Introduction to WBAPI
1. Overview
WebtoB provides a new, unique API to solve the challenges of existing CGI application programs.
The traditional CGI method initiates a new process for each user request, with the operating system creating (or "forking") a separate process to handle each incoming request in real time. This forking places a load on the system; frequent requests can create a heavy processing burden, potentially impacting overall system performance.
Because of these issues, existing CGI users gradually turned to new applications, such as Servlet, PHP, ASP, and JSP.
These programs are not written in C; instead, they are new types based on Java or scripting languages. While these may simplify new development, they impose challenges for those who have developed and maintained CGI programs in the traditional C style, requiring them to discard existing programs and create replacements.
CGI developers face a dilemma: whether to abandon their existing CGI setup in favor of an entirely new program or to continue using the current CGI program while increasing server capacity to reduce the load. WebtoB has developed a new API to address these concerns and ease the transition for CGI developers.
The provided API is designed for C-style programs, which reduces the load on CGI applications while minimizing the need for modifications to existing CGI programs. This alleviates the burden on many users who rely on legacy CGI-based services and enhances overall performance.
1.1. Concept
As mentioned above, the CGI method involves forking a new process for each user request and terminating it once processing is complete. This imposes a significant load on the system, as it must repeatedly perform tasks like loading new processes into memory and scheduling them—often at the expense of other, more critical jobs that need to be handled during that time.
The figure below shows how to use CGI on an Apache web server.
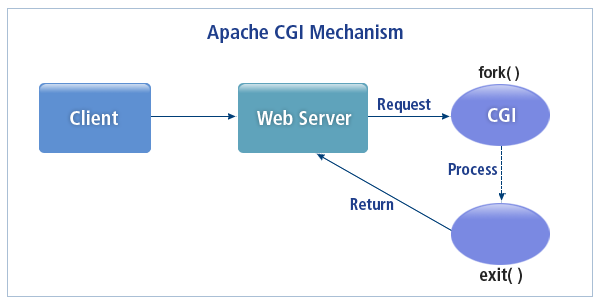
As shown in the previous diagram, each time the web server sends a request, it triggers a load due to the forking of a new CGI process. WebtoB’s WBAPI addresses this issue by pre-forking these processes and loading them into memory when the web server starts. Instead of exiting once a request is processed, these pre-forked programs stay in memory, ready to handle subsequent requests. This approach reduces the overhead associated with forking and exiting processes for each request.
In addition to simply reducing this type of load, WebtoB manages programs developed with WBAPI. If a program crashes or fails due to user error or design issues, a new feature allows for immediate re-execution of the program. WebtoB also adjusts the number of active programs; if it detects an increased demand for a specific program, it preemptively runs additional instances to alleviate the load.
The call to WebtoB’s WBAPI is shown in the figure below.
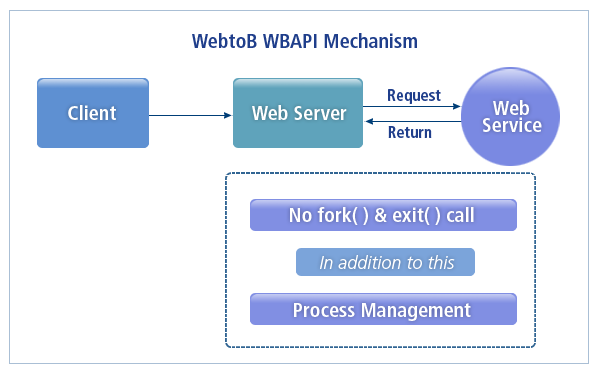
WBAPI differs from traditional functions that typically begin with main(). Programs written with WBAPI are generated as independent processes; however, they operate as processes integrated within WebtoB. Consequently, these functions start with a Service Name rather than the conventional main(). Therefore, the function name must be designated as Service Name and must be registered in the WebtoB configuration file. For more information, refer to Configuration, which will be explained later.
2. Configuration
To use WBAPI in WebtoB, configuration must first be completed alongside the programming. It’s essentially like declaring that you are developing an application using WBAPI within WebtoB, specifying how this application will be utilized.
The following is a sample configuration for WebtoB. The items written in bold indicate the basic settings required for using WBAPI.
*DOMAIN webtob1 *NODE webmain WebtoBDir = "/usr/local/webtob", SHMKEY = 99000, Docroot = "/usr/local/webtob/docs", AppDir = "/usr/local/webtob/ap", Hostname = "webmain.tmax.co.kr", Port = "8080", JsvPort = 9099 *SVRGROUP htmlg NodeName = webmain, SvrType = HTML cgig NodeName = webmain, SvrType = CGI jsvg NodeName = webmain, SvrType = JSV ssig NodeName = webmain, SvrType = SSI wbapg NodeName = webmain, SvrType = WEBSTD *SERVER html SvgName = htmlg, MinProc = 10, MaxProc = 10 cgi SvgName = cgig, MinProc = 10, MaxProc = 10 ssi SvgName = ssig, MinProc = 2, MaxProc =2 jsv1 SvgName = jsvg, MinProc = 10, MAXProc = 10 webaps SvgName = wbapg, MinProc = 5, MAXProc = 5 *SERVICE write_board SvrName = webaps *URI uri1 Uri ="/svct/", SvrType = WEBSTD, SvrName = webaps
-
SVRGROUP section
Set up a server group called wbapg on a Host Machine named webmain, and set this to a server type called WEBSTD to use WBAPI.
wbapg NodeName = webmain, SvrType = WEBSTD
-
SERVER section
Indicates that the wbapg set in the SVRGROUP section is defined as a server called webaps.
webaps SvgName = wbapg, MinProc = 5, MaxProc = 5
The SVRGROUP and SERVER sections are the minimum required settings that must always be configured. This is true in most environments, with the exception of the Host Machine name, which is the only difference. To implement actual services, additional settings specific to each service are required.
-
SERVICE section
Register a program developed with WBAPI. The function name of the program matches the actual program name; however, unlike general C-style programs, WBAPI programs start with write_board(). In other words, this function name becomes the service name. Use this name when registering the program and add the SERVICE section after the SERVER section.
*SERVICE write_board SvrName = webaps
-
URI section
When using a service created with WBAPI, set the URI section. This means that if the request starts with a specific URI, that is, a URI called "/svct/", it will be executed as a WBAPI service.
*URI uri1 Uri =”/svct/”, SvrType = WEBSTD, SvrName = webaps
3. Creating a service table
Normally, to start WebtoB, you only need to compile it using wscfl. However, to use WBAPI, you need to compile it using wscfl and then create a service table using the wsgst command, located in the bin/
directory.
When you run wsgst, WebtoB creates a service table with the extension _svctab.c for writing WBAPI in the specified path.
wsgst
The following describes how to use the service table using the wsgst command and its options.
$ wsgst [ -f binary WebtoB config file name ]
Options | explanation |
---|---|
[ -f binary WebtoB config file name ] |
Specify this name if the configuration file to reference for creating the service table is not wsconfig. |
Here is an example using wsgst:
$ wsgst ( References wsconfig by default) $ wsgst -f wsconfig2 ( if using wscfl -o )
Now, we are ready to use WBAPI. Now, compile the program developed with WBAPI to create an executable file and register it in the format above.
4. Compiling the program
Programs developed with WBAPI must be linked when compiling because they use special libraries and service table files provided by WebtoB in addition to the libraries provided by default in C.
The following is an example of a Makefile file for compilation on UNIX/Linux.
#Makefile.common TARGET= webaps APOBJS= ap.o #hp 32bit CFLAGS = -Ae +DA1.1 +DD32 -O -I$(WEBTOBDIR) #hp 64bit #CFLAGS = -Ae +DA2.0W +DD64 +DS2.0 -O -I$(WEBTOBDIR) #sun 32bit #CFLAGS = -O -I$(WEBTOBDIR) #sun 64bit #CFLAGS = -xarch=v9 -O -I$(WEBTOBDIR) #Linux #CFLAGS = -O -I$(WEBTOBDIR) #ibm 32bit #CFLAGS = -q32 -O -I$(WEBTOBDIR) -brtl #ibm 64bit #CFLAGS = -q64 -O -I$(WEBTOBDIR) -brtl WEBTOB_INCDIR = $(WEBTOBDIR)/usrinc WEBTOB_BINDIR = $(WEBTOBDIR)/bin WEBTOB_LIBDIR = $(WEBTOBDIR)/lib OBJS = $(APOBJS) $(SVCTOBJ) SVCTOBJ = $(TARGET)_svctab.o .SUFFIXES : .v .c.o: $(CC) $(CFLAGS) -c $< wbaps: $(APOBJS) svct $(CC) $(CFLAGS) -L$(WEBTOB_LIBDIR) -o $(TARGET) $(OBJS) $(LIBS) $(USERLIBS) svct: cp $(WEBTOBDIR)/svct/$(TARGET)_svctab.c . $(CC) $(CFLAGS) -I$(WEBTOB_INCDIR) –c $(TARGET)_svctab.c
The following is for Windows.
#Makefile for Windows TARGET = webaps.exe LIBS = /link $(WEBTOBDIR)\lib\aps.lib ws2_32.lib CFLAGS = /D _DEBUG /I $(WEBTOBDIR) /Gd /MD /nologo APOBJ = $(TARGET:.exe=.obj) SVCTSRC = $(TARGET:.exe=_svctab.c) SVCTOBJ = $(TARGET:.exe=_svctab.obj) OBJS = $(APOBJ) $(SVCTOBJ) $(TARGET): svct $(OBJS) cl $(CFLAGS) -o $@ $(OBJS) $(LIBS) copy $@ $(WEBTOBDIR)\ap svct: copy $(WEBTOBDIR)\svct\$(SVCTSRC) clean: -del $(OBJS) $(TARGET)
5. Getting started and applying WBAPI
When the task is completed, the program developed with WBAPI is executed alongside WebtoB and loaded into memory as a process.
CGI conversion is one of the many features of WBAPI. In addition to converting existing CGI programs, developers proficient in C-style programming can use WBAPI to create new applications, greatly enhancing development convenience and performance. WebtoB will continue to provide and improve WBAPI, ensuring compatibility with future expansions and ongoing support.
WBAPI also supports the use of existing services provided by TP-monitors.
6. Types of WBAPI
WBAPI consists of the INIT/DONE, ALLOC, GET, PUT/SET, SEND, COOKIE, SESSION, and ETC series. Each API is categorized by function, with a brief description of each provided below.
6.1. INIT/DONE API
The INIT/DONE series of functions handle the initialization tasks required before starting the service, as well as routines that should be executed when the service process ends.
The following is a list of INIT/DONE family functions.
Function name | Description |
---|---|
wbSvrInit() |
Initializes the server. |
wbSvrDone() |
Notifies the return of WebtoB. |
6.2. ALLOC API
The ALLOC series of functions allows users to allocate and free memory.
The following is a list of ALLOC family functions.
Function name | Description |
---|---|
wbMalloc() |
Allocates memory equal to the SIZE set by the user. |
wbFree() |
Frees memory allocated by wbMalloc(). |
6.3. GET API
The GET series of functions reads the user’s information about the user requests, and gets the user’s request data, method information value, etc.
The following is a list of GET family functions.
Function name | Description |
---|---|
wbGetAuthType() |
Gets the user’s authentication type. |
wbGetContentLength() |
Gets the size of the content sent by the client. |
wbGetDocumentRoot() |
Gets WebtoB’s home directory value. |
wbGetHdr() |
Gets the value corresponding to a specific key in the request header. |
wbGetDateHdr() |
Get the header representing the data. |
wbGetIntHdr() |
Gets the specified header value as an integer. |
wbGetNthHdr() |
Gets the header value corresponding to the `N`th element in the header. |
wbGetHdrCount() |
Gets the total number of headers in the request sent by the user. |
wbGetData() |
Gets the value corresponding to the key in the data field of the request. |
wbGetNthKey() |
Gets data for the 'N’th key in the request. |
wbGetNthData() |
Gets the 'N’th data from the request data. |
wbGetDataCount() |
Gets the number of data input from the request. |
wbGetValue() |
Gets the 'N’th data with a specific key value in the request. |
wbKeyOccur() |
Gets the number of key values from the data received through the request. |
wbGetMethod() |
Reads the HTTP Method received in the request as an integer. |
wbGetParsedURI() |
Gets the URI information from which a request was received from the client. |
wbGetPathInfo() |
Gets relative path information related to the request. |
wbGetPathTranslated() |
Gets absolute path information related to the request. |
wbGetQueryString() |
Gets a query string from the request URL. |
wbGetProtocol() |
Gets protocol information related to the request. |
wbGetRemoteUser() |
Gets the name of the requesting user. |
wbGetRequestURI() |
Gets the Request URI. |
wbGetRemoteAddr() |
Gets the IP of the requested remote host. |
wbGetRemoteHost() |
Gets the name of the requesting remote host. |
wbGetRemoteIdent() |
Gets the name of the user retrieved from the server. |
wbGetReqLine() |
Gets the first line pointer of the line that came in the request. |
wbGetFileName() |
Gets the name of the file. |
wbGetFileLen() |
Gets the size of the file. |
wbGetScheme() |
Gets protocol information for the requested service. |
wbGetScriptFileName() |
Gets the absolute path where the requested WBAPI service is running. |
wbGetScriptName() |
Gets the path where the requested service is executed. |
wbGetServerName() |
Gets the server’s hostname. |
wbGetServerPort() |
Gets the server’s port number. |
wbGetServerSoftware() |
Gets software information from the server. |
wbGetTranslatedURI() |
Interprets the URI of the requested service to get the actual path. |
wbGetRequestURL() |
Gets the request URL value. |
6.4. PUT/SET API
The PUT/SET series of functions are used to output values processed by WBAPI in response to a user’s request. For developers familiar with CGI, this is similar to using the printf function in C or the print statement in Perl to display output values.
In WBAPI, after executing the output script, the result is saved in a structure called WBSVCINFO, which is then sent to the browser. These functions store the data that will be returned as a response within the WBSVCINFO structure.
When using the PUT series of functions, keep in mind that the order of PUT-related function calls must be followed to ensure WBAPI executes correctly.
-
One function must be executed before other PUT family functions: wbSetStatus(WBSVCINFO *rqst, int status, char *status_msg). This function writes the response header and must be executed first.
-
Among the PUT-related functions, those that write headers must be declared before functions that write data fields.
The following is a list of PUT/SET family functions.
Function name | Description |
---|---|
wbPutHdr() |
Sets the response header. |
wbPutIntHdr() |
Adds the response header. |
wbSetStatus() |
Sets the status value of the request. |
wbPutStr() |
Prints a string value. |
wbPut() |
Outputs data of a specific size. |
wbPrint() |
Prints user-specified content. |
wbPutFile() |
Used for file downloads, this function reads a specific file and sends it all to the browser. |
wbPutPartialFile() |
Used for file downloads, this function reads a specified portion of a file and sends it to the browser. It reads from a defined offset up to the specified size and transmits this data. If the size is set to 0, the function reads from the offset to the end of the file. |
6.5. SEND API
The SEND series of functions return the service processing result stored in the WBSVCINFO structure to the browser using the PUT series function in response to the user’s request.
The following is a list of SEND family functions.
Function name | Description |
---|---|
wbFlush() |
Causes everything currently in the buffer to be written. |
wbSendError() |
Checks whether there is an error in the status code. |
wbSendRedirect() |
Returns a response to the specified address. |
wbReturn() |
Notifies the return of WebtoB. |
6.6. COOKIE API
The COOKIE series of functions perform operations to create, read, and process HTTP-style cookies.
The following is a list of COOKIE family functions.
Function name | Description |
---|---|
wbCreateCookie () |
Creates a new cookie. |
wbGetCookie() |
Returns the desired cookie from among the cookies sent by the browser. |
wbPutCookie() |
Appends a response to the specified cookie. |
wbCookieGetDomain () |
Returns the domain from the specified cookie. |
wbCookieGetName() |
Returns the name of the specified cookie. |
wbCookieGetPath() |
Returns the path to the cookie. |
wbCookieGetValue() |
Returns the value of the cookie. |
wbCookieGetVersion() |
Returns the version of the cookie. |
wbCookieSetComment() |
Sets the comment field of a cookie. |
wbCookieSetDomain() |
Set the domain of the cookie. |
wbCookieSetMaxAge() |
Sets the cookie’s lifetime. |
wbCookieSetPath() |
Sets the path for cookies. |
wbCookieSetSecure() |
Sets whether cookies should be transmitted over a secure protocol such as SSL. |
wbCookieSetValue() |
Sets a new value for a cookie. |
wbCookieSetVersion() |
Sets a new version for cookie. |
6.7. SESSION API
The SESSION series of functions create a session, obtain unique information about the session to perform other various tasks.
The following is a list of SESSION family functions.
Function name | Description |
---|---|
wbGetRequestedSessionId() |
Obtains a session ID that is made clear by request from the user. |
wbGetSession() |
If no session has been created, create a session and get its session values. |
wbIsRequestedSessionIdValid() |
Returns true if the requested session is a valid session and is currently in use. |
wbSessionGetId() |
Gets the unique ID assigned to the session. |
wbSessionGetValueNames() |
Gets an array containing the names of all objects bound to the session. |
wbSessionGetCreationTime() |
Gets the time the session was created. |
wbSessionGetValue() |
Gets the value and name of an object bound to the session. |
wbSessionSetValue() |
Binds the value and name of an object specified in the session. |
wbSessionIsNew() |
Gets a value indicating whether the session is new or not. |
wbSessionRemoveValue() |
Removes the object bound to the specified name. |
WbSessionInvalidate() |
Invalidates the session. |
wbSessionGetMaxInactiveInterval() |
Gets the session retention time set for the session. |
wbSessionSetMaxInactiveInterval() |
Sets the session retention time for a specified session. |
wbSessionGetLastAccessTime() |
Gets the time when the client last sent a request. |