User Class/Handler Editor
This chapter describes the functions and uses of the User Class/Handler editor.
1. User Class
A user class is used to define logic that is too complex to be expressed as a flow condition.
1.1. Creating a User Class
To create a user class, select [New] > [User Class] from the context menu of the Project Navigator. Enter the required items in the Create User Class window, and then click [Finish].
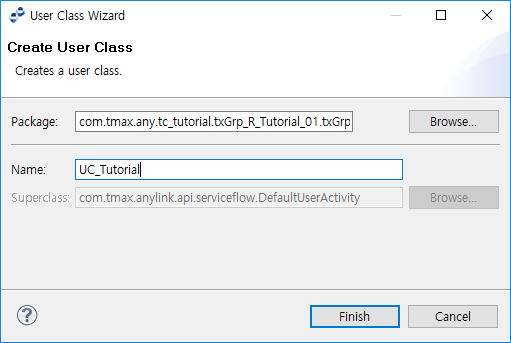
Item | Description |
---|---|
Package |
Package of the user class. |
Name |
User class name (follow the Java class naming convention). |
Superclass |
Superclass of the user class. (Default value: DefaultUserActivity) |
1.2. User Class Implementation
Once a user class is created, enter the class implementation in the editor.
A user class is implemented like a Java class, and it inherits the DefaultUserActivity class and overrides the action method.
package com.tmax.tc_tutorial.txGrp_R_tutorial.tx_L_A_tutorial; import com.tmax.anylink.api.serviceflow.ActivityContext; import com.tmax.anylink.api.serviceflow.DefaultUserActivity; import com.tmax.anylink.common.AnyLinkException; import com.tmax.anylink.logging.Logger; public class UC_Tutorial extends DefaultUserActivity { private static final Logger logger = Logger.getLogger(UC_Tutorial.class.getName()); public void action(ActivityContext arg0) throws AnyLinkException { // TODO Auto-generated method stub } }
1.3. getEnv Function
The getEnv function is used for a user class or handler to get system and BizTx information.
The following describes keys that are available in the function and values that can be obtained with the keys.
Key | Value Description |
---|---|
install.root |
Root path where AnyLink is installed. |
domain.home |
Domain home path. |
server.home |
Server home path. |
server.name |
Server name. |
adminServer.name |
adminServer name. |
cluster.name |
Cluster name. |
bizsystem.id |
Biz system ID. |
hostname |
hostname. |
biztx.id |
BizTx ID. |
guid |
GUID. |
startendpoint.id |
ID of an endpoint with started BizTx. |
startadapter.id |
ID of an adapter with started BizTx. |
startendpoint.sysid |
SysID of an endpoint with started BizTx. |
startendpoint.connection.count |
Connection count of an endpoint with started BizTx. |
request.ip |
IP address of a counterpart that requests BizTx, or job ID if a job schedule is used. |
request.port |
Port number of a counterpart that requests BizTx. |
request.url |
URL that requests BizTx. |
request.http.cookie |
Cookie set in the HTTP request through which BizTx is executed. |
connection.guid |
Connection GUID. |
biztx.name |
BizTx name. |
biztx.code |
BizTx code. |
biztx.type |
BizTx type. |
correlation.value |
Correlation value. Available for mapping handlers and mapping activities. |
local.ip |
Local IP address. |
local.port |
Local port number. |
connect.type |
Connection type. |
${sys:variable_name} |
System variable set in WebAdmin. |
2. Handler
2.1. Creating a Handler
To create a handler, select [New] > [Handler] and select the desired handler type from the context menu of the Project Navigator. Enter the required items in the Create Handler window, and then click [Finish].
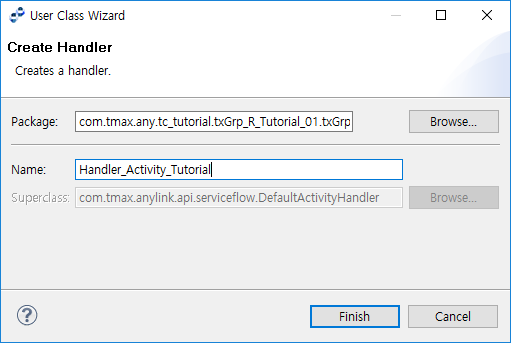
Item | Description |
---|---|
Package |
Package of the handler class. |
Name |
Handler class name (follow Java class naming convention). |
Superclass |
Superclass of the handler class. |
2.2. Handler Class Implementation
Once a handler is created, enter the class implementation in the editor. A handler class is implemented like a Java class, and it inherits its superclass.
The following are the handlers provided in AnyLink.
Handler Class Type | Super Class | Description |
---|---|---|
DefaultActivityHandler |
Defines Activity’s operation at a specific time, e.g., start or end time. |
|
DefaultActivityErrorHandler |
Defines the action to perform when an error occurs in the Activity. |
|
DefaultProcessHandler |
Defines the operation to perform at a specified time, such as the process start or end time. |
|
DefaultProcessErrorHandler |
Defines the action to perform when a process error occurs. |
|
DefaultAdapterMessageHandler |
Performs preprocessing before receiving a message or sending the message to an endpoint. |
|
DefaultEncryptionHandler |
Defines encryption method for logging. |
|
DefaultUserMappingHandler |
Defines complex mapping that is not supported in the Mapping window. |
|
DefaultParsingHandler |
Parses messages. |
|
DefaultUserTimer |
Defines additional Timer event configuration. |
|
DefaultMessageValidityHandler |
Defines message validation logic. |
|
DefaultRoutingHandler |
Implements logic for multi-binding. |
|
DefaultSsoLoginHandler |
Implements logic for SSO login. |
|
DefaultMonitoringEventHandler |
Implements logic for processing events occurred during monitoring. |
|
DefaultMsgTransferHandler |
Implements logic for sharing messages. |
|
DefaultPartnerAddressHandler |
Implements logic to call by using Administrator Info set in External Contacts. |
Activity Handler
Activity handler is called when an Activity is started, finished, cancelled, or finished handling an error.
Handler | Description |
---|---|
started |
Called when Activity starts. |
finished |
Called when Activity is finished. |
cancelled |
Called when Activity is cancelled. |
errorOccurred |
Called when Activity is finished handling an error. |
The following handler logs the Activity ID when an Activity is started, finished, cancelled, or finished handling an error.
package com.tmax.pkg.handlerCase1; import com.tmax.anylink.api.serviceflow.ActivityContext; import com.tmax.anylink.api.serviceflow.DefaultActivityHandler; import com.tmax.anylink.common.AnyLinkException; import com.tmax.anylink.logging.Logger; public class ActivityHandlerImpl extends DefaultActivityHandler { private static Logger logger = Logger.getLogger(ActivityHandlerImpl.class.getName()); @Override public void started(ActivityContext paramActivityContext) throws AnyLinkException { // TODO Auto-generated method stub logger.info("==== Activity Started : " + paramActivityContext.getActivityId() ); } @Override public void finished(ActivityContext paramActivityContext) throws AnyLinkException { // TODO Auto-generated method stub logger.info("==== Activity Finished : " + paramActivityContext.getActivityId() ); } @Override public void cancelled(ActivityContext paramActivityContext, String paramString) throws AnyLinkException { // TODO Auto-generated method stub logger.info("==== Activity Canceled : " + paramActivityContext.getActivityId() ); } @Override public void errorOccurred(ActivityContext paramActivityContext, Throwable paramThrowable) throws AnyLinkException { // TODO Auto-generated method stub logger.info("==== Acitivity Error Occured : " + paramActivityContext.getActivityId() ); } }
After implementing the handler, click [Add…] from the Flow Activity’s [Activity Preferences] > Activity Handler page to register the handler.
Activity Error Handler
Activity Error Handler is called when an error occurs while executing an Activity.
The following handler logs the Activity ID and error message when an error occurs while executing an Activity.
package com.tmax.pkg.handlerCase1; import com.tmax.anylink.api.serviceflow.ActivityContext; import com.tmax.anylink.api.serviceflow.DefaultActivityErrorHandler; import com.tmax.anylink.common.AnyLinkException; import com.tmax.anylink.logging.Logger; public class ActivityErrorHandlerImpl extends DefaultActivityErrorHandler { private static Logger logger = Logger.getLogger(ActivityErrorHandlerImpl.class.getName()); @Override public void handle(ActivityContext paramActivityContext, Throwable paramThrowable) throws AnyLinkException { // TODO Auto-generated method stub logger.info("==== Activity ID : "+paramActivityContext.getActivityId()); logger.info("==== Error Message : "+paramThrowable.getMessage()); }
After implementing the handler, click [Add…] from the Flow Activity’s [Activity Preferences] > Activity Handler page to register the handler with the error code so that the handler is invoked when an error with the specified error code occurs.
Process Handler
Process Handler is called when a Service Flow starts or ends.
The following handler logs the Flow ID when the flow starts or ends.
package com.tmax.pkg.handlerCase1; import com.tmax.anylink.api.serviceflow.DefaultProcessHandler; import com.tmax.anylink.api.serviceflow.ProcessContext; import com.tmax.anylink.common.AnyLinkException; import com.tmax.anylink.logging.Logger; public class ProcessHandlerImpl extends DefaultProcessHandler { private Logger logger = Logger.getLogger(ProcessHandlerImpl.class.getName()); @Override public void finished(ProcessContext ctx) throws AnyLinkException { // TODO Auto-generated method stub super.finished(ctx); logger.info("==== Flow Finished : " + ctx.getProcessId()); } @Override public void started(ProcessContext ctx) throws AnyLinkException { // TODO Auto-generated method stub super.started(ctx); logger.info("==== Flow Started : " + ctx.getProcessId()); } }
After implementing the handler, click [Properties] from the context menu of the editor and then click [Add…] from the [Process Handler] tab to register the handler.
Process Error Handler
Process Error Handler is called when an error occurs while executing a Flow.
The following handler logs the Flow ID and error message when an error occurs while executing a Flow.
package com.tmax.pkg.handlerCase1; import com.tmax.anylink.api.serviceflow.DefaultProcessErrorHandler; import com.tmax.anylink.api.serviceflow.ProcessContext; import com.tmax.anylink.common.AnyLinkException; import com.tmax.anylink.logging.Logger; public class ProcessErrorHandlerImpl extends DefaultProcessErrorHandler { private static Logger logger = Logger.getLogger(ProcessHandlerImpl.class.getName()); @Override public void handle(ProcessContext context, Throwable error) throws AnyLinkException { // TODO Auto-generated method stub logger.info("==== Flow ID : " + context.getProcessId()); logger.info("==== Error Message : " + error.getMessage()); }
After implementing the handler, click [Properties] from the context menu of the editor and then click [Add…] from the [Process Handler] tab to register the handler with the error code so that the handler is invoked when an error with the specified error code occurs.
Adapter Message Handler
Adapter Message Handler transforms messages that are received and sent through an endpoint. The receive method transforms incoming messages and the send method transforms outgoing messages.
The following handler changes the input message to all lower cases and the output message to all upper cases.
package com.tmax.pkg.adapterMSGCase1.tx1; import com.tmax.anylink.api.adapter.DefaultAdapterMessageHandler; import com.tmax.anylink.common.AnyLinkException; import com.tmax.anylink.logging.Logger; public class AdapterMessageHandlerImpl extends DefaultAdapterMessageHandler { private static Logger logger = Logger.getLogger(AdapterMessageHandlerImpl.class.getName()); @Override public Object receive(Object arg0) throws AnyLinkException { // TODO Auto-generated method stub String receiveMsg = new String((byte[]) arg0); logger.info("==== RECEIVE MSG : " + receiveMsg ); return receiveMsg.toLowerCase().getBytes(); } @Override public Object send(Object arg0) throws AnyLinkException { // TODO Auto-generated method stub String sendMsg = new String((byte[]) arg0); logger.info("==== SEND MSG : " + sendMsg ); return sendMsg.toUpperCase().getBytes(); } }
After implementing the handler, deploy the BizTx that includes the handler. In WebAdmin, register the handler as the 'Message Handler' in the [Advanced Settings] tab of the Endpoint settings page.
Encryption Handler
Encryption Handler encrypts or decrypts a message when saving or retrieving a trace or custom log. The decrypt method decrypts and the encrypt method encrypts a message.
The following handler uses BASE64 encoding method for encrypting or decrypting a message.
package com.tmax.pkg.encryptionCase1.tx1; import com.tmax.anylink.api.log.DefaultEncryptionHandler; import com.tmax.anylink.common.AnyLinkException; import com.tmax.anylink.common.util.Base64; import com.tmax.anylink.logging.Logger; public class EncryptionHandlerImpl extends DefaultEncryptionHandler { private static Logger logger = Logger.getLogger(EncryptionHandlerImpl.class.getName()); @Override public byte[] decrypt(byte[] arg0) throws AnyLinkException { // TODO Auto-generated method stub logger.info("==== Encrypted MSG : " + new String(arg0) ); Base64 decoder = new Base64(); byte[] decryptedMessage = decoder.decode(new String(arg0)); return decryptedMessage; } @Override public byte[] encrypt(byte[] arg0) throws AnyLinkException { // TODO Auto-generated method stub logger.info("==== Original MSG : " + new String(arg0) ); Base64 eecoder = new Base64(); byte[] encryptedMessage = encrypt(arg0); return encryptedMessage; } }
After implementing the handler, select the 'Use Encryption/Decryption' option in Custom Log Outbound Rule and select the handler in 'Select User Class' option. After deploying the BizTx that includes the handler, go to WebAdmin and register the handler with the package name as the 'Encryption User Class Name' in the [Trace Log] tab of the Log Adapter settings page.
User Mapping Handler
User Mapping Handler is used to implement custom mapping that is usually created from the Mapping dialog box. The Source is passed in as arg1 and the Target is returned as Object[].
The following handler performs one-to-one mapping of input and output sequentially.
package com.tmax.pkg.handlerCase1; import com.tmax.anylink.api.serviceflow.ActivityContext; import com.tmax.anylink.api.serviceflow.DefaultUserMapping; import com.tmax.anylink.common.AnyLinkException; public class UserMappingHandlerImpl extends DefaultUserMapping { @Override public Object[] mapping(ActivityContext arg0, Object[] arg1) throws AnyLinkException { // TODO Auto-generated method stub Object[] mappedTarget = new Object[arg1.length]; for(int i = 0; i < arg1.length; i++){ mappedTarget[i] = arg1[i]; } return mappedTarget; } }
After implementing the handler, click [Add User Class] from the Mapping dialog box to register the handler.
Parsing Handler
Parsing Handler can be used when the 'Child BizTx Identification Method' under Parsing Info for a [BizTx Group] is set to 'HANDLER'. The handler’s return value, BizTx Identifier Code, is used to find the BizTx for parsing.
The following handler that returns the first four characters of the request message as the BizTx Identifier Code.
package com.tmax.pkg.handlerCase1; import com.tmax.anylink.api.adapter.DefaultParsingHandler; import com.tmax.anylink.common.AnyLinkException; import com.tmax.anylink.logging.Logger; public class ParsingHandlerImpl extends DefaultParsingHandler { private static Logger logger = Logger.getLogger(ParsingHandlerImpl.class.getName()); @Override public String parsing(Object arg0) throws AnyLinkException { // TODO Auto-generated method stub String inputMsg = new String((byte[]) arg0); logger.info("====Input Message : " + inputMsg); String parsingMsg = inputMsg.substring(0, 3); logger.info("====Parsing Message : " + parsingMsg); return parsingMsg; } }
After implementing the handler, set the 'Child BizTx Identification Method' under Parsing Info in the BizTx Group Editor to 'HANDLER' and then register the handler.
Timer Handler
Timer Handler fires timer events. The nextTime method returns the next time to fire the timer event, and shouldFireTimerEvent method returns whether or not to fire the timer event.
The following handler fires the timer event at 500 ms interval unconditionally.
package com.tmax.pkg.handlerCase1; import java.util.logging.Logger; import com.tmax.anylink.api.serviceflow.ActivityContext; import com.tmax.anylink.api.serviceflow.DefaultUserTimer; public class TimerHandlerImpl extends DefaultUserTimer { private static Logger logger = Logger.getLogger(TimerHandlerImpl.class.getName()); private long startTime = -1; private final static long PERIOD = 20; @Override public long nextTime(ActivityContext arg0) { // TODO Auto-generated method stub if(startTime < 0) { startTime = System.currentTimeMillis() + PERIOD ; } else { startTime += PERIOD ; } logger.info("####### TimerHandlerImpl startTime : " + startTime); return startTime; } @Override public boolean shouldFireTimerEvent(ActivityContext arg0, long arg1) { // TODO Auto-generated method stub return true; } }
After implementing the handler, set the 'Timer Class Name' to the handler name in the [Timer] tab of the [Timer Event] > [Event Preference] page.
Message Validation Handler
Message Validation Handler performs message validation (including meta data).
The following handler checks the message’s Physical Name and returns false if it contains the string "ab".
package com.tmax.pkg.msgValidityCase1; import java.util.HashMap; import java.util.Map; import com.tmax.anylink.api.validation.DefaultMessageValidityHandler; import com.tmax.anylink.common.AnyLinkException; import com.tmaxsoft.promapper.structure.StructureFieldType; public class MsgValidityImpl extends DefaultMessageValidityHandler { @Override public Map<StructureFieldType, Boolean> messageMetaCheck( StructureFieldType[] arg0) throws AnyLinkException { // TODO Auto-generated method stub Map<StructureFieldType, Boolean> ret = new HashMap<StructureFieldType, Boolean>(); for(StructureFieldType type : arg0){ if(type.getPhysicalName().contains("ab")){ // invalid message field ret.put(type, true); // save the message field as'true' in the map }else{ // valid message field ret.put(type, false); // save the message field as'true' in the map } } return ret; } }
After implementing the handler, click [Upload Handler] from the context menu of the handler in the Navigator to upload the handler to DIS. In WebAdmin, enter the full package handler name in [Admin] > [DIS Configuration] > [User Class Setting] > [message-validity-class-name].
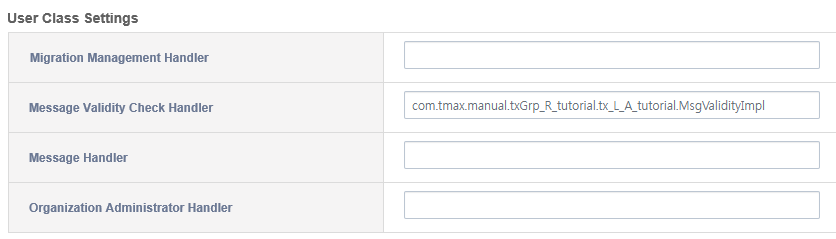
Click [Validate] from the Message Editor to validate the message.
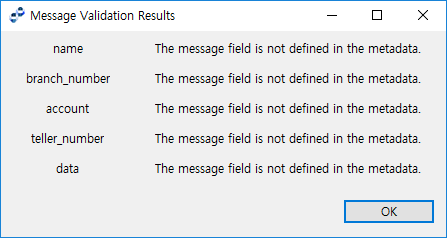
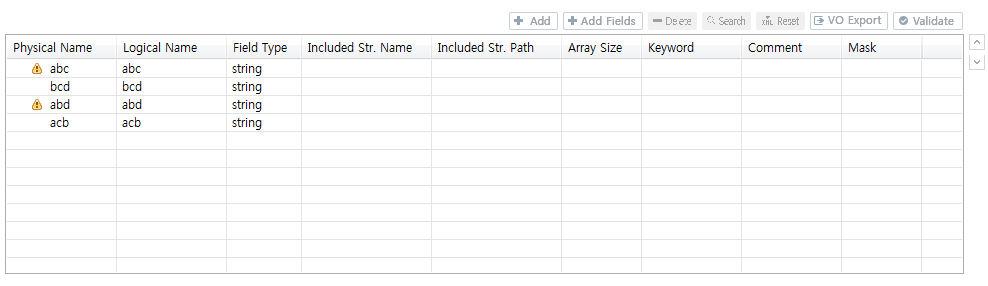
To use the message validation handler, the compiler version must match the Java version of the DIS server. ([Window] > [Preferences] > [Java] > [Compiler] > [Compiler compliance level] : 1.6) |
Routing Handler
Routing Handler is used for multi-binding. The route method is executed when multi-binding is called. A service that has the same value as the return value is called.
The following handler performs routing by using message contents as a value.
package com.tmax.pkg.handlerCase1; import com.tmax.anylink.api.MessageContext; import com.tmax.anylink.api.multibinding.DefaultRoutingHandler; import com.tmax.anylink.common.AnyLinkException; import com.tmax.anylink.logging.Logger; public class routing extends DefaultRoutingHandler { private static final Logger logger = Logger.getLogger(routing.class.getName()); @Override public String route(MessageContext arg0) throws AnyLinkException { return (String)new String((byte[])arg0.getContent()); } }
After implementing the handler, set the multi-binding binding option to 'HANDLER' and enter the handler to additional information of the setting.
SSO Login Handler
SSO Login Handler is used for SSO login. The login method, called when a user tries to log in, determines whether the login is successful or not by using the return value, SsoHandlerResult. The method has two parameters: user ID and user password.
The following handler outputs the ID and password of a user who tries to log in as log and makes the user succeed in login.
package com.tmax.pkg.handlerCase1; import com.tmax.anylink.api.SsoLogin.DefaultSsoLoginHandler; import com.tmax.anylink.api.SsoLogin.SsoHandlerResult; import com.tmax.anylink.api.SsoLogin.SsoHandlerResult.HandlerResultType; import com.tmax.anylink.common.AnyLinkException; import com.tmax.anylink.logging.Logger; public class ssologin extends DefaultSsoLoginHandler { private static final Logger logger = Logger.getLogger(ssologin.class.getName()); @Override public SsoHandlerResult login(String arg0, String arg1) throws AnyLinkException { logger.info("SSO ID : " + arg0 + ", PW : " + arg1); SsoHandlerResult result = new SsoHandlerResult(); result.setResult(HandlerResultType.SUCCESS); return result; } }
After implementing the handler, set the 'SSO Method' item to 'UserClass Method' in the WebAdmin page shown by selecting [Admin] > [DIS Configuration] and enter the handler to the SSO handler.
Monitoring Event Handler
Monitoring Event Handler is called when a monitoring event occurs. The event method, called when an event occurs, gets the event type with the first argument and monitoring event information with the second argument as a map. Information that can be obtained from the map can be checked in the 'Event Message' item in the WebAdmin page shown by selecting [Configuration] > [Event] > [Events].
The following handler outputs occurred event information as log.
package com.tmax.pkg.handlerCase1; import java.util.Map; import com.tmax.anylink.api.event.DefaultMonitoringEventHandler; import com.tmax.anylink.common.AnyLinkException; import com.tmax.anylink.logging.Logger; import com.tmaxsoft.schemas.anylink.MonitoringEventType; public class MonitoringEvent extends DefaultMonitoringEventHandler { private static final Logger logger = Logger.getLogger(MonitoringEvent.class.getName()); @Override public void event(MonitoringEventType arg0, Map<String, Object> arg1) throws AnyLinkException { logger.info(arg0.toString()); } }
After implementing the handler, deploy it and then search, configure, and save the handler in the 'Event Handler' item in the WebAdmin page shown by selecting [Configuration] > [Event] > [Events].
Message Management Handler
Message Management Handler is called by clicking [Send] in the WebAdmin page shown by selecting [Configuration] > [Message]. The callHandler method, called by clicking [Send], receives a checked message list as an argument and gets the message information.
The following handler outputs sysid of messages to send as log.
package com.tmax.pkg.handlerCase1; import java.util.List; import com.tmax.anylink.api.msgtransfer.DefaultMsgTransferHandler; import com.tmax.anylink.common.AnyLinkException; import com.tmax.anylink.dis.msg.ResourceInfoPK; import com.tmax.anylink.logging.Logger; public class MsgTransfer extends DefaultMsgTransferHandler { private static final Logger logger = Logger.getLogger(MsgTransfer.class.getName()); public String callHandler(List<ResourceInfoPK> arg0) throws AnyLinkException { for(int i = 0; i<arg0.size(); i ++) { ResourceInfoPK resource = arg0.get(i); logger.info("resource sysid = " + resource.getSysId()); } return null; } }
After implementing the handler, deploy it and then enter the handler class in the 'Message Handler' item in the WebAdmin page shown by selecting [Admin] > [DIS Configuration]. Select messages to send in the WebAdmin page shown by selecting [Configuration] > [Message] and then click [Send] to call the handler.
Administrator Handler
Administrator Handler is called by clicking [Send] in the WebAdmin page shown by selecting [Configuration] > [External Contact] > [Administrators]. The callHandler method, called by clicking [Send], gets a checked administrator list.
The following handler outputs selected administrators' names as log.
package com.tmax.pkg.handlerCase1; import java.util.List; import com.tmax.anylink.api.partneraddress.DefaultPartnerAddressHandler; import com.tmax.anylink.api.partneraddress.PartnerUser; import com.tmax.anylink.common.AnyLinkException; import com.tmax.anylink.logging.Logger; public class ParnerAddress extends DefaultPartnerAddressHandler { private static final Logger logger = Logger.getLogger(ParnerAddress.class.getName()); @Override public String callHandler(List<PartnerUser> partnertUserList) throws AnyLinkException { for(int i = 0; i<partnertUserList.size(); i++) { PartnerUser user = partnertUserList.get(i); logger.info("User Name = " + user.getUserName()); } return super.callHandler(partnertUserList); } }
After implementing the handler, deploy it and then enter the handler class in the 'Organization Administrator Handler' item in the WebAdmin page shown by selecting [Admin] > [DIS Configuration]. Select administrators to call in the WebAdmin page shown by selecting [Configuration] > [External Contact] > [Administrators] and then click [Send] to call the handler.