1. Web Service Ant Task
This section describes the Ant tasks provided by JEUS for creating Web services and for web service clients.
1.1. java2wsdl
The java2wsdl task creates the following from service endpoint interface classes (and classes implemented as Java).
-
WSDL file for web services
-
JAX-RPC mapping file
The class that defines the java2wsdl Ant task is jeus.util.ant.webservices.Java2WsdlTask. |
The following describes the java2wsdl properties.
Property | Description |
---|---|
configfilepath |
Path to the web service configuration file. (Required input, String type) |
classpath |
Path to Java web services classes. (Required input, String type) |
destDir |
Absolute path to the directory in which WSDL files are created. (String type) |
level |
Logging level. (String type) |
verbose |
Displays verbose messages. (Default value: false) |
Nested Element
<java2wsdl> contains the <classpath> element of Ant.
Example
The following is an example of a build.xml that executes java2wsdl.
-
build.xml example
java2wsdl Ant Task Build File Example: <build.xml><?xml version="1.0" encoding="UTF-8"?> <project name="java2wsdl" default="build" basedir="."> <property name="is.app-client.module" value="true" /> <import file="../../common/common-build.xml" /> <taskdef name="java2wsdl" classname="jeus.util.ant.webservices.Java2WsdlTask"> <classpath refid="jeus.libraries.classpath" /> </taskdef> <target name="-post-compile"> <java2wsdl destDir="${build.classes.dir}" verbose="true" configfilepath="${src.conf}/service-config.xml"> <classpath refid="classpath" /> </java2wsdl> </target> </project>
-
Execution example
$ jant ... [java2wsdl] Building Web Services : DocLitEchoService [java2wsdl] Generating WSDL File - jeus/build/classes/DocLitEchoService.wsdl [java2wsdl] Generating JAX-RPC Mapping File - jeus/build/classes/DocLitEchoService-mapping.xml ... BUILD SUCCESSFUL Total time: 11 seconds
1.2. wsdl2java
The wsdl2java task creates one of the following WSDL of a web service.
-
Java program stubs for web service clients
-
Java program interfaces for web service servers
The class that defines the wsdl2java Ant task is jeus.util.ant.webservices.Wsdl2JavaTask. |
The following describes the wsdl2java properties.
Property | Description |
---|---|
wsdl |
Absolute path or URL to the WSDL file used to create Java source files. (Required option, String type) |
mode |
Mode used to create Java source files. (Required option, String type) Input options:
|
destDir |
Absolute path to the directory in which Java files are created. (Required input, String type) |
classDestDir |
Directory in which compiled class files are created. (String type) |
inputMapping |
Input JAX-RPC mapping file used to create Java classes. (String type) |
package |
Java package name for all namespace URIs in WSDL. (String type) (String type) |
outputMapping |
Output JAX-RPC mapping file for input WSDL. (String type) |
doCompile |
Option to compile the created Java source files. (Default value: true) |
username |
Username required to access the URL of WSDL. (String type) |
password |
Password required to access the URL of WSDL. (String type) |
keepSrc |
Saves created Java source files. (Default value: true) |
nowrapped |
Option to disable the wrapped mode detection for WSDL. (Default value: false) |
dataHandlerOnly |
Option to apply jakarta.activation.DataHandler to the MIME type. (Default value: false) |
nodatabinding |
Option to apply jakarta.xml.soap.SOAPElement to all WSDL message parts. (Default value: false) |
soapver |
SOAP version used in stub/tie classes.
|
resolveDir |
Local storage for remote WSDL files. (String type) |
DDGen |
Option to create a basic deployment descriptor for JEUS (webservices.xml file, web.xml when MODE is web, and ejb-jar.xml file when MODE is ejb). (String type) |
level |
Logging level. (String type) |
verbose |
Displays verbose messages. (Default value: false) |
Nested Element
<wsdl2java> has nested Ant <classpath> and <mapping> elements. The structure of a <wsdl2java> Ant Task is as follows (+ means it can have more than one element):
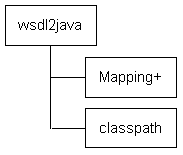
<mapping>
Mapping is performed between a Java package and a WSDL namespace. If the <mapping> element is omitted, all namespace URIs are mapped to the package specified by the package property of the <wsdl2java> element.
The following describes the <mapping> properties.
Property | Description |
---|---|
package |
Java package name. (Required option, String type) |
namespace |
Namespace URI of WSDL. (Required option, String type) |
If the package property is specified in the <wsdl2java> element, this value has a higher priority than the <mapping> element value. |
Example
The following is an example of a build.xml that executes wsdl2java.
-
build.xml example
wsdl2java Ant Task Build File Example: <build.xml><?xml version="1.0" encoding="UTF-8"?> <project name="wsdl2java" default="build" basedir="."> <property name="is.app-client.module" value="true" /> <import file="../../../common/common-build.xml" /> <taskdef name="wsdl2java" classname="jeus.util.ant.webservices.Wsdl2JavaTask"> <classpath refid="jeus.libraries.classpath" /> </taskdef> <target name="-pre-compile"> <mkdir dir="${build.classes.dir}" /> <wsdl2java destDir="${build.classes.dir}" verbose="true" mode="import:server" doCompile="true" noDataBinding="true" package="sample.nodatabinding.service" outputmapping="${build.classes.dir}/BookQuoteService-mapping.xml" wsdl="${src.conf}/BookQuoteService.wsdl"> <classpath refid="classpath" /> </wsdl2java> </target> </project>
-
Execution example
$ jant Buildfile: build.xml ... [wsdl2java] Compiling generated sources(1)... ... BUILD SUCCESSFUL Total time: 6 seconds
1.3. wsgen
The wsgen task creates the following from service endpoint interface classes and classes implemented in Java.
-
Portable Artifacts
-
Web service WSDL file (option)
The class that defines the wsgen Ant task is jeus.webservices.jaxws.tools.WsGen2. |
The following describes the wsgen properties.
Property | Description |
---|---|
sei |
Name of the service endpoint interface class. (Required input, String type) |
destdir |
Absolute path to the directory in which class files are created. (Required input, String type) |
classpath |
Directory of input class files. (String type) |
cp |
Alias for the classpath property. (String type) |
resourcedestdir |
Used with the genwsdl property. This property configures the location in which WSDL files are created. (String type) |
sourcedestdir |
Directory in which source files are created. (String type) |
keep |
Saves created files. (Default value: false) |
verbose |
Displays verbose messages. (Default value: false) |
genwsdl |
Option to create WSDL files. (Default value: false) |
protocol |
Used with the genwsdl property. Protocol to be used in the wsdl:binding element. The default value is soap1.1. Xsoap1.2 values can be used with the property extension. (String type) |
servicename |
Used with the genwsdl property. Name of a specific wsdl:service element of the created WSDL file. (String type) |
portname |
Used with the genwsdl property. Name of a specific wsdl:portname element of the created WSDL file. (String type) |
extension |
Allows vendor extensions. This option may cause compatibility and portability issues. (Boolean type) |
policy |
Reads the web service policy configuration file. (String type) |
Example
The following is an example of a build.xml that executes wsgen.
-
build.xml example
wsgen Ant Task Build File Example: <build.xml><?xml version="1.0" encoding="UTF-8"?> <project name="wsgen" default="build" basedir="."> <property name="is.app-client.module" value="true" /> <import file="../../common/common-build.xml" /> <taskdef name="wsgen" classname="com.sun.tools.ws.ant.WsGen"> <classpath refid="jeus.libraries.classpath" /> </taskdef> <target name="-post-compile"> <wsgen sei="fromjava.server.AddNumbersImpl" destdir="${build.classes.dir}" classpath="${build.classes.dir}" resourcedestdir="${build.classes.dir}" sourcedestdir="${build.classes.dir}" genwsdl="true" /> </target> </project>
-
Execution example
$ jant Buildfile: build.xml ... [echo] Compiling wsgen... ... BUILD SUCCESSFUL Total time: 6 seconds
1.4. wsimport
The wsimport task creates one of the following from the web service WSDL.
-
Java program stubs for web service clients
-
Java program interfaces for web service servers
The class that defines the wsimport Ant task is jeus.webservices.jaxws.tools.WsImport2. |
The following describes the wsimport properties.
Property | Description |
---|---|
wsdl |
Absolute path or URL to the WSDL file used to create Java source files. (Required option, String type) |
destDir |
Absolute path to the directory in which Java files are created. (String type) |
sourcedestdir |
Directory in which source files are created. If this property is set, the keep property is automatically set. (String type) |
keep |
Saves created files. (Default value: false) |
verbose |
Displays verbose messages. (Default value: false) |
binding |
External JAS-WS or JAXB binding files. (String type) |
extension |
Allows vendor extensions. This option may cause compatibility and portability issues. (Boolean type) |
wsdllocation |
If WSDL URI is specified, the URI value is set to the service endpoint interface and the @WebService.wsdlLocation Annotation and @WebServiceClient.wsdlLocation annotations of the service interface. (String type) |
catalog |
External entity reference values like those in TR9401, XCatalog, and OASIS XML Catalog. The Ant xmlcatalog type can also be used. (String type) |
package |
Java package name for all namespace URIs in WSDL. (String type) (String type) |
target |
Creates code according to the specified version of JAX-WS specifications. (String type) |
quiet |
Hides output messages. (Default value: false) |
policy |
Reads the web service policy configuration file. (String type) |
Example
The following is an example of a build.xml that executes wsimport.
-
build.xml example
wsimport Ant Task Build File Example: <build.xml><?xml version="1.0" encoding="UTF-8"?> <project name="wsimport" default="build" basedir="."> <property name="is.app-client.module" value="true" /> <import file="../../common/common-build.xml" /> <taskdef name="wsimport" classname="com.sun.tools.ws.ant.WsImport"> <classpath refid="jeus.libraries.classpath" /> </taskdef> <target name="-pre-compile"> <mkdir dir="${build.classes.dir}" /> <wsimport wsdl="${src.conf}/AddNumbers.wsdl" destDir="${build.classes.dir}" sourcedestdir="${build.classes.dir}" package="fromwsdl.server" /> </target> </project>
-
Execution example
$ jant Buildfile: build.xml ... [wsimport] Consider using <depends>/<produces> so that wsimport won't do unnecessary compilation [wsimport] parsing WSDL... [wsimport] [wsimport] [wsimport] generating code... ... BUILD SUCCESSFUL Total time: 6 seconds
1.5. xjc
The xjc task converts XML schema files to JAXB content classes in Java language.
The following describes the xjc properties.
Property | Description |
---|---|
wsdl |
Schema files to be compiled. (Required option, String type) |
binding |
Externally added binding files to be applied to the schema files. (String type) |
package |
Java package below which source code is created. This option has the same effect as the -p option for a command line. (String type) |
destdir |
Directory in which source code is created. (Required option, String type) |
readonly |
Option to set Java source files to readonly mode. (Default value: false) |
extension |
Option to execute the XJC binding compiler in extension mode. (Default value: false) |
catalog |
External entity reference values like those in TR9401, XCatalog, and OASIS XML Catalog. (String type) |
removeOldOutput |
Option to delete all files specified by the produces element before the XJC binding compiler recompiles the source files. (String type, "yes"/"no") |
source |
Schema version of the compiler to be used. (String type, 1.0/2.0) |
Example
The following is an example of a build.xml that executes xjc.
-
build.xml example
xjc Ant Task Build File Example: <build.xml><?xml version="1.0" encoding="UTF-8"?> <project name="xjc" default="build" basedir="."> <property name="is.app-client.module" value="true" /> <import file="../../common/common-build.xml" /> <taskdef name="xjc" classname="com.sun.tools.xjc.XJCTask"> <classpath refid="jeus.libraries.classpath" /> </taskdef> <target name="-pre-compile"> <mkdir dir="${build.classes.dir}" /> <xjc schema="${src.conf}/ts.xsd" package="com.tmaxsoft" destdir="${build.classes.dir}"> <produces dir="${build.classes.dir}/com/tmaxsoft" includes="**/*.java" /> <classpath refid="jeus.libraries.classpath" /> <classpath refid="classpath" /> </xjc> </target> </project>
-
Execution example
$ jant Buildfile: build.xml ... [xjc] jeus/build/classes/com/tmaxsoft is not found and thus excluded from the dependency check [xjc] Compiling file:/jeus/src/conf/ts.xsd [xjc] Writing output to jeus/build/classes ... BUILD SUCCESSFUL Total time: 4 seconds
1.6. schemagen
The schemagen task creates a single schema file for each individual namespace of a Java class.
The following describes the schemagen properties.
Property | Description |
---|---|
destdir |
Directory in which schema files are created. (String type) |
classpath |
Classpath. (String type) |
Example
The following is an example of a build.xml that executes schemagen.
-
build.xml example
schemagen Ant Task Build File Example: <build.xml><?xml version="1.0" encoding="UTF-8"?> <project name="schemagen" default="build" basedir="."> <property name="is.app-client.module" value="true" /> <import file="../../common/common-build.xml" /> <taskdef name="schemagen" classname="com.sun.tools.jxc.SchemaGenTask"> <classpath refid="jeus.libraries.classpath" /> </taskdef> <target name="-pre-compile"> <mkdir dir="${build.classes.dir}" /> <schemagen destdir="${build.classes.dir}"> <src path="${src.dir}" /> <classpath refid="jeus.libraries.classpath" /> <classpath refid="classpath" /> </schemagen> </target> </project>
-
Execution example
$ jant Buildfile: build.xml ... [schemagen] Generating schema from 2 source files [schemagen] Note: Writing jeus/schema1.xsd ... BUILD SUCCESSFUL Total time: 5 seconds