Statements
This chapter describes how to use OpenFrame PL/I statements.
1. ALLOCATE Statement (Abbreviation: ALLOC)
The ALLOCATE statement allocates storage for controlled or based variables.
1.1. ALLOCATE Statement for Controlled Variables
The ALLOCATE statement allocates storage for controlled variables. Controlled parameters can also be allocated. An ALLOCATE statement can describe the array bounds and string length of a controlled variable.
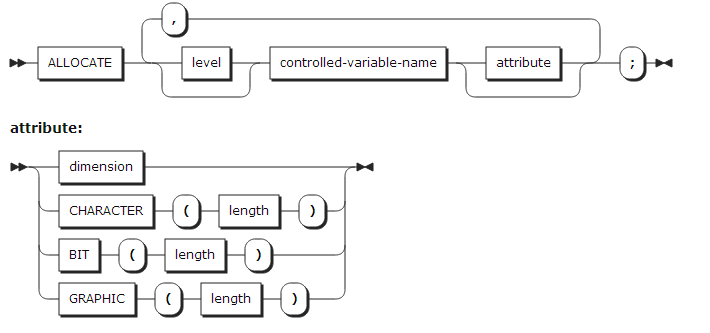
Array bounds and string lengths are evaluated when an ALLOCATE statement is executed.
-
An ALLOCATE statement or a DECLARE or DEFAULT statement must specify required size and string length information.
-
If the DIMENSION attribute or a string length are specified in an ALLOCATE statement, they will override attributes specified during a variable declaration.
-
If a bound or string length is specified as an asterisk (*) in an ALLOCATE statement, the information of the current generation will be used. If there is no current generation, the information will be undefined and a program error will occur.
-
The DIMENSION attribute specified in an ALLOCATE statement must match the number of declared dimensions. That is, n DIMENSION attributes must be specified for an n-dimensional array.
-
The BIT, CHARACTER, and GRAPHIC attributes must be the same as the attributes declared in a DECLARE statement.
The following example uses an ALLOCATE statement:
DCL X(M,N) CHAR(L) CTL; M = 10; N = 5; L = 100; ALLOCATE X(5,5) CHAR(200);
1.2. ALLOCATE Statement for Based Variables
The ALLOCATE statement allocates storage for based variables and saves a storage address to a locator variable.

Allocated storage size is determined according to a based variable’s attributes and the length and the size determined when the ALLOCATE statement is executed.
2. ASSIGN Statement
The assignment statement evaluates an expression and assigns a result value to one or more target variables. If a target or pseudo variable attributes are different from source attributes, data conversion will be required.

Component | Description |
---|---|
reference |
A target or pseudo variable. |
expression |
A source expression. |
BY NAME |
An option used in a structure assignment. For more information, refer to "Assignment Execution". |
Compound Assignment Statement
A compound assignment statement uses compound assignment operations.

Component | Description |
---|---|
reference |
Target variable or pseudo variable. |
compound operator |
Operator to apply to the source and target before the assignment is made. Compound operators that can be used in an assignment statement are:
|
expression |
Expression of the source. |
The following are examples of executing compound assignment statements.
X += 5; ==> X = X + 5; X *= Y - 1; ==> X = X * (Y - 1);
Target Variables
Target variables can be elements, arrays, structures, or pseudo variables.
-
Array
-
A target variable must be an array of scalars or structures. A source must be a scalar expression or an expression with the same number of dimensions and the same bounds as a target.
-
-
Structure
-
If BY NAME is specified, each target and source must be a structure.
-
If BY NAME is not specified, each target must be a structure and each source must be a scalar or a structure that is the same as a target.
-
If BY NAME is not specified and a source is described as a NULL string("") then:
-
all targets will be padded with '00’X.
-
all numeric targets will 0.
-
all character and graphic targets will be padded with blanks.
-
-
Assignment Execution
-
Array
-
All array operands (of sources and targets) must have the same number of dimensions and the same bounds.
-
An array assignment is expanded into a loop as follows:
DO I1 = LBOUND( target-variable, 1 ) to HBOUND( target-variable, 1 ); DO I2 = LBOUND( target-variable, 2 ) to HBOUND( target-variable, 2 ); ... DO IN = LBOUND( target-variable, N ) to HBOUND( target-variable, N ); generated element assignment ; END;
-
N is the number of dimensions of a target. In the generated element assignment, I1 through IN are used as subscripts. If a source is scalar, subscripts will not be used.
-
-
Structure
-
If BY NAME is not specified:
-
All operands must be the same structure, not an array. The member of a structure can be an array.
-
An assignment will be executed on each member. If the number of members is n, assignments will be executed n times.
-
If a member is an aggregate, assignments will be executed on all members of the member.
-
-
If BY NAME is specified:
-
The same name as the name of a target structure member will be searched from a source from the first member. The members will be direct children of the structure, not children of a child of the structure.
-
A search will be repeated until a same name is found.
-
If a searched member is a structure or an array of structures, an assignment with the BY NAME option will be executed.
-
If a searched member is a scalar or an array of scalars, an element assignment will be executed.
-
The above steps will be repeated for following members.
-
-
The following is an example of a structure assignment using BY NAME:
DCL 1 ONE, DCL 1 TWO, DCL 1 THREE 2 PART1, 2 PART1, 2 PART1, 3 RED, 3 BLUE, 3 RED, 3 ORANGE, 3 GREEN, 3 BLUE, 2 PART2, 3 RED, 3 BROWN, 3 YELLOW, 2 PART2, 2 PART2, 3 BLUE, 3 BROWN, 3 YELLOW, 3 GREEN; 3 YELLOW; 3 GREEN;
-
4. CALL Statement
A CALL statement calls a subroutine.
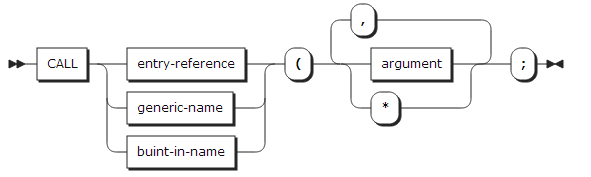
Component | Description |
---|---|
entry-reference |
Subroutine name. |
generic-name |
Variable name declared as GENERIC. |
built-in name |
Built-in function name. |
argument |
List of arguments to pass into the subroutine. |
5. CLOSE Statement
The CLOSE statement closes a specified file.
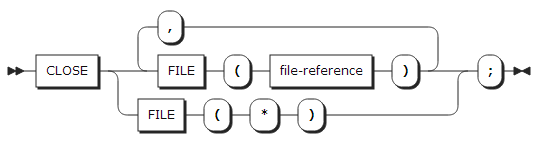
Component | Description |
---|---|
FILE |
File to close. Use an asterisk (*) to close all open files. |
6. DECLARE Statement
The DECLARE statement specifies a data item’s name and some or all attributes. If the attributes are not declared explicitly and cannot be determined by context, default attributes will be used.
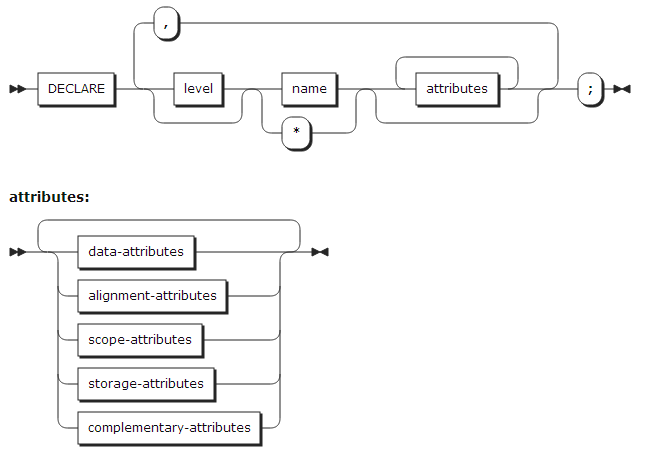
Component | Description |
---|---|
* |
Cannot be used for the names of scalar and level-1 data. |
attributes |
Data attributes in any order. For more information, refer to Data Types and Attributes. |
level |
Positive integer. The default level of scalar or array data is level-1. |
name |
Data name. A level-1 name must be unique in a block. |
Condition prefixes and labels must not be specified in a DECLARE statement. |
7. DEFAULT Statement (Abbreviation: DFT)
The DEFAULT statement defines data-attribute defaults when a set of attributes is not complete.
Attributes to which a DEFAULT statement for implicit, contextual, and partially-complete declarations are not applied are determined by language-specified defaults.
A DEFAULT statement redefines all attributes except for a name declared with the ENTRY or FILE attributes. However, the CONSTANT attribute is applied implicitly to a name without attributes that implies the VARIABLE attribute by PL/I before the DEFAULT statement is applied.

identifiers:
Component | Description |
---|---|
RANGE(identifier) |
Defaults to apply to names that start with the same letter. |
RANGE(identifier : identifier) |
Defaults to apply to names that start with one of the letters between the letters specified when using two identifiers. |
RANGE(*) |
Defaults to apply to all names in the scope of a DEFAULT statement. |
DESCRIPTORS |
Defaults to apply to attributes included in the description of a parameter in a parameter description list of an explicit Entry declaration. At least one attribute must exist. DESCRIPTORS will not be applied to NULL descriptions. |
attribute_specification |
|
Multiple DEFAULT statements can be used in a block. The scope of a DEFAULT statement is the block in which the statement is declared, all blocks within the block that do not include another DEFAULT statement with the same range, and all blocks that are not contained in a block with a DEFAULT statement with the same range.
A DEFAULT statement affects only names declared explicitly in an internal block because the scope of an implicit declaration is determined implicitly as if the names were declared implicitly using a DECLARE statement when the names immediately follows a PROCEDURE statement of an external procedure.
The following is an example:
X: PROC; LABEL1: DEFAULT RANGE(AB) FLOAT; Y: BEGIN; LABEL2: DEFAULT RANGE(ABC) FIXED; END X;
The scope of the DEFAULT statement of LABEL1 is the procedure X and begin-block Y included in the procedure. The scope specified by the DEFAULT statement of LABEL1 are all names that start with AB and are declared in the procedure X and begin-block Y. However, the names that start with ABC and declared in begin-block Y are excluded and can be used only within begin-block Y.
8. DEFINE Statement
The DEFINE statement specifies an alias for a PL/I data type or defines an ordinal data type.
8.1. DEFINE ALIAS Statement
The DEFINE ALIAS statement specifies an alias for PL/I data type.

Component | Description |
---|---|
alias-name |
Alias name. |
attributes |
Attributes that can be specified for the data type of the alias. |
The following is an example of using the DEFINE ALIAS statement to define an alias and using it to declare a variable.
DEFINE ALIAS NAME CHAR(32); DECLARE COMPANY_NAME TYPE NAME;
8.2. DEFINE ORDINAL Statement
The DEFINE ORDINAL statement defines an ordinal data type.

ordinal-value-list:
Component | Description |
---|---|
ordinal-name |
Name of the ordinal type. |
member |
Name of a member of the ordinal type. |
VALUE |
Value of a member of the ordinal type.
|
PRECISION |
Precision of VALUE. If PRECISION is omitted, PRECISION is determined by the range of VALUE. |
SIGNED, UNSIGNED |
Specifies VALUE as SIGNED or UNSIGNED. |
The following is an example of using the DEFINE ORDINAL statement to define an ordinal data type and using it to declare a variable.
DEFINE ORDINAL LANG ( PLI, COBOL, ASSEMBLER, C, CPP ); DECLARE COMPILER ORDINAL LANG;
9. DELAY Statement
The DELAY statement suspends program execution for a specified period of time.

Component | Description |
---|---|
expression |
Time to suspend. (Unit: milliseconds) |
10. DELETE Statement
The DELETE statement deletes a record from a file.

Component | Description |
---|---|
FILE |
File that includes a record to delete. The file must have the UPDATE attribute. |
KEY |
Record that corresponds with a specified KEY is removed. To use the KEY option, the file must have the DIRECT or SEQUENTIAL KEYED attributes. |
11. DISPLAY Statement
The DISPLAY statement displays messages on stdout.

Component | Description |
---|---|
expression |
If necessary, this expression is converted to a character string and the converted string will be displayed. However, GRAPHIC strings are not converted. |
12. DO Statement
The DO statement defines conditions for repeated execution on a multiple statements groups. A DO statement must be used along with an END statement. Statements between a DO statement and an END statement are grouped as a do-group.
A DO statement can be one of the following types:
-
Type 1
-
Type 2
-
Type 3
-
Type 4
Type 1
Type 1 defines a do-group and executes statements within the do-group. Type 1 does not define conditions for repeated execution.
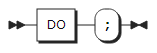
Type 2 and Type 3
Type 2 and Type 3 define a do-group and executes statements within the do-group. They can define conditions for repeated execution.
Type 2:

Type 3:
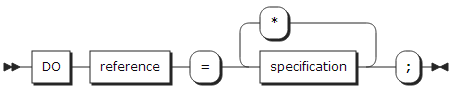
specification:
Component | Description |
---|---|
WHILE(expression) |
Evaluates an expression and converts it to a BIT string before executing a do-group. If any BIT in a string are 1, a do-group will be executed. If all BITs are 0 or a string is NULL, a do-group will not be executed. If there are multiple specifications, the next specification will be executed. |
UNTIL(expression) |
Evaluates an expression and converts it to a BIT string after executing a do-group. If all BITs in a string are 0 or the string is NULL, a do-group will be executed. If any BIT is 1, a do-group will not be executed. If there are multiple specifications, the next specification will be executed. |
reference |
The value of expression1 will be used for initialization before executing a do-group. If there are multiple specifications, each specification’s value of expression1 will be used for initialization. |
expression1 |
Initial value of a reference. If TO, BY, and REPEAT are omitted, the value of expression1 will always be used for initialization. |
TO expression2 |
The end value of a reference. If a value of a reference is not in the range of values between expression1 and expression2, a corresponding do-group will be ended. If TO expression2 is omitted, a do-group will be ended by the WHILE or UNTIL options. |
BY expression |
Increment to a reference. A reference is added by the value of an expression after executing a do-group. If TO expression2 is specified and BY expression is omitted, BY 1 will be the default value. |
UPTHRU expression |
The end value of a reference. A reference is increased by 1 and saved after executing a do-group. The TO option is compared before executing a do-group but the UPTHRU option is compared after executing a do-group. Therefore, the UPTHRU option allows a do-group to be executed at least once. |
DOWNTHRU expression |
The end value of a reference. A reference is subtracted by 1 and saved after executing a do-group. The TO option is compared before executing a do-group but the DOWNTHRU option is compared after executing a do-group. Therefore, the DOWNTHRU option allows a do-group to be executed at least once. |
REPEAT expression |
The expression is evaluated, the result value is saved to a reference, and a do-group is executed. A do-group execution is repeated until ended by the WHILE or UNTIL options. |
Type 4
Type 4 defines a do-group and executes statements within the do-group repeatedly and infinitely.
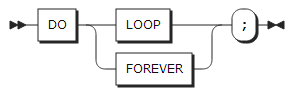
Component | Description |
---|---|
FOREVER |
Same as LOOP. |
LOOP |
Sets infinite repetition. To end the repetition, use a GOTO or LEAVE statement or end the procedure or program. |
13. END Statement
An END statement specifies the end of one or more blocks or groups. Every block or group must have an END statement.
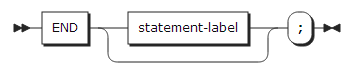
Component | Description |
---|---|
statement-label |
Label of the nearest preceding DO, SELECT, PACKAGE, BEGIN or PROCEDURE statement before the END statement to indicate the end of a block or group. If omitted, the END statement closes the nearest preceding block or group. |
14. ENTRY Statement
All non-pointer parameters of any procedures that include an ENTRY statement must be BYADDR (pass by address).

Component | Description |
---|---|
entry-label |
Additional entry point name of the procedure. |
parameter-list |
Parameters of the entry point. |
returns-options |
RETURNS option of the entry point. |
15. EXIT Statement
The EXIT statement ends a currently running PL/I program. In OpenFrame PL/I, this statement is the same as a STOP statement.
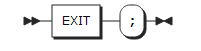
16. FETCH Statement
The FETCH statement loads a procedure or program dynamically if its entry-point is not in memory.
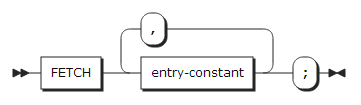
Component | Description |
---|---|
entry-constant |
Name of a procedure or program to load. |
17. FLUSH Statement
The FLUSH statement flushes the buffer of a specified file. To use a FLUSH statement for a file, the file must have the BUFFERED attribute.

Component | Description |
---|---|
FILE |
File to flush. Use an asterisk (*) to flush all open files. |
18. FORMAT Statement
The FORMAT statement specifies a format list used for an edit-directed I/O.

Component | Description |
---|---|
label |
Label to be specified by an R-format item. For more information, refer to R-format. |
format list |
Format list used for an edit-directed I/O. |
19. FREE Statement
20. GET Statement
The GET statement reads records from a stream that can be fetched from a dataset or string.
When reading data from a dataset, the syntax of the GET statement is as follows:

Component | Description |
---|---|
FILE |
Target file. (Default value: SYSIN file) |
data-specification |
Refer to Data Specification. |
COPY |
Saves a copy of the fetched stream in the specified file. If no file is specified, the copy is saved in the SYSPRINT file. |
SKIP (expression) |
Sets an expression value and skips the amount of data set, then reads the data after the skipped data. (Default value: 1) |
When reading data from a string, the syntax of the GET statement is as follows:

Component | Description |
---|---|
STRING |
Target string. |
data-specification |
Refer to Data Specification. |
21. GOTO Statement
The GOTO statement moves the program control to the position specified by a label.
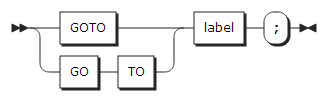
Component | Description |
---|---|
label |
Label constant or variable. If a label is a variable, the value at the point in time when the GOTO statement is executed will be referred to. |
A GOTO statement can be used to move a control to a label within a same block or an outer block. A control can also be moved from an ON-unit to an outer block.
A GOTO statement cannot be used in the following cases:
-
To an outer procedure from an inner procedure.
-
To an inner block or procedure from an outer block or procedure.
-
To a FORMAT statement.
22. IF Statement
The IF statement evaluates a condition and selects an action according to the result.

Component | Description |
---|---|
expression |
Expression that has a true or false result. Multiple conditions can be specified using '&' and '|'. |
unit |
Statements to be executed according to a condition result. A unit can be a single statement, a do-group, or a begin-block. If a condition is true, a unit described in THEN will be executed. If false, a unit described in ELSE will be executed, or the IF statement will be terminated when ELSE is not used. |
23. ITERATE Statement
The ITERATE statement skips a running do-group and executes the next do-group with an iteration condition.
An ITERATE statement must be used within a do-group.

Component | Description |
---|---|
label-constant |
Must be the label of a DO statement. If omitted, the most recent do-group with an ITERATE statement will be skipped. |
24. LEAVE Statement
The LEAVE statement ends a running do-group. This statement must be used within a do-group.
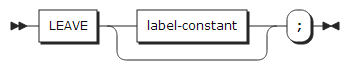
Component | Description |
---|---|
label-constant |
Must be the label of a DO statement. If omitted, the most recent do-group with a LEAVE statement will be terminated. |
25. LOCATE Statement
The LOCATE statement specifies the location of an output buffer using a pointer to enable access to the buffer through a based variable.

Component | Description |
---|---|
FILE |
Target file with a OUTPUT SEQUENTIAL BUFFERED attribute. |
based variable |
Based variable that enables access to an output buffer. |
SET |
Pointer to which the address of an output buffer is saved. |
KEYFROM |
Key of a record to write. To use the KEYFROM option, a file must have the KEYED attribute. The value of KEYFROM is converted to a CHARACTER in an indexed dataset or a FIXED DECIMAL in a relative dataset. |
A record will be written when a buffer is flushed using a FLUSH statement, or when the next LOCATE statement is executed, not when a LOCATE statement is executed. Therefore, a record to be written to a dataset can be modified using a based variable even if a LOCATE statement is executed. |
26. NULL Statement
The NULL statement executes nothing. In general, this statement is used to perform nothing when THEN, ELSE, WHEN, or OTHER is used.
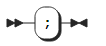
27. ON Statement
The ON statement registers an ON-unit to execute when a specified condition occurs.
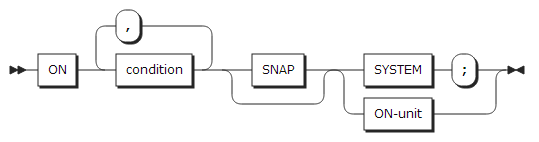
Component | Description |
---|---|
condition |
One or more conditions. For more information, refer to Conditions. |
SNAP |
Not supported. |
SYSTEM |
Default action for a condition. For more information, refer to Conditions. |
ON-unit |
Statements to execute when a condition occurs. An ON-unit can be a single statement or a begin-block. An ON-unit is executed when a corresponding condition is met, not when an ON statement is executed. |
If a specified condition occurs, an ON-unit will be executed. If a same condition is specified multiple times, the ON-unit of the last ON statement will be executed. Conditions specified using an ON statement are released when a block or procedure with the ON statement is terminated or by a user using a REVERT statement.
The following example registers the ZERODIVIDE condition:
ON ZERODIVIDE BEGIN; DISPLAY('The ZERODIVIDE condition occurs.'); END; A = B / 0;
The ZERODIVIDE condition occurs when a division by 0 is attempted. The condition occurs due to "A = B / 0", so that the DISPLAY statement is executed because an ON-unit registered through the ON statement is executed.
28. OPEN Statement
The OPEN statement opens a specified file.
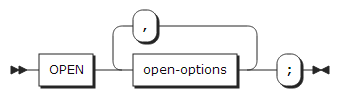
open-options:
Component | Description |
---|---|
FILE |
File to open. |
FILE attribute |
Refer to File. |
TITLE |
DD name of an external dataset described in a JCL. |
LINESIZE |
Length of a line. (Default value: 120) If a stream length exceeds LINESIZE, the next line will be used from the first column. This option can be used only for STREAM OUTPUT files. |
PAGESIZE |
Number of lines in a page. (Default value: 60) If a line exceeds PAGESIZE, the ENDFILE condition will occur. A new page can start using a PAGE format item or the PAGE option in a PUT statement. |
A file can be opened explicitly by using an OPEN statement. An unopened file opens when using a READ, WRITE, REWRITE, DELETE, LOCATE, PUT, or GET statement.
A FILE attribute that was defined during a declaration can be redefined by defining the attribute when explicitly opening a file using an OPEN statement. The following example redefines the FILE attribute using an OPEN statement.
DECLARE TEST FILE RECORD OUTPUT; OPEN FILE(TEST) STREAM INPUT; CLOSE FILE(TEST); OPEN FILE(TEST);
In the first OPEN statement, TEST has the STREAM and INPUT attributes. Once a file is closed, the FILE attribute is lost and not applied when the file is reopened. Therefore, in the second OPEN statement, TEST has the RECORD and OUTPUT attributes specified in the DECLARE statement.
If the FILE attribute defined during declaration is different from the attribute defined during opening, an error will occur. |
29. OTHERWISE Statement
For information about the OTHERWISE statement, refer to SELECT Statement.
30. PACKAGE Statement
A PACKAGE statement can only include variable declarations, DEFAULT statements, and procedure blocks. OpenFrame PL/I currently only supports procedure blocks in a PACKAGE statement and does not support PACKAGE statement options.

Component | Description |
---|---|
condition_prefix |
Condition prefix that applies to all procedures of a package. For more information, refer to the description of Condition Prefix in Conditions. |
package_name |
Package name. |
31. PUT Statement
The PUT statement creates a record of saves as a stream that can be saved to a dataset or string.
When saving data to a dataset, the syntax of the PUT statement is as follows:
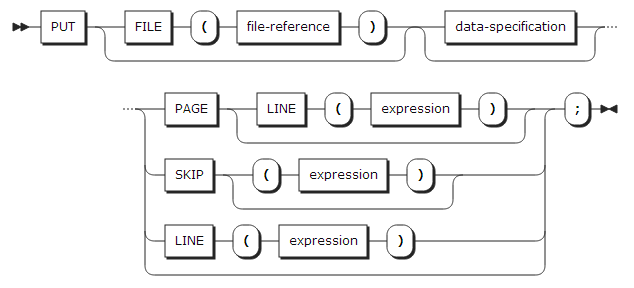
Component | Description |
---|---|
FILE |
Target file. (Default value: SYSIN file) |
data-specification |
Refer to Data Specification. |
SKIP (expression) |
Sets a number of lines to skip. (Default value: 1) |
LINE (expression) |
Sets a value of lines to move. To use the LINE option, a file must have the PRINT attribute. |
PAGE |
Starts a new page. The default start line value is 1. To use the PAGE option, a file must have the PRINT attribute. |
When saving data to a string, the syntax of the PUT statement is as follows:

Component | Description |
---|---|
STRING |
Target string. |
data-specification |
Refer to Data Specification. |
32. READ Statement
The READ statement reads records from a dataset. The read record can be saved to a variable or pointed by a pointer.
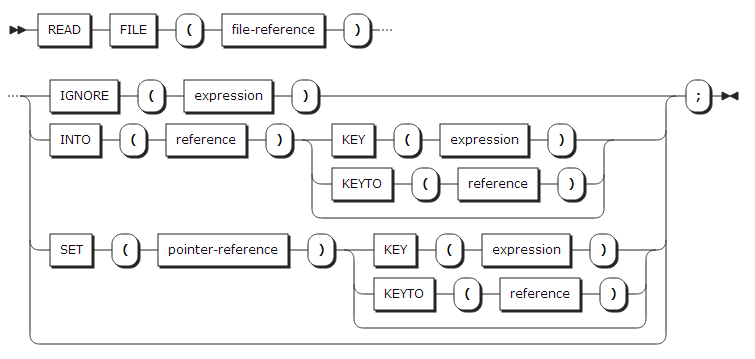
Component | Description |
---|---|
FILE |
File to read. The file must have the INPUT or UPDATE attributes. |
IGNORE |
Sets a value for the number of records to be ignored. If an evaluated expression value is n and the IGNORE option is used, the (n+1)th record will be read. |
INTO |
Saves a read record to a specified variable. If the variable is an array or a structure, it must be assigned to storage. |
SET |
Specified pointer that points to a read record. |
KEY |
Record that corresponds to a specified KEY to be read. To use the KEY option, the file must have the DIRECT or SEQUENTIAL KEYED attribute. |
KEYTO |
Reads a record’s key value when a record is read. To use the KEYTO option, a file must have the SEQUENTIAL KEYED attribute. |
33. RETURN Statement
A RETURN statement terminates the execution of a procedure and the control is returned to the calling code.
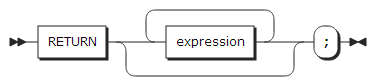
-
A RETURN statement with an expression cannot be used within a procedure with OPTIONS(MAIN).
-
A RETURN statement without an expression cannot be used within a procedure with RETURNS options.
-
A procedure with RETURNS options must have one or more RETURN statements.
34. REVERT Statement
The REVERT statement unregisters an ON-unit that was registered using an ON statement.
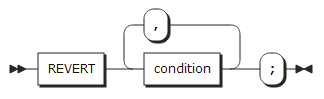
Component | Description |
---|---|
condition |
One or more conditions. For more information, refer to Conditions. |
A REVERT statement only unregisters an ON-unit registered in a current block or procedure and cannot unregister an ON-unit registered in an upper block or procedure. If there is no ON-unit registered in a current block or procedure, a REVERT statement will not be executed.
The following example unregisters an ON-unit by using a REVERT statement:
ON ZERODIVIDE BEGIN; DISPLAY('ZERODIVIDE condition raised'); END; REVERT ZERODIVIDE; A = B / 0;
The ZERODIVIDE condition occurs due to "A = B / 0;" but the ON-unit registered using the ON statement is not executed because it has been unregistered by using the REVERT statement. If there is no registered ON-unit, a default action is executed according to a condition; either the ERROR condition occurs or the program execution continues. The ZERODIVIDE condition set an ERROR condition as the default action.
35. REWRITE Statement
The REWRITE statement updates a record in a dataset.
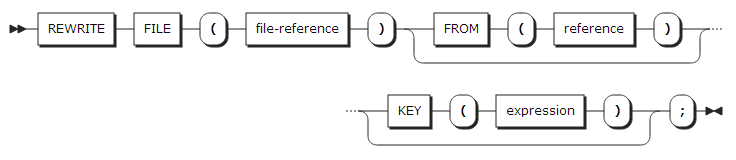
Component | Description |
---|---|
FILE |
File to update. The file must have the UPDATE attribute. |
FROM |
Variable to which a record is saved. If the variable is an array or a structure, it must be assigned to storage. |
KEY |
Record that corresponds to a specified KEY is updated. To use the KEY option, the file must have the DIRECT or SEQUENTIAL KEYED attributes. |
36. SELECT Statement
The SELECT statement evaluates a condition and then selects a branch according to the result from multiple branches.
A SELECT statement can include one or more WHEN statements and either zero or one OTHERWISE statements. A SELECT statement must be paired with an END statement.

Component | Description |
---|---|
SELECT (expression) |
Expression to be evaluated and saved. |
WHEN (expression) unit |
There can be one or more expressions. To specify multiple expressions, separate each expression with '&' or '|' as delimiter. Each expression is evaluated and compared with the expression value of a SELECT statement. If any of the expression values are the same as the expression value of a SELECT statement, the unit is executed. |
OTHERWISE unit |
If all the expression values in a WHEN statement are different from the expression value of a SELECT statement, the OTHERWISE statement will be executed. If there is no OTHERWISE statement, the SELECT statement will terminate. |
unit |
Statements to be executed if the WHEN or OTHERWISE statement is met. A unit can be a single statement, a do-group, or a begin-block. |
37. SIGNAL Statement
The SIGNAL statement causes a condition to enable a user to execute an ON-unit.
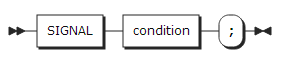
Component | Description |
---|---|
condition |
Condition. For more information, refer to Conditions. |
If an ON-unit is registered by a user, the ON-unit will be executed. If there are none, a default action specified internally will be executed.
In the following example, an ON-unit using a SIGNAL statement has been executed:
ON ZERODIVIDE BEGIN; DISPLAY('ZERODIVIDE condition occurs.'); END; SIGNAL ZERODIVIDE;
The ON-unit of the ZERODIVIDE condition is executed because of the SIGNAL statement. Even though a condition related to I/O has occurred, the corresponding ON-unit is not executed if the relevant files are different.
38. STOP statement
The STOP statement ends a currently running PL/I program. In OpenFrame PL/I, this statement is the same as an EXIT statement.
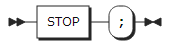
39. WHEN Statement
For information about the WHEN statement, refer to SELECT Statement.
40. WRITE Statement
The WRITE statement writes a record to a dataset.
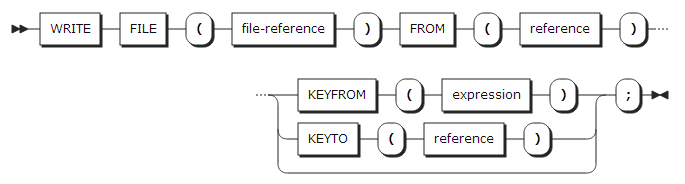
Component | Description |
---|---|
FILE |
File to write. The file must have the OUTPUT or UPDATE attributes. |
FROM |
Variable to which a record is written. If the variable is an array or a structure, it must be assigned to storage. |
KEYFROM |
Key of a record to write. To use the KEYFROM option, a file must have the KEYED attribute. The value of KEYFROM is converted to a CHARACTER in an indexed dataset, or a FIXED DECIMAL in a relative dataset. |
KEYTO |
Key of the next record to write. To use the KEYTO option, a file must have the SEQUENTIAL KEYED attribute. |