Conditions
The chapter describes conditions used in OpenFrame PL/I.
1. Condition Handling
Condition handling is handling of exceptions that can occur during PL/I program executions.
A condition occurs if a specific condition is met. A user can cause a condition manually. If a condition occurs, a current program is stopped and ON-unit specified by a user is executed. If there is no ON-unit specified by a user, a default action specified internally is executed. After the ON-unit is executed, the PL/I program continues from the line in which the execution was stopped or ends depending on the condition.
1.1. ON Statement
The ON statement registers an ON-unit to execute when a specified condition occurs.
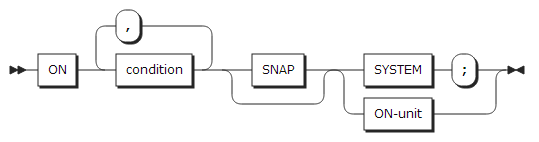
Component | Description |
---|---|
condition |
One or more conditions. For more information, refer to Conditions. |
SNAP |
Not supported. |
SYSTEM |
Default action for a condition. For more information, refer to Conditions. |
ON-unit |
Statements to execute when a condition occurs. An ON-unit can be a single statement or a begin-block. An ON-unit is executed when a corresponding condition is met, not when an ON statement is executed. |
If a specified condition occurs, an ON-unit will be executed. If a same condition is specified multiple times, the ON-unit of the last ON statement will be executed. Conditions specified using an ON statement are released when a block or procedure with the ON statement is terminated or by a user using a REVERT statement.
The following example registers the ZERODIVIDE condition:
ON ZERODIVIDE BEGIN; DISPLAY('The ZERODIVIDE condition occurs.'); END; A = B / 0;
The ZERODIVIDE condition occurs when a division by 0 is attempted. The condition occurs due to "A = B / 0", so that the DISPLAY statement is executed because an ON-unit registered through the ON statement is executed.
1.2. REVERT Statement
The REVERT statement unregisters an ON-unit that was registered using an ON statement.
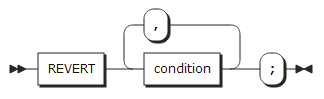
Component | Description |
---|---|
condition |
One or more conditions. For more information, refer to Conditions. |
A REVERT statement only unregisters an ON-unit registered in a current block or procedure and cannot unregister an ON-unit registered in an upper block or procedure. If there is no ON-unit registered in a current block or procedure, a REVERT statement will not be executed.
The following example unregisters an ON-unit by using a REVERT statement:
ON ZERODIVIDE BEGIN; DISPLAY('ZERODIVIDE condition raised'); END; REVERT ZERODIVIDE; A = B / 0;
The ZERODIVIDE condition occurs due to "A = B / 0;" but the ON-unit registered using the ON statement is not executed because it has been unregistered by using the REVERT statement. If there is no registered ON-unit, a default action is executed according to a condition; either the ERROR condition occurs or the program execution continues. The ZERODIVIDE condition set an ERROR condition as the default action.
1.3. SIGNAL Statement
The SIGNAL statement causes a condition to enable a user to execute an ON-unit.
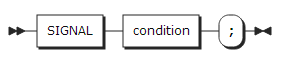
Component | Description |
---|---|
condition |
Condition. For more information, refer to Conditions. |
If an ON-unit is registered by a user, the ON-unit will be executed. If there are none, a default action specified internally will be executed.
In the following example, an ON-unit using a SIGNAL statement has been executed:
ON ZERODIVIDE BEGIN; DISPLAY('ZERODIVIDE condition occurs.'); END; SIGNAL ZERODIVIDE;
The ON-unit of the ZERODIVIDE condition is executed because of the SIGNAL statement. Even though a condition related to I/O has occurred, the corresponding ON-unit is not executed if the relevant files are different.
1.4. Condition Prefixes
A user can enable and disable a condition by using a condition prefix.
A condition prefix comes before a statement, begin-block, or procedure. If a condition prefix comes before a begin-block or procedure, it is applied to all statements within the block or procedure.
The following example enables and disables a condition using a condition prefix:
(SIZE):A:PROCEDURE; ... (NOSIZE) B = C; D = E; ... END A;
The SIZE condition is checked for all statements within PROCEDURE A using the SIZE prefix but not for the "B = C;" statement preceded by the NOSIZE prefix. If there is a conditional prefix immediately followed by a statement, the prefix has top priority. If there is no conditional prefix immediately followed by a statement, a conditional prefix in an upper block has higher priority. If a conditional prefix does not exist, a default prefix will be used.
If a condition is enabled, a compiler will check whether a condition can occur and execute an ON-unit for the condition if the condition is met. If a condition is disabled by a conditional prefix but the condition occurs, a program error may occur.
Some conditions related to I/O cannot be disabled using conditional prefixes. For information about default values (enabled or disabled), refer to the status of each condition in Conditions.
2. Conditions
OpenFrame PL/I supports the following conditions:
Condition | Description |
---|---|
Occurs when any other condition occurred but there is no ON-unit for the condition. |
|
Caused by an area-related issue. |
|
Enables users to define a condition name, register the condition using the name, and cause the condition. |
|
Occurs in an ASSIGNMENT statement or during I/O operations. |
|
Occurs when the last record of a dataset is read by a GET or READ statement. |
|
Occurs when the number of lines is greater than the PAGESIZE value (default value: 60) specified in the PRINT file. |
|
Occurs as a default or normal action of other conditions. |
|
Occurs if the length of a result of a FIXED DECIMAL arithmetic operation exceeds 15. |
|
Used according to various statements. |
|
Occurs during data-directed I/O from a GET statement. |
|
Occurs if a floating-point value exceeds a maximum value. |
|
Used according to the length of a variable in a READ, WRITE, or REWRITE statement. |
|
Occurs when an error except for the RECORD and KEY conditions occurs during I/O operations. |
|
Occurs when a file cannot open. |
|
Occurs when a floating-point value is less than the minimum value that can be expressed as a non-zero IEEE floating-point value. |
|
Occurs when division by 0 is attempted. |
OpenFrame PL/I does not support the ATTENTION, FINISH, INVALIDOP, SIZE, STORAGE, STRINGRANGE, STRINGSIZE, and SUBSCRIPTRANGE conditions. |
The following clauses provide each condition’s description along with its status, syntax, default and normal actions, and code:
Item | Description |
---|---|
Status |
Default status of a condition: enabled, disabled, and always enabled. |
Syntax |
Syntax of a condition. |
Default Action |
Action to perform when a condition occurs but there is no ON-unit for the condition. |
Normal Action |
ON-unit to perform when a condition occurs. There must be ON-unit for the condition. |
Code |
Condition code. For more information, refer to ONCODE. |
2.1. ANYCONDITION (Abbreviation: ANYCOND)
The ANYCONDITION condition occurs when any other condition occurred but there is no ON-unit for the condition.
A user cannot manually cause the ANYCONDITION condition to occur by using a SIGNAL statement.
A detailed description is as follows:
-
Status
Always enabled
-
Syntax
ANYCONDITION -
Action
Action Description Default Action
Default action of an occurred condition.
Normal Action
Normal action of an occurred condition.
-
Code
None
2.2. AREA
The AREA condition occurs in the following cases:
-
When a based variable is assigned to an area and the area is insufficient for the variable.
-
When an AREA variable is assigned to another AREA variable and the size of the assigned target area is bigger than that of the source area.
A detailed description is as follows:
-
Status
Always enabled
-
Syntax
AREA -
Action
Action Description Default Action
The ERROR condition occurs.
Normal Action
The program execution continues from the next statement of the statement that caused the AREA condition. If the AREA condition occurs while assigning a based variable, the variable will not be assigned so that a program error can occur afterward.
-
Code
360, 361, 362
2.3. CONDITION (Abbreviation: COND)
The CONDITION condition enables users to define a condition name, register the condition using the name, and cause the condition.
The CONDITION condition can only be caused by a SIGNAL statement. To register and cause the CONDITION condition, the condition name must be defined using the CONDITION attribute in advance.
The following example defines a condition name using the CONDITION attribute:
DCL TEST CONDITION;
The following example registers a condition using a defined name and causes the condition.
ON CONDITION (TEST) BEGIN; ... END; ... SIGNAL CONDITION (TEST);
A detailed description is as follows:
-
Status
Always enabled
-
Syntax
CONDITION -
Action
Action Description Default Action
The program execution continues from the next statement of the SIGNAL statement.
Normal Action
The program execution continues from the next statement of the SIGNAL statement.
-
Code
500
2.4. CONVERSION (Abbreviation: CONV)
The CONVERSION condition can occur in an ASSIGNMENT statement or during I/O operations.
The CONVERSION condition occurs in the following cases:
-
When character data is converted to bit data and the character data has a character that is not 0 or 1.
-
When character data is converted to arithmetic data and the character data has a character that is not a digit.
-
When character data is converted to character picture data and the character data has a character that is not allowed by a picture specification.
If the CONVERSION condition occurs, a character that caused the condition can be queried using the built-in functions, ONCHAR or ONSOURCE, in an ON-unit. The character can be modified using a pseudo variable of the built-in function. For more information, refer to ONSOURCE and ONCHAR.
A detailed description is as follows:
-
Status
Enabled
-
Syntax
CONVERSION -
Action
Action Description Default Action
The ERROR condition occurs.
Normal Action
If data that caused a condition was modified using a pseudo variable of ONCHAR or ONSOURCE in an ON-unit, the conversion of the data will be re-attempted. If the data was not modified, the ERROR condition occurs.
-
Code
600, 612, 615, 618
2.5. ENDFILE
The ENDFILE condition occurs when the last record of a dataset is read by a GET or READ statement.
A detailed description is as follows:
-
Status
Always enabled
-
Syntax
ENDFILEComponent Description file-reference
File for which an ENDFILE condition is checked. (Default value: SYSIN file)
-
Action
Action Description Default Action
The ERROR condition occurs.
Normal Action
The program execution will continue from the next statement of the statement that caused the ENDFILE condition.
-
Code
70
2.6. ENDPAGE
The ENDPAGE condition occurs when the number of lines specified in a PRINT file is greater than the PAGESIZE value (default value: 60).
An ON-unit can start a new page using the PAGE option or a PAGE format item. The start line will be initialized to 1. If an ON-unit does not start a new page, the number of lines will increase continuously, and the ENDPAGE condition will not occur until a new page starts.
A detailed description is as follows:
-
Status
Always enabled
-
Syntax
ENDPAGEComponent Description file-reference
File for which an ENDPAGE condition is checked. (Default value: SYSPRINT file)
-
Action
Action Description Default Action
The program is normally executed.
Normal Action
The program is normally executed.
-
Code
90
2.7. ERROR
The ERROR condition occurs as a default or normal action for other conditions. This condition can occur even if an undefined error occurs.
If the ERROR condition occurs while an ON-unit for an ERROR condition is performed, the ON-unit will be performed again. This may cause an infinite loop to occur. This issue can be prevented by adding the "ON ERROR SYSTEM;" statement to the ON-unit as the first statement.
A detailed description is as follows:
-
Status
Always enabled
-
Syntax
ERROR -
Action
Action Description Default Action
The FINISH condition occurs.
Normal Action
Same as the default action.
-
Code
1000 or more
2.8. FIXEDOVERFLOW (Abbreviation: FOFL)
The FIXEDOVERFLOW condition occurs if the length of the result of a FIXED DECIMAL arithmetic operation exceeds 15.
The following example causes the FIXEDOVERFLOW condition:
DCL A FIXED DEC(15) INIT(1234567890); DCL B FIXED DEC(15) INIT(123456); DISPLAY(A * B);
Since the length of the result of the A * B operation is 16, that exceeds the maximum length for a FIXED DECIMAL, thus the FIXEDOVERFLOW condition occurs.
If the operation result is a FIXED BINARY, the FIXEDOVERFLOW condition will not occur. This condition is not caused by the ADD, SUB, MUL, and DIV built-in functions.
A detailed description is as follows:
-
Status
Enabled
-
Syntax
FIXEDOVERFLOW -
Action
Action Description Default Action
The ERROR condition occurs.
Normal Action
The program is normally executed. The operation result can be incorrect.
-
Code
310
2.9. KEY
The KEY condition occurs in the following cases:
-
When a record for a KEY option does not exist in a READ, REWRITE, or DELETE statement.
-
When a KEY already exists in a dataset for a KEYFROM option in a WRITE or LOCATE statement.
-
When the length of a KEY defined for an indexed dataset is different from that of a variable specified by a KEY option.
When a LOCATE statement is used, a KEY condition can occur when the next LOCATE statement or a FLUSH or CLOSE statement is used.
A detailed description is as follows:
-
Status
Always enabled
-
Syntax
KEYComponent Description file-reference
File for which a KEY condition is checked.
-
Action
Action Description Default Action
The ERROR condition occurs.
Normal Action
The program is normally executed. The I/O statement can be not performed.
-
Code
50, 51, 52
2.10. NAME
The NAME condition occurs during a data-directed I/O from a GET statement in the following cases:
-
When there is no matching name or the name is incorrect.
-
When there is no subscript or the subscript is incorrect.
A detailed description is as follows:
-
Status
Always enabled
-
Syntax
NAMEComponent Description file-reference
File for which a NAME condition is checked.
-
Action
Action Description Default Action
Ignores incorrect data fields and continues to execute the GET statement.
Normal Action
Continues to execute the GET statement.
-
Code
10
2.11. OVERFLOW (Abbreviation: OFL)
The OVERFLOW condition occurs if a floating-point value exceeds a maximum value.
The maximum values of floating-point values are as follows:
-
FLOAT DECIMAL digit numbers 1 - 6 and FLOAT BINARY digit numbers 1 - 21: 3.40282347E+38F
-
FLOAT DECIMAL digit numbers 7 - 16 and FLOAT BINARY digit numbers 22 - 53: 1.7976931348623157E+308
A negative value can cause the OVERFLOW condition if the magnitude of the value exceeds a maximum value. |
A detailed description is as follows:
-
Status
Enabled
-
Syntax
OVERFLOW -
Action
Action Description Default Action
The ERROR condition occurs.
Normal Action
The ERROR condition occurs.
-
Code
300
2.12. RECORD
The RECORD condition occurs in the following cases:
-
When the length of a record in a dataset is longer than that of a variable in a READ statement.
-
When the length of a record in a dataset is shorter than that of a variable in a WRITE or REWRITE statement.
If a RECORD condition occurs, exceeded data will be truncated.
A detailed description is as follows:
-
Status
Always enabled
-
Syntax
RECORDComponent Description file-reference
File for which a RECORD condition is checked.
-
Action
Action Description Default Action
The ERROR condition occurs.
Normal Action
The program is normally executed. Exceeded data will be truncated.
-
Code
21, 22
2.13. TRANSMIT
The TRANSMIT condition occurs when an error (except for the RECORD and KEY conditions) occurs during I/O operations.
Since OpenFrame PL/I uses OpenFrame’s file system, OpenFrame’s error messages are referred to when a TRANSMIT condition occurs.
A detailed description is as follows:
-
Status
Always enabled
-
Syntax
TRANSMITComponent Description file-reference
File for which a TRANSMIT condition is checked.
-
Action
Action Description Default Action
The ERROR condition occurs.
Normal Action
The program will be normally executed.
The I/O statement can be not executed. If a dataset has an issue, the TRANSMIT condition can occur repeatedly.
-
Code
41, 42
2.14. UNDEFINEDFILE (Abbreviation: UNDF)
The UNDEFINEDFILE condition occurs if a file cannot open in the following cases:
-
When the file has already opened.
-
When DD cannot be found.
-
When dataset information cannot be fetched.
-
When dataset attributes are different from attributes specified by a file.
-
When the key length of an indexed dataset is 0.
-
When the value of the KEYLOC option is defined for an indexed dataset and the value of KEYLENGTH + KEYLOC is greater than the record length.
A detailed description is as follows:
-
Status
Always enabled
-
Syntax
UNDEFINEDFILEComponent Description file-reference
File for which an UNDEFINEDFILE condition is checked.
-
Action
Action Description Default Action
The ERROR condition occurs.
Normal Action
The program will be normally executed, but an error can occur if an I/O statement is used.
-
Code
93
2.15. UNDERFLOW (Abbreviation: UFL)
The UNDERFLOW condition occurs when a floating-point value is less than a minimum value that is expressed as a non-zero IEEE floating-point value.
The minimum values of floating-point values are as follows:
-
FLOAT DECIMAL digit numbers 1 - 6 and FLOAT BINARY digit numbers 1 - 21: 1.17549435E-38F
-
FLOAT DECIMAL digit numbers 7 - 16 and FLOAT BINARY digit numbers 22 - 53: 2.2250738585072014E-308
A negative value can cause the UNDERFLOW condition if the magnitude of the value is less than a minimum value. |
A detailed description is as follows:
-
Status
Enabled
-
Syntax
UNDERFLOW -
Action
Action Description Default Action
The ERROR condition occurs.
Normal Action
The ERROR condition occurs.
-
Code
330
2.16. ZERODIVIDE (Abbreviation: ZDIV)
The ZERODIVIDE condition occurs when a division by 0 for a fixed-point or float-point value is attempted. If the ZERODIVIDE condition is disabled, a program error can occur.
A detailed description is as follows:
-
Status
Enabled
-
Syntax
ZERODIVIDE -
Action
Action Description Default Action
The ERROR condition occurs.
Normal Action
The ERROR condition occurs.
-
Code
320