Data Management
This chapter describes OpenFrame PL/I data types and attributes. It also describes how to declare and use them.
1. Data Elements
This section describes data types and attributes used in a PL/I program.
1.1. Data Items
A data item is a value of a variable or constant. Data items can be single items called scalars, or a group of items called a data aggregate. A data aggregate has two types: arrays and structures.
-
Variables
A variable has a value that can be changed during program execution. A variable can be used after declaring its name and attributes.
A variable is referred to by one of the following:
-
A declared variable name
-
A reference derived through one or more of the following:
-
Pointer qualification
-
Structure qualification
-
Subscripting
The following refers to a variable:
DCL X(3) CHAR(10); DCL 1 A, 3 B CHAR(10); DCL Y(3) CHAR(10) BASED(P); DCL P PTR; X(1) = 'TEST'; /* subscripting - X(1) */ A.B = 'MEMBER'; /* Reference using a structure qualification - A.B*/ P = ADDR( X ); /* Reference using a variable name - P, X */ DISPLAY( P->Y(1) ) /* Reference using a pointer qualification and subscripting. - P->Y(1) */
-
-
-
Constants
A constant value is not changed during program execution.
-
Punctuating Constants
Underscore (_) characters can be used in arithmetic, bit, and hexadecimal constants for readability.
43_345_842 ==> 45345842 1100_0011B ==> 11000011B '1001_0011'B ==> '10010011'B 'C_A'B4 ==> 'CA'B4
1.2. Data Types and Attributes
Data used in a PL/I program is either computational data or program-control data.
-
Computational data
-
Used in computations to create a result. Arithmetic and string data are computational data.
-
Arithmetic data is either coded arithmetic data or numeric picture data.
-
Coded arithmetic data has a number value with base (BINARY or DECIMAL), scale (FLOAT or FIXED), and precision attributes.
-
Numeric picture data is saved in a string format. For more information, refer to Picture Specification.
-
-
Program-control data
-
Used to control program execution.
-
Has the following data types: area, entry, label, file, format, pointer, and offset.
-
In the following example, VAR1 and VAR2 are coded arithmetic variables and the numbers, 2 and 3.14, are coded arithmetic constants:
VAR1 = (VAR2**2) * 3.14
Program-control data constants are determined by a compiler.
In the following example, LAB1 is a label constant of program-control data. The LAB1 value is the address of "statement X = Y + 3;":
LAB1: X = Y + 3; A = B || C;
To use a data item, PL/I must know the data type and how to process it. Attributes provide the information.
There are two kinds of attributes: data attributes and nondata attributes.
-
Data attributes
Describe computational data and program-control data characteristics.
AREA BINARY BIT CHARACTER DECIMAL DIMENSION ENTRY FILE FIXED FLOAT FORMAT GRAPHIC LABEL NONVARYING PICTURE POINTER PRECISION RETURNS SIGNED STRUCTURE UNSIGNED VARYING
-
CHARACTER specifies the string type of computational data.
-
FILE specifies the file type of program-control data.
-
-
Nondata attributes
Describe nondata elements such as built-in functions or other attributes.
ALIGNED AUTOMATIC BASED BUILTIN BYADDR BYVALUE CONDITION CONTROLLED DEFIEND DIRECT EXTERNAL GENERIC INITIAL INTERNAL LIKE LIST PARAMETER POSITION RECORD SEQUENTIAL STATIC STEAM UNALIGNED VARIABLE
-
INTERNAL scope attribute specifies that a data item can only be used in a block in which an item is declared.
-
1.3. Computational Data Types and Attributes
The following section describes computational data types and their attributes.
1.3.1. Coded Arithmetic data and attributes
The syntax of coded arithmetic data is as follows:
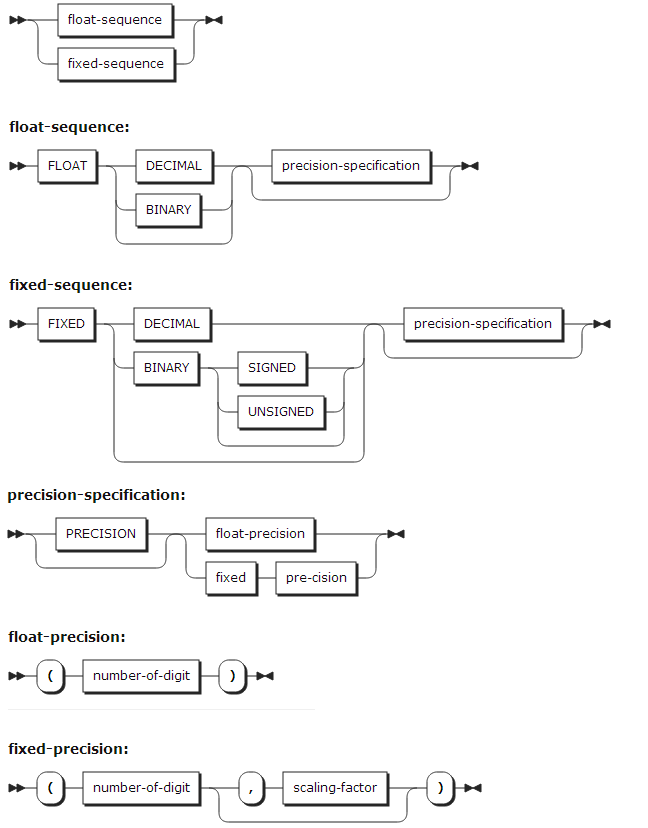
Attribute | Abbreviation |
---|---|
BINARY |
BIN |
DECIMAL |
DEC |
PRECISION |
PREC |
-
BINARY and DECIMAL attributes
-
The base of coded arithmetic data is either decimal or binary.
-
DECIMAL is the default.
-
-
FIXED and FLOAT attributes
-
The scale of coded arithmetic data is either fixed-point or floating-point.
-
-
PRECISION attribute
-
The number of digits and the scaling factor of coded arithmetic data (the scaling factor can be used only for fixed-point data items).
-
The number of digits is an integer that specifies how many digits the data item value can have.
-
The number of digits for fixed-point data items means the total number of digits in a binary or decimal value.
-
The number of digits for floating-point data items means the number of digits in a mantissa part.
-
The scaling factor is the available number of decimal places.
-
-
SIGNED and UNSIGNED attributes
-
Used only for FIXED BINARY variables.
-
If the SIGNED attribute is specified, a variable value can be negative. If the UNSIGNED attribute is specified, a variable value must be positive.
The attributes have the following affects on storage size:
Classification Precision Storage Size (Bytes) FIXED BINARY SIGNED data storage size
p ⇐ 7
1
7 < p ⇐ 15
2
15 < p ⇐ 31
4
31 < p ⇐ 63
8
FIXED BINARY UNSIGNED data storage size
p ⇐ 8
1
8 < p ⇐ 16
2
16 < p ⇐ 32
4
32 < p ⇐ 64
8
-
-
Binary fixed-point data
-
In the following example, the VAR1 variable is a binary fixed-point data item with 20 bits. The rightmost 2 bits are used to express a binary point.
DECLARE VAR1 FIXED BINARY (20,2);
The declared number of data bits (20 bits in the above example) is located in low-order positions. Therefore, extra high-order position bits also exist and they can be used during operation. If overflow occurs in extra high-order bit positions, the SIZE condition is used if the condition is enabled.
-
-
Binary fixed-point constant
-
Binary constant that consists of 0 and 1. It is followed by the character B.
-
Has a precision(p,q). The following are examples:
Constant Precision 1011_0B
(5,0)
1011_111B
(7,0)
1001.010B
(7,3)
-
-
XN (hex) binary fixed-point constant
-
Constant that has a hexadecimal number value with the SIGNED, REAL, FIXED, and BINARY data attributes. It is followed by XN.
-
Each hexadecimal digit is expressed with 4 bits.
-
If the number of digits of a constant is less than or equal to 8, the precision is 31. Otherwise, the precision is 63.
-
The following shows its syntax:
XN Binary Fixed-point Constant -
'100’XN is the same as 256.
-
-
XU (hex) binary fixed-point constant
-
Constant that has a hexadecimal number value with the UNSIGNED, REAL, FIXED, and BINARY data attributes. It is followed by XU.
-
Each hexadecimal digit is expressed with four bits.
-
If the number of digits of a constant is equal to or less than 8, the precision is 31. Otherwise, the precision is 63.
-
The following shows its syntax:
XU Binary Fixed-point Constant -
'8000’XU is the same as 32,768.
-
-
Decimal fixed-point data
-
Saved in the packed decimal format. Two digits are saved as 1 byte, and a sign value is saved in the rightmost 4 bits of the byte.
-
In the following example, the VAR1 variable is a decimal fixed-point data item with 7 digits. The right 3 digits are after the decimal point.
DECLARE VAR1 FIXED DECIMAL (7,3);
-
-
Decimal fixed-point constant
-
Constant that consists of decimal digits with an optional decimal point.
-
Has a precision(p,q). The following are examples:
Constant Precision 43.436
(5,3)
12.453154
(8,6)
0043
(4,0)
-
-
Binary floating-point data
-
If a precision is less than or equal to 21, a data item is a floating-point with a storage of 4 bytes. If it is greater than 21 and less than or equal to 53, the data item is double with a storage of 8 bytes. A precision greater than 53 is not supported.
-
In the following example, the VAR1 variable is a binary floating-point data item with a precision of 16 binary digits.
DECLARE VAR1 BINARY FLOAT (16);
-
-
Binary floating-point constant
-
Binary constant that consists of a mantissa and an exponent. It is followed by the character B. The exponent can be E, S, and D. S and D use as the maximum precision value of floating-point and double type data items respectively.
-
The following are examples:
Constant Precision 1101011E+4B
(7)
1101.011E-6B
(7)
101.0E-12B
(4)
1S0B
(21)
1D0B
(53)
-
-
Decimal floating-point data
-
If a precision is less than or equal to 6, a data item is a floating-point with a storage of 4 bytes. If it is greater than 6 and less than or equal to 16, the data item is double with a storage of 8 bytes. A precision greater than 16 is not supported.
-
In the following example, the VAR1 variable is a decimal floating-point data item with a precision of 5 decimal digits.
DECLARE VAR1 DECIMAL FLOAT (5);
-
-
Decimal floating-point constant
-
Decimal constant that consists of a mantissa and an exponent. The exponent can be E, S, and D. S and D use the maximum precision value of floating-point and double type data items respectively.
Constant Precision 435E-1
(3)
435E+1
(3)
3.235E-4
(4)
1S0
(6)
1D0
(16)
-
1.3.2. String Data and Attributes
The following describes string data types and their attributes:
-
BIT, CHARACTER, and GRAPHIC, WIDECHAR attributes
-
The BIT attribute specifies a bit string.
-
The CHARACTER attribute specifies a character string. A character string can also be declared using the PICTURE attribute.
-
The GRAPHIC attribute specifies a double-byte character s (DBCS) string.
-
WIDECHAR attribute specifies a UTF-16 character string.
-
The following is the syntax of the attributes:
String DataAttribute Abbreviation CHARACTER
CHAR
GRAPHIC
G
WIDECHAR
WCHAR
NONVARYING
NONVAR
VARYING
VAR
-
A string length is specified in 'length'. If VARYING is specified, a string will have a variable length that does not exceed the value specified in 'length'.
-
An asterisk (*) can be specified in the 'length' of a parameter. The parameter’s actual length is determined by the argument.
-
For information about the REFER attribute, refer to "REFER Attribute".
-
-
VARYING and NONVARYING attributes
-
The NONVARYING attribute specifies that a string has a fixed length.
-
The VARYING attribute specifies that a string has a variable length. Storage for a string with the attribute is 2 bytes longer than a declared length. The leftmost 2 bytes has the string’s length information.
-
-
PICTURE attribute (abbreviation: PIC)
-
The PICTURE attribute specifies a picture character for each position of a data item.
-
For information about picture-specification see "Picture Specification".
PICTURE Attribute -
Numeric character data has an arithmetic value even though it is stored in a string format. It is converted to coded arithmetic to be used for arithmetic operations.
-
-
Character data
-
Data item that saves a 1 byte character.
-
Uses ASCII codes as a character set.
-
-
Character constant
-
String enclosed with single quotation marks (' ') or double quotation marks (" ").
-
The following is the syntax:
Character Constant -
For example, 'Conan Doyle''s "Sherlock Holmes"' is a character constant with 31 characters.
Single quotes enclose Conan Doyle’s "Sherlock Holmes" as delimiters. Two single quotes immediately preceded by Doyle mean one single quote, not delimiters. Therefore, Doyle''s becomes Doyle’s in actual string contents. Since single quotes are used as delimiters, double quotes are treated as string contents.
-
A string repetition factor can be used. For example, (2)'TEST' is the same as 'TESTTEST'.
-
-
X (hex) character constant
-
String that consists of an even number of hexadecimal digits.
-
Enclosed with single quotes (' ') or double quotes (" "). It is followed by the character X.
X Character Constant -
The length of 'od0A’X is 2.
-
-
Bit data
-
Data with the BIT attribute enables bit string processing.
-
-
Bit constant
-
String that consists of binary digits (0 and 1).
-
Enclosed with single quotes (' ') or double quotes (" "). It is followed by the character B.
-
The following is the syntax:
Bit Constant -
'1101’B is a bit constant, which is different from 1101B which is coded arithmetic data.
-
-
B4 (hex) bit constant
-
String that consists of hexadecimal digits.
-
Enclosed with single quotes (' ') or double quotes (" "). It is followed by the character B4. BX is a synonym for B4.
-
Each hexadecimal digit is expressed with 4 bits.
-
The following is the syntax:
B4 Bit Constant -
'CA’B4 is the same as '11001010’B.
-
-
B3 (octal) bit constant
-
String that consists of octal digits.
-
Enclosed with single quotes (' ') or double quotes (" "). It is followed by the character B3.
-
Each octal digit is expressed with 3 bits.
-
The following is the syntax:
B3 Bit Constant -
'22’B3 is the same as '010010’B.
-
-
Graphic data
-
Can include DBCS characters.
-
Each DBCS character takes 2 bytes of storage.
-
-
Graphic constant
-
DBCS string enclosed with single quotes (' ') or double quotes (" "). It is followed by the character G.
-
Single and double quotes and the character G can be used as DBCS characters.
-
-
GX (hex) graphic constant
-
String that consists of hexadecimal digits.
-
Enclosed with single quotes (' ') or double quotes (" "). It is followed by the character GX.
-
Each 4 hexadecimal digit expresses a single DBCS character.
-
The following is the syntax:
GX Graphic Constant
-
-
Widechar data
-
Consists of a UTF-16 character string.
-
A single UTF-16 character is 2 bytes in length.
-
-
WX(hexa) widechar constant
-
Consists of hexadecimal digits enclosed in single quotes (' ') or double quotes (" ").
-
WX comes at the end. Each group of 4 hex digits represents one UTF-16 character.
-
The following is the syntax:
WX Graphic Constant
-
1.3.3. Picture Specification
A picture specification consists of picture characters enclosed with single quotation marks (' ') or double quotation marks (" "). Each picture character has information about a corresponding position of a data item.
A picture specification specifies whether a data item is a character string or numeric. If a picture character A or X exists, a picture specification is a character picture specification. Otherwise, it is numeric character picture specification.
-
Picture repetition factor
-
Number of repetitions. It is enclosed with parentheses and followed by a character.
-
For example, PIC '(4)9V(2)9' is the same as PIC '9999V99'.
-
-
Picture characters for character data
-
The picture characters X, A, and 9 represent character data.
Picture Character Description X
1 byte character.
A
Alphabetic character, #, @, $, and blank.
9
Digit or blank.
-
The following is an example:
DCL PIC_DATA PICTURE 'AAA99X';
If the above example picture specification is declared, the following are valid. 'b' represents a blank.
'ABC12M' 'bbb09/' 'XYZb13'
The following are invalid:
'AB123M' ==> The 3rd character '1' is invalid for the picture character A. 'ABC1/2' ==> The 5th character '/' is invalid for the picture character 9. 'Mb#A5;’ ==> The 4th character 'A' is invalid for the picture character 9.
-
-
Picture characters for numeric character data
-
Numeric character data represents numeric values. The picture characters X and A cannot be used in a picture specification for numeric character data.
-
Numeric character data has either arithmetic value or character value according to its usage.
Classification Description Arithmetic value
An arithmetic value is used in the following cases:
-
A variable is used in an expression from a result of a coded arithmetic or bit value.
-
A variable is assigned to a coded arithmetic, numeric character, or bit variable.
-
A variable is used with the C, E, F, B, and P (numeric) format in an edit-directed I/O.
An arithmetic value of a numeric character variable is converted to a coded arithmetic value internally.
Character value
A character value is used in the following cases:
-
A variable is used in a character expression.
-
A variable is assigned to a character variable.
-
Data is outputted using list-directed or data-directed output.
-
A variable is outputted using edit-directed output with the A or P (character) format items.
-
-
-
Digits and decimal points
-
The picture characters 9 and V represent fixed-point decimal values.
Picture Character Description 9
Decimal digit.
V
Decimal point. Storage is not allocated for data.
-
The following are examples:
Source Attribute Source Data Picture Specification Character Value FIXED(5)
12345
99999
12345
FIXED(5)
12345
99999V
12345
FIXED(5)
12345
999V99
undefined (the SIZE condition occurs.)
FIXED(5)
12345
V99999
undefined (the SIZE condition occurs.)
FIXED(7)
1234567
99999
undefined (the SIZE condition occurs.)
FIXED(3)
123
99999
00123
FIXED(5,2)
123.45
999V99
12345
FIXED(7,2)
123.45.67
9V9
undefined (the SIZE condition occurs.)
FIXED(5.2)
123.45
99999
00123
-
-
Zero suppression
-
The picture character Z and asterisk (*) replace leading zeros with blanks or asterisks.
Leading zeros are positions located in the left of the leftmost digit of a data item. An actual digit is saved in positions that are not leading zeros.
Picture Character Description Z
Replaces leading zeros with blanks.
*
Replaces leading zeros with asterisks.
-
The following are examples:
Source Attribute Source Data Picture Specification Character Value FIXED(5)
12345
ZZZ99
12345
FIXED(5)
00100
ZZZ99
bb100
FIXED(5)
00100
ZZZZZ
bb100
FIXED(5)
00000
ZZZZZ
bbbbb
FIXED(5,2)
123.45
ZZZ99
bb123
FIXED(5,2)
001.23
ZZZV99
bb123
FIXED(5)
12345
ZZZV99
undefined (the SIZE condition occurs.)
FIXED(5)
000.08
ZZZVZZ
bbb08
FIXED(5.2)
000.00
ZZZVZZ
bbbbb
FIXED(5)
00100
*****
**100
FIXED(5)
00000
*****
*****
FIXED(5.2)
000.01
***V**
***01
FIXED(5.2)
95
$**9.99
$**0.95
FIXED(5.2)
12350
$**9.99
$123.50
-
-
Insertion characters
-
The picture characters comma (,), point (.), slash (/), and B inserts a character into a corresponding position.
Picture Character Description comma (,), point (.), slash (/)
Inserts a picture character if zero suppression does not occur. If zero suppression occurs, the picture character will be inserted only in the following cases:
-
An unsuppressed digit is located in the left of a character’s position.
-
A V exists in the left position of a character and the fractional part is a non-zero.
-
A picture character is in the first position.
In other cases that zero suppression occurs, the picture character will be treated as a zero suppression character.
B
Inserts an unconditional blank.
-
-
The following are examples:
Source Attribute Source Data Picture Specification Character Value FIXED(4)
1234
9,999
1,234
FIXED(6,2)
1234.56
9,999V.99
1,234.56
FIXED(4,2)
12.34
ZZ.VZZ
12.34
FIXED(4,2)
00.03
ZZ.VZZ
bbb03
FIXED(4,2)
00.03
ZZV.ZZ
bb.03
FIXED(4,2)
12.34
ZZ.VZZ
12.34
FIXED(4,2)
00.00
ZZZV.ZZ
bbbbb
FIXED(9.2)
1234567.89
9,999,999V.99
1,234,567.89
FIXED(7.2)
12345.67
**,999V.99
12.345.67
FIXED(7,2)
00123.45
**,999V.99
***123.45
FIXED(9.2)
1234567.89
9.999.999V,99
1.234.567,89
FIXED(6)
123456
99/99/99
12/34/56
FIXED(6)
123456
99.9/99.9
12.3/45.6
FIXED(6)
001234
ZZ/ZZ/ZZ
bbb12/34
FIXED(6)
000012
ZZ/ZZ/ZZ
bbbbbb12
FIXED(6)
000000
ZZ/ZZ/ZZ
bbbbbbbb
FIXED(6)
000000
**/**/**
********
FIXED(6)
000000
**B**B**
**b**b**
FIXED(6)
123456
99B99B99
12b34b56
FIXED(3)
123
9BB9BB9
1bb2bb3
FIXED(2)
12
9BB/9BB
1bb/2bb
-
-
Signs and currency symbols
-
The picture characters S, +, and - represent sign symbols.
Picture Character Description $, \
Represents the currency symbol. Zero suppression will occur for leading zeros.
S
Represents either the plus sign character (+) if an arithmetic value of a data item is greater than or equal to 0, or the minus sign character (-) if it is less than 0. Zero suppression will occur for leading zeros.
+
Represents either the plus sign character (+) if an arithmetic value of a data item is greater than or equal to 0, or a blank if it is less than 0. Zero suppression will occur for leading zeros.
-
Represents either a blank if an arithmetic value of a data item is greater than or equal to 0, or a minus sign character (-) if it is less than 0. Zero suppression will occur for leading zeros.
-
The following are examples:
Source Attributes Source Data Picture Specification Character value FIXED(5,2)
123.45
$999V.99
$123.45
FIXED(5,2)
012.00
99$
12$
FIXED(5,2)
001.23
$ZZZV.99
$bb1.23
FIXED(5,2)
000.00
$ZZZV.ZZ
bbbbbbb
FIXED(1)
0
$$$.$$
bbbbbb
FIXED(5,2)
123.45
$$$9V.99
$123.45
FIXED(5,2)
001.23
$$$9V.99
bb$1.23
FIXED(2)
12
$$$,999
bbb$012
FIXED(4)
1234
$$$,999
b$1,234
FIXED(5,2)
2.45
SZZZV.99
+bb2.45
FIXED(5)
214
SS,SS9
bb+214
FIXED(5)
-4
SS,SS9
bbbb-4
FIXED(5.2)
-123.45
+999V.99
b123.45
FIXED(5.2)
-123.45
-999V.99
-123.45
FIXED(5.2)
123.45
999V.99S
123.45+
FIXED(5.2)
001.23
++B+9V.99
bbb+1.23
FIXED(5.2)
001.23
---9V.99
bbb1.23
FIXED(5.2)
-001.23
SSS9V.99
bb-1.23
-
-
Overpunched characters
-
The picture characters T, I, and R replaces a digit (0 - 9) with another character according to the sign of the digit.
-
A digit is replaced as follows:
Overpunched Characters -
The following are examples:
Source Attributes Source Data Picture Specification Character value FIXED(4,2)
21.05
ZZV9T
210E
FIXED(3,2)
-0.07
ZZV9T
bb0P
FIXED(4,2)
21.05
ZZV9I
210E
FIXED(3,2)
-0.07
ZZV9I
bb07
FIXED(3)
132
R99
132
FIXED(3)
-132
R99
J32
FIXED(5,2)
001.23
$ZZZV.99
$ 1.23
-
-
Zero replacement
-
The picture character Y replaces 0 with a blank.
Source Attributes Source Data Picture Specification Character value FIXED(5)
00100
YYYYY
bb1bb
FIXED(5)
10203
9Y9Y9
1b2b3
FIXED(5,2)
000.04
YYYVY9
bbbb4
-
-
Exponent characters
-
The picture characters K and E represent an exponent of a floating-point value.
Picture Character Description K
Indicates that the right positions are for an exponent of a floating-point value.
K only represents information and does not require storage.
E
Indicates that the right positions are for an exponent of a floating-point value.
E requires storage.
-
The following are examples:
Source Attribute Source Data Picture Specification Character Value FLOAT(5)
0.12345E06
V.99999E99
.12345E06
FLOAT(5)
0.12345E-06
V.99999ES99
.12345E-06
FLOAT(5)
0.12345E+06
V.99999KS99
.12345+06
FLOAT(5)
-123.45E+12
S999V.99ES99
-123.45E+12
FLOAT(5)
001.23E-01
SSS9.V99ESS9
123.00Eb-3
FLOAT(5)
001.23E+04
ZZZV.99KS99
123.00E+02
FLOAT(5)
001.23E+04
SZ99V.99ES99
+123.00E+02
FLOAT(5)
001.23E+04
SSSSV.99E-99
+123.00Eb02
-
2. Data Conversion
This section describes computational data conversions.
PL/I converts data to assign a data item with attributes to another data item with different attributes.
Conversion of a computational data value can change its internal attributes such as its precision to represent an arithmetic value, and the length to represent a string value.
Data is converted in the following cases:
Case | Target Attribute |
---|---|
Assignment |
Attributes of a variable to assign. |
Operand in expression |
Determined by the rules for evaluating expressions. |
Stream input (GET statement) |
Receiving field attributes. |
Stream output (PUT statement) |
Determined by a specified format if a stream is edit-directed. If not, it is converted to a string. |
Argument to PROCEDURE or ENTRY |
Corresponding parameter attributes. |
Argument to built-in function or pseudo variable |
Determined according to a built-in function or pseudo variable type. |
RETURN statement expression |
The RETURN attributes specified in a PROCEDURE statement. |
All attributes of a source and target data items, except for the length of a string, must be determined during compilation. Conversion requires a specific process.
In the following example, the decimal representation (packed decimal) 1.23 is converted to binary (11,7) before precision conversion is performed. The result is a binary (15,5) value of 1.00111B.
DCL A FIXED DEC(3,2) INIT(1.23); DCL B FIXED BIN(15,5); B = A;
2.1. Converting String Lengths
A source string is saved to a target string in a left-to-right order.
If a source string is longer than a target string, the excess part of the source string will be ignored and the STRINGSIZE condition will be triggered. If a target string is fixed-length and longer than a source string, the remaining part of the target string will be padded with the following characters:
-
Blanks for a CHARACTER string
-
'0’B characters for a BIT string
-
DBCS blanks for a GRAPHIC string
2.2. Converting Precision
If an arithmetic value of a source has the same attributes as a target except for its precision, a precision conversion must be performed.
For fixed-point data, point alignment is maintained during precision conversion. Therefore, padding or truncation can occur. For floating-point data, padding with 0 or truncation can occur.
2.3. Converting Other Data Attributes
Source-to-target rules are applied to conversions of data with the attributes listed below. For more information, refer to Source-to-Target Conversion Rules.
-
Coded arithmetic
-
FIXED BINARY
-
FIXED DECIMAL
-
FLOAT BINARY
-
FLOAT DECIMAL
-
-
Arithmetic character PICTURE
-
CHARACTER
-
BIT
-
GRAPHIC
A value converted between decimal data and binary data can change. The conversion is performed using the factor 3.32 as follows:
-
n decimal digits are converted to CEIL(n * 3.32) binary digits.
-
n binary digits are converted to CEIL(n / 3.32) decimal digits.
2.4. Source-to-Target Conversion Rules
The following describes how to convert each type:
2.4.1. Target: Coded Arithmetic
-
Source: FIXED BINARY, FIXED DECIMAL, FLOAT BINARY, FLOAT DECIMAL
-
Each type will be described below.
-
-
Source: Arithmetic char PICTURE
-
Data is converted to a FIXED DECIMAL or FLOAT DECIMAL value with scale and precision determined by the PICTURE attribute, and then the decimal value is converted according to target attributes.
-
-
Source: CHARACTER
-
A source CHARACTER string must represent a valid arithmetic constant which can start with a sign symbol. A blank is not allowed between a constant and its sign symbol.
-
A constant has base, scale, mode, and precision attributes. These attributes are converted according to target attributes during assignment.
-
If an intermediate result is required (e.g., during evaluation of an operation expression), the result will be a decimal fixed-point value of a precision (N, 0). The fractional part will be lost.
-
-
Source: BIT
-
If a conversion occurs during the evaluation of an operation expression, a BIT string is converted to an unsigned FIXED BINARY(M,0) value. After the conversion, the value is converted according to target attributes.
-
If a source BIT string is longer than the maximum precision, bits on the left are ignored. If non-zero bits are ignored, the SIZE condition occurs.
-
-
Source: GRAPHIC
-
A GRAPHIC string is converted to a CHARACTER value, and then the value is converted according to the CHARACTER conversion rule.
-
2.4.2. Target: FIXED BINARY(p2, q2)
-
Source: FIXED DECIMAL(p1, q1)
-
The following shows a result precision:
p2 = min(M, 1+ CEIL(p1 * 3.32)) , q2 = CEIL(ABS(q1 * 3.32)) * SIGN(q1)
-
-
Source: FLOAT BINARY(p1)
-
The following shows a result precision.
p2 = p1
q2 is calculated using a binary point position.
-
-
Source: FLOAT DECIMAL(p1)
-
A result precision is calculated using the same equations as the conversion from FIXED DECIMAL to FIXED BINARY.
-
p1 is a declared value and q1 is calculated using a decimal point position.
-
-
Source: Arithmetic character PICTURE
-
For more information, refer to Target: Coded Arithmetic.
-
-
Source: CHARACTER
-
For more information, refer to Target: Coded Arithmetic.
-
-
Source: BIT
-
For more information, refer to Target: Coded Arithmetic.
-
-
Source: GRAPHIC
-
For more information, refer to Target: Coded Arithmetic.
-
2.4.3. Target: FIXED DECIMAL(p2, q2)
-
Source: FIXED BINARY(p1, q1)
-
The following shows a result precision:
p2 = 1 + CEIL(p1 / 3.32), q2 = CEIL(ABS(q1 / 3.32)) * SIGN(q1)
-
-
Source: FLOAT BINARY(p1)
-
The following shows a result precision:
p2 = p1
p1 is a declared value and q1 is calculated using a binary point position.
-
-
Source: FLOAT DECIMAL (p1)
-
The following shows a result precision:
p2 = p1
q2 is calculated using a decimal point position.
-
-
Source: Arithmetic character PICTURE
-
For more information, refer to Target: Coded Arithmetic.
-
-
Source: CHARACTER
-
For more information, refer to Target: Coded Arithmetic.
-
-
Source: BIT
-
For more information, refer to Target: Coded Arithmetic.
-
-
Source: GRAPHIC
-
For more information, refer to Target: Coded Arithmetic.
-
2.4.4. Target: FLOAT BINARY(p2)
-
Source: FIXED BINARY(p1, q1)
-
The following shows a result precision:
p2 = p1
-
-
Source: FIXED DECIMAL(p1,q1)
-
The following shows a result precision:
p2 = CEIL(p1 * 3.32)
-
-
Source: FLOAT DECIMAL(p1)
-
The following shows a result precision:
p2 = CEIL(p1 * 3.32)
-
-
Source: Arithmetic character PICTURE
-
For more information, refer to Target: Coded Arithmetic.
-
-
Source: CHARACTER
-
For more information, refer to Target: Coded Arithmetic.
-
-
Source: BIT
-
For more information, refer to Target: Coded Arithmetic.
-
-
Source: GRAPHIC
-
For more information, refer to Target: Coded Arithmetic.
-
2.4.5. Target: FLOAT DECIMAL(p2)
-
Source: FIXED BINARY(p1, q1)
-
The following shows a result precision:
p2 = CEIL( p1 / 3.32 )
-
-
Source: FIXED DECIMAL(p1, q1)
-
The following shows a result precision:
p2 = p1
-
-
Source: FLOAT BINARY(p1)
-
The following shows a result precision:
p2 = CEIL(p1 / 3.32)
-
-
Source: Arithmetic character PICTURE
-
For more information, refer to Target: Coded Arithmetic.
-
-
Source: CHARACTER
-
For more information, refer to Target: Coded Arithmetic.
-
-
Source: BIT
-
For more information, refer to Target: Coded Arithmetic.
-
-
Source: GRAPHIC
-
For more information, refer to Target: Coded Arithmetic.
-
2.4.6. Target: Arithmetic character PICTURE
An arithmetic character PICTURE data item is a character representation of a fixed-point or floating-point decimal value.
The following describes the rules of conversion from each source to an arithmetic character PICTURE data item without loss of left and right digits:
-
Source: FIXED BINARY(p1, q1)
-
A target must imply the following:
fixed decimal(1 + x + q - y, q) or float decimal(x)
Where x >= CEIL(p1 / 3.32), y = CEIL(q1 /3.32), and q >= y.
-
-
Source: FIXED DECIMAL(p1, q1)
-
A target must imply the following:
fixed decimal(x + q - q1, q) or float decimal(x)
Where x >= p1 and q >= q1.
-
-
Source: FLOAT BINARY(p1)
-
A target must imply the following:
fixed decimal(p, q) or float decimal(p)
Where p >= CEIL(p1 / 3.32).
-
-
Source: FLOAT DECIMAL(p1)
-
A target must imply the following:
fixed decimal(p, q) or float decimal(p)
Where p >= p1.
-
-
Source: CHARACTER
-
For more information, refer to Target: Coded Arithmetic.
-
-
Source: BIT(n)
-
A target must imply the following:
fixed decimal(1 + x + q, q) or float decimal(x)
Where x >= CEIL(n / 3.32) and q >= 0.
-
-
Source: GRAPHIC
-
For more information, refer to Target: Coded Arithmetic.
-
2.4.7. Target: CHARACTER
-
Source: FIXED BINARY(p1, q1)
-
Converted to a FIXED DECIMAL value according to conversion rules, and then the value is converted according to the FIXED DECIMAL to CHARACTER conversion rule.
-
-
Source: FIXED DECIMAL(p1, q1)
-
Converted to a character constant.
-
If p1 >= q1 >= 0, a constant is right adjusted and has the length of p1 + 3. The 3 is required for a possible minus sign, decimal or binary point, and a leading zero before the point.
-
Leading zeros are replaced with blanks. If an integer part is 0, 0 before the decimal point can be an exception.
-
A minus sign comes after the first digit of a negative number. A positive number has no sign symbol.
-
If q1 = 0, no decimal point is used.
-
-
If p1 < q1 or q1 < 0, a scaling factor exists in the right-most position.
The following is the syntax of a scaling factor:
F{+|-}nn
Where {+|-}nn has the value of -q1. The length of a character string is p1 + k + 3, where k is the length excluding the scaling factor’s F and sign symbol.
-
The following are examples:
Precision Value String (6, 0)
4352
'bbbbb4352'
(3, 1)
-43.2
'b-43.2'
(4, -3)
-4352000
'-4352F+3'
-
-
Source: FLOAT BINARY(p1)
-
Converted to a FLOAT DECIMAL value according to conversion rules, and then the value is converted according to the FLOAT DECIMAL to CHARACTER conversion rule.
-
-
Source: FLOAT DECIMAL(p1)
-
Converted according to an E-format item.
-
The intermediate string length is p1 + 8, the number of fractional digits is p1 - 1, and the number of significant digits is p1.
-
The following are examples.
Precision Value String (6)
4384*10**5
'b4.38400E+0008'
(6)
-.005832
'-5.83200E-0003'
(4)
1
'b1.000E+0000'
-
-
Source: Arithmetic character PICTURE
-
An arithmetic character PICTURE data item saves an arithmetic value in a string format. Therefore, string data will become an identical character string of the arithmetic value.
-
When a source is assigned to character data, the rules for converting string lengths are applied.
-
-
Source: BIT
-
Bit 0 and 1 are converted to the character 0 and 1 respectively.
-
A null bit string is converted to a null character string.
-
-
Source: GRAPHIC
-
Converted to SBCS (Single-byte Character Set) if there is SBCS that corresponds to DBCS. Otherwise, the CONVERSION condition occurs.
-
2.4.8. Target: BIT
-
Source: FIXED BINARY, FIXED DECIMAL, FLOAT BINARY, FLOAT DECIMAL
-
An arithmetic value is converted to binary. A sign and fractional parts are ignored. The resulting binary value is treated as a bit string.
-
Converted to a FIXED DECIMAL value according to conversion rules, and then the value is converted according to the FIXED DECIMAL to CHARACTER conversion rule.
-
-
Source: FIXED BINARY(p1, q1)
-
The length of an intermediate bit string is calculated as follows:
min(M, p1 - q1)
If p1 - q1 ⇐ 0, the result is a null bit string.
-
The following are examples:
Precision Value String (1)
1
'1’B
(3)
-5
'101’B
(4, 2)
2.43
'10’B
-
-
Source: FIXED DECIMAL( p1, q1 )
-
The length of an intermediate bit string is calculated as follows:
min(M, CEIL( (p1 - q1) * 3.32))
If p1 - q1 ⇐ 0, the result is a null bit string.
-
The following are examples:
Precision Value String (1)
1
'00001’B
(2, 1)
-1.8
'0001’B
-
-
Source: FLOAT BINARY(p1)
-
The length of an intermediate bit string is calculated as follows:
min(M, p1)
-
-
Source: FLOAT DECIMAL(p1)
-
The length of an intermediate bit string is calculated as follows:
min(M, p1 * 3.32)
-
-
Source: Arithmetic character PICTURE
-
A source is interpreted as a DECIMAL value, and then the value is converted according to the FIXED DECIMAL or FLOAT DECIMAL to BIT conversion rule.
-
-
Source: CHARACTER
-
Character 0 and 1 are converted to the 0 and 1 bits respectively. For other characters, the CONVERSION condition occurs.
-
The null character string is converted to a null bit string.
-
-
Source: GRAPHIC
-
DBCS 0 and 1 are converted to the 0 and 1 bits respectively. For other DBCS characters, the CONVERSION condition occurs.
-
The null string is converted to a null bit string.
-
3. Data Declarations
When a PL/I program is executed, it processes various data items with various types. Before using data items (except for constants without a name), their information such as attributes and names must be declared. For more information about data items, types, and attributes, refer to Data Elements.
This section describes explicit and implicit declarations, declaration scope, data alignment, defaults for attributes, arrays, and structures.
3.1. Explicit Declaration
A data item name is declared explicitly in the following cases. The scope of a data item declared explicitly is the block that includes the declaration.
-
When a name is declared in a DECLARE statement. Its name and attributes are declared explicitly in a DECLARE statement.
-
When a name is declared as an entry constant. A label in a PROCEDURE or ENTRY statement is an explicitly declared entry constant.
-
When a name is declared as a format constant. A label in a FORMAT statement is a explicitly declared format constant.
-
When a name is declared as a label constant. A label constant explicitly declares a label.
The following shows an example scope of an explicit declaration. B and B' (C and C') indicate two different uses of the same name.
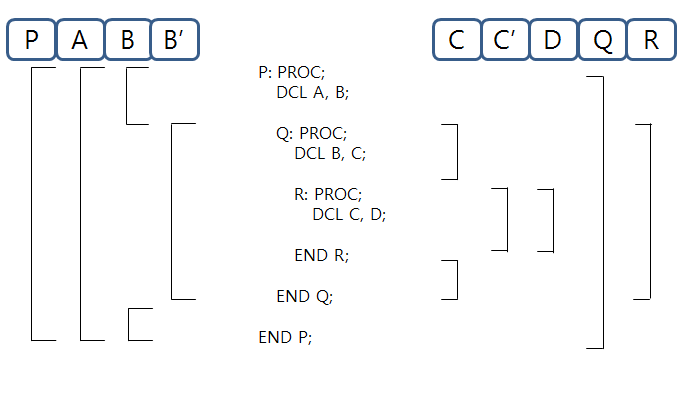
3.1.1. DECLARE Statement
The DECLARE statement specifies a data item’s name and some or all attributes. If the attributes are not declared explicitly and cannot be determined by context, default attributes will be used.
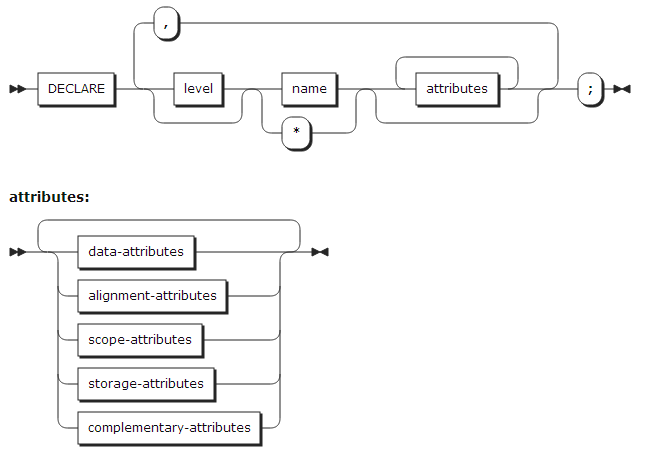
Component | Description |
---|---|
* |
Cannot be used for the names of scalar and level-1 data. |
attributes |
Data attributes in any order. For more information, refer to Data Types and Attributes. |
level |
Positive integer. The default level of scalar or array data is level-1. |
name |
Data name. A level-1 name must be unique in a block. |
Condition prefixes and labels must not be specified in a DECLARE statement. |
3.2. Implicit Declaration
If a data item name is used in a program without explicit declaration, it is declared implicitly. The scope is the same as when a data item name is declared immediately after an external PROCEDURE statement in which the name is used. Some attributes of a data item declared implicitly are determined by context. This is called a contextual declaration and occurs in the following cases:
-
For built-in function names
-
When a data item in a parameter list of a PROCEDURE or ENTRY statement has the PARAMETER attribute.
-
When a data item’s name is in the place of a file name, the data item will have the FILE attribute.
-
When a data item in a ON CONDITION, SIGNAL CONDITION, or REVERT CONDITION statement has the CONDITION attribute.
-
When a data item’s name is in the BASED attribute or in the left-hand side of a locator qualification symbol, the data item will have the POINTER attribute.
If an attribute is declared implicitly but not determined contextually, the default attribute is determined according to Defaults for Attributes. Since a contextual declaration cannot exist in the scope of an explicit declaration, it is impossible to add attributes by contextually evaluating the explicit declaration.
3.3. Scope of Declarations
In most cases, the scope of a declared data item is determined by the position in which it is declared. The scope for an implicit declaration is the same as when a DECLARE statement is used immediately after the PROCEDURE statement of an external procedure.
The scope of a name that is explicitly declared in a block is the block. The name is unknown in outer blocks unless the same name is declared in the outer blocks. Even though the same name is declared in outer blocks, a data item that is different from the data item referred to by the inner block is referred to by the outer blocks. This characteristics allows a local definition in a program.
In the following example, the result for A in the PUT statement is 'FUNC1' because C.A is referred to and the result for B is 'TEST' declared in the TEST procedure.
TEST: PROC OPTIONS(MAIN); DCL (X,Y) CHAR(5) INIT('TEST '); CALL FUNC1; FUNC1: PROC ; DCL 1 C, 3 A CHAR(5) INIT('FUNC1'); PUT DATA( A, B ); END FUNC1; END TEST;
INTERNAL and EXTERNAL Attributes (Abbreviations: INT and EXT)
The INTERNAL and EXTERNAL attributes define the scope of reference.
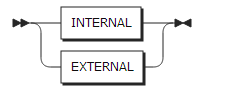
INTERNAL is the default attribute for entry names of internal procedures and all other variables except for entry constants, file constants, and user-defined conditions. It specifies that the scope of reference for a name is a block in which it is declared.
EXTERNAL is the default attribute for file constants, user-defined conditions, and entry constants, except for internal procedures. A name with this attribute can be declared multiple times in other external procedures. All declarations use the same name with matching EXTERNAL attribute and refer to the same data.
In the following example, if X.A in TEST1 is changed, X.A in TEST2 will be also changed. If X.B in TEST1 is changed, X.Y in TEST2 will be also changed.
TEST1: PROC; DCL 1 X EXTERNAL, 3 A. 3 B; ... END TEST1; TEST2: PROC; DCL 1 X EXTERNAL, 3 A. 3 Y; ... END TEST2;
Structure members always have the INTERNAL attribute.
Since EXTERNAL declarations for the same name refer to the same data, they must have the same attributes.
3.4. Data Alignment
A computer accesses data in byte, halfword, fullword, or doubleword units. A halfword, fullword, and doubleword are 2, 4, and 8 consecutive bytes, respectively. Therefore, for halfword, fullword, and doubleword alignments, addresses with multiples of 2, 4, and 8 are accessed.
PL/I supports data alignment in a unit that a computer accesses. This allows unused bytes between successive data items, so that additional storage is required.
Whether to use data alignment in a unit is specified using the ALIGNED and UNALIGNED attributes.
3.4.1. ALIGNED and UNALIGNED Attributes
The ALIGNED attribute aligns a data item according to the data type’s scope.
The UNALIGNED attribute aligns a data item according to its next byte (or next bit for fixed-length bit strings).
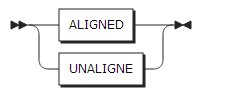
Variable Type | Storage Size (Bytes) | Alignment for ALIGNED Data | Alignment for UNALIGNED Data |
---|---|---|---|
BIT(n) |
ALIGNED: CEIL(n / 8) UNALIGNED: n bits |
Byte |
Bit |
CHARACTER(n) |
n |
Byte |
Byte |
GRAPHIC(n) |
2n |
Byte |
Byte |
PICTURE |
Number of PICTURE characters (excluding V, K, and F) |
Byte |
Byte |
DECIMAL FIXED(p,q) |
CEIL((p + 1) / 2) |
Byte |
Byte |
BINARY FIXED(p, q) SIGNED 1 ⇐ p ⇐ 7 UNSIGNED 1 ⇐ p ⇐ 8 |
1 |
Byte |
Byte |
CHARACTER(n) VARYING |
n + 2 |
Halfword |
Byte |
BINARY FIXED(p, q) SIGNED 8 ⇐ p ⇐ 15 UNSIGNED 9 ⇐ p ⇐ 16 |
2 |
Halfword |
Byte |
BINARY FIXED(p, q) SIGNED 16 ⇐ p ⇐ 31 UNSIGNED 17 ⇐ p ⇐ 32 |
4 |
Fullword |
Byte |
BINARY FLOAT(p) 1 ⇐ p ⇐ 21 |
4 |
Fullword |
Byte |
DECIMAL FLOAT(p) 1 ⇐ p ⇐ 6 |
4 |
Fullword |
Byte |
POINTER |
8 for 64bit |
Doubleword |
Byte |
FILE |
8 for 64bit |
Doubleword |
Byte |
ENTRY |
8 for 64bit |
Doubleword |
Byte |
LABEL |
8 for 64bit |
Doubleword |
Byte |
FORMAT |
8 for 64bit |
Doubleword |
Byte |
BINARY FIXED(p, q) SIGNED 32 ⇐ p ⇐ 63 UNSIGNED 33 ⇐ p ⇐ 64 |
8 |
Doubleword |
Byte |
BINARY FLOAT(p) 22 ⇐ p ⇐ 53 |
8 |
Doubleword |
Byte |
DECIMAL FLOAT(p) 7 ⇐ p ⇐ 16 |
8 |
Doubleword |
Byte |
3.5. Defaults for Attributes
Every name in a PL/I program requires a complete set of attributes. Arguments passed to a procedure must have attributes that match the procedure’s parameters. Values returned by a function must have expected attributes. However, user-specified attributes do not always require a complete set of attributes.
The following must use language-specified defaults or a new set of defaults determined by modifying language-specified defaults using a DEFAULT statement:
-
Explicitly declared names
-
Implicitly or contextually declared names
-
Attributes included in parameter descriptions
-
Values returned by function procedures
Attributes for explicitly or contextually declared names cannot be redefined by default attributes.
3.5.1. Language-specified Defaults
If a variable is not declared with any data attributes, it uses arithmetic attributes by default.
If mode, scale, and base are not specified by a DECLARE or a DEFAULT statement, the DEFAULT statement provided by a compiler determines attributes as follows:
-
If DEFAULT is applied, variables with names that starts with the letter I through N will have the REAL FIXED BINARY(15,0) attribute.
-
The other variable has the REAL FLOAT DECIMAL(6) attribute.
If a scaling factor is specified in a precision attribute, the FIXED attribute will be applied before other attributes. Therefore, a declaration with the attribute BINARY(p,q) will always be the same as a declaration with the FIXED BINARY(p,q) attribute.
If a precision is not specified in an arithmetic declaration, it is determined according to the DEFAULT attribute provided by the compiler as follows:
Attribute | DEFAULT (IBM) |
---|---|
FIXED DECIMAL |
(5,0) |
FIXED BINARY |
(15,0) |
FLOAT DECIMAL |
(6) |
FLOAT BINARY |
(21) |
If no description is in an ENTRY declaration, argument attributes must be the same as a procedure’s parameter attributes.
In the following example, the argument has the attribute REAL FIXED DECIMAL(1, 0):
DECLARE X ENTRY; CALL X(1);
If a procedure X has a parameter with a different attribute an error will occur as shown below.
X: PROC(Y); DECLARE Y BINARY FIXED(15);
If all parameter attributes are specified in an ENTRY declaration, the error will be prevented.
3.5.2. DEFAULT Statement (Abbreviation: DFT)
The DEFAULT statement defines data-attribute defaults when a set of attributes is not complete.
Attributes to which a DEFAULT statement for implicit, contextual, and partially-complete declarations are not applied are determined by language-specified defaults.
A DEFAULT statement redefines all attributes except for a name declared with the ENTRY or FILE attributes. However, the CONSTANT attribute is applied implicitly to a name without attributes that implies the VARIABLE attribute by PL/I before the DEFAULT statement is applied.

identifiers:
Component | Description |
---|---|
RANGE(identifier) |
Defaults to apply to names that start with the same letter. |
RANGE(identifier : identifier) |
Defaults to apply to names that start with one of the letters between the letters specified when using two identifiers. |
RANGE(*) |
Defaults to apply to all names in the scope of a DEFAULT statement. |
DESCRIPTORS |
Defaults to apply to attributes included in the description of a parameter in a parameter description list of an explicit Entry declaration. At least one attribute must exist. DESCRIPTORS will not be applied to NULL descriptions. |
attribute_specification |
|
Multiple DEFAULT statements can be used in a block. The scope of a DEFAULT statement is the block in which the statement is declared, all blocks within the block that do not include another DEFAULT statement with the same range, and all blocks that are not contained in a block with a DEFAULT statement with the same range.
A DEFAULT statement affects only names declared explicitly in an internal block because the scope of an implicit declaration is determined implicitly as if the names were declared implicitly using a DECLARE statement when the names immediately follows a PROCEDURE statement of an external procedure.
The following is an example:
X: PROC; LABEL1: DEFAULT RANGE(AB) FLOAT; Y: BEGIN; LABEL2: DEFAULT RANGE(ABC) FIXED; END X;
The scope of the DEFAULT statement of LABEL1 is the procedure X and begin-block Y included in the procedure. The scope specified by the DEFAULT statement of LABEL1 are all names that start with AB and are declared in the procedure X and begin-block Y. However, the names that start with ABC and declared in begin-block Y are excluded and can be used only within begin-block Y.
3.6. Arrays
An array is an n-dimensional collection of elements with the same attributes. Each item of an array can be referred to based on the location in the array using a subscript. If an array is not specified using REFER, it must have at least one element for every dimension.
3.6.1. DIMENSION Attribute (Abbreviation: DIM)
The DIMENSION attribute describes the number of dimensions and upper and lower bounds of an array. The number of integers between and including the lower and upper bounds is the extent.
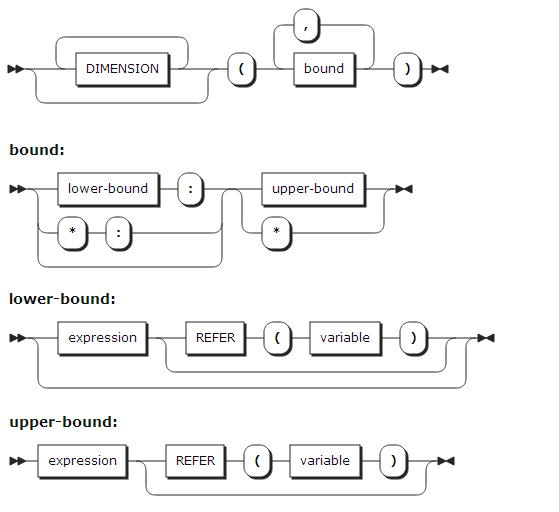
If the DIMENSION keyword is omitted, the DIMENSION attribute must come after the name of a declared data item.
Specifications for bounds are as follows:
-
If only an upper-bound is specified, a lower-bound is 1 by default.
-
A lower-bound must be less than or equal to an upper-bound.
-
If an asterisk (*) is used for a lower-bound or an upper-bound, the lower-bound or upper-bound can be obtained from the argument corresponding to the parameter.
3.6.2. Subscript
In the following example, an array with the extent of 7 and bounds of -2:4 is declared:
DCL X(-2:4) FIXED DEC(7);
If 1, 2, 3, 4, 5, 6, and 7 are assigned to the array, each element value is as follows:
X(-2) = 1 X(-1) = 2 X(0) = 3 X(1) = 4 X(2) = 5 X(3) = 6 X(4) = 7
The number (-2 through 4 in the above example) specified within parentheses is used to refer to each element in an array as a subscript.
3.7. Structures
A structure is a collection of member elements that can be structures, arrays, and scalar variables. The name of a structure is used to refer to all data of the structure. Unlike an array, members of a structure can have different names and attributes. An asterisk (*) can be used to describe members that are not referred.
A structure has a level. A name described in level-1 is called a major structure. Names described under level-1 are called minor structures. Names described in the lowest level are called elementary variables.
A structure is declared by specifying a level-number (integer) before the name of a variable in a DECLARE statement.
The following example declares a structure.
DCL 1 EMPLOYEE, /* major structure name */ 3 NAME, /* minor structure name */ 5 LAST CHAR(20), /* elementary name */ 5 FIRST CHAR(20), /* elementary name */ 3 EMP_NO FIXED DEC(7); /* minor structure name */
If a member name of a structure is unique, the member can be referred to by using its name. If the same name is used by another member, the member cannot be referred to by only using its name. To refer to an exact member, a qualifier is required.
A qualifier is described by connecting parent member names using a point (.) in the order of levels. To refer to LAST in the above example, EMPLOYEE.LAST or EMPLOYEE.NAME.LAST can be used as a qualifier.
DCL Y FIXED INIT(1); BEGIN; DCL 1 X, 3 Y FIXED INIT(10); DISPLAY (Y); END;
Since the scope of a variable is determined in a block unit, the variable Y referred to by the DISPLAY statement in the above example is checked from variables declared in the begin-block first.
Since only one Y is used in the begin-block, member variable Y of the structure X in the begin-block is referred to and 10 is outputted as the result value. If the variable Y is declared with the initialization value of 1 within the begin-block, the scalar variable Y, not the member variable Y of the structure X, is referred to and 1 is outputted as the result value.
DCL 1 A, 5 B, 5 C, 10 D, 5 D;
In the above example, D is declared in two places. A.D refers to the second D, which is in the level number 5. To refer to the first D, A.C.D must be used as a qualifier.
DCL 1 A, 5 B, 10 C, 5 D, 10 C;
In the above example, A.C cannot determine which C must be referred to.
DCL 1 A, 5 A. 10 A;
In the above example, A, A.A, and A.A.A refer to the first, second, and third A, respectively.
DCL X; DCL 1 Y, 5 X. 10 Z. 10 A. 5 Y, 10 Z, 10 A;
In the above example, X refers to the first X. Y.Z cannot determine which Z must be referred to. Y.X.Z and Y.Y.Z refer to the first and second Z, respectively.
3.8. LIKE Attribute
The LIKE attribute is used for a variable that is declared to use the same logical organization of another structure. The member names and their attributes of a target variable are declared in the same way as the members of a variable to declare. A target variable’s name and attributes are ignored.

A new member cannot be added to a structure.
DCL 1 A(10) ALIGNED STATIC, 3 B CHAR(10), 3 C CHAR(10); DCL 1 X LIKE A;
The above declaration is the same as the following:
DCL 1 A(10) ALIGNED STATIC, 3 B CHAR(10), 3 C CHAR(10); DCL 1 X, 3 B CHAR(10), 3 C CHAR(10);
The variable A’s DIM(10), ALIGNED, and STATIC attributes are not affected by LIKE.
4. Storage Control
All variables require storage. This section describes how to control the storage used in PL/I.
Storage Classes
There are four classes for storage: automatic, static, controlled, and based.
Class | Description |
---|---|
automatic |
Storage is allocated when a block in which a variable is declared is activated. It is released when the block is deactivated. For more information, refer to AUTOMATIC Attribute (Abbreviation: AUTO). |
static |
Storage is allocated when a program is loaded. It is not freed until the program execution completes. For more information, refer to STATIC Attribute. |
controlled |
Storage is allocated and released using the ALLOCATE and FREE statements, respectively. If storage is allocated multiple times for one controlled variable, storages for variables will be stacked. To refer to an earlier generation, later ones must be released. For more information, refer to CONTROLLED Attribute (Abbreviation: CTL). |
based |
Storage is allocated and released using the ALLOCATE and FREE statements, respectively. Unlike a controlled variable, storages for variables are not stacked. For more information, refer to BASED Attributes. |
Storage class attributes can be specified explicitly for elements, arrays, and major structure variables but cannot be specified for the following:
-
CONDITION conditions
-
Defined data items
-
Entry constants
-
File constants
-
Format constants
-
Label constants
-
Structure members
4.1. AUTOMATIC Attribute (Abbreviation: AUTO)
Storage is allocated when a block in which a variable is declared is activated, and released when the block is deactivated.
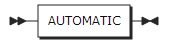
AUTOMATIC is the default attribute. Automatic variables are always internal.
The extent of an automatic variable can be described using restricted expressions that are expressions for which constant values are determined during compilation. Expressions for referring to another variable can also be used for this if the referred variable has an initial value when declared.
DCL N FIXED BIN (31) INIT(15), X CHAR(N);
In the above example, the string length of the variable X is specified using the variable N. Since N has an initial value of 15, X becomes a string variable with the length of 15.
4.2. STATIC Attribute
Storage is allocated when a program is loaded. It is not freed until the program execution completes.
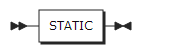
STATIC is the default attribute for external variables and can be specified for internal variables. Variables declared outside a procedure have the STATIC attribute.
P: PACKAGE; DCL A CHAR(10); FUNC1: PROC OPTIONS(MAIN); FUNC2: PROC; DCL B STATIC INTERNAL; END FUNC2; END FUNC1; FUNC3: PROC; Y = 'TEST'; END FUNC3; END P;
In the above example, the variable B has the STATIC attribute so that storage for B is allocated when the program execution starts. B can be referred to only within the block FUNC2 that includes the declaration of B. The variable A also has the STATIC attribute.
Static variables are initialized using the INITIAL attribute, and the initial variable must be a restricted expression. An array extent must also be specified using a restricted expression.
4.3. CONTROLLED Attribute (Abbreviation: CTL)
For variables declared with the CONTROLLED attribute, storage is allocated using an ALLOCATE statement. Storage is freed using a FREE statement or when a program execution ends. If a controlled variable for which storage is not allocated is referred to, an error will occur.
A non-controlled variable cannot be used to call a procedure that uses a controlled variable as a parameter.
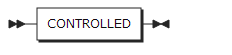
The following example uses a controlled variable:
FUNC1: PROC; DCL X(M,N) CONTROLLED; DCL M FIXED, N FIXED; ... CALL FUNC2; FUNC2: PROC; GET LIST(M,N); ALLOCATE X; GET LIST(X); ... END FUNC2; ... FREE X; END FUNC1;
Generation Management for Controlled Variables
When an ALLOCATE statement is executed, storage for a controlled variable is allocated and stacked. Previously allocated and unreleased storage is pushed down. This stacking generates a new generation for the variable. The newly created generation becomes a current generation. The previous generation cannot be accessed unless the current generation is released. If a FREE statement is executed, the current generation storage is brought up and removed from the stack, and the previous generation becomes the current generation.
4.3.1. ALLOCATE Statement for Controlled Variables
The ALLOCATE statement allocates storage for controlled variables. Controlled parameters can also be allocated. An ALLOCATE statement can describe the array bounds and string length of a controlled variable.
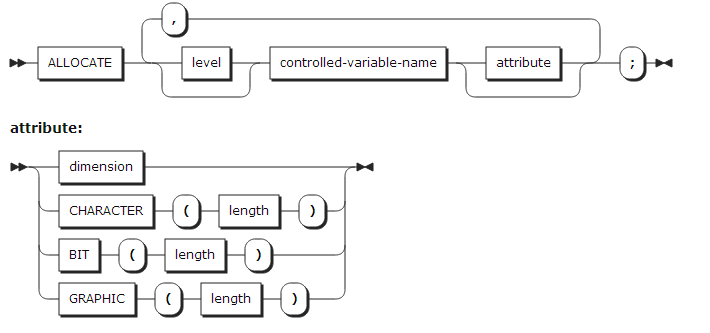
Array bounds and string lengths are evaluated when an ALLOCATE statement is executed.
-
An ALLOCATE statement or a DECLARE or DEFAULT statement must specify required size and string length information.
-
If the DIMENSION attribute or a string length are specified in an ALLOCATE statement, they will override attributes specified during a variable declaration.
-
If a bound or string length is specified as an asterisk (*) in an ALLOCATE statement, the information of the current generation will be used. If there is no current generation, the information will be undefined and a program error will occur.
-
The DIMENSION attribute specified in an ALLOCATE statement must match the number of declared dimensions. That is, n DIMENSION attributes must be specified for an n-dimensional array.
-
The BIT, CHARACTER, and GRAPHIC attributes must be the same as the attributes declared in a DECLARE statement.
The following example uses an ALLOCATE statement:
DCL X(M,N) CHAR(L) CTL; M = 10; N = 5; L = 100; ALLOCATE X(5,5) CHAR(200);
4.3.2. FREE Statement for Controlled Variables
The FREE statement releases storage allocated for controlled variables.
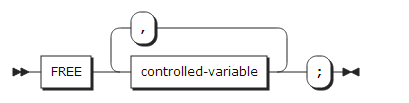
4.3.3. Asterisk Notation
If a dimension or string length is specified as an asterisk (*) in an ALLOCATE statement, the value of a current generation will be used. The same amount of asterisks must be specified as the number of dimensions of an array.
DCL X(M,N) CHAR(L) CTL; M = 10; N = 5; L = 100; ALLOCATE X; ALLOCATE X(5,5) CHAR(200); ALLOCATE X(*,*) CHAR(*);
In the above example, the first generation of the variable X has dimensions of (10,5) and a string length of 100. The second and third generations have dimensions of (5,5) and string lengths of 200.
4.3.4. Adjusting Extents
Controlled scalars, arrays, and members of structures can have adjustable array extents and string lengths.
In the following example, when a structure is allocated using the ALLOCATE statement, X.Y has the extent 1 to 8 and X.Z is a variable-length string with the maximum length of 4.
DCL 1 X CTL, 3 Y(M:N). 3 Z CHAR(*) VARYING; M = -4; N = 4; ALLOC 1 X, 3 Y(1:8), 3 Z CHAR(4); FREE A;
4.4. BASED Attributes
A based variable declaration is a description of a required storage size and its attributes. The location of the storage is identified using a locator. If an unallocated based variable is referred to, an error will occur.
Storage is allocated using the ALLOCATE statement and released using the FREE statement.

Based variables cannot have the EXTERNAL attribute. Any variable can be referred to using a locator.
Extent Specifications in Based Variable Declarations
If the REFER option is not used, the extent specifications in the BASED declarations must be restricted expressions with the following exceptions:
-
A non-constant array extent must meet the following conditions:
-
It is a one-dimensional array.
-
A lower bound is a constant.
-
When it is a part of a structure, extents of all other members are constant and there is no following member of the array and its parent structures.
-
-
A non-constant CHARACTER string extent must meet the following conditions:
-
It is the last member of a structure.
-
It has no parents that are arrays.
-
It has one of the UNALIGNED and NONVARYING attributes.
-
-
The following non-constant extents are valid:
-
BIT extent
-
GRAPHIC extent
-
4.4.1. Locator Variables
A locator variable indicates a location of a based variable in storage. PL/I currently supports only pointer locator variables.
In the following example, X can be referred to using the locator variable P, which indicates the address of X in storage.
DCL X CHAR(10) BASED (P);
If a based variable is declared without a locator declaration, it can be referred to explicitly using a locator-qualifier as in the following example:
DCL X CHAR(5) BASED ; DCL Y CHAR(10); DCL Z CHAR(20); DCL P PTR; P = ADDR(Y); Z = P->X;
In the following example, the arrays A and C have the same storage. B and C(2,1) also have the same storage.
DCL A(3,2) CHAR(5) BASED (P); DCL B CHAR(5) BASED(Q); DCL C(3,2) CHAR(5); P = ADDR(C); Q = ADDR(A(2,1));
4.4.2. Locator Qualification
A locator qualification specifies one or more qualifiers by using the symbol '→'.

In the following example, X is a based variable, P is a locator variable, and Q is a based locator variable:
P->Q->X
The value of P is the address of Q. The value of Q in the address is the address of X. X can be referred to using the address that Q indicates.
Based variables can also be referred to using an implicit locator-qualifier.
In the following example, the ALLOCATE statement saves the address of storage allocated for X to the pointer variable P.
DCL X FIXED BIN BASED(P); ALLOC X; X = 3;
In "X = 3;", X is referred to using the P implicit locator-qualifier. The statement is the same as "P→X = 3;".
DCL (P(10),Q) PTR, R PTR BASED(Q), V BASED(P(3)), W BASED(R), Y BASED; ALLOCATE R,V,W;
For the above example, the following reference is valid:
P(3)->V V Q->R->W R->W W
The first two references are the same and the last three references are the same. Y must be referred to using a locator variable qualifier.
4.4.3. POINTER Variable and Attribute (Abbreviation: PTR)
A pointer variable is declared contextually if it is specified in a based variable declaration or in the SET option in an ALLOCATE, LOCATE, READ, or FETCH statement. It can also be declared by explicitly specifying the POINTER attribute.
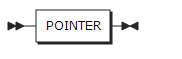
4.4.4. ALLOCATE Statement for Based Variables
The ALLOCATE statement allocates storage for based variables and saves a storage address to a locator variable.

Allocated storage size is determined according to a based variable’s attributes and the length and the size determined when the ALLOCATE statement is executed.
4.4.5. FREE Statement for Based Variables
The FREE statement releases storage allocated for based variables.

4.4.6. REFER Attribute
String lengths or array bounds can be defined in a based structure using a variable called a refer object. When storage is allocated for the structure, a value will be assigned to it.

The following is an example:
DCL 1 VAR BASED(P), 3 A FIXED BINARY (31), 3 B (UBND REFER(A)); DCL UBND FIXED BINARY(31) INIT(100);
When VAR is allocated, the upper bound of the B array will be set to the current value of the variable UBND. The value is assigned to the variable A. When referring to B, the upper bound value is obtained from A.
4.5. INITIAL Attribute (Abbreviation: INIT)
The INITIAL attribute sets an initial value to a variable when storage is allocated for the variable. This attribute cannot be used for defined data or parameters.
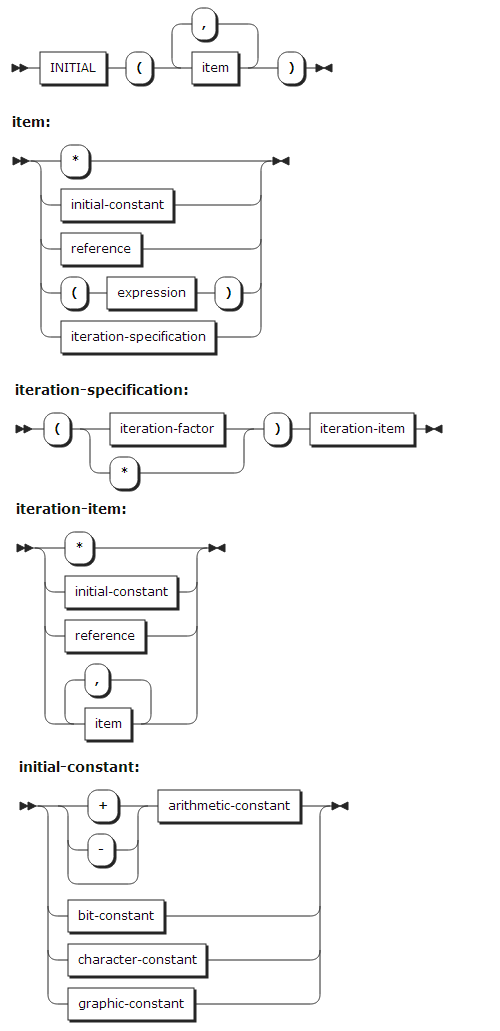
Component | Description |
---|---|
* |
Used to refrain remaining elements from being initialized, except when the elements are used as iteration factors. |
iteration factor |
Number of times an Iteration item repeats. This is mainly used for arrays. If an asterisk (*) is used, remaining elements are initialized to the value specified in an iteration item. |
constant, reference, expression |
Initial values. |
The following are examples of using iteration factors:
((2)'A') ==> ('AA') ((2)('A')) ==> ('A', 'A') ((2)(1)'A') ==> ('A', 'A') ((*)(1)('A') ==> ('A', 'A', ..., 'A')
The following example initializes variables using the INITIAL attribute:
DCL X FIXED DEC (7,3) INIT( 34.503 ); DCL Y CHAR(10) INIT( 'TEST INIT'); DCL Z (100,10) FIXED DEC(5) INIT( (920)0, (20) ((3)5, 9) );
Z is an array that has 1,000 extents. Its 920 extents are initialized to 1 by the INITIAL attribute, and the remaining 80 extents are initialized according to repetitions of the sequence 5, 5, 5, and 9.
4.6. AREA Data
An AREA variable defines an area of storage allocated and released for a based variable. It can be declared using the AREA attribute and will have one of the 4 storage classes.
An area size can be specified using the same method for specifying string length and array size or the REFER option. It can also be specified as an asterisk (*).

Component | Description |
---|---|
expression |
Area size. If no expression or asterisk is specified, the default value will be 1,000. |
* |
Used to specify an area size if the AREA variable is a parameter. |
REFER |
Refer to "REFER Attribute". |
The following example declares an AREA variable:
DECLARE AREA1 AREA(1500), AREA2 AREA;
An area has an additional 16 bytes of memory for control information about current storage usage in addition to its defined size. Therefore, the sizes of AREA1 and AREA2 are 1,516 and 1,016 respectively.
When a based variable is allocated to an area, a part of the area is returned. When it is released, the area used by it becomes available for other variables. An area manages currently allocated storages using a chain. A head of a chain is included in the control information. Therefore, if control information is modified, an unexpected error can occur.
If the size of a based variable to allocate is less than a remaining area size, the AREA condition will occur.
4.7. DEFINED and POSITION Attributes (Abbreviation: DEF and POS)
The DEFINED attribute enables a part of storage or all of it for a base variable to be used as storage for a declared variable.

Component | Description |
---|---|
reference |
Base variable that has storage for a declared variable. Base variables must not have the BASED or DEFINED attributes. |
Defined variables do not inherit attributes from base variables. They are internal and must be level-1 variables. They can have the DIMENSION attribute, but cannot have the INITIAL, AUTOMATIC, BASED, CONTROLLED, STATIC, and PARAMETER attributes.
There are 3 types of defined variables:
-
simple
-
iSUB (not supported)
-
string overlay
The type is determined according to the following:
-
If the POSITION attribute is specified, the type will be a string overlay.
-
If the POSITION attribute is not specified, attributes of a defined variable and a base variable will be compared using the matching rule used when a program is called to compare parameters and arguments. If they match (except for the DEFINED attribute), the type will be simple. When the matching rule is applied, dimension bounds and string lengths are regarded as asterisks and they are not compared.
-
For all other situations the type will be a string overlay.
Simple Defining
Simple defining can refer to other variables such as scalar, array, structure, and so on. The ALIGNED and UNALIGNED attributes of a defined variable and a base variable must be the same.
DCL X(5,5,5); DCL A(2,2,2) DEF X; DCL B(5,5) DEF X((*,*,5); DCL C DEF X(L,M,N);
A is a three-dimensional array of X(2,2,2). B is a two-dimensional array of X(1,1,5) through X(5,5,5). The two defined variables are stored to unconnected storage, not adjacent storage.
OpenFrame PL/I currently does not support unconnected storage. |
C refers to the storage of X(L,M,N) that corresponds to the current values of L, M, and N.
DCL X CHAR(100), Y CHAR(10) DEF X;
Y is a string that has the first 10 characters of X.
String Overlay Defining
Defined and base variables must be a string or picture data. They cannot have ALIGNED and VARYING attributes.
DCL X CHAR(100), Y(10,10) CHAR(1) DEF X; DCL A(10) CHAR(1), B CHAR(10) DEF A;
POSITION Attribute
The POSITION attribute is used only for a string overlay. Base variables specify the starting position of defined variables.
DCL B(10,10) BIT(1), X BIT(40) DEF B POS(20);
X is a bit string that has 40 elements of B, starting from the 20th element.
DCL A PIC '999V.999', X(7) CHAR(1) DEF (A), Y CHAR(3) DEF (A) POS(5), Z(4) CHAR(1) DEF(A) POS(3);
X is a string array that consists of strings of the picture data A. Y is a string corresponding to the last 3 characters of A which are '999'. X is a string corresponding to the '9.99' value of A.
DCL X(20) CHAR(10), Y(10) CHAR(5) DEF (X) POSITION(1);
Y is a string that has the first 50 characters of the X string. POSITION(1) must be specified. Otherwise, it will have a simple definition which will produce a different result.
4.8. OFFSET Data
The OFFSET variable indicates the distance of an allocated based variable from the starting position of an area.

An OFFSET variable can be converted to a pointer value by being associated with an area if an AREA variable is specified. If an AREA variable is not specified, it must be specified explicitly using a POINTER built-in function.
A value can be saved to an OFFSET variable as follows:
-
By using an ALLOCATE statement
-
By saving a value of another OFFSET or POINTER variable
-
By using a built-in function such as ADDR, OFFSET, and NULL