_1 is COMPLEX FIXED(p,q).
-
4.1.2. CEIL
The CEIL function calculates the smallest integer value greater than or equal to an argument.

-
Argument
Argument Description argument_1
Real expression.
-
Return Value
-
The smallest integer value greater than or equal to argument_1.
-
A result has the mode, base, scale, and precision of argument_1.
-
The precision of a result is as follows except when the precision of argument_1 is fixed-point with a precision (p,q).
(min(N, max(p-q+1)),0)
N is the maximum number of allowed digits.
-
4.1.3. FLOOR
The FLOOR function calculates the largest integer value less than or equal to an argument.

-
Argument
Argument Description argument_1
Real expression.
-
Return Value
-
The largest integer value less than or equal to argument_1.
-
A result has the mode, base, scale, and precision of argument_1.
-
The precision of a result is as follows except when the precision of argument_1 is fixed-point with a precision (p,q).
(min(n,max(p-q+1,1)),0)
n is the maximum number of allowed digits.
-
4.1.4. MAX
The MAX function calculates the largest value from two or more arguments.

-
Argument
Argument Description argument_1
Expression.
argument_2
Expressions.
All arguments must be real. A result must be real with the base and scale of an argument. The number of arguments can be up to 64.
-
Return Value
-
The largest value from two or more arguments.
-
If only argument-1 is specified and argument-2 is omitted, argument-1 becomes the return value.
-
If arguments are fixed-point with precisions (p1,q1),(p2,q2),…,(pn,qn), the precision of a result will be as follows:
(min(N,max(p1-q1,p2-q2,...,pn-qn) + max(q1,q2,...,qn)),max(q1,q2,...,qn))
N is the maximum number of allowed digits.
-
If arguments are floating-point with precisions p1,p2,..,pn, the precision of a result will be as follows:
max(p1,p2,...,pn)
-
4.1.5. MIN
The MIN function calculates the smallest value from a set of one or more arguments.

-
Argument
Argument Description argument_1
Expression.
argument_2
Expression.
All arguments must be real. A result must be real with the base and scale of an argument. The number of arguments can be up to 64.
-
Return Value
-
The smallest value from two or more arguments.
-
If only argument-1 is specified and argument-2 is omitted, argument-1 becomes the return value.
-
If arguments are fixed-point with precisions (p1,q1),(p2,q2),…,(pn,qn), the precision of a result will be as follows:
(min(N,max(p1-q1,p2-q2,...,pn-qn) + max(q1,q2,...,qn)),max(q1,q2,...,qn))
N is the maximum number of allowed digits.
-
If arguments are floating-point with precisions p1,p2,..,pn, the precision of a result will be as follows:
max(p1,p2,...,pn)
-
4.1.6. MOD
The MOD function calculates the smallest non-negative value that must be subtracted from a first argument to make it divisible by a second argument.

-
Argument
Argument Description argument_1
Real expression.
argument_2
Real expression.
-
If argument_2 is 0, the ZERODIVIDE condition will occur.
-
-
Return Value
-
The smallest non-negative value R in the formula (argument_1 - R) / argument_2 = n, where n is an integer.
-
A result R that is real with a base and scale of arguments.
If the result is floating-point, its precision will be greater than those of argument_1 and argument_2.
If the result is fixed-point, its precision will be as listed below when precisions of argument_1 and argument_2 are (p1,q1) and (p2,q2) respectively.
(min(n,p2-q2+max(q1,q2)),max(q1,q2))
-
If the scaling factors of argument_1 and argument_2 are different fixed-points, an argument with the smaller scaling factor will be converted to a larger scaling factor before R is calculated. If the conversion fails, the result will be unpredictable.
-
4.1.7. ROUND
The ROUND function rounds off an argument to a specified place.

-
Argument
Argument Description argument_1
Real expression.
-
If argument_1 is negative, its absolute value will be rounded off and the sign will be restored.
argument_2
Integer with an optional sign.
-
Specifies the place in which rounding will be calculated.
-
-
Return Value
-
The value of argument_1 rounded off to a place specified by argument_2. The result will have the mode, base, and scale of argument_1.
-
If the precision of argument_1 is (p,q), then the precision of a FIXED result will be as follows:
(max(1,min(p-q+1+argument_2,N)),argument_2)
N is the maximum number of allowed digits. Therefore, argument_2 specifies the scaling factor of a result.
-
argument_2 must follow the limits of scaling factor for FIXED data. If it is greater than 0, rounding is calculated at the (argument_2) digit to the right of the place. If it is 0 or negative, rounding is calculated at the (1-argument_2) digit to the left of the place.
-
4.1.8. SIGN
The SIGN function returns -1, 0, or 1 according to whether an argument is negative, zero, or positive.

-
Argument
Argument Description argument_1
A real expression.
-
Return Value
-
A REAL FIXED BINARY value. If argument_1 is negative, zero, or positive, the result will be -1, 0, or 1, respectively.
-
4.1.9. TRUNC
The TRUNC function truncates an argument to make it an integer value.

-
Argument
Argument Description argument_1
Real expression.
-
Return Value
-
The integer value of truncated argument_1.
-
If argument_1 is 0 or positive, the result will be the largest value less than or equal to argument_1. If it is negative, the result will be the smallest integer value greater than or equal to argument_1.
-
A result will have a base, mode, scale, and precision.
-
The precision of a result will be as follows except when argument_1 is a fixed point with a precision (p,q).
(min(N,max(p-q+1,1)),0)
N is the maximum number of allowed digits.
-
4.2. Array-handling Built-in Functions
Array-handling built-in functions handles array arguments and returns element values.
The following briefly describes each function. For more information, refer to the relevant section.
Function | Description |
---|---|
Calculates bitwise AND on all elements of the dimension. |
|
Calculates bitwise OR on all elements of the dimension |
|
Returns a value that specifies the number of elements of the dimension. |
|
Returns a value that specifies the upper bound of the dimension. |
|
Returns a value that specifies the lower bound of the dimension. |
|
Calculates sum of all the elements in the dimension. |
4.2.1. ALL
The ALL function calculates a bitwise AND for all elements of an argument.

-
Argument
Argument Description argument_1
Computational array expression.
-
If argument_1 is not a bit string array, it will be converted to a bit string array.
-
-
Return Value
-
A bit string in which each bit is 1, if a corresponding bit in each element of argument_1 exists and is 1.
-
The length of a result that is the same as that of the longest element.
-
4.2.2. ANY
The ANY function calculates a bitwise OR for all elements of an argument.

-
Argument
Argument Description argument_1
Computational array expression.
-
If argument_1 is not a bit string array, it will be converted to a bit string array.
-
-
Return Value
-
A bit string in which each bit is 1, if a corresponding bit in each element of argument_1 exists and is 1.
-
The length of a result that is the same as that of the longest element.
-
4.2.3. DIMENSION (Abbreviation: DIM)
The DIMENSION function returns a value that specifies the number of elements of the dimension

-
Argument
Argument Description argument_1
Array reference expression.
-
argument_1 cannot have a dimension less than specified by argument_2.
argument_2
Expression that specifies a particular dimension of argument_1.
-
If necessary, argument_2 will be converted to a FIXED BINARY(31,0). argument_2 must be greater than or equal to 1.
-
argument_2 can be omitted if argument_1 is one-dimensional. (Default value: 1)
-
-
Return Value
-
A FIXED BINARY(31,0) value that specifies the number of elements in the specified dimension (argument_2) of the given array (argument_1).
-
An undefined value is returned if argument_2 is greater than the number of dimensions of argument_1. It is recommended to use LBOUND and HBOUND instead of DIMENSION.
-
4.2.4. HBOUND
The HBOUND function returns a value that specifies the upper bound of dimension the second argument of the first argument.

-
Argument
Argument Description argument_1
Array reference expression.
-
argument_1 cannot have dimensions less than argument_2.
argument_2
Expression that specifies a particular dimension of argument_1.
-
If necessary, argument_2 will be converted to a FIXED BINARY(31,0). argument_2 must be greater than or equal to 1.
-
argument_2 can be omitted if argument_1 is on-dimensional. (Default value: 1)
-
-
Return Value
-
A FIXED BINARY value that specifies the upper bound of dimension argument_2 of argument_1.
-
4.2.5. LBOUND
The LBOUND function returns a value that specifies the lower bound of dimension the second argument of the first argument.

-
Argument
Argument Description argument_1
Array reference expression.
-
argument_1 cannot have dimensions less than argument_2.
argument_2
Expression that specifies a particular dimension of argument_1.
-
If necessary, argument_2 will be converted to a FIXED BINARY(31,0). argument_2 must be greater than or equal to 1.
-
argument_2 can be omitted if argument_1 is on-dimensional. (Default value: 1)
-
-
Return Value
-
A FIXED BINARY value that specifies the lower bound of dimension argument_2 of argument_1.
-
4.2.6. SUM
The SUM function calculates the sum of all elements in an argument.

-
Argument
Argument Description argument_1
Array expression.
-
If elements of argument_1 are strings, they will be converted to fixed-point integers.
-
If elements of argument_1 are fixed-point, the precision of a result will be (N,q), where N is the maximum number of allowed digits, and q is the scaling factor of argument_1.
-
If elements of argument_1 are floating-point, the precision of a result will be the same as that of argument_1.
-
-
Return Value
-
Sum of all the elements in argument_1. The result will have the base, mode, and scale of argument_1.
-
4.3. Buffer-management Built-in Functions
Buffer-management built-in functions are used on the buffer specified by the given byte count and address.
The following describes each function. For more information, refer to the relevant section.
Function | Description |
---|---|
Returns a character string with the hexadecimal representation of the value of the buffer. |
4.3.1. HEXIMAGE
The HEXIMAGE function returns a character string with the hexadecimal representation of the value of the buffer at the specified location.

-
Argument
Argument Description argument-1
Restricted expression that contain locator type such as a POINTER or OFFSET type.
-
An OFFSET type must have an AREA attribute.
argument-2
Expression
-
Must be a computational type and is converted to a FIXED BINARY(31,0) type.
argument-3
If argument-3 is specified, it must be a CHARACTER(1) NONVARYING type.
-
-
Return Value
-
If argument-3 is not specified
A character string with the hexadecimal representation of [argument-2] byte of the variable value at location specified by argument-1. The length of the return value is 2 * [argument-2].
-
If argument-3 is specified
Same as when argument-3 is not specified (above case) except that the character specified by argument-3 is inserted after every set of eight characters. The length of the return value is (2 * [argument-2]) + ([argument-2] - 1) / 4.
-
4.4. Condition-handling Built-in Functions
Condition-handling built-in functions enable users to determine the reason why a condition occurs. The functions are valid only within the scope of an ON-unit or the following conditions that occurs dynamically:
-
A condition specific for a built-in function
-
The ERROR or FINISH condition that occurs as an implicit action.
The following describes each function. For more information, refer to the relevant section.
Function | Description |
---|---|
Returns the field value that caused a NAME condition as a character string. |
|
Returns the character that caused a CONVERSION condition. |
|
Returns a condition code. |
|
Returns the name of a file in which a condition occurred. |
|
Returns the name of a procedure in which a condition occurred. |
|
Returns the field value on which the CONVERSION condition occurred as a character string. |
4.4.1. DATAFIELD
The DATAFIELD function returns the field value that caused a NAME condition as a character string.
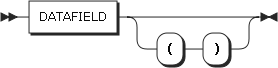
-
Return Value
-
A character string value of the field where the condition occurred.
-
If the character string contains DBCS identifiers, GRAPHIC data, or mixed character data, a mixed character string is returned.
-
If DATAFIELD is used out of context, a null string is returned.
-
4.4.2. ONCHAR
The ONCHAR function returns a character that causes a CONVERSION condition.
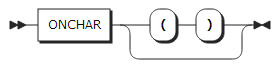
-
Return Value
-
A character string with the length of 1 that includes the character that caused a CONVERSION condition. It will be in context of an ON-unit for the CONVERSION condition or for the ERROR or FINISH conditions, which will occur as an implicit action for the CONVERSION condition.
-
A blank if the ONCHAR function is used out of context.
-
4.4.3. ONCODE
The ONCODE function returns a condition code. This function can be used to distinguish various cases that cause a specific condition. For codes corresponding to detected errors and conditions, refer to the specific condition.
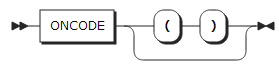
-
Return Value
-
A condition code that is a fixed-point binary value that depends on the reason why the last condition occurred. It will be in context of any ON-unit or its dynamic descendant.
-
Zero if the ONCODE function is used out of context.
-
4.4.4. ONFILE
The ONFILE function returns the name of a file in which a condition occurred.
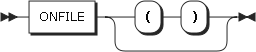
-
Return Value
-
A character string with the value of the name of a file in which an input or output condition occurred. If the name is DBCS, a character string that includes both upper and lower cases has been returned. It will be in context of any ON-unit or its dynamic descendant.
-
A null character if the ONFILE function is used out of context.
-
4.4.5. ONLOC
The ONLOC function returns the name of a procedure in which a condition occurred.
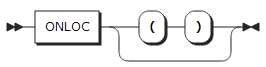
-
Return Value
-
A character string with the value of the name of the entry-point used to call the current procedure in which a condition occurred.
-
A null character if the ONLOC function is used out of context. The ONLOC function will alway return the leftmost name from multiple labels regardless of a name in a CALL or GOTO statement.
-
4.4.6. ONSOURCE
The ONSOURCE function returns the field value on which the CONVERSION condition occurred as a character string.
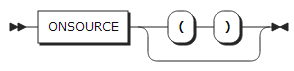
-
Return Value
-
A character string with the value of contents in which the CONVERSION condition occurred. It will be in context of an ON-unit for a CONVERSION condition, or for the ERROR or FINISH conditions that will occur as an implicit action for the CONVERSION condition.
-
A null character if the ONSOURCE function is used out of context.
-
4.5. Date/time Built-in Functions
Date/time built-in functions manipulate or return date and time information as days, seconds, and date and time stamps. In some of functions, the date and time patterns to be used can be specified. The time zone and accuracy for the functions depend on systems.
The formats representing date and time are as follows:
Format | Description |
---|---|
YYYY |
Four-digit year |
YY |
Two-digit year |
ZY |
Two-digit year with any leading zero suppressed |
MM |
Two-digit month |
ZM |
Two-digit month with any leading zero suppressed |
MMM |
Three-letter month (Ex: DEC) |
Mmm |
Three-letter month (Ex: Dec) |
DD |
Two-digit day within a given month |
ZD |
Two-digit day within a given month with any leading zero suppressed |
DDD |
Number of days within a given year |
HH |
Number of hours within a given day |
MI |
Number of minutes within a given hour |
SS |
Number of seconds within a given minute |
999 |
Number of milliseconds within a given second |
Upper and lower cases must be exactly matched in 'MMM' and 'Mmm'. The only supported pattern that can use 'HH', 'MM', 'SS', or '999' is 'YYYYMMDDHHMISS999'. |
The patterns representing date and time are as follows:
Type | Four-digit Years | Two-digit Years |
---|---|---|
Year first |
YYYYMMDD YYYYMMMDD YYYYMmmDD YYYYDDD YYYYMM YYYYMMM YYYYMmm YYYY YYYYMMDDHHMISS999 |
YYMMDD YYMMMDD YYMmmDD YYDDD YYMM YYMMM YYMmm YY |
Month first |
MMDDYYYY MMMDDYYYY MmmDDYYYY MMYYYY MMMYYYY MmmYYYY |
MMDDYY MMMDDYY MmmDDYY MMYY MMMYY MmmYY |
Day first |
DDMMYYYY DDMMMYYYY DDMmmYYYY DDDYYYY |
DDMMYY DDMMMYY DDMmmYY DDDYY |
DB2 format |
YYYY-MM-DD MM/DD/YYYY DD.MM.YYYY |
YY-MM-DD MM/DD/YY DD.MM.YY |
Without zeros |
ZY-ZM-ZD YY-ZM-ZD ZM/ZD/ZY ZM/ZD/YY ZD.ZM.ZY ZD.ZM.YY |
If day is omitted from a pattern, it will be assumed as 1. If both month and day are omitted, they will be assumed as 1. On input, a date value with "without zeros" pattern can be less than 8 characters long. For example, the date "Jan 20, 2009" can be represented as "9-1-20" to match the pattern "ZY-ZM-ZD". On output, the string with "without zeros" pattern will always be 8 characters long with any suppressed zeros replaced by a blank space.
The following describes each function. For more information, refer to the relevant section.
Function | Description |
---|---|
Returns the current date in the YYMMDD format. |
|
Returns the current date and time in the user-specified format or the YYYYMMDDHHMISS999 default format. |
|
Returns the total number of days of the current date or date with the specified format. |
|
Returns the current time in the HHMISS999 format. |
|
Returns the day of the week of the current day or the specified DAYS value. |
4.5.1. DATE
The DATE function returns the current date in the YYMMDD format.
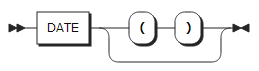
-
Return Value
-
A character string with the length of 6 that contains the date in the YYMMDD format.
-
4.5.2. DATETIME
The DATETIME function returns the current date and time in a user-specified format or the YYYYMMDDHHMISS999 default format.

-
Argument
Argument Description argument_1
Expression.
-
If argument_1 exists, it will specify the date/time pattern in which a date is returned.
-
If argument_1 is omitted, the default date/time pattern will be YYYYMMDDHHMISS999.
-
argument_1 must be computational and have a character type. Otherwise, it will be converted to a character.
-
-
Return Value
-
A character string timestamp of the current day’s date in a user-specified format or the default format.
-
For a list of allowed patterns, refer to the "Date/Time Patterns" table.
-
4.5.3. DAYS
The DAYS function returns the total number of days of the current or specified date in Lilian format.
-
Lilian date
Number of days that have passed since 10/14/1582. If the given date is 10/15/1582, then DAYS is 1.

-
Argument
Argument Description argument-1
String expression of a date.
-
If omitted, the date returned by the DATETIME() built-in function is used.
argument-2
Date/time pattern
-
For allowed patterns, refer to the "Date/Time Patterns" table.
-
Must be a computational type.
-
This is converted to a character string.
-
If omitted, YYYYMMDDHHMISS9999 is used.
argument-3
Century window to be used If the year format in argument-2 is 'YY'.
-
If argument-3 is a positive number
argument-3 is used as the century window value to determine the 100-year interval for 'YY'. For example, if argument-3 is 1950, the range of years for 'YY' is from 1950 to 2049.
-
If argument-3 is 0 or a negative number
argument-3 + system supplied year is used as the century window to determine the 100-year interval for 'YY'. For example, if the system supplied year is 2017 and argument-3 is -3, century window value is 2017 - 3 = 2014, and the 100-year interval is from 2014 to 2113.
-
If argument-3 is omitted
the default century window value is 1950.
-
-
Return Value
-
The number of days from the start of the Lilian calendar to argument-1 as a FIXED BINARY(31,0) type.
-
4.5.4. TIME
The TIME function returns the current time in the HHMISS999 format.
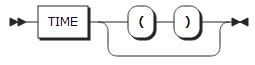
-
Return Value
-
A character string timestamp in the HHMISS999 format.
-
4.5.5. WEEKDAY
The WEEKDAY function returns the day of the week of the current day or the specified DAYS value.
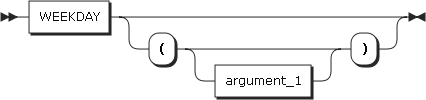
-
Argument
Argument Description argument-1
Expression
-
Must be a computational type and is converted to FIXED BINARY(31,0) type if necessary.
-
If omitted, the value returned by the DAYS() built-in function is used.
-
If argument-1 is omitted and the current date is not available from the system, 0 is returned.
-
-
Return Value
-
The default value or the day of the week of argument-1 as a FIXED BINARY(31,0) type.
1 = Sunday 2 = Monday ... 7 = Saturday
-
4.6. Input/output Built-in Functions
Input/output built-in functions determine the current state of a file.
The following describes the function. For more information, refer to the relevant section.
Function | Description |
---|---|
Returns the current line number of a specified file. |
4.6.1. LINENO
The LINENO function returns the current line number of a specified file.

-
Argument
Argument Description argument_1
File reference expression.
-
Return Value
-
The REAL FIXED BINARY value of the current line number of argument_1.
-
A file that must be open and have the PRINT attribute. If a file is not open or does not have the PRINT attribute, 0 will be returned.
-
4.7. Mathematical Built-in Functions
Mathematical built-in functions operate on floating-point values to generate a floating-point result. If an argument is not a floating-point value, it will be converted to a floating-point.
The following describes each function. For more information, refer to the relevant section.
Function | Description |
---|---|
Calculates the inverse (arc) cosine of an argument in radians. |
|
Calculates the inverse (arc) sine of an argument in radians. |
|
Calculates the inverse (arc) tangent of an argument or a ratio of arguments in radians. |
|
Calculates the inverse (arc) tangent of an argument or a ratio of arguments in degrees. |
|
Calculates the cosine of an argument in radians. |
|
Calculates the cosine of an argument in degrees. |
|
Calculates the base, e, of the natural logarithm system raised to the power of an argument. |
|
Calculates the natural logarithm of an argument. |
|
Calculates the common logarithm of an argument. |
|
Calculates the sine of an argument in radians. |
|
Calculates the sine of an argument in degrees. |
|
Calculates the positive square root of an argument. |
|
Calculates the tangent of an argument in radians. |
|
Calculates the tangent of an argument in degrees. |
4.7.1. ACOS
The ACOS function calculates the inverse (arc) cosine of an argument in radians.

-
Argument
Argument Description argument_1
Real expression, where ABS(argument_1) ≤ 1.
-
Return Value
-
A real floating-point value that is an approximation of the inverse (arc) cosine of argument_1 in radians.
-
A result that is in the range of 0 ≤ ACOS(argument_1) ≤ π and has the base and precision of argument_1.
-
4.7.2. ASIN
The ASIN function calculates the inverse (arc) sine of an argument in radians.

-
Argument
Argument Description argument_1
Real expression, where ABS(argument_1) ≤ 1.
-
Return Value
-
A real floating-point value that is an approximation of the inverse (arc) sine of argument_1 in radians.
-
A result that is in the range of -π/2 ≤ ASIN(argument_1) ≤ π/2 and has the base and precision of argument_1.
-
4.7.3. ATAN
The ATAN function calculates the inverse (arc) tangent of an argument or a ratio of arguments in radians.

-
Argument
Argument Description argument_1
Expression.
argument_2
Expression.
-
Return Value
-
A floating-point value that is an approximation of the inverse (arc) tangent of argument_1 in radians or a ration argument_1/argument_2.
-
If argument_1 is the only argument, the result will have the base and precision of argument_1 and will be in the range of -π/2 ≤ ATAN(argument_1) ≤ π/2.
-
If both argument_1 and argument_2 are specified, each must be real. If both of them are 0, an error will occur. When both of them are not 0, the result will have the precision of the longer argument, which will be a base determined by the expression rules. It will also have the following values:
-
ATAN(argument_1/argument_2) if argument_2>0.
-
π/2 if argument_2=0 and argument_1>0.
-
-π/2 if argument_2=0 and argument_1<0.
-
π + ATAN(argument_1/argument_2) if argument_2<0 and argument_1≥0.
-
-π + ATAN(argument_1/argument_2) if argument_2<0 and argument_1<0.
-
-
4.7.4. ATAND
The ATAND function calculates the inverse (arc) tangent of an argument or a ratio of arguments in degrees.

-
Argument
Argument Description argument_1
Expression.
argument_2
Expression.
-
Return Value
-
A real floating-point value that is an approximation of the inverse (arc) tangent in degrees of argument_1 or a ration argument_1/argument_2.
-
If argument_1 is the only argument, it must be real. The result has the base and precision of argument_1 and is in the range of -90 ≤ ATAND(argument_1) ≤ 90.
-
If both argument_1 and argument_2 are specified, each must be real. The result is (180/π) * ATAN(argument_1,argument_2).
-
For argument requirements and attributes of the result, refer to ATAN.
-
4.7.5. COS
The COS function calculates the cosine of an argument in radians.

-
Argument
Argument Description argument_1
Expression with a value in radians.
-
Return Value
-
A floating-point value that is an approximation of the cosine of argument_1 in radians, and has the mode, base, and precision of argument_1.
-
4.7.6. COSD
The COSD function calculates the cosine of an argument in degrees.

-
Argument
Argument Description argument_1
Expression with a value in degrees.
-
Return Value
-
A real floating-point value that is an approximation of the cosine of argument_1 in degrees, and has the mode, base, and precision of argument_1.
-
4.7.7. EXP
The EXP function calculates the base, e, of a natural logarithm system raised to the power of an argument.

-
Argument
Argument Description argument_1
Expression.
-
Return Value
-
A floating-point value that is an approximation of the base, e, of a natural logarithm system raised to the power of argument_1.
-
The result will have the base, mode, and precision of argument_1.
-
4.7.8. LOG
The LOG function calculates the natural logarithm of an argument.

-
Argument
Argument Description argument_1
Expression.
-
argument_1 must be greater than 0.
-
-
Return Value
-
A floating-point value that is an approximation of a natural logarithm (the logarithm to the base e) of argument_1.
-
The result will have the base, mode, and precision of argument_1.
-
4.7.9. LOG10
The LOG10 function calculates the common logarithm of an argument.

-
Argument
Argument Description argument_1
Real expression.
-
argument_1 must be greater than 0.
-
-
Return Value
-
A floating-point value that is an approximation of a common logarithm (the logarithm to the base 10) of argument_1.
-
The result will have the base, mode, and precision of argument_1.
-
4.7.10. SIN
The SIN function calculates the sine of an argument in radians.

-
Argument
Argument Description argument_1
Expression with a value in radians.
-
Return Value
-
A floating-point value that is an approximation of the sine of argument_1 in radians, and has the mode, base, and precision of argument_1.
-
4.7.11. SIND
The SIND function calculates the sine of an argument in degrees.

-
Argument
Argument Description argument_1
Expression with a value in degrees.
-
Return Value
-
A floating-point value that is an approximation of the sine of argument_1 in degrees, and has the mode, base, and precision of argument_1.
-
4.7.12. SQRT
The SQRT function calculates the positive square root of an argument.

-
Argument
Argument Description argument_1
Expression.
-
If argumen-t1 is real, it must be greater than or equal to 0.
-
-
Return Value
-
A floating-point value that is an approximation of the positive square root of argument_1, and has the mode, base, and precision of argument_1.
-
4.7.13. TAN
The TAN function calculates the tangent of an argument in radians.

-
Argument
Argument Description argument_1
Expression with a value in radians.
-
Return Value
-
A floating-point value that is an approximation of the tangent of argument_1 in radians, and has the mode, base, and precision of argument_1.
-
4.7.14. TAND
The TAND function calculates the tangent of an argument in degrees.

-
Argument
Argument Description argument_1
Expression with a value in degrees.
-
Return Value
-
A real floating-point value that is an approximation of the tangent of argument_1 in degrees, and has the base, and precision of argument_1.
-
4.8. Miscellaneous Built-in Functions
Miscellaneous built-in functions do not belong to any of the previous built-in function categories.
The following describes each function. For more information, refer to the relevant section.
Function | Description |
---|---|
Returns the character value of an integer. |
|
Returns the PL/I return code value. |
|
Returns the name of a procedure. |
|
Returns the integer value of a character. |
|
Returns the string that is the concatenation of all elements of a string aggregate. |
|
Returns the bit string that is the internal representation of the value. |
4.8.1. CHARVAL
The CHARVAL function returns the character value of an integer.

-
Argument
Argument Description argument-1
Expression.
-
Return Value
-
An EBCDIC character of the integer that corresponds to element of argument-1.
-
A CHARACTER(1) value.
-
4.8.2. PLIRETV
The PLIRETV function returns the PL/I return code value.
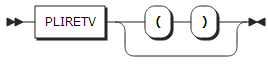
-
Return Value
-
A PL/I return code as a FIXED BINARY(31,0).
-
The PL/I return code is the latest return code set via CALL PLIRETC.
-
4.8.3. PROCEDURENAME (Abbreviation: PROCNAME)
The PROCEDURENAME function returns the character-string name of a procedure whose built-in function has been called.

-
Return Value
-
A procedure name as a NONVARYING character string.
-
For a procedure with more than one name, the left-most name is returned regardless of whether it is used for the CALL statement or the GOTO statement.
-
4.8.4. RANK
The RANK function returns the integer value of a character.

-
Argument
Argument Description argument-1
Expression.
-
argument_1 must be CHARACTER(1) NONVARYING.
-
-
Return Value
-
An integer that corresponds to an EBCDIC character of argument_1.
-
4.8.5. STRING
The STRING function returns the string that is the concatenation of all elements of a string aggregate.

-
Argument
Argument Description argument-1
Aggregate or element reference.
-
Return Value
-
A string to which all elements in argument_1 are concatenated.
-
The STRING function has the following restrictions:
-
Cannot be used for unions or structures that include unions.
-
To use a scalar, the scalar must be converted to a string.
-
To use a structure, the structure must not include padding bytes. All elements of the structure must be unaligned bit strings or strings.
-
To use an array, all elements in an array must be unaligned bit strings or strings.
-
-
4.8.6. UNSPEC
The UNSPEC function returns the bit string that is the internal representation of the value.

-
Argument
Argument Description argument_1
Scalar, array, structure, or union expression.
-
Return Value
-
A bit string that is the internal code of argument_1.
-
If the USAGE( UNSPEC(IBM) ) compiler option is used, a structure will not be allowed and an array will yield a BIT array.
-
The UNSPEC function can affect the portability of a program. |
4.9. Precision-handling Built-in Functions
Precision-handling built-in functions manipulate variables with specified precisions and returns the results.
The following describes each function. For more information, refer to the relevant section.
Function | Description |
---|---|
Adds two values using specified precision. |
|
Converts a value to binary. |
|
Converts a value to decimal. |
|
Divides an argument value by another argument value using specified precision. |
|
Converts a value to fixed-point. |
|
Converts a value to a floating-point. |
|
Multiplies two values using a specified precision. |
|
Converts a value to make it have a specified precision. |
4.9.1. ADD
The ADD function adds two values using specified precision. This function can be used for subtraction by using a minus sign for an operand to be subtracted as a prefix.

-
Argument
Argument Description argument_1
Expression.
argument_2
Expression.
argument_3
Restricted expression that specifies the number of digits of a result.
argument_4
Restricted expression that specifies the scaling factor of a result.
-
For a fixed-point result, if argument_4 is omitted, the scaling factor will be 0 by default.
-
For a floating-point result, argument_4 must be omitted.
-
-
Return Value
-
The sum of argument_1 and argument_2 with a precision specified by argument_3 and argument_4.
-
The base, scale, and mode of a result that are determined by expression evaluation rules if a PRECTYPE compiler option does not have an influence on them.
-
4.9.2. BINARY
The BINARY function converts a value to binary.

-
Argument
Argument Description argument_1
Expression.
argument_2
Restricted expression that specifies the number of digits of a result. It must not exceed the implementation limit.
argument_3
Restricted expression that specifies the scaling factor of a result.
-
For a fixed-point result, if argument_2 is given and argument_3 is omitted, the scaling factor will be 0 by default.
-
For a floating-point result, argument_3 must be omitted.
-
-
Return Value
-
The binary value of argument_1 with a precision specified by arbument_2 and argument_3.
-
If argument_2 and argument_3 are omitted, the precision of a result is determined by base conversion rules.
-
4.9.3. DECIMAL
The DECIMAL function converts a value to a decimal.

-
Argument
Argument Description argument_1
Restricted expression.
argument_2
Restricted expression that specifies the number of digits of a result.
argument_3
Restricted expression that specifies the scaling factor of a result.
-
For a fixed-point result, if argument_2 is given and argument_3 is omitted, the scaling factor will be 0 by default.
-
For a floating-point result, argument_3 must be omitted.
-
-
Return Value
-
The decimal value of argument_1 with a precision specified by argument_2 and argument_3. The result will have the mode and scale of argument_1.
-
If argument_2 and argument_3 are omitted, the precision of a result will be determined by base conversion rules.
-
4.9.4. DIVIDE
The DIVIDE function divides an argument value by another argument value using specified precision.

-
Argument
Argument Description argument_1
Expression.
argument_2
Expression.
-
If argument_2 is 0, the ZERODIVIDE condition will occur.
argument_3
Restricted expression that specifies the number of digits of a result.
argument_4
Restricted expression that specifies the scaling factor of a result.
-
For a fixed-point result, if argument_4 is omitted, a scaling factor is 0 by default.
-
For a floating-point result, argument_4 must be omitted.
-
-
Return Value
-
The quotient of argument_1/argument_2 with a precision specified by argument_3 and argument_4.
-
The base, scale, and mode of a result that are determined by expression evaluation rules if a PRECTYPE compiler option does not have an influence on them.
-
4.9.5. FIXED
The FIXED function converts a value to a fixed-point.

-
Argument
Argument Description argument_1
Expression.
argument_2
Restricted expression that specifies the number of digits of a result. It must not exceed the implementation limit.
argument_3
Restricted expression that specifies the scaling factor of a result.
-
If argument_3 is omitted, a scaling factor is 0 by default.
-
-
Return Value
-
The fixed-point value of argument_1 with a precision specified by argument_2 and argument_3. The result will have the base and mode of argument_1.
-
If argument_2 and argument_3 are omitted, (15,0) and (5,0) will be used for binary and decimal results, respectively.
-
4.9.6. FLOAT
The FLOAT function converts a value to a floating-point.

-
Argument
Argument Description argument_1
Expression.
argument_2
Restricted expression that specifies the minimum number of digits of a result.
-
Return Value
-
The approximate floating-point value of argument_1 with a precision specified by argument_2. The result will have the base and mode of argument_1.
-
If argument_2 is omitted, the precision of a result is determined by base conversion rules, and 15 and 5 will be used for binary and decimal results, respectively.
-
4.9.7. MULTIPLY
The MULTIPLY function multiplies two values using specified precision.

-
Argument
Argument Description argument_1
Expression.
argument_2
Expression.
argument_3
Restricted expression that specifies the number of digits of a result.
argument_4
Restricted expression that specifies the scaling factor of a result.
-
For a fixed-point result, if argument_4 is omitted, the scaling factor will be 0 by default.
-
For a floating-point result, argument_4 must be omitted.
-
-
Return Value
-
The product of argument_1 and argument_2 with a precision specified by argument_3 and argument_4.
-
The base, scale, and mode of a result that are determined by expression evaluation rules if a PRECTYPE compiler option does not have an influence on them.
-
4.9.8. PRECISION
The PRECISION function converts a value to make it have a specified precision.

-
Argument
Argument Description argument_1
Expression.
argument_2
Restricted expression that specifies the number of digits of a result.
argument_3
Restricted expression that specifies the scaling factor of a result.
-
For a fixed-point result, if argument_3 is omitted, the scaling factor will be 0 by default.
-
For a floating-point result, argument_3 must be omitted.
-
-
Return Value
-
The value of argument_1 with a precision specified by argument_2 and argument_3.
-
A result that has the base, mode, and scale of argument_1.
-
4.10. Storage Control Built-in Functions
Storage control built-in functions enables the retrieval of storage requirements and variable locations, the assignment of specific values to area and locator variables, and the execution of conversion between offset and pointer They also allow a user to obtain the number of generations of a controlled variable, and to refer to the data and methods of objects and classes.
The following describes each function. For more information, refer to the relevant section.
Function | Description |
---|---|
Returns the address of a variable. |
|
Returns the generation value of a controlled variable. |
|
Converts a pointer or offset value to an integer. |
|
Returns the current size of a variable. |
|
Same as the CURRENTSIZE function. |
|
Returns the null pointer value. |
|
Converts a pointer to OFFSET. |
|
Converts OFFSET to a pointer. |
|
Adds an integer to the pointer value. |
|
Returns the maximum size of a variable. |
|
Same as the SIZE function. |
4.10.1. ADDR
The ADDR function returns the address of a variable.

-
Argument
Argument Description argument_1
Reference expression.
It refers to a variable of any data type, data organization, alignment, or storage class except for the following:
-
A subscripted reference expression to an unaligned fixed-length bit string variable
-
A reference expression of a DEFINED or BASED variable or simple parameter that is an unaligned fixed-length bit string
-
A minor structure or union that has a first base element that is an unaligned fixed-length bit string
-
A major structure or union that has the DEFINED attribute or is a parameter, and that has an unaligned fixed-length bit string as its first element
-
A reference expression that is not connected storage
If argument_1 is a reference expression to:
-
An aggregate variable, it must have the CONNECTED attribute.
-
An aggregate, a return value will identify the first element.
-
A component or cross section of an aggregate, a return will be subscripting, and structure or union qualification.
-
A changeable string, a return value will identify a 2-byte prefix.
-
An area, a return value will identify control information.
-
A controlled variable that is not allocated in a current program, a return value will be a NULL pointer value.
-
A based variable, a result will be the value of the pointer explicitly qualifying argument_1, or related to argument_1 in its declaration, or a NULL pointer.
-
A parameter, and a temporary argument is created, a return value will identify a temporary argument.
-
-
Return Value
-
A pointer value that identifies the generation of argument_1.
-
4.10.2. ALLOCATION (Abbreviation: ALLOCN)
The ALLOCATION function returns the generation value of a controlled variable.

-
Argument
Argument Description argument-1
Level-1 controlled variable.
-
Return Value
-
The generation value of argument-1.
-
4.10.3. BINARYVALUE (Abbreviation: BINVALUE)
The BINARYVALUE function converts a pointer or offset value to an integer.

-
Argument
Argument Description argument_1
Expression. It can be a pointer or offset.
-
Return Value
-
A FIXED BINARY(31,0) value that is converted from argument_1.
-
4.10.4. CURRENTSIZE
The CURRENTSIZE function returns the current size of a variable. This function cannot be used for a based variable with adjustable extents if the variable is not allocated in advance.

-
Argument
Argument Description argument_1
Variable of any data type, data organization, and storage class except for the following:
-
A BASED, DEFINED, parameter, subscripted, or structure or union based element variable that is an unaligned fixed-length bit string.
-
A minor structure or union whose first or last base element is an unaligned fixed-length bit string.
-
A major structure or union that has the BASED, DEFINED, or parameter variable attributes and an unaligned fixed-length bit string as its first or last element.
-
A variable in unconnected storage.
-
-
Return Value
-
A FIXED BINARY value of the implementation-defined storage value (in bytes) that is required by argument_1.
-
4.10.5. CURRENTSTORAGE (Abbreviation: CSTG)
The CURRENTSTORAGE function is the same as the CURRENTSIZE function. For information, refer to CURRENTSIZE.
4.10.6. NULL
The NULL function returns the null pointer value.
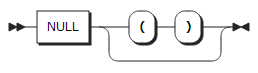
-
Return Value
-
The null pointer value. It does not identify a variable generation. It can be assigned to and compared with handles.
-
The null pointer value can be converted to OFFSET by assigning a built-in function value to an offset variable.
-
4.10.7. OFFSET
The OFFSET function converts a pointer to OFFSET.

-
Argument
Argument Description argument_1
Pointer reference expression.
argument_2
AREA reference expression.
-
Return Value
-
An OFFSET value that indicates the relative location of argument_1 from argument_2.
-
The null OFFSET if argument_1 is the null pointer.
-
4.10.8. POINTER (Abbreviation: PTR)
The POINTER function converts OFFSET to a pointer.

-
Argument
Argument Description argument_1
OFFSET reference expression.
argument_2
AREA reference expression.
-
Return Value
-
A pointer value that indicates the relative location of argument_1 from argument_2.
-
The null pointer if argument_1 is null OFFSET.
-
4.10.9. POINTERADD (Abbreviation: PTRADD)
The POINTERADD function adds an integer to the pointer value.

-
Argument
Argument Description argument-1
Pointer expression
argument-2
Expression
-
Must be a computational type and is converted to a FIXED BINARY(31,0) type.
-
-
Return Value
-
A pointer value that is the sum of the pointer value of argument-1 and the value of argument-2.
-
4.10.10. SIZE
The SIZE function returns the maximum size of a variable. This function cannot be used for a based variable with adjustable extents if the variable is not allocated in advance.

-
Argument
Argument Description argument_1
Variable of any data type, data organization, alignment, and storage class except for the following:
-
A BASED, DEFINED, parameter, subscripted, or structure or union based element variable that is an unaligned fixed-length bit string.
-
A minor structure or union whose first or last base element is an unaligned fixed-length bit string.
-
A major structure or union that has the BASED, DEFINED, or parameter variable attributes and an unaligned fixed-length bit string as its first or last element.
-
A variable in unconnected storage.
-
-
Return Value
-
A FIXED BINARY value of the implementation-defined storage value (in bytes) that is allocated to argument_1.
-
4.10.11. STORAGE (Abbreviation: STG)
The STORAGE function is the same as the SIZE function. For information about the STORAGE function, refer to SIZE.
4.11. String-handling Built-in Functions
String-handling built-in functions simplify the handling of bit, character, graphic, and widechar strings. String arguments can be expressed by arithmetic expressions that can be converted to strings according to data conversion rules or given rules.
The following describes each function. For more information, refer to the relevant section.
Function | Description |
---|---|
Converts a value to a character string. |
|
Returns a string of a value converted to a picture specification. |
|
Returns a string consisting of n number of the 0xFF character. |
|
Searches a string from another string. |
|
Returns a string of a specified length consisting of a value that is left-justified and padded with a specified character. |
|
Returns the current length of a string. |
|
Returns a string consisting of n number of the 0x00 character. |
|
Returns a string with all alphabet characters converted to lowercase. |
|
Returns a string consisting of n+1 copies of a string. |
|
Returns a string of a specified length consisting of a value that is right-justified and padded with a specified character. |
|
Searches and returns the location of the first occurrence of any one of the elements of a string within another string starting from the left. |
|
Searches and returns the location of the first occurrence of any one of the elements of a string within another string starting from the right. |
|
Returns a substring of a string. |
|
Converts a character string based on other character strings. |
|
Returns a string trimmed from the left and/or right. |
|
Returns a string with all alphabet characters converted to uppercase. |
|
Searches and returns the location of the first nonoccurrence of any one of the elements of a string within another string starting from the left. |
|
Searches and returns the location of the first nonoccurrence of any one of the elements of a string within another string starting from the right. |
4.11.1. CHARACTER (Abbreviation: CHAR)
The CHARACTER function converts a value to a character string.

-
Argument
Argument Description argument_1
Expression.
-
argument_1 must be computational.
-
If argument_1 is not graphic, the CHARACTER function will convert it to characters and return the result. If argument_1 is graphic, the function will convert it to SBCS characters and return the result.
-
If a DBCS character cannot be translated into a corresponding SBCS character, the CONVERSION condition will occur.
-
The argument_1 value is not checked.
argument_2
Expression.
-
If necessary, argument_2 will be converted to a real fixed-point binary value.
-
If argument_2 is omitted, the length will be determined by the type conversion rules.
-
argument_2 cannot be negative. If argument_2 is 0, the result will be a null character string.
-
-
Return Value
-
The character value of argument_1 with a length specified by argument_2.
-
A result that has the mode, base, scale, and precision of argument_1.
-
The precision of a result is as follows except when the precision of argument_1 is a fixed-point with a precision (p,q).
(min(N, max(p-q+1)), 0)
N is the maximum number of allowed digits.
-
4.11.2. EDIT
The EDIT function returns a string of a value converted to a picture specification.

-
Argument
Argument Description argument-1
Expression.
-
Must be a computational type.
argument-2
String expression.
-
Must be a string and include a valid picture specification.
-
An invalid picture specification will trigger an error.
-
-
Return Value
-
A string of the value of argument-1 that is converted to the picture-specification of argument-2 and with the size of argument-2.
-
4.11.3. HIGH
The HIGH function returns a string consisting of n number of the 0xFF character.

-
Argument
Argument Description argument_1
Expression.
-
If necessary, argument_1 will be converted to a positive real fixed-point binary value.
-
If argument_1 is 0, the result will be the null character string.
-
-
Return Value
-
A character string with the length of argument_1, where each character is the highest character in a collating sequence (hexadecimal FF).
-
4.11.4. INDEX
The INDEX function searches a string from another string.

-
Argument
Argument Description argument_1
String expression from which a search is performed.
argument_2
Target string expression to search.
argument_3
-
Specifies the location in argument_1 from which a search starts.
-
Must be computational. If necessary, it will be converted to a FIXED BINARY(31,0).
-
-
Return Value
-
A REAL FIXED BINARY value that indicates the start position of a substring that is the same as argument_2 within argument_1.
-
0 if argument_2 is not in argument_1, or the length of either argument_1 or argument_2 is 0.
-
0 if argument_3 is less than 1 or greater than 1 + length(argument_1). The STRINGRANGE condition will occur.
-
4.11.5. LEFT
The LEFT function returns a string of a specified length consisting of a value that is left-justified and padded with a specified character.

-
Argument
Argument Description argument-1
Expression.
-
Must be a computational type and is converted to a CHARACTER.
argument-2
Expression.
-
Must be a computational type and is converted to a FIXED BINARY(31,0).
argument-3
Expression.
-
A character used as a padding to fill empty spaces in a string of length specified by argument-2 after inserting argument-1 into it to be left justified.
-
Must be a CHARACTER(1) NONVARYING.
-
If omitted, an blank character is used.
-
-
Return Value
-
A string of the length argument-2 consisting of argument-1 value that is left-justified and padded with character argument-3.
-
4.11.6. LENGTH
The LENGTH function returns the current length of a string.

-
Argument
Argument Description argument_1
String expression.
-
If argument_1 is binary, it will be converted to a bit string. Otherwise, it will be converted to a character string.
-
-
Return Value
-
A REAL FIXED BINARY that specifies the current length of argument_1.
-
4.11.7. LOW
The LOW function returns a string consisting of n number of the 0x00 character.

-
Argument
Argument Description argument_1
Expression.
-
If necessary, argument_1 will be converted to a positive real fixed-point binary value.
-
If argument_1 is 0, the result will be a null character string.
-
-
Return Value
-
A character string with the length of argument_1, where each character is the lowest character in a collating sequence (hexadecimal 00).
-
4.11.8. LOWERCASE
The LOWERCASE function returns a string with all alphabet characters converted to lowercase.

-
Argument
Argument Description argument-1
Expression.
-
If necessary, argument_1 will be converted to a string.
-
-
Return Value
-
A string with all characters of argument-1 converted to lowercase.
-
4.11.9. REPEAT
The REPEAT function returns a string consisting of n+1 copies of a string.

-
Argument
Argument Description argument_1
Bit, character, graphic, or widechar expression to repeat.
-
If argument_1 is arithmetic, its conversion will occur. If it is binary or decimal, it will be converted to a bit string or a character string respectively.
argument_2
Expression.
-
If necessary, argument_2 will be converted to a real fixed-point binary value.
-
-
Return Value
-
A bit, character, graphic, or widechar string that consists of as many argument_1 as the number specified by argument_2 + 1.
-
4.11.10. RIGHT
The RIGHT function returns a string of a specified length consisting of a value that is right-justified and padded with a specified character.

-
Argument
Argument Description argument-1
Expression.
-
Must be a computational type and is converted to a CHARACTER.
argument-2
Expression.
-
Must be a computational type and is converted to a FIXED BINARY(31,0).
argument-3
Expression.
-
A character used as a padding to fill empty spaces in a string of length specified by argument-2 after inserting argument-1 into it to be right justified.
-
Must be a CHARACTER(1) NONVARYING.
-
If omitted, an blank character is used.
-
-
Return Value
-
A string of the length argument-2 consisting of argument-1 value that is right-justified and padded with character argument-3.
-
4.11.11. SEARCH
The SEARCH function searches and returns the location of the first occurrence of any one of the elements of a string within another string starting from the left.

-
Argument
Argument Description argument-1
String expression.
argument-2
String expression.
-
A string whose CHARACTER, BIT, GRAPHIC, or WIDECHAR to search for in argument-1.
argument-3
Expression.
-
Position to start the search.
-
Must be a computational type and is converted to a FIXED BINARY(31,0).
-
If omitted, 0 is used.
-
-
Return Value
-
A REAL FIXED BINARY that represents the location of the first occurrence of any of the elements of argument-2 within argument-1 searching right starting from the position specified by argument-3.
-
0 (zero) if argument-1 or argument-2 is a null string.
-
0 (zer0) of no match is found.
-
4.11.12. SEARCHR
The SEARCHR function searches and returns the location of the first occurrence of any one of the elements of a string within another string starting from the right.

-
Argument
Argument Description argument-1
String expression.
argument-2
String expression.
-
A string whose CHARACTER, BIT, GRAPHIC, or WIDECHAR to search for in argument-1.
argument-3
Expression.
-
Position to start the search.
-
Must be a computational type and is converted to a FIXED BINARY(31,0).
-
If omitted, LENGTH(argument-1) is used.
-
-
Return Value
-
A REAL FIXED BINARY that represents the location of the first occurrence of any of the elements of argument-2 within argument-1 searching left starting from the position specified by argument-3.
-
0 (zero) if argument-1 or argument-2 is a null string.
-
0 (zer0) of no match is found.
-
4.11.13. SUBSTR
The SUBSTR function returns a substring in a string.

-
Argument
Argument Description argument_1
String expression.
-
Specifies a string from which a substring is extracted.
-
If argument_1 is not a string, it will be converted to a character.
argument_2
Expression that is converted to a FIXED BINARY(31,0).
-
Specifies the start position of a substring in argument_1.
argument_3
Expression that is converted to a FIXED BINARY(31,0).
-
Specifies the length of a substring in argument_1.
-
If it is 0, a null string will be returned. If it is omitted, a substring that starts from argument_2 and ends at the end of argument_1 will be returned.
-
-
Return Value
-
A substring specified by argument_2 and argument_3 within argument_1.
-
If argument_3 is negative or a substring specified by argument_2 and argument_3 is not within argument_1, the STRINGRANGE condition will occur. However, the condition will not occur if argument_2 is LEGNTH(argument_1)+1 and argument_3 is 0.
-
4.11.14. TRANSLATE
The TRANSLATE function converts a character string based on other character strings. This function does not support GRAPHIC or WIDECHAR data. Any arithmetic or bit arguments are converted to characters.

-
Argument
Argument Description argument_1
Character expression to be searched for translations of characters.
argument_2
Character expression that contains translated character values.
argument_3
Character expression that contains characters to translate.
-
If it is omitted, COLLATE() will be used by default.
-
-
Return Value
-
A character string with the same length as that of argument_1.
-
4.11.15. TRIM
The TRIM function returns a string trimmed from the left and/or right. All arguments must be computational. If they are not character strings, they will be converted to character strings.

-
Argument
Argument Description argument_1
String expression.
argument_2
String expression to trim from the left.
-
If it is omitted, the default will be a CHAR(1) NONVARYING with a blank.
argument_3
String expression to trim from the right.
-
If it is omitted, the default will be a CHAR(1) NONVARYING with a blank.
-
-
Return Value
-
A character string where argument_2 is trimmed from the left repeatedly until argument_2 does not exist and argument_3 is trimmed from the right repeatedly until argument_3 does not exist.
-
4.11.16. UPPERCASE
The UPPERCASE function returns a string with all alphabet characters converted to uppercase.

-
Argument
Argument Description argument-1
Expression.
-
If necessary, argument_1 will be converted to a string.
-
-
Return Value
-
A string with all characters of argument-1 converted to uppercase.
-
4.11.17. VERIFY
The VERIFY function searches and returns the location of the first nonoccurrence of any one of the elements of a string within another string starting from the left.

-
Argument
Argument Description argument_1
String expression.
argument_2
String expression.
argument_3
Expression that indicates the location in argument_1 from which search will start. (Default value: 1)
-
Must be computational. If necessary, it will be converted to FIXED BINARY(31,0).
-
-
Return Value
-
A REAL FIXED BINARY value that indicates the position in argument_1 of the leftmost character, widechar, graphic, or bit that does not exist in argument_2.
-
0 if all characters, widechars, graphics, or bits in argument_1 exist in argument_2. If argument_1 is a null string, 0 will be returned. If argument_1 is not a null string and argument_2 is a null string, the value of argument_3 will be returned.
-
Unless 1 ≤ argument_3 ≤ LENGTH(argument_1) + 1, the STRINGRANGE condition will occur if the condition is enabled. Its implicit action and normal return will have the result of 0.
-
If argument_3 is LENGTH(argument_1) + 1, then the result will be 0.
-
4.11.18. VERIFYR
The VERIFYR function searches and returns the location of the first nonoccurrence of any one of the elements of a string within another string starting from the right.

-
Argument
Argument Description argument-1
String expression.
argument-2
String expression.
argument-3
Expression that indicates the location in argument_1 from which search will start.
-
Must be computational. If necessary, it will be converted to FIXED BINARY(31,0).
-
LENGTH(argument-1) is the default value.
-
-
Return Value
-
A REAL FIXED BINARY value that indicates the position in argument_1 of the rightmost character, widechar, graphic, or bit that does not exist in argument_2 starting from position specified by argument-3.
-
0 if all characters, widechars, graphics, or bits in argument_1 exist in argument_2. If argument_1 is a null string, 0 will be returned. If argument_1 is not a null string and argument_2 is a null string, the value of argument_3 will be returned.
-
Unless 1 ≤ argument_3 ≤ LENGTH(argument_1) + 1, the STRINGRANGE condition will occur if the condition is enabled. Its implicit action and normal return will have the result of 0.
-
If argument_3 is LENGTH(argument_1) + 1, then the result will be 0.
-